The Issue While developing my CampusX project, I built a custom error-handling class, ApiError, to standardize error messages. ✅ Errors were thrown properly in the backend. ❌ But on the frontend, I received an HTML error page instead of a JSON response. This made it impossible to handle errors correctly in my React app. The Root Cause By default, Express sends HTML error pages when an unhandled error occurs. Example: app.get("/error-route", (req, res) => { throw new Error("Something went wrong"); }); Frontend response (❌ Incorrect) Error Internal Server Error I needed a way to always return JSON responses for errors. The Fix – Creating a Custom API Error Handler ✅ Step 1: Create an ApiError Class I created a reusable error class for consistent error messages: class ApiError extends Error { constructor(statusCode, message) { super(message); this.statusCode = statusCode; } } module.exports = ApiError; Now, I could throw errors like this: const ApiError = require("./ApiError"); app.get("/error-route", (req, res) => { throw new ApiError(400, "Invalid Request"); }); ✅ Step 2: Create a Global Error Middleware To ensure Express sends JSON responses for errors, I added this middleware: const errorHandler = (err, req, res, next) => { const statusCode = err.statusCode || 500; return res.status(statusCode).json({ success: false, message: err.message || "Internal Server Error", }); }; // Register this middleware at the end of all routes app.use(errorHandler); Now, API Errors Return JSON Frontend response (✅ Correct) { "success": false, "message": "Invalid Request" } No more HTML errors—now the frontend gets a structured JSON response that can be handled properly!
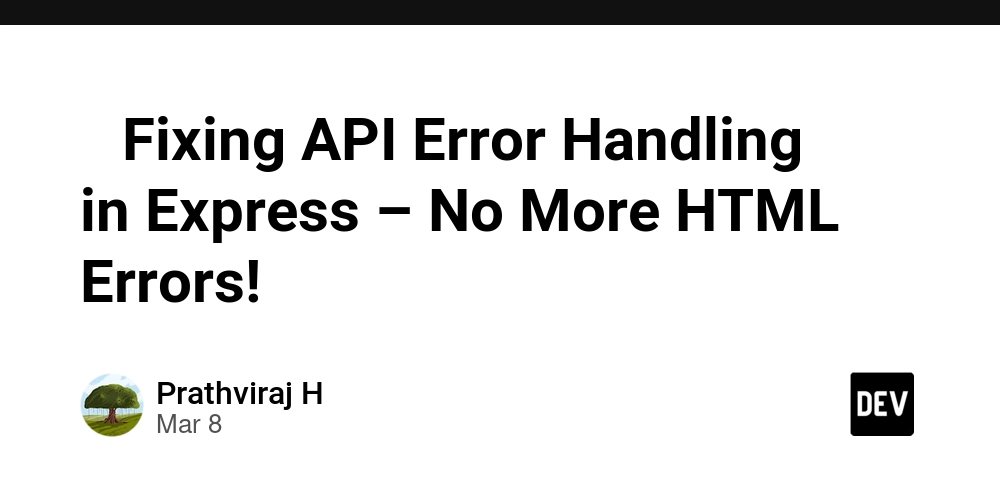
The Issue
While developing my CampusX project, I built a custom error-handling class, ApiError, to standardize error messages.
✅ Errors were thrown properly in the backend.
❌ But on the frontend, I received an HTML error page instead of a JSON response.
This made it impossible to handle errors correctly in my React app.
The Root Cause
By default, Express sends HTML error pages when an unhandled error occurs.
Example:
app.get("/error-route", (req, res) => {
throw new Error("Something went wrong");
});
Frontend response (❌ Incorrect)
Error
Internal Server Error
I needed a way to always return JSON responses for errors.
The Fix – Creating a Custom API Error Handler
✅ Step 1: Create an ApiError Class
I created a reusable error class for consistent error messages:
class ApiError extends Error {
constructor(statusCode, message) {
super(message);
this.statusCode = statusCode;
}
}
module.exports = ApiError;
Now, I could throw errors like this:
const ApiError = require("./ApiError");
app.get("/error-route", (req, res) => {
throw new ApiError(400, "Invalid Request");
});
✅ Step 2: Create a Global Error Middleware
To ensure Express sends JSON responses for errors, I added this middleware:
const errorHandler = (err, req, res, next) => {
const statusCode = err.statusCode || 500;
return res.status(statusCode).json({
success: false,
message: err.message || "Internal Server Error",
});
};
// Register this middleware at the end of all routes
app.use(errorHandler);
Now, API Errors Return JSON
Frontend response (✅ Correct)
{
"success": false,
"message": "Invalid Request"
}
No more HTML errors—now the frontend gets a structured JSON response that can be handled properly!