How to Use Drizzle ORM with PostgreSQL in Next.js 15
This post is about adopting Drizzle ORM in a Next.js application. It explores Drizzle essential concepts & APIs and demonstrates how to integrate a Drizzle powered PostgreSQL database in the backend of an existing app router based Next.js dashboard application. Introduction Drizzle ORM is a type-safe, SQL-focused ORM for TypeScript that simplifies database modeling and queries with familiar SQL-like syntax. It integrates smoothly with PostgreSQL and modern frameworks like Next.js. This means, we can easily connect a Drizzle powered Node.js based environment of a Next.js application to a running PostgreSQL database, and then power the React side with Drizzle based queries and server actions. In this post, we explain with working code snippets how to adopt Drizzle ORM in an existing Next.js 15 admin panel dashboard application. At the end of this tutorial we should have pages that fetches data to/from a PostgreSQL database and perform mutations for customers and invoices resources: Prerequisites This demo demands the audience: are somewhat familiar with Next.js and want to build data intensive applications with Drizzle. If you are not up to speed with the Next.js app router, please follow along till chapter 13 from here. It’s a great starter. are familiar or are hands on with React Hook Form and Zod. It would be great if you have already worked with these headless libraries, as they are industry standards with React and Next.js. This is particularly, because, we do not discuss them in depth, as the focus is on their integration in a Drizzle backed dashboard application. If you don’t know them, there’s not much harm, as the Drizzle related steps are easy to follow. GitHub Repo And Starter Files The starter code for this demo application is available in this GitHub repository. You can begin with the code at the prepare branch and then follow along with this tutorial. The completed code is available inside the drizzle branch. Drizzle in Next.js: Our Goals Throughout the coming sections and subsections till the end of the post, we aim to rework and replace the data fetching functions in ./app/lib/mock.data.ts by setting up a Drizzle powered PostgreSQL database first and then performing necessary queries using Drizzle. We’ll also use Drizzle mutations in the server actions inside the ./app/lib/actions.ts file in order to perform database insertion, update and deletion. In due steps, we aim to: install & configure Drizzle for connecting PostgreSQL to Node.js in a Next.js application. declare Drizzle schema files with tables, schemas, partial queries, views, relations and type definitions. generate migration files and perform migrations & seeding. use Drizzle for data fetching in a Next.js server side. use Drizzle for database mutations from client side forms with React Hook Form and Zod. use Drizzle Query APIs for performing relational queries that return related resources as nested objects. Technical Requirements Developers should: have Node.js installed in their system. have PostgreSQL installed locally. have a local PG database named nextjs_drizzle created and it's credentials ready for use in an app. If you need some guidelines, please feel free to follow this Youtube tutorial. for convenience, have PgAdmin installed and know how to create a server, login to a database and perform PostgreSQL queries. Overview of Drizzle ORM Concepts and TypeScript APIs Drizzle ORM wraps SQL in TypeScript, mirroring SQL syntax with strong type safety. It brings relational modeling, querying, and migrations into your codebase with a developer-friendly API. Using SQL-Like Operations in Drizzle ORM Drizzle covers core SQL features like schemas, tables, relations, views, and migrations. It uses SQL-like methods ( select, insert, where, etc.) for intuitive query building in TypeScript. Using SQL-Like Operations in Drizzle ORM Drizzle offers dialect-specific features through opt-in packages like pg-core for PostgreSQL and modules for MySQL, SQLite, and cloud services like Supabase or PlanetScale. How to Connect Drizzle ORM to PostgreSQL, Supabase & Serverless Backends Drizzle supports multiple database clients with adapters for environments like Node.js ( node-postgres), serverless (Neon), and more. It connects via the drizzle() function to run queries. Creating Schemas, Tables, and Relations with Drizzle ORM Drizzle uses pgTable(), pgView(), and relation() to define your schema in TypeScript. You can also generate Zod-based validation schemas with createSelectSchema() and createInsertSchema(). Using Type-Safe Column Types in Drizzle ORM with PostgreSQL Drizzle provides SQL-like TypeScript APIs for defining columns, like uuid() for PostgreSQL's UUID type. These methods also support chaining constraints like .primaryKey() or .defaultRandom(). It supports all common SQL types- uuid, text,
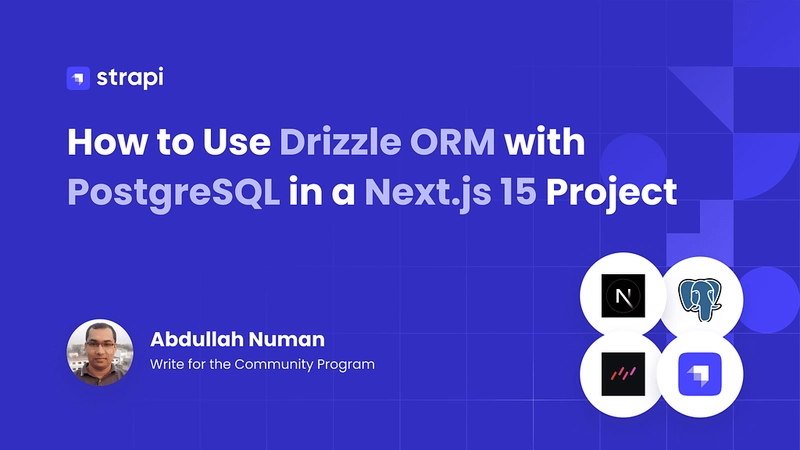
This post is about adopting Drizzle ORM in a Next.js application. It explores Drizzle essential concepts & APIs and demonstrates how to integrate a Drizzle powered PostgreSQL database in the backend of an existing app router based Next.js dashboard application.
Introduction
Drizzle ORM is a type-safe, SQL-focused ORM for TypeScript that simplifies database modeling and queries with familiar SQL-like syntax. It integrates smoothly with PostgreSQL and modern frameworks like Next.js.
This means, we can easily connect a Drizzle powered Node.js based environment of a Next.js application to a running PostgreSQL database, and then power the React side with Drizzle based queries and server actions.
In this post, we explain with working code snippets how to adopt Drizzle ORM in an existing Next.js 15 admin panel dashboard application.
At the end of this tutorial we should have pages that fetches data to/from a PostgreSQL database and perform mutations for customers and invoices resources:
Prerequisites
This demo demands the audience:
- are somewhat familiar with Next.js and want to build data intensive applications with Drizzle. If you are not up to speed with the Next.js app router, please follow along till chapter 13 from here. It’s a great starter.
- are familiar or are hands on with React Hook Form and Zod. It would be great if you have already worked with these headless libraries, as they are industry standards with React and Next.js. This is particularly, because, we do not discuss them in depth, as the focus is on their integration in a Drizzle backed dashboard application. If you don’t know them, there’s not much harm, as the Drizzle related steps are easy to follow.
GitHub Repo And Starter Files
The starter code for this demo application is available in this GitHub repository. You can begin with the code at the prepare branch and then follow along with this tutorial. The completed code is available inside the drizzle branch.
Drizzle in Next.js: Our Goals
Throughout the coming sections and subsections till the end of the post, we aim to rework and replace the data fetching functions in ./app/lib/mock.data.ts by setting up a Drizzle powered PostgreSQL database first and then performing necessary queries using Drizzle.
We’ll also use Drizzle mutations in the server actions inside the ./app/lib/actions.ts file in order to perform database insertion, update and deletion.
In due steps, we aim to:
- install & configure Drizzle for connecting PostgreSQL to Node.js in a Next.js application.
- declare Drizzle schema files with tables, schemas, partial queries, views, relations and type definitions.
- generate migration files and perform migrations & seeding.
- use Drizzle for data fetching in a Next.js server side.
- use Drizzle for database mutations from client side forms with React Hook Form and Zod.
- use Drizzle Query APIs for performing relational queries that return related resources as nested objects.
Technical Requirements
Developers should:
- have Node.js installed in their system.
- have PostgreSQL installed locally.
- have a local PG database named nextjs_drizzle created and it's credentials ready for use in an app. If you need some guidelines, please feel free to follow this Youtube tutorial.
- for convenience, have PgAdmin installed and know how to create a server, login to a database and perform PostgreSQL queries.
Overview of Drizzle ORM Concepts and TypeScript APIs
Drizzle ORM wraps SQL in TypeScript, mirroring SQL syntax with strong type safety. It brings relational modeling, querying, and migrations into your codebase with a developer-friendly API.
Using SQL-Like Operations in Drizzle ORM
Drizzle covers core SQL features like schemas, tables, relations, views, and migrations. It uses SQL-like methods ( select, insert, where, etc.) for intuitive query building in TypeScript.
Using SQL-Like Operations in Drizzle ORM
Drizzle offers dialect-specific features through opt-in packages like pg-core for PostgreSQL and modules for MySQL, SQLite, and cloud services like Supabase or PlanetScale.
How to Connect Drizzle ORM to PostgreSQL, Supabase & Serverless Backends
Drizzle supports multiple database clients with adapters for environments like Node.js ( node-postgres), serverless (Neon), and more. It connects via the drizzle() function to run queries.
Creating Schemas, Tables, and Relations with Drizzle ORM
Drizzle uses pgTable(), pgView(), and relation() to define your schema in TypeScript. You can also generate Zod-based validation schemas with createSelectSchema() and createInsertSchema().
Using Type-Safe Column Types in Drizzle ORM with PostgreSQL
Drizzle provides SQL-like TypeScript APIs for defining columns, like uuid() for PostgreSQL's UUID type. These methods also support chaining constraints like .primaryKey() or .defaultRandom(). It supports all common SQL types- uuid, text, int, boolean, json, enum, etc.-through dialect-specific packages like pg-core.