Building a TypeScript Blog API: A Beginner's Guide
When I decided to build a blog API using TypeScript, I discovered how much typed programming can transform web development. Let me walk you through this project that helped me understand the power of TypeScript in creating robust web services. The Problem I Needed to Solve As a web developer looking to improve my portfolio, I wanted to create a RESTful API that could handle blog content efficiently. Many content management systems felt too bloated for my needs, and I wanted something lightweight that I could fully understand and customize. Understanding the Core: The Post Interface At the heart of my blog API is the Post interface - essentially a blueprint that defines exactly what a blog post should look like. This interface ensures that every post in our system has consistent structure, making our code more predictable and less prone to errors. The interface defines properties like id, title, content, author, and timestamps that track when posts are created and updated. Building the API Endpoints I implemented four essential operations (CRUD) that any blog needs: Create - Adding new blog posts Read - Fetching all posts or finding specific ones Update - Modifying existing content Delete - Removing unwanted posts A Practical Example To understand how this works in practice, consider how a content creator might use this API: When creating a new recipe post, they would send data including the title, content, and their name as the author. The API would automatically generate a unique ID and add timestamps. If they later discover a mistake in the instructions, they can update just the content field. The API would keep the original creation date but update the "updatedAt" timestamp to reflect the changes. When they want to see all their published work, a simple API call retrieves all posts, properly formatted according to our Post interface. The Benefits of TypeScript in This Project The most valuable aspect of using TypeScript was catching errors before they happened. For instance, if I accidentally tried to submit a post without a required field, the TypeScript compiler would flag this immediately rather than causing mysterious runtime errors. When retrieving post data, the type system ensured I always received correctly formatted data, making front-end development work much more reliable. What I Learned Creating this project taught me how TypeScript can transform API development: Type Safety: Prevents common errors like sending malformed data to the database Code Clarity: Anyone looking at the code can immediately understand what a Post object contains Better Collaboration: When other developers join the project, they can quickly understand how data flows through the system Easier Maintenance: When adding new features later, TypeScript guides developers to update all the necessary code Conclusion Building this Blog API with TypeScript taught me the importance of well-defined data structures in web development. The type safety provided clear guardrails for my code, making debugging easier and preventing many common issues before they occurred. For beginners looking to build something similar, remember that TypeScript's initial learning curve pays off quickly with more reliable code and fewer bugs in production. Start small with interfaces, and gradually build up the complexity of your API as your needs grow.
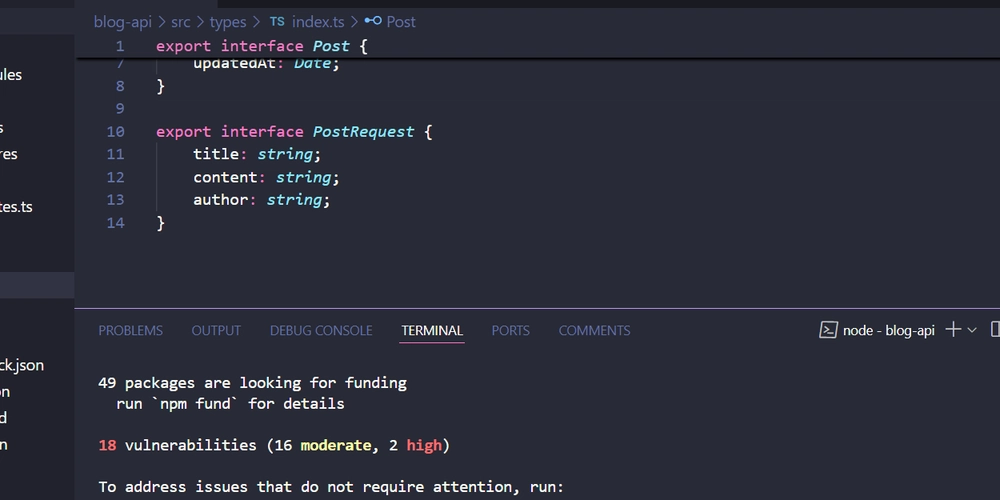
When I decided to build a blog API using TypeScript, I discovered how much typed programming can transform web development. Let me walk you through this project that helped me understand the power of TypeScript in creating robust web services.
The Problem I Needed to Solve
As a web developer looking to improve my portfolio, I wanted to create a RESTful API that could handle blog content efficiently. Many content management systems felt too bloated for my needs, and I wanted something lightweight that I could fully understand and customize.
Understanding the Core: The Post Interface
At the heart of my blog API is the Post interface - essentially a blueprint that defines exactly what a blog post should look like. This interface ensures that every post in our system has consistent structure, making our code more predictable and less prone to errors.
The interface defines properties like id, title, content, author, and timestamps that track when posts are created and updated.
Building the API Endpoints
I implemented four essential operations (CRUD) that any blog needs:
- Create - Adding new blog posts
- Read - Fetching all posts or finding specific ones
- Update - Modifying existing content
- Delete - Removing unwanted posts
A Practical Example
To understand how this works in practice, consider how a content creator might use this API:
When creating a new recipe post, they would send data including the title, content, and their name as the author. The API would automatically generate a unique ID and add timestamps.
If they later discover a mistake in the instructions, they can update just the content field. The API would keep the original creation date but update the "updatedAt" timestamp to reflect the changes.
When they want to see all their published work, a simple API call retrieves all posts, properly formatted according to our Post interface.
The Benefits of TypeScript in This Project
The most valuable aspect of using TypeScript was catching errors before they happened. For instance, if I accidentally tried to submit a post without a required field, the TypeScript compiler would flag this immediately rather than causing mysterious runtime errors.
When retrieving post data, the type system ensured I always received correctly formatted data, making front-end development work much more reliable.
What I Learned
Creating this project taught me how TypeScript can transform API development:
- Type Safety: Prevents common errors like sending malformed data to the database
- Code Clarity: Anyone looking at the code can immediately understand what a Post object contains
- Better Collaboration: When other developers join the project, they can quickly understand how data flows through the system
- Easier Maintenance: When adding new features later, TypeScript guides developers to update all the necessary code
Conclusion
Building this Blog API with TypeScript taught me the importance of well-defined data structures in web development. The type safety provided clear guardrails for my code, making debugging easier and preventing many common issues before they occurred.
For beginners looking to build something similar, remember that TypeScript's initial learning curve pays off quickly with more reliable code and fewer bugs in production. Start small with interfaces, and gradually build up the complexity of your API as your needs grow.