HarmonyOS NEXT Practical: Logging Tool
Goal: Encapsulate the logging tool to make it simple and usable. During the application development process, log information can be output at critical code points. After running the application, analyze the execution status of the application by checking the log information (such as whether the application is running normally, the timing of code execution, whether the logical branches are running normally, etc.). The system provides different APIs for developers to call and output log information, namely HiLog and console. The two APIs have slight differences in usage. This article focuses on the usage of HiLog, and the specific usage of console can be found in the API reference console. HiLog defines five log levels: DEBUG, INFO, Warning, ERROR, and FATAL, and provides corresponding methods to output logs of different levels. Parameter parsing Domain: Used to specify the business domain corresponding to the output log, with a value range of 0x0000~0xFFFF. Developers can customize it according to their needs. Tag: Used to specify the log identifier, which can be any string. It is recommended to identify the class or business behavior where the call is made. The maximum size of a tag is 31 bytes, and it will be truncated if exceeded. It is not recommended to use Chinese characters as they may cause garbled characters or alignment issues. Level: Used to specify the log level. The value can be found in LogLevel. Format: a format string used for formatting output of logs. The formatting parameters for log printing should be printed in the format of 'private flag specification'. describe -The domain and tag used for isLoggable() and the specific log printing interface should be consistent. -The level used by isLoggable() should be consistent with the specific log printing interface level. Constraints and limitations: The maximum print size for logs is 4096 bytes. Text exceeding this limit will be truncated. Actual combat: import { hilog } from '@kit.PerformanceAnalysisKit'; /** * 日志级别 */ export enum LogLevel { debug = "debug", info = "info", warn = 'warn', error = "error" } /** * 日志工具 * ###待考虑:设置打印日志级别 */ export class LoggerKit { private domain: number; private prefix: string; private enableLogLevels: LogLevel[]; /** * 日志记录器工具 * @param domain 域,默认:0xABCD * @param prefix 前缀,默认:LoggerKit * @param enableLogLevels 启用日志级别,默认:全部启用 */ constructor(domain: number = 0xABCD, prefix: string = 'LoggerKit', enableLogLevels = [LogLevel.debug, LogLevel.info, LogLevel.warn, LogLevel.error]) { this.domain = domain; this.prefix = prefix; this.enableLogLevels = enableLogLevels; } debug(...args: string[]) { if (this.enableLogLevels.includes(LogLevel.debug)) { hilog.debug(this.domain, this.prefix, getFormat(args), args); } } info(...args: string[]) { if (this.enableLogLevels.includes(LogLevel.info)) { hilog.info(this.domain, this.prefix, getFormat(args), args); } } warn(...args: string[]) { if (this.enableLogLevels.includes(LogLevel.warn)) { hilog.warn(this.domain, this.prefix, getFormat(args), args); } } error(...args: string[]) { if (this.enableLogLevels.includes(LogLevel.error)) { hilog.error(this.domain, this.prefix, getFormat(args), args); } } static LK_domain: number = 0xABCD; static LK_prefix: string = 'LoggerKit'; static LK_enableLogLevels: LogLevel[] = [LogLevel.debug, LogLevel.info, LogLevel.warn, LogLevel.error]; static debug(...args: string[]) { if (LoggerKit.LK_enableLogLevels.includes(LogLevel.debug)) { hilog.debug(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args); } } static info(...args: string[]) { if (LoggerKit.LK_enableLogLevels.includes(LogLevel.info)) { hilog.info(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args); } } static warn(...args: string[]) { if (LoggerKit.LK_enableLogLevels.includes(LogLevel.warn)) { hilog.warn(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args); } } static error(...args: string[]) { if (LoggerKit.LK_enableLogLevels.includes(LogLevel.error)) { hilog.error(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args); } } } function getFormat(args: string[]) { let format = '' for (let i = 0; i < args.length; i++) { if (i == 0) { format = '%{public}s' } else { format += ', %{public}s' } } return format }
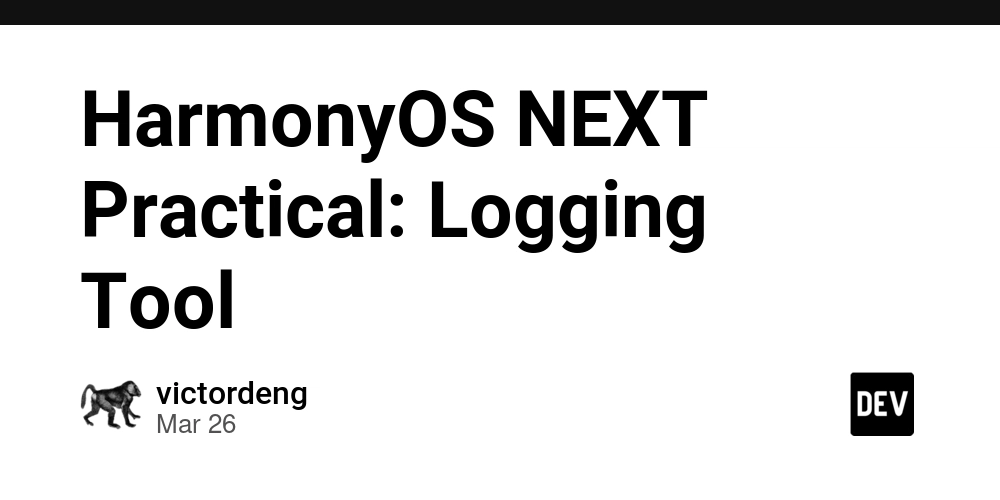
Goal: Encapsulate the logging tool to make it simple and usable.
During the application development process, log information can be output at critical code points. After running the application, analyze the execution status of the application by checking the log information (such as whether the application is running normally, the timing of code execution, whether the logical branches are running normally, etc.).
The system provides different APIs for developers to call and output log information, namely HiLog and console. The two APIs have slight differences in usage. This article focuses on the usage of HiLog, and the specific usage of console can be found in the API reference console.
HiLog defines five log levels: DEBUG, INFO, Warning, ERROR, and FATAL, and provides corresponding methods to output logs of different levels.
Parameter parsing
- Domain: Used to specify the business domain corresponding to the output log, with a value range of 0x0000~0xFFFF. Developers can customize it according to their needs.
- Tag: Used to specify the log identifier, which can be any string. It is recommended to identify the class or business behavior where the call is made. The maximum size of a tag is 31 bytes, and it will be truncated if exceeded. It is not recommended to use Chinese characters as they may cause garbled characters or alignment issues.
- Level: Used to specify the log level. The value can be found in LogLevel.
- Format: a format string used for formatting output of logs. The formatting parameters for log printing should be printed in the format of 'private flag specification'.
describe
-The domain and tag used for isLoggable() and the specific log printing interface should be consistent.
-The level used by isLoggable() should be consistent with the specific log printing interface level.
Constraints and limitations: The maximum print size for logs is 4096 bytes. Text exceeding this limit will be truncated.
Actual combat:
import { hilog } from '@kit.PerformanceAnalysisKit';
/**
* 日志级别
*/
export enum LogLevel {
debug = "debug",
info = "info",
warn = 'warn',
error = "error"
}
/**
* 日志工具
* ###待考虑:设置打印日志级别
*/
export class LoggerKit {
private domain: number;
private prefix: string;
private enableLogLevels: LogLevel[];
/**
* 日志记录器工具
* @param domain 域,默认:0xABCD
* @param prefix 前缀,默认:LoggerKit
* @param enableLogLevels 启用日志级别,默认:全部启用
*/
constructor(domain: number = 0xABCD, prefix: string = 'LoggerKit',
enableLogLevels = [LogLevel.debug, LogLevel.info, LogLevel.warn, LogLevel.error]) {
this.domain = domain;
this.prefix = prefix;
this.enableLogLevels = enableLogLevels;
}
debug(...args: string[]) {
if (this.enableLogLevels.includes(LogLevel.debug)) {
hilog.debug(this.domain, this.prefix, getFormat(args), args);
}
}
info(...args: string[]) {
if (this.enableLogLevels.includes(LogLevel.info)) {
hilog.info(this.domain, this.prefix, getFormat(args), args);
}
}
warn(...args: string[]) {
if (this.enableLogLevels.includes(LogLevel.warn)) {
hilog.warn(this.domain, this.prefix, getFormat(args), args);
}
}
error(...args: string[]) {
if (this.enableLogLevels.includes(LogLevel.error)) {
hilog.error(this.domain, this.prefix, getFormat(args), args);
}
}
static LK_domain: number = 0xABCD;
static LK_prefix: string = 'LoggerKit';
static LK_enableLogLevels: LogLevel[] = [LogLevel.debug, LogLevel.info, LogLevel.warn, LogLevel.error];
static debug(...args: string[]) {
if (LoggerKit.LK_enableLogLevels.includes(LogLevel.debug)) {
hilog.debug(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args);
}
}
static info(...args: string[]) {
if (LoggerKit.LK_enableLogLevels.includes(LogLevel.info)) {
hilog.info(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args);
}
}
static warn(...args: string[]) {
if (LoggerKit.LK_enableLogLevels.includes(LogLevel.warn)) {
hilog.warn(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args);
}
}
static error(...args: string[]) {
if (LoggerKit.LK_enableLogLevels.includes(LogLevel.error)) {
hilog.error(LoggerKit.LK_domain, LoggerKit.LK_prefix, getFormat(args), args);
}
}
}
function getFormat(args: string[]) {
let format = ''
for (let i = 0; i < args.length; i++) {
if (i == 0) {
format = '%{public}s'
} else {
format += ', %{public}s'
}
}
return format
}