All the special files in NextJS
The new Next.js app router system gives a handy way to manage routing in an application. NextJS has some conventional or special files that serve some useful purposes. Let's deep dive into learning those files. Just to mention that Next.js supports 2 types of extensions on files. One is tsx for TypeScript app builders, and another is for .js for vanilla JavaScript builders. In this blog, I will only mention the .tsx extension, but you can definitely use the .js extension if you don't want to use TypeScript. page.tsx This is the special file that tells the compiler that 'This file should render a route.' The folder name automatically becomes a route and the page.tsx file returns a React element that tells what to render on that particular route. app/ about/ page.tsx { return
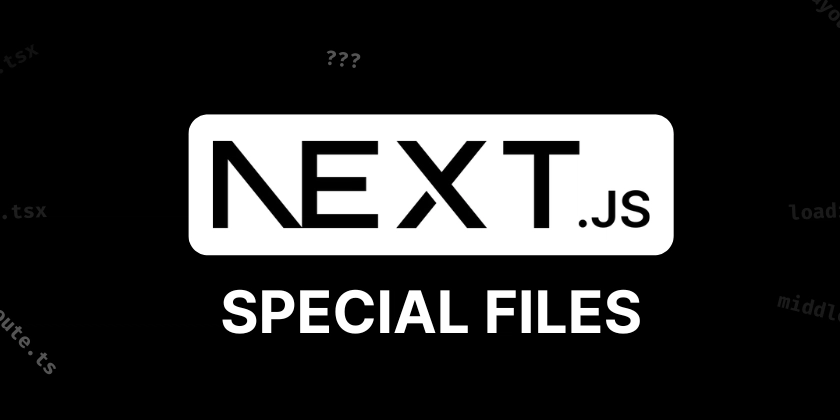
The new Next.js app router system gives a handy way to manage routing in an application. NextJS has some conventional or special files that serve some useful purposes. Let's deep dive into learning those files.
Just to mention that Next.js supports 2 types of extensions on files. One is tsx
for TypeScript app builders, and another is for .js
for vanilla JavaScript builders. In this blog, I will only mention the .tsx
extension, but you can definitely use the .js
extension if you don't want to use TypeScript.
page.tsx
This is the special file that tells the compiler that 'This file should render a route.' The folder name automatically becomes a route and the page.tsx file returns a React element that tells what to render on that particular route.
app/
about/
page.tsx <-- This is the route for /about
contact/
page.tsx <-- This is the route for /contact
Example Code:
// app/about/page.tsx
const About = () => {
return (
<div>About page</div>
);
};
export default About;
layout.tsx
This special file plays a very powerful role. It defines the structure nested and shared across all the pages. layout.tsx gets all the pages through the children
prop and displays them according to the route hit.
It is particularly useful as it gives layouts of the shared piece of UI and doesn't re-render on client-side route changes.
app/
layout.tsx <-- Root layout, wraps all pages
about/
page.tsx <-- /about
layout.tsx <-- Layout specific to /about and its children
contact/
page.tsx <-- /contact
Example Code:
// app/laout.tsx
import Footer from "../_ui/Footer";
import Header from "../_ui/Header";
const RootLayout = ({ children }: { children: React.ReactElement }) => {
return (
<>
<Header />
<main>{children}</main>
<Footer />
</>
);
};
export default RootLayout;
loading.tsx
This file is seen when the page or layout (or data for them) is in pending state and coming from external sources. It is super useful for server components. No need to manually select the loading state and use useEffect
to make the state change. One cool thing about this file is that all the nested folders can use this file to show a loading state.
NOTE: It is a client component. use the 'use client' directive
Just place this file inside the layout.tsx or page.tsx
Example Code:
// app/blog/loading.tsx
export default function Loading() {
return <p>⏳ Loading blog post...</p>;
}
error.tsx
This file is useful to catch and display errors if an error is thrown in the layout or page. Similar to loading.tsx
, it is also a client component.
This component receives two props, error
and reset
. The error
prop is to get access to the error that occurred in the page or layout. The reset
prop is a function that retries to the route.
one key thing to remember is that error.tsx file only catches the error in the nested pages and layouts. It cannot catch the errors of the same folder files (i.e. page.tsx & template.tsx)
app/
error.tsx <-- The main error-catching file
layout.tsx <-- cannot catch the error of this layout
about/
page.tsx <-- can catch the error of this page
Example Code:
// app/blog/error.tsx
'use client';
const Error = ({ error, reset }: { error: Error; reset: () => void })=> {
return (
<div>
<h2>