Full Stack Tips for Senior Software Engineers
As a Senior Software Engineer, you're expected to have a deep understanding of both frontend and backend development, along with system design, DevOps, and best practices. Whether you're mentoring junior developers, optimizing performance, or making architectural decisions, your role is critical in building scalable and maintainable applications. Here are some full-stack tips to help you excel in your role: 1. Master Both Frontend & Backend Deeply Frontend: Stay updated with modern frameworks (React, Vue, Angular) and understand core JavaScript deeply. Backend: Know how to design RESTful/GraphQL APIs, handle authentication, and optimize database queries. Full-Stack Synergy: Understand how frontend and backend interact—avoid over-fetching data, optimize API calls, and use efficient state management. Tip: Learn how backend decisions (like caching strategies) impact frontend performance. 2. Optimize Performance at Every Level Frontend: Lazy-load components, optimize images, reduce bundle size, and use efficient state management. Backend: Implement caching (Redis), database indexing, query optimization, and connection pooling. DevOps: Use CDNs, enable Gzip/Brotli compression, and leverage HTTP/2 for faster load times. Example: // Bad: Fetching all data when only some is needed const data = await fetchAllUsers(); // Better: Pagination or query filtering const data = await fetchUsers({ limit: 10, offset: 0 }); 3. Security Should Be a Priority Frontend: Sanitize user inputs, prevent XSS attacks, and use CSP headers. Backend: Validate requests, use parameterized queries to prevent SQL injection, and enforce rate limiting. Auth: Implement JWT/OAuth securely (store tokens properly, use HTTPS, and rotate secrets). Pro Tip: Always hash passwords (bcrypt/scrypt) and never log sensitive data. 4. Write Clean, Maintainable, and Testable Code Follow SOLID principles and DRY (Don’t Repeat Yourself). Use TypeScript to catch errors early and improve code readability. Write unit tests (Jest, Mocha) and integration tests (Cypress, Postman). Example: // Bad: Any type defeats TypeScript's purpose function getUser(id: any) { ... } // Better: Explicit types function getUser(id: string): Promise { ... } 5. Embrace DevOps & CI/CD Automate deployments using GitHub Actions, GitLab CI, or Jenkins. Use Docker for containerization and Kubernetes for orchestration (if needed). Monitor apps with Prometheus, Grafana, or New Relic. Tip: Infrastructure as Code (IaC) tools like Terraform can help manage cloud resources efficiently. 6. Design Scalable Systems Database: Choose SQL (PostgreSQL) vs. NoSQL (MongoDB) based on use case. Caching: Use Redis/Memcached for frequent queries. Microservices vs. Monolith: Evaluate tradeoffs before splitting services. Pro Tip: Learn about Event-Driven Architecture (Kafka, RabbitMQ) for decoupled systems. 7. Mentor & Collaborate Effectively Conduct code reviews with constructive feedback. Document decisions (ADR - Architecture Decision Records). Encourage pair programming to share knowledge. Final Thoughts Being a Senior Full-Stack Engineer isn’t just about coding—it’s about architecture, optimization, security, and leadership. Keep learning, stay curious, and share your knowledge with the team! What are your top full-stack tips? Drop them in the comments!
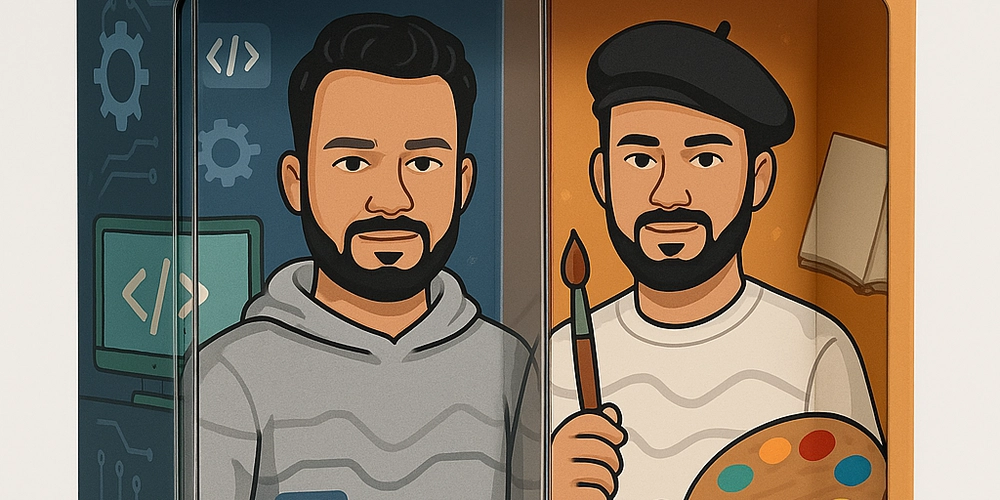
As a Senior Software Engineer, you're expected to have a deep understanding of both frontend and backend development, along with system design, DevOps, and best practices. Whether you're mentoring junior developers, optimizing performance, or making architectural decisions, your role is critical in building scalable and maintainable applications.
Here are some full-stack tips to help you excel in your role:
1. Master Both Frontend & Backend Deeply
- Frontend: Stay updated with modern frameworks (React, Vue, Angular) and understand core JavaScript deeply.
- Backend: Know how to design RESTful/GraphQL APIs, handle authentication, and optimize database queries.
- Full-Stack Synergy: Understand how frontend and backend interact—avoid over-fetching data, optimize API calls, and use efficient state management.
Tip: Learn how backend decisions (like caching strategies) impact frontend performance.
2. Optimize Performance at Every Level
- Frontend: Lazy-load components, optimize images, reduce bundle size, and use efficient state management.
- Backend: Implement caching (Redis), database indexing, query optimization, and connection pooling.
- DevOps: Use CDNs, enable Gzip/Brotli compression, and leverage HTTP/2 for faster load times.
Example:
// Bad: Fetching all data when only some is needed
const data = await fetchAllUsers();
// Better: Pagination or query filtering
const data = await fetchUsers({ limit: 10, offset: 0 });
3. Security Should Be a Priority
- Frontend: Sanitize user inputs, prevent XSS attacks, and use CSP headers.
- Backend: Validate requests, use parameterized queries to prevent SQL injection, and enforce rate limiting.
- Auth: Implement JWT/OAuth securely (store tokens properly, use HTTPS, and rotate secrets).
Pro Tip: Always hash passwords (bcrypt/scrypt) and never log sensitive data.
4. Write Clean, Maintainable, and Testable Code
- Follow SOLID principles and DRY (Don’t Repeat Yourself).
- Use TypeScript to catch errors early and improve code readability.
- Write unit tests (Jest, Mocha) and integration tests (Cypress, Postman).
Example:
// Bad: Any type defeats TypeScript's purpose
function getUser(id: any) { ... }
// Better: Explicit types
function getUser(id: string): Promise<User> { ... }
5. Embrace DevOps & CI/CD
- Automate deployments using GitHub Actions, GitLab CI, or Jenkins.
- Use Docker for containerization and Kubernetes for orchestration (if needed).
- Monitor apps with Prometheus, Grafana, or New Relic.
Tip: Infrastructure as Code (IaC) tools like Terraform can help manage cloud resources efficiently.
6. Design Scalable Systems
- Database: Choose SQL (PostgreSQL) vs. NoSQL (MongoDB) based on use case.
- Caching: Use Redis/Memcached for frequent queries.
- Microservices vs. Monolith: Evaluate tradeoffs before splitting services.
Pro Tip: Learn about Event-Driven Architecture (Kafka, RabbitMQ) for decoupled systems.
7. Mentor & Collaborate Effectively
- Conduct code reviews with constructive feedback.
- Document decisions (ADR - Architecture Decision Records).
- Encourage pair programming to share knowledge.
Final Thoughts
Being a Senior Full-Stack Engineer isn’t just about coding—it’s about architecture, optimization, security, and leadership. Keep learning, stay curious, and share your knowledge with the team!
What are your top full-stack tips? Drop them in the comments!