How to Easily Implement RTMP Live Streaming in Rust: A Practical Guide
Introduction With the rapid growth of live streaming, RTMP (Real-Time Messaging Protocol) has become a standard choice for broadcasting real-time media content. However, directly working with low-level APIs to implement RTMP streaming can be complex, error-prone, and may introduce memory safety issues, posing significant challenges for developers. This article explores how you can leverage Rust’s safety, efficiency, and developer-friendly features to simplify RTMP live streaming. We'll discuss common streaming scenarios, technical challenges, and provide practical solutions with ready-to-use examples. Why Use Rust for RTMP Streaming? Common pain points when implementing RTMP streaming include: Complex low-level APIs with a steep learning curve. Memory management and safety risks when directly interfacing with FFmpeg APIs. High demands for real-time performance and stability. Rust, by its very design, addresses these challenges effectively: Offers zero-cost abstractions and built-in memory safety. Performance comparable to C/C++ with a significantly improved developer experience. Rich ecosystem and seamless integration with existing C/C++ libraries. Thus, Rust is an excellent choice for developing robust and efficient RTMP streaming applications. Common RTMP Streaming Scenarios Based on real-world requirements, RTMP streaming usually falls into two scenarios: 1. Streaming to Public Platforms Use Case: Platforms like Twitch, YouTube, or Facebook Live for large audience broadcasts. Key Requirements: Stability, low latency, ease of use. 2. Local or Internal Streaming Use Case: Suitable for internal live streams, testing, development, or LAN video monitoring. Key Requirements: Easy deployment, flexibility, quick setup, and convenient debugging. Solution: Rust with ez-ffmpeg To address these challenges and requirements, we introduce ez-ffmpeg, a Rust library providing a clean abstraction over FFmpeg, significantly simplifying RTMP streaming through user-friendly interfaces and automatic memory management. Technical Highlights: FFI Bindings: Safe and efficient interfacing with native FFmpeg APIs. Automatic Memory Management: Eliminates manual memory handling, enhancing safety and productivity. Ergonomic Design: Chainable method calls, improving developer experience. Quick Start Examples Environment Setup Install FFmpeg macOS: brew install ffmpeg Windows: vcpkg install ffmpeg Rust Dependency [dependencies] ez-ffmpeg = { version = "*", features = ["rtmp"] } Scenario 1: Stream to Public RTMP Servers The following example shows how to push a local video file to public RTMP platforms (e.g., YouTube, Twitch): use ez_ffmpeg::{FfmpegContext, Input, Output}; fn main() -> Result { let input = Input::from("video.mp4"); let output = Output::from("rtmp://your-platform-address/app/stream_key"); FfmpegContext::builder() .input(input) .output(output) .build()? .start()? .wait()?; Ok(()) } Scenario 2: Embedded Local RTMP Server Ideal for local testing and quick setup: use ez_ffmpeg::rtmp::embed_rtmp_server::EmbedRtmpServer; use ez_ffmpeg::{FfmpegContext, Input}; fn main() -> Result { let mut server = EmbedRtmpServer::new("localhost:1935").start()?; let output = server.create_rtmp_input("test-app", "test-stream")?; let input = Input::from("video.mp4"); FfmpegContext::builder() .input(input) .output(output) .build()? .start()? .wait()?; Ok(()) } Clients can access the stream using the URL: rtmp://localhost:1935/test-app/test-stream Conclusion By leveraging Rust and the ez-ffmpeg library, developers can easily and safely implement RTMP streaming applications. Whether targeting public platforms or setting up local streaming environments, this approach makes development faster and more reliable.
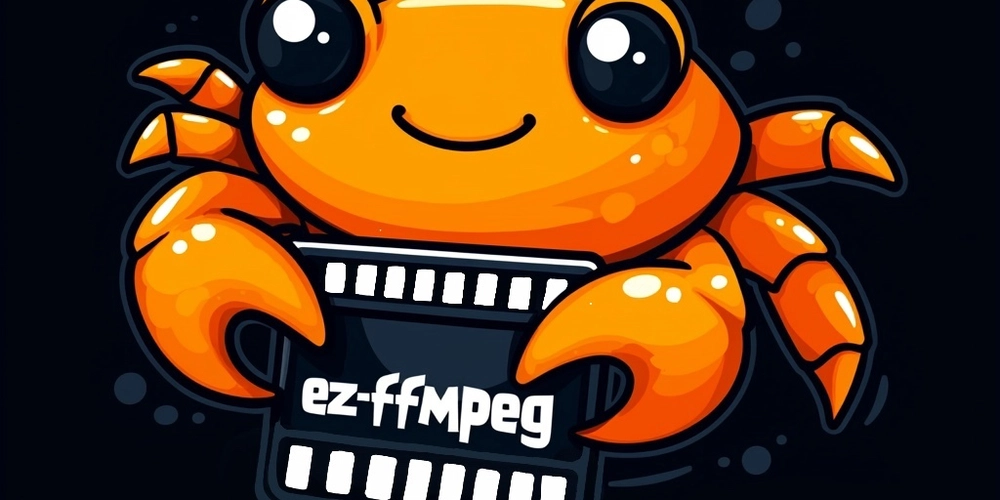
Introduction
With the rapid growth of live streaming, RTMP (Real-Time Messaging Protocol) has become a standard choice for broadcasting real-time media content. However, directly working with low-level APIs to implement RTMP streaming can be complex, error-prone, and may introduce memory safety issues, posing significant challenges for developers.
This article explores how you can leverage Rust’s safety, efficiency, and developer-friendly features to simplify RTMP live streaming. We'll discuss common streaming scenarios, technical challenges, and provide practical solutions with ready-to-use examples.
Why Use Rust for RTMP Streaming?
Common pain points when implementing RTMP streaming include:
- Complex low-level APIs with a steep learning curve.
- Memory management and safety risks when directly interfacing with FFmpeg APIs.
- High demands for real-time performance and stability.
Rust, by its very design, addresses these challenges effectively:
- Offers zero-cost abstractions and built-in memory safety.
- Performance comparable to C/C++ with a significantly improved developer experience.
- Rich ecosystem and seamless integration with existing C/C++ libraries.
Thus, Rust is an excellent choice for developing robust and efficient RTMP streaming applications.
Common RTMP Streaming Scenarios
Based on real-world requirements, RTMP streaming usually falls into two scenarios:
1. Streaming to Public Platforms
- Use Case: Platforms like Twitch, YouTube, or Facebook Live for large audience broadcasts.
- Key Requirements: Stability, low latency, ease of use.
2. Local or Internal Streaming
- Use Case: Suitable for internal live streams, testing, development, or LAN video monitoring.
- Key Requirements: Easy deployment, flexibility, quick setup, and convenient debugging.
Solution: Rust with ez-ffmpeg
To address these challenges and requirements, we introduce ez-ffmpeg
, a Rust library providing a clean abstraction over FFmpeg, significantly simplifying RTMP streaming through user-friendly interfaces and automatic memory management.
Technical Highlights:
- FFI Bindings: Safe and efficient interfacing with native FFmpeg APIs.
- Automatic Memory Management: Eliminates manual memory handling, enhancing safety and productivity.
- Ergonomic Design: Chainable method calls, improving developer experience.
Quick Start Examples
Environment Setup
Install FFmpeg
- macOS:
brew install ffmpeg
- Windows:
vcpkg install ffmpeg
Rust Dependency
[dependencies]
ez-ffmpeg = { version = "*", features = ["rtmp"] }
Scenario 1: Stream to Public RTMP Servers
The following example shows how to push a local video file to public RTMP platforms (e.g., YouTube, Twitch):
use ez_ffmpeg::{FfmpegContext, Input, Output};
fn main() -> Result<(), Box<dyn std::error::Error>> {
let input = Input::from("video.mp4");
let output = Output::from("rtmp://your-platform-address/app/stream_key");
FfmpegContext::builder()
.input(input)
.output(output)
.build()?
.start()?
.wait()?;
Ok(())
}
Scenario 2: Embedded Local RTMP Server
Ideal for local testing and quick setup:
use ez_ffmpeg::rtmp::embed_rtmp_server::EmbedRtmpServer;
use ez_ffmpeg::{FfmpegContext, Input};
fn main() -> Result<(), Box<dyn std::error::Error>> {
let mut server = EmbedRtmpServer::new("localhost:1935").start()?;
let output = server.create_rtmp_input("test-app", "test-stream")?;
let input = Input::from("video.mp4");
FfmpegContext::builder()
.input(input)
.output(output)
.build()?
.start()?
.wait()?;
Ok(())
}
Clients can access the stream using the URL:
rtmp://localhost:1935/test-app/test-stream
Conclusion
By leveraging Rust and the ez-ffmpeg library, developers can easily and safely implement RTMP streaming applications. Whether targeting public platforms or setting up local streaming environments, this approach makes development faster and more reliable.