Before You Code: A Senior Frontend Dev’s Thought Process
Good code is not just about making things work—it's about making things work well, together. When starting a new feature, junior devs often jump into coding. Senior devs pause to think first. In this post, I’ll walk you through a mental checklist I use before writing a single line of code—something I’ve honed over years of building frontend features in React, Next.js, and beyond. 1. Understand the Feature Before writing JSX... What does this component need to do? Are there dynamic behaviors like modals, dropdowns, and toggles? Will it fetch data? From where? How often? Can I reuse existing hooks, utilities, or services? Think: What problem am I solving, and has someone already solved parts of it? 2. Map the Data Flow Clarity here avoids spaghetti later. What are the inputs? (props, query params, context) What outputs or events does it trigger? (onClick, onChange) How will data flow across components? Do I need a wrapper or a layout component? Think: Can a future teammate trace this flow in 30 seconds? 3. Choose the Right State Strategy Every piece of state needs a home. Local (useState) or lifted to a parent? Global (Zustand, Redux, Context) if shared across features? Should it persist? (e.g., localStorage) Does it affect multiple components or pages? Think: Am I overengineering or under-planning the state? 4. Design for Reusability Avoid copy-pasting tomorrow what you hardcode today. Can this component or part of it be reused? Should I make it generic (e.g., Dropdown) or contextual (e.g., UserDropdown)? Do we already have something similar I can tweak? Should this logic live in a custom hook or utility? Think: Will I regret hardcoding this next week? 5. Don’t Ignore Performance Bad UX often hides behind slow code. Are we dealing with large datasets, rapid user input, or complex visuals? Use debounce, memoization, or virtualization where needed. Can I break this into smaller pieces to reduce re-renders? Are there animations or blocking tasks that need concurrency? Think: Will this slow down on low-end devices? 6. Plan for Failure (Because It Will) A broken UI is a bad experience. Silence is worse. What happens if the API fails? Or returns no data? Do we need retries? Fallbacks? Should we use ErrorBoundary, Suspense, or manual error states? How do we inform the user and log the issue? Think: Can this fail gracefully? Final Thoughts The goal isn’t to overthink—it’s to pre-think. Spending 10–15 minutes planning a feature can save hours of refactoring, debugging, and technical debt.
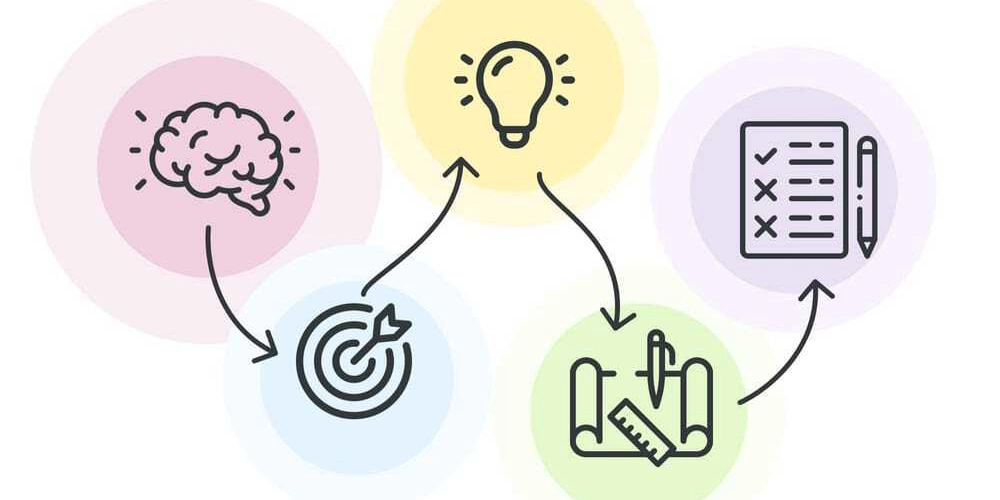
Good code is not just about making things work—it's about making things work well, together.
When starting a new feature, junior devs often jump into coding. Senior devs pause to think first.
In this post, I’ll walk you through a mental checklist I use before writing a single line of code—something I’ve honed over years of building frontend features in React, Next.js, and beyond.
1. Understand the Feature
Before writing JSX...
- What does this component need to do?
- Are there dynamic behaviors like modals, dropdowns, and toggles?
- Will it fetch data? From where? How often?
- Can I reuse existing hooks, utilities, or services?
Think: What problem am I solving, and has someone already solved parts of it?
2. Map the Data Flow
Clarity here avoids spaghetti later.
- What are the inputs? (props, query params, context)
- What outputs or events does it trigger? (onClick, onChange)
- How will data flow across components?
- Do I need a wrapper or a layout component?
Think: Can a future teammate trace this flow in 30 seconds?
3. Choose the Right State Strategy
Every piece of state needs a home.
- Local (useState) or lifted to a parent?
- Global (Zustand, Redux, Context) if shared across features?
- Should it persist? (e.g., localStorage)
- Does it affect multiple components or pages?
Think: Am I overengineering or under-planning the state?
4. Design for Reusability
Avoid copy-pasting tomorrow what you hardcode today.
- Can this component or part of it be reused?
- Should I make it generic (e.g., Dropdown) or contextual (e.g., UserDropdown)?
- Do we already have something similar I can tweak?
- Should this logic live in a custom hook or utility?
Think: Will I regret hardcoding this next week?
5. Don’t Ignore Performance
Bad UX often hides behind slow code.
- Are we dealing with large datasets, rapid user input, or complex visuals?
- Use debounce, memoization, or virtualization where needed.
- Can I break this into smaller pieces to reduce re-renders?
- Are there animations or blocking tasks that need concurrency?
Think: Will this slow down on low-end devices?
6. Plan for Failure (Because It Will)
A broken UI is a bad experience. Silence is worse.
- What happens if the API fails? Or returns no data?
- Do we need retries? Fallbacks?
- Should we use ErrorBoundary, Suspense, or manual error states?
- How do we inform the user and log the issue?
Think: Can this fail gracefully?
Final Thoughts
The goal isn’t to overthink—it’s to pre-think.
Spending 10–15 minutes planning a feature can save hours of refactoring, debugging, and technical debt.