HashCode() in Java
` The hashCode() method is a fundamental part of Java's object model, defined in the Object class. It returns an integer value that represents the object's hash code, which is used primarily in hash-based collections like HashMap, HashSet, and Hashtable. Key Characteristics Default Implementation: The Object class provides a default implementation that typically converts the memory address of the object into an integer. Contract with equals(): If two objects are equal according to equals(), they must have the same hash code. Consistency: The hash code should remain consistent across multiple invocations on the same object (unless the object is modified in ways that affect equality comparisons). Performance: Hash codes should distribute objects uniformly across hash buckets for optimal performance in collections. Basic Usage `java public class Person { private String name; private int age; // Constructor, getters, setters @Override public int hashCode() { return Objects.hash(name, age); } @Override public boolean equals(Object o) { if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; Person person = (Person) o; return age == person.age && Objects.equals(name, person.name); } } ` Best Practices for Implementing hashCode() Use same fields as equals(): The fields used in equals() must be used in hashCode(). Use Objects.hash(): For simpler implementations (Java 7+). Cache hash codes: For immutable objects, you can calculate and cache the hash code. Avoid complex computations: Keep hash code computation efficient. Common Implementation Approaches Java 7+ (Recommended) java @Override public int hashCode() { return Objects.hash(field1, field2, field3); } Traditional Implementation java @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + ((field1 == null) ? 0 : field1.hashCode()); result = prime * result + ((field2 == null) ? 0 : field2.hashCode()); result = prime * result + field3; return result; } Important Rules Consistency: Multiple invocations of hashCode() on the same object must return the same value (unless object is modified). Equality implication: If a.equals(b) is true, then a.hashCode() must equal b.hashCode(). Reverse not required: Equal hash codes don't necessarily mean objects are equal (hash collisions are allowed). Example with Collections `java Map personMap = new HashMap(); Person p1 = new Person("Alice", 30); Person p2 = new Person("Bob", 25); personMap.put(p1, "Engineer"); personMap.put(p2, "Doctor"); // Retrieval works based on hashCode() and equals() String job = personMap.get(new Person("Alice", 30)); // Returns "Engineer" ` Why is hashCode() Important? Critical for performance in hash-based collections Helps distribute objects evenly across buckets Enables efficient object retrieval from collections Required for proper functioning of HashSet, HashMap, etc. Remember to always override hashCode() when you override equals() to maintain the contract between these methods.
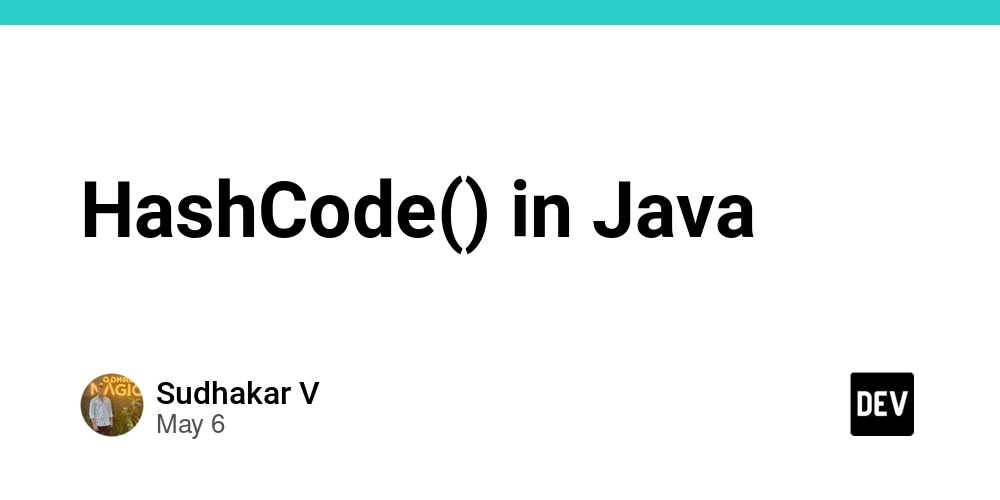
`
The hashCode()
method is a fundamental part of Java's object model, defined in the Object
class. It returns an integer value that represents the object's hash code, which is used primarily in hash-based collections like HashMap
, HashSet
, and Hashtable
.
Key Characteristics
-
Default Implementation: The
Object
class provides a default implementation that typically converts the memory address of the object into an integer. -
Contract with
equals()
: If two objects are equal according toequals()
, they must have the same hash code. - Consistency: The hash code should remain consistent across multiple invocations on the same object (unless the object is modified in ways that affect equality comparisons).
- Performance: Hash codes should distribute objects uniformly across hash buckets for optimal performance in collections.
Basic Usage
`java
public class Person {
private String name;
private int age;
// Constructor, getters, setters
@Override
public int hashCode() {
return Objects.hash(name, age);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
return age == person.age && Objects.equals(name, person.name);
}
}
`
Best Practices for Implementing hashCode()
-
Use same fields as
equals()
: The fields used inequals()
must be used inhashCode()
. -
Use
Objects.hash()
: For simpler implementations (Java 7+). - Cache hash codes: For immutable objects, you can calculate and cache the hash code.
- Avoid complex computations: Keep hash code computation efficient.
Common Implementation Approaches
Java 7+ (Recommended)
java
@Override
public int hashCode() {
return Objects.hash(field1, field2, field3);
}
Traditional Implementation
java
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((field1 == null) ? 0 : field1.hashCode());
result = prime * result + ((field2 == null) ? 0 : field2.hashCode());
result = prime * result + field3;
return result;
}
Important Rules
-
Consistency: Multiple invocations of
hashCode()
on the same object must return the same value (unless object is modified). -
Equality implication: If
a.equals(b)
istrue
, thena.hashCode()
must equalb.hashCode()
. - Reverse not required: Equal hash codes don't necessarily mean objects are equal (hash collisions are allowed).
Example with Collections
`java
Map personMap = new HashMap<>();
Person p1 = new Person("Alice", 30);
Person p2 = new Person("Bob", 25);
personMap.put(p1, "Engineer");
personMap.put(p2, "Doctor");
// Retrieval works based on hashCode() and equals()
String job = personMap.get(new Person("Alice", 30)); // Returns "Engineer"
`
Why is hashCode()
Important?
- Critical for performance in hash-based collections
- Helps distribute objects evenly across buckets
- Enables efficient object retrieval from collections
- Required for proper functioning of
HashSet
,HashMap
, etc.
Remember to always override hashCode()
when you override equals()
to maintain the contract between these methods.