How to Use LocalStorage, SessionStorage and Cookies in JavaScript
Web storage is a powerful feature in modern browsers that allows you to store data directly on a user's computer. In JavaScript, the three primary ways to handle client-side storage are LocalStorage, SessionStorage and Cookies. In this article, we'll explore each method, explain their differences and provide simple examples to get you started. LocalStorage LocalStorage is designed for storing data with no expiration date. This means that the data you store remains available even after the browser is closed and reopened. It’s perfect for settings, user preferences, or any other information you’d like to persist across sessions. Key Characteristics: Persistence: Data persists until explicitly removed. Storage Limit: Approximately 5-10 MB per origin (depending on the browser). Scope: Accessible only within the domain that stored it. Basic Operations: Setting an Item: // Save data to localStorage localStorage.setItem('username', 'Alice'); Getting an Item: // Retrieve data from localStorage const username = localStorage.getItem('username'); console.log(username); // Outputs: Alice Removing an Item: // Remove a specific item localStorage.removeItem('username'); Clearing All Items: // Remove all data from localStorage localStorage.clear(); SessionStorage SessionStorage is similar to LocalStorage, but with one key difference: data stored in SessionStorage is cleared when the page session ends. A page session lasts as long as the browser is open, and survives over page reloads and restores. However, once the browser is closed, the data is lost. Key Characteristics: Session-Based: Data is available only during the page session. Scope: Accessible only within the tab or window where it was stored. Usage: Ideal for temporary data, such as shopping cart contents that don’t need to persist after the session. Basic Operations: Setting an Item: // Save data to sessionStorage sessionStorage.setItem('sessionData', 'This is temporary data'); Getting an Item: // Retrieve data from sessionStorage const data = sessionStorage.getItem('sessionData'); console.log(data); // Outputs: This is temporary data Removing an Item: // Remove a specific item sessionStorage.removeItem('sessionData'); Clearing All Items: // Remove all data from sessionStorage sessionStorage.clear(); Cookies Cookies have been around much longer than the Web Storage APIs and are primarily used for storing small pieces of data that need to be sent back to the server with each HTTP request. They can have an expiration date, and you can set options like path, domain, and security flags. Key Characteristics: Small Data Size: Typically limited to around 4 KB per cookie. Server Communication: Automatically sent with every HTTP request to the same domain. Expiration: Can be set to expire at a specific time. Basic Operations: Unlike localStorage and sessionStorage, cookies do not have a simple API for get/set operations in JavaScript. Instead, you manipulate the document.cookie string. Setting a Cookie: // Set a cookie that expires in 7 days const setCookie = (name, value, days) => { const date = new Date(); date.setTime(date.getTime() + days * 24 * 60 * 60 * 1000); const expires = "expires=" + date.toUTCString(); document.cookie = name + "=" + value + ";" + expires + ";path=/"; }; setCookie('user', 'Bob', 7); Getting a Cookie: // Retrieve a cookie value by name const getCookie = (name) => { const cname = name + "="; const decodedCookie = decodeURIComponent(document.cookie); const cookieArray = decodedCookie.split(';'); for (let cookie of cookieArray) { cookie = cookie.trim(); if (cookie.indexOf(cname) === 0) { return cookie.substring(cname.length, cookie.length); } } return ""; }; console.log(getCookie('user')); // Outputs: Bob Deleting a Cookie: // To delete a cookie, set its expiry date to a past date const deleteCookie = (name) => { document.cookie = name + "=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/;"; }; deleteCookie('user'); Choosing the Right Storage Option Each storage method serves a different purpose: LocalStorage: Best for storing data that you want to persist over long periods, such as user preferences. SessionStorage: Ideal for data that should only be available during a single session, such as temporary form data. Cookies: Useful when you need to send data back to the server with HTTP requests or when working with authentication tokens (keeping in mind the data size limitations). When building your web application, consider the nature and lifetime of the data you’re storing. For example, if your application needs to remember user preferences even after closing the browser, LocalStorage is your go-to. Conversely, if you need data only during the
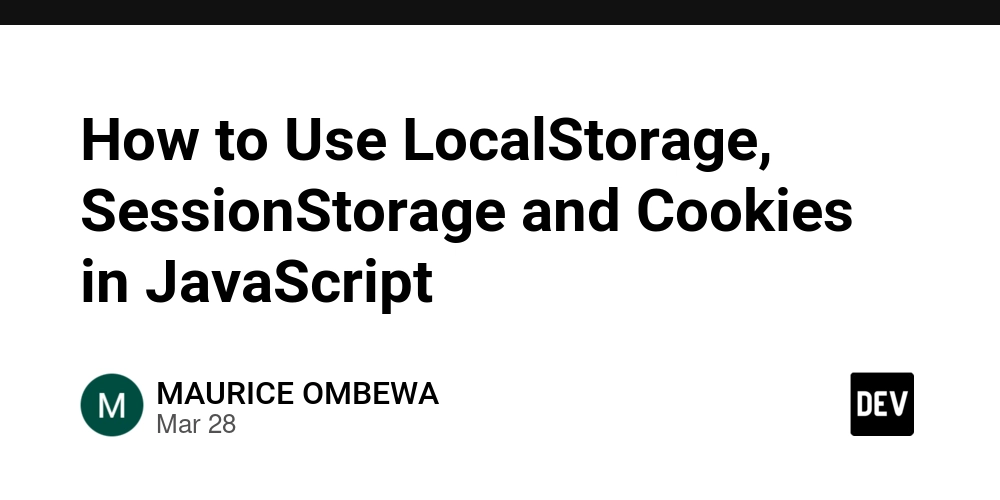
Web storage is a powerful feature in modern browsers that allows you to store data directly on a user's computer. In JavaScript, the three primary ways to handle client-side storage are LocalStorage, SessionStorage and Cookies. In this article, we'll explore each method, explain their differences and provide simple examples to get you started.
LocalStorage
LocalStorage is designed for storing data with no expiration date. This means that the data you store remains available even after the browser is closed and reopened. It’s perfect for settings, user preferences, or any other information you’d like to persist across sessions.
Key Characteristics:
- Persistence: Data persists until explicitly removed.
- Storage Limit: Approximately 5-10 MB per origin (depending on the browser).
- Scope: Accessible only within the domain that stored it.
Basic Operations:
- Setting an Item:
// Save data to localStorage
localStorage.setItem('username', 'Alice');
- Getting an Item:
// Retrieve data from localStorage
const username = localStorage.getItem('username');
console.log(username); // Outputs: Alice
- Removing an Item:
// Remove a specific item
localStorage.removeItem('username');
- Clearing All Items:
// Remove all data from localStorage
localStorage.clear();
SessionStorage
SessionStorage is similar to LocalStorage, but with one key difference: data stored in SessionStorage is cleared when the page session ends. A page session lasts as long as the browser is open, and survives over page reloads and restores. However, once the browser is closed, the data is lost.
Key Characteristics:
- Session-Based: Data is available only during the page session.
- Scope: Accessible only within the tab or window where it was stored.
- Usage: Ideal for temporary data, such as shopping cart contents that don’t need to persist after the session.
Basic Operations:
- Setting an Item:
// Save data to sessionStorage
sessionStorage.setItem('sessionData', 'This is temporary data');
- Getting an Item:
// Retrieve data from sessionStorage
const data = sessionStorage.getItem('sessionData');
console.log(data); // Outputs: This is temporary data
- Removing an Item:
// Remove a specific item
sessionStorage.removeItem('sessionData');
- Clearing All Items:
// Remove all data from sessionStorage
sessionStorage.clear();
Cookies
Cookies have been around much longer than the Web Storage APIs and are primarily used for storing small pieces of data that need to be sent back to the server with each HTTP request. They can have an expiration date, and you can set options like path, domain, and security flags.
Key Characteristics:
- Small Data Size: Typically limited to around 4 KB per cookie.
- Server Communication: Automatically sent with every HTTP request to the same domain.
- Expiration: Can be set to expire at a specific time.
Basic Operations:
Unlike localStorage and sessionStorage, cookies do not have a simple API for get/set operations in JavaScript. Instead, you manipulate the document.cookie string.
- Setting a Cookie:
// Set a cookie that expires in 7 days
const setCookie = (name, value, days) => {
const date = new Date();
date.setTime(date.getTime() + days * 24 * 60 * 60 * 1000);
const expires = "expires=" + date.toUTCString();
document.cookie = name + "=" + value + ";" + expires + ";path=/";
};
setCookie('user', 'Bob', 7);
- Getting a Cookie:
// Retrieve a cookie value by name
const getCookie = (name) => {
const cname = name + "=";
const decodedCookie = decodeURIComponent(document.cookie);
const cookieArray = decodedCookie.split(';');
for (let cookie of cookieArray) {
cookie = cookie.trim();
if (cookie.indexOf(cname) === 0) {
return cookie.substring(cname.length, cookie.length);
}
}
return "";
};
console.log(getCookie('user')); // Outputs: Bob
- Deleting a Cookie:
// To delete a cookie, set its expiry date to a past date
const deleteCookie = (name) => {
document.cookie = name + "=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/;";
};
deleteCookie('user');
Choosing the Right Storage Option
Each storage method serves a different purpose:
- LocalStorage: Best for storing data that you want to persist over long periods, such as user preferences.
- SessionStorage: Ideal for data that should only be available during a single session, such as temporary form data.
- Cookies: Useful when you need to send data back to the server with HTTP requests or when working with authentication tokens (keeping in mind the data size limitations).
When building your web application, consider the nature and lifetime of the data you’re storing. For example, if your application needs to remember user preferences even after closing the browser, LocalStorage is your go-to. Conversely, if you need data only during the session, SessionStorage is more appropriate.
Conclusion
Understanding the differences between LocalStorage, SessionStorage, and Cookies is essential for effective client-side data management in JavaScript. Each method has its unique benefits and limitations:
_LocalStorage_ provides persistent storage across sessions.
_SessionStorage_ offers temporary storage within a single session.
_Cookies_ enable small data transfers between the client and server.
By choosing the appropriate storage solution, you can optimize your web application's performance and ensure that data is handled in the most efficient manner. Experiment with these examples and see how they can enhance the user experience on your site!
Happy coding!