Linux First: Your Step-by-Step DevOps Foundation Guide
After my post about learning Linux before diving into Kubernetes, some of you asked for more specific guidance. Shout out to KC, who perfectly captured what many were thinking: "Can you detail those 5 steps? I've been using WSL but had no idea you could build a web server in it." Challenge accepted! Let's break down how to build that Linux foundation that makes everything else in DevOps click—whether you're on Windows, macOS, or already running Linux. Step 1: Install a Linux distro (and actually use it daily) The goal here isn't just having Linux—it's making it part of your daily workflow until it becomes second nature. Windows Users: WSL (Windows Subsystem for Linux) is your best friend. Here's how to get started: Open PowerShell as administrator and run: wsl --install This installs Ubuntu by default, which is perfect for beginners. After installation, create your Linux user and password when prompted. Make WSL part of your daily routine: Set up Windows Terminal to open WSL by default Keep a terminal open while coding Challenge: Try doing your git operations from the WSL terminal instead of GUI tools for a week macOS Users: You're already on a Unix-based system, which is helpful, but I still recommend a Linux VM for the full experience: Install UTM (free) or Parallels (paid) to run Linux VMs Install Ubuntu or Debian and allocate at least 2GB RAM Or if you prefer containers: brew install docker and run a Linux container Linux Desktop Users: You're already ahead! Just make sure you're: Using the command line regularly instead of GUI tools Learning your distro's package manager inside out Managing your system via the terminal Step 2: Learn Bash (it's your DevOps superpower) I used to think Bash was just "that thing where I type commands." Now I know it's the secret weapon of productive DevOps engineers. Bash Basics to Advanced: Create a practice.sh file with: #!/bin/bash echo "Hello, $(whoami)! Today is $(date)" Make it executable: chmod +x practice.sh ./practice.sh Build a real automation script (start simple): #!/bin/bash # Simple backup script SOURCE_DIR="$HOME/projects" BACKUP_DIR="$HOME/backups" TIMESTAMP=$(date +"%Y%m%d_%H%M%S") # Create backup directory if it doesn't exist mkdir -p "$BACKUP_DIR" # Create the backup tar -czf "$BACKUP_DIR/backup_$TIMESTAMP.tar.gz" "$SOURCE_DIR" echo "Backup created at $BACKUP_DIR/backup_$TIMESTAMP.tar.gz" Learn these bash concepts systematically: Variables and environment variables If/else conditionals Loops (for, while) Functions Processing command output Exit codes and error handling I became comfortable with Bash by building small utility scripts for things I did repeatedly, like setting up development environments or cleaning up temp files. Step 3: Build stuff manually (before automating) This is the most transformative step. Before containerizing or automating, build it by hand. Set Up a Basic Web Server in WSL/Linux: Install Nginx: sudo apt update sudo apt install nginx Start the service: sudo service nginx start Create a custom HTML page: echo "My First Linux Web Server" | sudo tee /var/www/html/index.html Access it: From WSL: curl localhost From Windows host with WSL: Open browser to http://localhost or http://[WSL-IP] To find your WSL IP: ip addr show eth0 | grep "inet\b" | awk '{print $2}' | cut -d/ -f1 Other essentials to build manually: Database server (MySQL/PostgreSQL) SSH keys and secure server setup Firewall configuration with ufw or iptables Basic LAMP/LEMP stack Cron jobs for scheduled tasks When I first set up Nginx manually, I suddenly understood what all those Docker port mappings were doing—it wasn't magic anymore! Step 4: Google like a pro (and understand what you're copying) We all Google, but are you doing it effectively? Sharpen your search skills: Use specific error messages in quotes Add your exact Linux distro to searches Include version numbers Try site-specific searches like site:stackoverflow.com nginx 403 forbidden When reading solutions: Break down each command: systemctl status nginx What is systemctl? What does status do? What's the difference with service nginx status? Use man pages and --help flags: man systemctl systemctl --help Understand permissions and processes: ps aux | grep nginx ls -la /var/www/html I keep a personal "command dictionary" where I document commands I've learned and what each flag does. It's become my most valuable resource. Step 5: Document everything (your future self will thank you) Documentation isn't just nice—it's necessary. Here's my system: Set up a personal knowledge base: Use Markdown files in a git repo Try Obsidian or Not
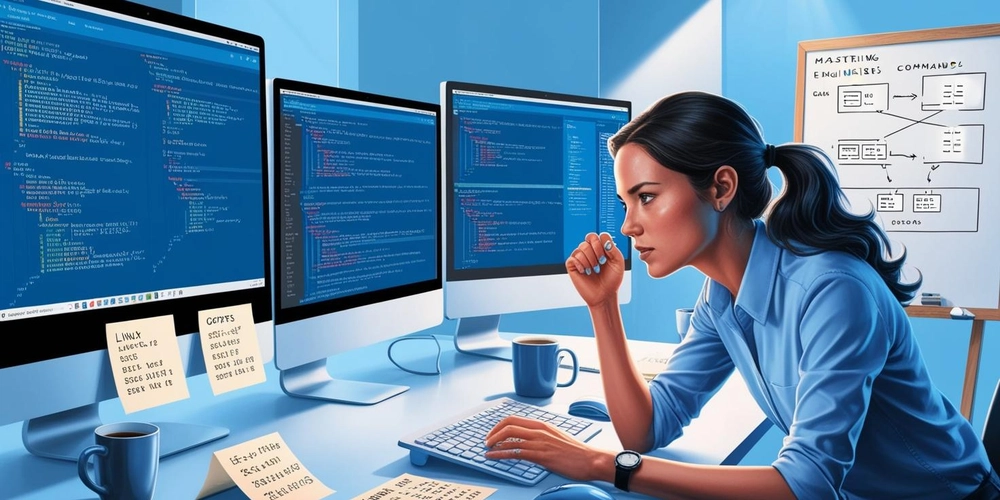
After my post about learning Linux before diving into Kubernetes, some of you asked for more specific guidance. Shout out to KC, who perfectly captured what many were thinking: "Can you detail those 5 steps? I've been using WSL but had no idea you could build a web server in it."
Challenge accepted! Let's break down how to build that Linux foundation that makes everything else in DevOps click—whether you're on Windows, macOS, or already running Linux.
Step 1: Install a Linux distro (and actually use it daily)
The goal here isn't just having Linux—it's making it part of your daily workflow until it becomes second nature.
Windows Users:
WSL (Windows Subsystem for Linux) is your best friend. Here's how to get started:
- Open PowerShell as administrator and run:
wsl --install
This installs Ubuntu by default, which is perfect for beginners.
After installation, create your Linux user and password when prompted.
-
Make WSL part of your daily routine:
- Set up Windows Terminal to open WSL by default
- Keep a terminal open while coding
- Challenge: Try doing your git operations from the WSL terminal instead of GUI tools for a week
macOS Users:
You're already on a Unix-based system, which is helpful, but I still recommend a Linux VM for the full experience:
- Install UTM (free) or Parallels (paid) to run Linux VMs
- Install Ubuntu or Debian and allocate at least 2GB RAM
- Or if you prefer containers:
brew install docker
and run a Linux container
Linux Desktop Users:
You're already ahead! Just make sure you're:
- Using the command line regularly instead of GUI tools
- Learning your distro's package manager inside out
- Managing your system via the terminal
Step 2: Learn Bash (it's your DevOps superpower)
I used to think Bash was just "that thing where I type commands." Now I know it's the secret weapon of productive DevOps engineers.
Bash Basics to Advanced:
- Create a
practice.sh
file with:
#!/bin/bash
echo "Hello, $(whoami)! Today is $(date)"
- Make it executable:
chmod +x practice.sh
./practice.sh
- Build a real automation script (start simple):
#!/bin/bash
# Simple backup script
SOURCE_DIR="$HOME/projects"
BACKUP_DIR="$HOME/backups"
TIMESTAMP=$(date +"%Y%m%d_%H%M%S")
# Create backup directory if it doesn't exist
mkdir -p "$BACKUP_DIR"
# Create the backup
tar -czf "$BACKUP_DIR/backup_$TIMESTAMP.tar.gz" "$SOURCE_DIR"
echo "Backup created at $BACKUP_DIR/backup_$TIMESTAMP.tar.gz"
- Learn these bash concepts systematically:
- Variables and environment variables
- If/else conditionals
- Loops (for, while)
- Functions
- Processing command output
- Exit codes and error handling
I became comfortable with Bash by building small utility scripts for things I did repeatedly, like setting up development environments or cleaning up temp files.
Step 3: Build stuff manually (before automating)
This is the most transformative step. Before containerizing or automating, build it by hand.
Set Up a Basic Web Server in WSL/Linux:
- Install Nginx:
sudo apt update
sudo apt install nginx
- Start the service:
sudo service nginx start
- Create a custom HTML page:
echo "My First Linux Web Server
" | sudo tee /var/www/html/index.html
- Access it:
- From WSL:
curl localhost
- From Windows host with WSL: Open browser to http://localhost or http://[WSL-IP]
- From WSL:
To find your WSL IP: ip addr show eth0 | grep "inet\b" | awk '{print $2}' | cut -d/ -f1
Other essentials to build manually:
- Database server (MySQL/PostgreSQL)
- SSH keys and secure server setup
- Firewall configuration with
ufw
oriptables
- Basic LAMP/LEMP stack
- Cron jobs for scheduled tasks
When I first set up Nginx manually, I suddenly understood what all those Docker port mappings were doing—it wasn't magic anymore!
Step 4: Google like a pro (and understand what you're copying)
We all Google, but are you doing it effectively?
Sharpen your search skills:
- Use specific error messages in quotes
- Add your exact Linux distro to searches
- Include version numbers
- Try site-specific searches like
site:stackoverflow.com nginx 403 forbidden
When reading solutions:
- Break down each command:
systemctl status nginx
What is systemctl? What does status do? What's the difference with service nginx status
?
- Use
man
pages and--help
flags:
man systemctl
systemctl --help
- Understand permissions and processes:
ps aux | grep nginx
ls -la /var/www/html
I keep a personal "command dictionary" where I document commands I've learned and what each flag does. It's become my most valuable resource.
Step 5: Document everything (your future self will thank you)
Documentation isn't just nice—it's necessary. Here's my system:
-
Set up a personal knowledge base:
- Use Markdown files in a git repo
- Try Obsidian or Notion
- Even a simple Google Doc works
Document even "obvious" things:
# Setting up Nginx on Ubuntu 22.04
1. Install: `sudo apt install nginx`
2. Start service: `sudo service nginx start`
3. Check status: `sudo service nginx status`
4. Default config location: /etc/nginx/sites-available/default
5. Web root: /var/www/html
6. Logs: /var/log/nginx/
- Include what didn't work (and why):
Note: Initially tried using port 80 but got permission errors.
Fixed by either:
- Using port above 1024 (no sudo needed)
- Or adding capability: `sudo setcap 'cap_net_bind_service=+ep' /usr/sbin/nginx`
I can't tell you how many times my documentation has saved me hours of frustration when revisiting something months later.
Bonus: Little-Known Linux Skills That Made Me Better
These smaller skills dramatically improved my Linux confidence:
1. Process and port management:
# Find what's using port 3000
sudo lsof -i :3000
# Kill a process
kill -9
# Check resource usage
htop
2. System monitoring:
# Monitor logs in real-time
tail -f /var/log/nginx/error.log
# Check disk space
df -h
3. Network troubleshooting:
# Test connectivity
ping google.com
# Trace route
traceroute google.com
# DNS lookup
nslookup example.com
4. User and permission management:
# Add user to group
sudo usermod -aG docker $USER
# Change ownership
sudo chown -R user:group /path/to/directory
Putting It All Together
After getting comfortable with these steps, you'll find that:
- Docker makes sense because you understand the Linux processes it's containerizing
- Kubernetes feels logical because you know how networking and processes work
- CI/CD is clearer because you understand the underlying shell commands
- Configuration management tools like Ansible are intuitive because you've done the manual steps
Learning Linux first gave me something invaluable: confidence. Not the temporary confidence of following a tutorial, but the lasting confidence of understanding what's happening under the hood.
When things break in production (and they will), you won't freeze—you'll know exactly where to look and how to fix it.
So what are you waiting for? Open that terminal and start building your Linux foundation today. Your future DevOps self will thank you.
If this helped you, let me know what you build first! I'm always curious to see how others are starting their Linux journey. Drop a comment or DM me with your progress or questions.