Clean Code Naming: A Senior Developer’s Code Review Checklist
Getting the names in your code right is crucial — it makes your code easier to read, maintain, and debug. But honestly, it’s not always obvious what makes a name good or bad, especially when you’re reviewing someone else’s code. In this post, I’ll share my mental checklist for reviewing names during code reviews. It’s based on principles from Clean Code book, but expressed in a straightforward, practical way so you can start applying it today. 1. Does the name clearly tell you what it’s for? The name should make the purpose of the variable, function, or class obvious. It should not require you to dig through the code or run around to figure it out. Be descriptive to provide enough context if it helps clarify the intent. Avoid vague or overly broad names. Examples: 1.1 Vague name // Problematic List data; // what kind of data? Better: Clear purpose List userEmails; // clearer purpose 1.2 Names missing key info void process(String input); // process what? how? Fix: void validateEmailFormat(String email); // clear purpose 2. Does the name match the actual meaning in the domain? Use terms that make sense in the real world or in your tech stack. Mixing up terms can make the code confusing: developers might misinterpret what a variable or method actually does. Examples: 2.1 Using a term incorrectly class CacheKeyMapper { // 'mapper' sounds like converting objects String generateKey(String cacheValue); } Correct: Use "generator" when you’re creating something class CacheKeyGenerator { String generateKey(String cacheValue); } 2.2 Use of vague term class UserAccountDetails { // Sounds like this holds user info private String username; private String password; private String email; } Correct: actually, it only deals with login info. class UserCredentials { private String username; private String password; private String email; } 3. Is the name helping distinguish similar things? If you have multiple similar methods or classes, give them clear, specific names so you know exactly what each one does. Also, stick to consistent patterns — it reduces mental overhead. Examples: 3.1 No real difference class OrderService { // Unclear distinctions Order getOrder(String id); Order fetchOrder(String id); Order retrieveOrder(String id); } Better: Specific roles class OrderService { // Clear distinctions Order getOrderById(String id); Order getOrderByReference(String ref); List getOrdersByCustomer(String customerId); } 3.2 Keep your method names uniform class Account { float getBalance(); } class CustomerInfo { String fetchShippingAddress(); } Instead of mixing get and fetch, pick one: class Account { float getBalance(); } class CustomerInfo { String getShippingAddress(); } Bonus tip: take inspiration from open-source projects Open-source projects like Guava and Apache Commons provide excellent examples of effective naming. Aligning your naming conventions with theirs is always a good idea. Wrap-up: Make it practical Reviewing names isn’t about nitpicking. It’s about making your code understandable for others and for future you. Take a moment to ask: Does this name tell me what it’s for? Does it match the real-world or technical terms I understand? Is it distinct enough from similar names? Is it consistent with the rest of the code? Good names save time and reduce bugs in both immediate development and long-term maintenance. Clean code starts with clear naming.
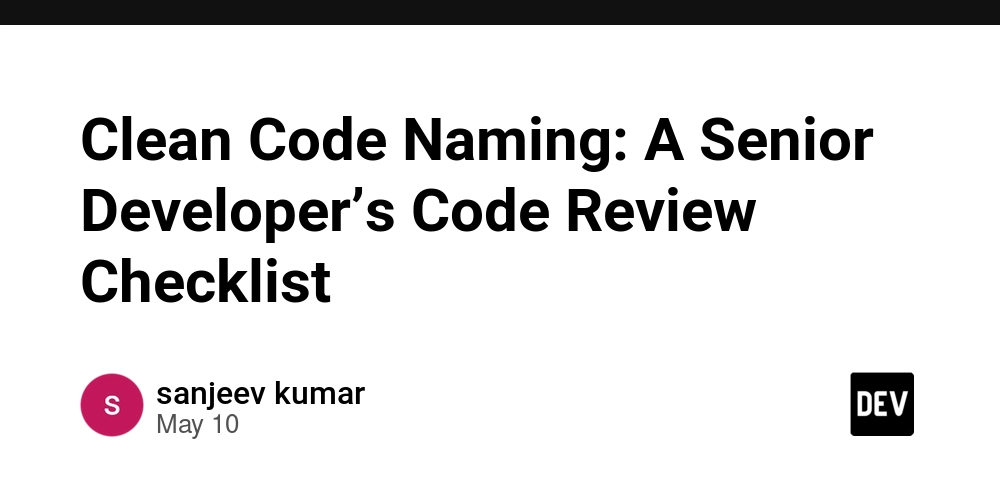
Getting the names in your code right is crucial — it makes your code easier to read, maintain, and debug. But honestly, it’s not always obvious what makes a name good or bad, especially when you’re reviewing someone else’s code.
In this post, I’ll share my mental checklist for reviewing names during code reviews. It’s based on principles from Clean Code book, but expressed in a straightforward, practical way so you can start applying it today.
1. Does the name clearly tell you what it’s for?
The name should make the purpose of the variable, function, or class obvious. It should not require you to dig through the code or run around to figure it out. Be descriptive to provide enough context if it helps clarify the intent. Avoid vague or overly broad names.
Examples:
1.1 Vague name
// Problematic
List<String> data; // what kind of data?
Better: Clear purpose
List<String> userEmails; // clearer purpose
1.2 Names missing key info
void process(String input); // process what? how?
Fix:
void validateEmailFormat(String email); // clear purpose
2. Does the name match the actual meaning in the domain?
Use terms that make sense in the real world or in your tech stack. Mixing up terms can make the code confusing: developers might misinterpret what a variable or method actually does.
Examples:
2.1 Using a term incorrectly
class CacheKeyMapper {
// 'mapper' sounds like converting objects
String generateKey(String cacheValue);
}
Correct: Use "generator" when you’re creating something
class CacheKeyGenerator {
String generateKey(String cacheValue);
}
2.2 Use of vague term
class UserAccountDetails {
// Sounds like this holds user info
private String username;
private String password;
private String email;
}
Correct: actually, it only deals with login info.
class UserCredentials {
private String username;
private String password;
private String email;
}
3. Is the name helping distinguish similar things?
If you have multiple similar methods or classes, give them clear, specific names so you know exactly what each one does. Also, stick to consistent patterns — it reduces mental overhead.
Examples:
3.1 No real difference
class OrderService {
// Unclear distinctions
Order getOrder(String id);
Order fetchOrder(String id);
Order retrieveOrder(String id);
}
Better: Specific roles
class OrderService {
// Clear distinctions
Order getOrderById(String id);
Order getOrderByReference(String ref);
List<Order> getOrdersByCustomer(String customerId);
}
3.2 Keep your method names uniform
class Account {
float getBalance();
}
class CustomerInfo {
String fetchShippingAddress();
}
Instead of mixing get and fetch, pick one:
class Account {
float getBalance();
}
class CustomerInfo {
String getShippingAddress();
}
Bonus tip: take inspiration from open-source projects
Open-source projects like Guava and Apache Commons provide excellent examples of effective naming. Aligning your naming conventions with theirs is always a good idea.
Wrap-up: Make it practical
Reviewing names isn’t about nitpicking. It’s about making your code understandable for others and for future you. Take a moment to ask:
- Does this name tell me what it’s for?
- Does it match the real-world or technical terms I understand?
- Is it distinct enough from similar names?
- Is it consistent with the rest of the code?
Good names save time and reduce bugs in both immediate development and long-term maintenance. Clean code starts with clear naming.