How to Fix Google OAuth Error 403 User Agent Issue on iPhone?
Introduction When integrating Google OAuth for authentication in your application, you might encounter various errors. One common issue is the ERROR 403: Google disallowed user agent, especially if your code works perfectly on Android but fails on iPhone. This error message usually indicates that Google is rejecting the request due to the user agent string that your app is sending. Let's explore why this happens and how to fix it. Understanding the Issue The ERROR 403: Google disallowed user agent typically occurs when Google’s servers identify an untrusted or unsupported user agent accessing their OAuth service. User agents represent the application type, operating system, vendor, and version of the browser, and if the string does not match Google's expectations or security policies, the API rejects it. Causes of the 403 Error Unsupported User Agents: Certain user agents are flagged by Google as insecure or not allowed to access its services. For example, mobile web views or certain in-app browsers can trigger this error. Invalid Redirect URI: The redirect URI used in the OAuth process must match the ones registered in the Google Developer Console. CORS Issues: Cross-Origin Resource Sharing (CORS) can also be a factor affecting the request, especially if the request is coming from a non-browser environment. Step-by-Step Solution to Resolve the 403 Error Here’s how to address the issue and get your Google OAuth authentication working smoothly on iPhones: Step 1: Use a Native Browser Instead of opening the OAuth authorization URL in an in-app web view, try launching it in the device's native browser. This helps in preventing the disallowed user agent issue. Here’s how you can modify your JavaScript code: var openAuthWindow = function(){ var urlAuth = "https://accounts.google.com/o/oauth2/auth?" + "scope=https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile&" + "redirect_uri=http://localhost&" + "response_type=code&" + "client_id=" + clientId; // Instead of InAppBrowser, open in default browser window.open(urlAuth, '_system', 'location=no'); }; Step 2: Check Client Configuration Make sure your client_id is correctly set up in the Google Developer Console. Ensure that the redirect_uri matches exactly with the URLs specified in your OAuth client configuration. Note that the localhost URL is often not valid in production or mobile contexts, so consider using a valid redirect URI. Step 3: Adjust Allowed Redirect URIs In your Google API Console settings, ensure that you have specified proper redirect URIs. For mobile applications, you might want to use: https://yourapp.com/auth or any valid URL that can handle the redirect. Step 4: Implement Error Handling Implement error handling in your authentication call to help diagnose issues better. Here’s an enhanced version of your function with error handling: var openAuthWindow = function() { var urlAuth = "https://accounts.google.com/o/oauth2/auth?" + "scope=https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile&" + "redirect_uri=http://localhost&" + "response_type=code&" + "client_id=" + clientId; // Open in the system browser const authWindow = window.open(urlAuth, '_system', 'location=no'); // Add error handling authWindow.addEventListener('exit', function() { console.warn('Authentication window closed unexpectedly.'); }); }; Step 5: Testing and Validation After making the changes, test the implemented solution on an iPhone. Monitor and log responses to ensure that the authentication is successful. If you're still facing issues, check the console logs for additional error messages that could provide further insights. Frequently Asked Questions What is a user agent? A user agent is a string that identifies the web browser and the operating system to the web server. It helps the server determine how to respond to requests. Why does the 403 error occur only on iPhones? iPhone browsers and in-app web views may have different user agent strings that Google’s OAuth service doesn't recognize as valid. Hence, the disallowed user agent error appears. Can I use localhost for mobile OAuth redirects? Localhost is generally not recommended for mobile applications, unless you are doing local testing. It’s better to use a live URL that you control. Conclusion Resolving the ERROR 403: Google disallowed user agent issue requires adjustments in how you handle OAuth authentication across platforms. By opening the authentication URL in the device's native browser and ensuring proper client configuration, you can allow users on iPhone devices to authenticate seamlessly. By following the steps outlined and implementing robust error handling, you can enhance the OAuth experience and avoid common pitfalls.
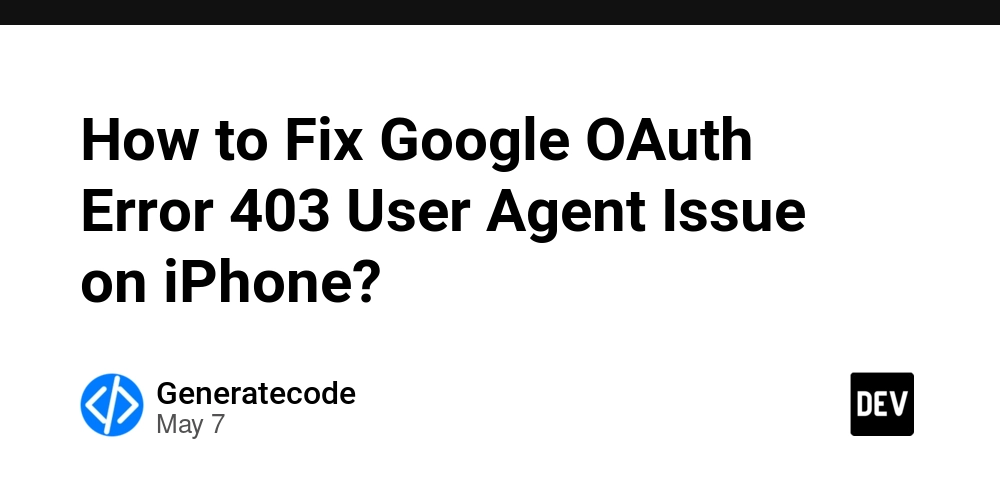
Introduction
When integrating Google OAuth for authentication in your application, you might encounter various errors. One common issue is the ERROR 403: Google disallowed user agent, especially if your code works perfectly on Android but fails on iPhone. This error message usually indicates that Google is rejecting the request due to the user agent string that your app is sending. Let's explore why this happens and how to fix it.
Understanding the Issue
The ERROR 403: Google disallowed user agent typically occurs when Google’s servers identify an untrusted or unsupported user agent accessing their OAuth service. User agents represent the application type, operating system, vendor, and version of the browser, and if the string does not match Google's expectations or security policies, the API rejects it.
Causes of the 403 Error
- Unsupported User Agents: Certain user agents are flagged by Google as insecure or not allowed to access its services. For example, mobile web views or certain in-app browsers can trigger this error.
- Invalid Redirect URI: The redirect URI used in the OAuth process must match the ones registered in the Google Developer Console.
- CORS Issues: Cross-Origin Resource Sharing (CORS) can also be a factor affecting the request, especially if the request is coming from a non-browser environment.
Step-by-Step Solution to Resolve the 403 Error
Here’s how to address the issue and get your Google OAuth authentication working smoothly on iPhones:
Step 1: Use a Native Browser
Instead of opening the OAuth authorization URL in an in-app web view, try launching it in the device's native browser. This helps in preventing the disallowed user agent issue. Here’s how you can modify your JavaScript code:
var openAuthWindow = function(){
var urlAuth = "https://accounts.google.com/o/oauth2/auth?"
+ "scope=https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile&"
+ "redirect_uri=http://localhost&"
+ "response_type=code&"
+ "client_id=" + clientId;
// Instead of InAppBrowser, open in default browser
window.open(urlAuth, '_system', 'location=no');
};
Step 2: Check Client Configuration
Make sure your client_id is correctly set up in the Google Developer Console. Ensure that the redirect_uri matches exactly with the URLs specified in your OAuth client configuration. Note that the localhost URL is often not valid in production or mobile contexts, so consider using a valid redirect URI.
Step 3: Adjust Allowed Redirect URIs
In your Google API Console settings, ensure that you have specified proper redirect URIs. For mobile applications, you might want to use:
https://yourapp.com/auth
- or any valid URL that can handle the redirect.
Step 4: Implement Error Handling
Implement error handling in your authentication call to help diagnose issues better. Here’s an enhanced version of your function with error handling:
var openAuthWindow = function() {
var urlAuth = "https://accounts.google.com/o/oauth2/auth?"
+ "scope=https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile&"
+ "redirect_uri=http://localhost&"
+ "response_type=code&"
+ "client_id=" + clientId;
// Open in the system browser
const authWindow = window.open(urlAuth, '_system', 'location=no');
// Add error handling
authWindow.addEventListener('exit', function() {
console.warn('Authentication window closed unexpectedly.');
});
};
Step 5: Testing and Validation
After making the changes, test the implemented solution on an iPhone. Monitor and log responses to ensure that the authentication is successful. If you're still facing issues, check the console logs for additional error messages that could provide further insights.
Frequently Asked Questions
What is a user agent?
A user agent is a string that identifies the web browser and the operating system to the web server. It helps the server determine how to respond to requests.
Why does the 403 error occur only on iPhones?
iPhone browsers and in-app web views may have different user agent strings that Google’s OAuth service doesn't recognize as valid. Hence, the disallowed user agent error appears.
Can I use localhost for mobile OAuth redirects?
Localhost is generally not recommended for mobile applications, unless you are doing local testing. It’s better to use a live URL that you control.
Conclusion
Resolving the ERROR 403: Google disallowed user agent issue requires adjustments in how you handle OAuth authentication across platforms. By opening the authentication URL in the device's native browser and ensuring proper client configuration, you can allow users on iPhone devices to authenticate seamlessly. By following the steps outlined and implementing robust error handling, you can enhance the OAuth experience and avoid common pitfalls.