How to Extract File Attachments in C from ZUGFeRD Documents?
Introduction Extracting file attachments from documents can be a challenging task, especially when working with specific formats like ZUGFeRD. ZUGFeRD (Zentraler User Guide für die elektronische Rechnungsstellung im Deutschland) is a standard for electronic invoices. This article will focus on providing a function written in C that allows you to effectively extract file attachments following the specifications outlined in the ZUGFeRD Format, specifically from the AF (Attachment File) array in your document. Understanding the Structure of ZUGFeRD Before we delve into the code, let's recap how the ZUGFeRD format works with attachments. AF refers to the array that contains file specifications of attachments, and EF denotes a dictionary associated with actual file content. Understanding this structure is crucial for extracting attachments correctly. Why Does It Work for Most Files? In a typical ZUGFeRD compliant file, the AF array usually contains valid file specifications pointing to the EF dictionary. However, issues arise when these entries are absent or improperly linked, as seen with the document you mentioned. In this case, the extraction process fails because the references don’t lead to valid content streams. Step-by-Step Solution Here's a clear approach to implement the extraction functionality, focusing on both robust error handling and verification of references. Step 1: Include Necessary Libraries You will need to include standard libraries for file operations and potentially a JSON library if your ZUGFeRD file is structured in JSON or needs JSON parsing. #include #include #include // Include your JSON or XML library as needed Step 2: Define Structures Next, you must define the necessary structures to hold your attachment data. For simplification, let’s use structures for AF and EF. typedef struct { char *fileSpec; } AttachmentFile; typedef struct { char *content; } FileContent; Step 3: Function to Extract Attachments Below is the core function which extracts attachments from the provided document structure. This assumes you have parsed your ZUGFeRD file into readable data. void extractAttachments(AttachmentFile *afArray, int size) { for (int i = 0; i < size; i++) { char *fileSpec = afArray[i].fileSpec; FileContent *fileContent = getContentFromSpec(fileSpec); if (fileContent) { // Process your file content. Save or display. printf("Content for file: %s - %s\n", fileSpec, fileContent->content); } else { printf("No content found for file spec: %s\n", fileSpec); } } } Step 4: Getting Content from Specification You will need to implement getContentFromSpec which searches for the EF dictionary linked to your AF entry. FileContent* getContentFromSpec(char *fileSpec) { // Simulate fetching the content from EF // Here you would have your logic to retrieve and parse EF return NULL; // Return content or NULL if not found. } Handling Cases Where the References Aren't Found In cases where the attachment doesn't lead anywhere, as you've seen with the problematic document, it may not be possible to extract the content directly. As a workaround, examine the format closely for potential misreferences or structure anomalies. This requires a manual inspection against the ZUGFeRD documentation. Frequently Asked Questions Is It Possible to Extract Attachments in Plain C? Yes, it is possible to extract attachments using plain C, as demonstrated in the examples. However, working with advanced file structures and parsing may require additional libraries for handling specific formats (e.g., JSON, XML). What If the References Are Not Valid? If the references in the AF array or /Names dictionary do not lead to valid file specs, you'll need to validate the document against ZUGFeRD standards and possibly correct any structural issues. Conclusion While extracting attachments from ZUGFeRD documents can be straightforward, complications arise with malformed entries. The provided C code offers a basic structure for attaining this extraction. Ensure rigorous validation of input documents to achieve the best results.
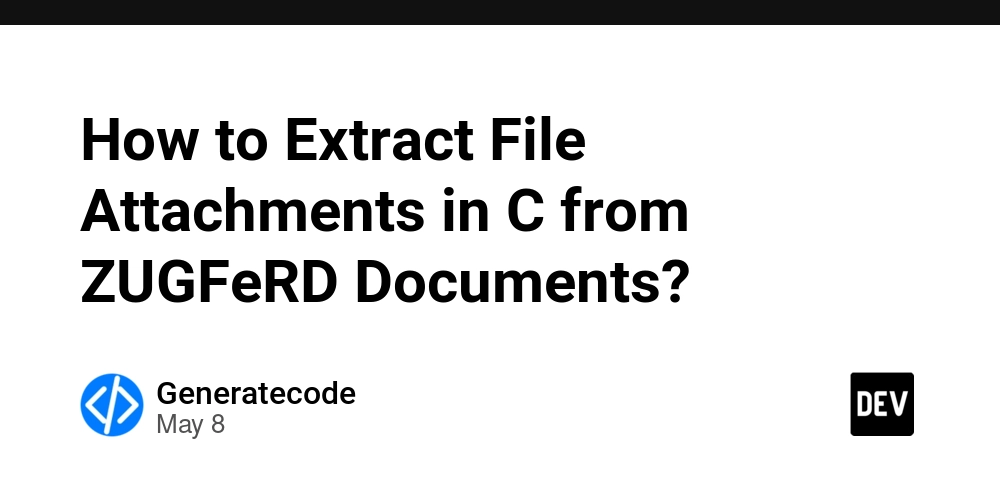
Introduction
Extracting file attachments from documents can be a challenging task, especially when working with specific formats like ZUGFeRD. ZUGFeRD (Zentraler User Guide für die elektronische Rechnungsstellung im Deutschland) is a standard for electronic invoices. This article will focus on providing a function written in C that allows you to effectively extract file attachments following the specifications outlined in the ZUGFeRD Format, specifically from the AF (Attachment File) array in your document.
Understanding the Structure of ZUGFeRD
Before we delve into the code, let's recap how the ZUGFeRD format works with attachments. AF refers to the array that contains file specifications of attachments, and EF denotes a dictionary associated with actual file content. Understanding this structure is crucial for extracting attachments correctly.
Why Does It Work for Most Files?
In a typical ZUGFeRD compliant file, the AF array usually contains valid file specifications pointing to the EF dictionary. However, issues arise when these entries are absent or improperly linked, as seen with the document you mentioned. In this case, the extraction process fails because the references don’t lead to valid content streams.
Step-by-Step Solution
Here's a clear approach to implement the extraction functionality, focusing on both robust error handling and verification of references.
Step 1: Include Necessary Libraries
You will need to include standard libraries for file operations and potentially a JSON library if your ZUGFeRD file is structured in JSON or needs JSON parsing.
#include
#include
#include
// Include your JSON or XML library as needed
Step 2: Define Structures
Next, you must define the necessary structures to hold your attachment data. For simplification, let’s use structures for AF and EF.
typedef struct {
char *fileSpec;
} AttachmentFile;
typedef struct {
char *content;
} FileContent;
Step 3: Function to Extract Attachments
Below is the core function which extracts attachments from the provided document structure. This assumes you have parsed your ZUGFeRD file into readable data.
void extractAttachments(AttachmentFile *afArray, int size) {
for (int i = 0; i < size; i++) {
char *fileSpec = afArray[i].fileSpec;
FileContent *fileContent = getContentFromSpec(fileSpec);
if (fileContent) {
// Process your file content. Save or display.
printf("Content for file: %s - %s\n", fileSpec, fileContent->content);
} else {
printf("No content found for file spec: %s\n", fileSpec);
}
}
}
Step 4: Getting Content from Specification
You will need to implement getContentFromSpec
which searches for the EF dictionary linked to your AF entry.
FileContent* getContentFromSpec(char *fileSpec) {
// Simulate fetching the content from EF
// Here you would have your logic to retrieve and parse EF
return NULL; // Return content or NULL if not found.
}
Handling Cases Where the References Aren't Found
In cases where the attachment doesn't lead anywhere, as you've seen with the problematic document, it may not be possible to extract the content directly. As a workaround, examine the format closely for potential misreferences or structure anomalies. This requires a manual inspection against the ZUGFeRD documentation.
Frequently Asked Questions
Is It Possible to Extract Attachments in Plain C?
Yes, it is possible to extract attachments using plain C, as demonstrated in the examples. However, working with advanced file structures and parsing may require additional libraries for handling specific formats (e.g., JSON, XML).
What If the References Are Not Valid?
If the references in the AF array or /Names dictionary do not lead to valid file specs, you'll need to validate the document against ZUGFeRD standards and possibly correct any structural issues.
Conclusion
While extracting attachments from ZUGFeRD documents can be straightforward, complications arise with malformed entries. The provided C code offers a basic structure for attaining this extraction. Ensure rigorous validation of input documents to achieve the best results.