A Beginner’s Guide to Terraform on AWS
Introduction Terraform, developed by HashiCorp, is an Infrastructure as Code (IaC) tool that allows you to define and manage your infrastructure using easy-to-read, declarative configuration files. It automates the entire lifecycle of your infrastructure—from provisioning to updates and teardown. Why Use Terraform for AWS? Repeatable and consistent environments Trackable via Version control for infrastructure Automated infrastructure setup Prerequisites Terraform Installed: You need to install Terraform before you can start using it. Refer to the official Terraform documentation for detailed installation steps, whether you're using a package manager or installing manually based on your operating system. The AWS CLI installed: Check the official AWS CLI installation guide for step-by-step instructions tailored to your operating system. Getting Started A. Create AWS account and associated credentials If you don't already have one, create an AWS account, this serves as your entry point to Amazon Web Services, where you can manage cloud resources such as servers, storage, databases e.t.c for your applications. The associated credentials enable you to create and manage resources within your account. B. Steps to Create AWS Credentials for Terraform Login to AWS Console: Access the AWS Management Console and search for the IAM service. Create a New User: Click on Users in the IAM dashboard. Click the Create user button and enter the required user details. Configure User Access: Uncheck the option "Provide user access to the AWS Management Console" (this is not needed because Terraform is managed via code, not the UI). Set Permissions: Click Next: To set user permissions. For testing, assign the "AdministratorAccess" policy to the user. Review and Create User: Click Next: To review your configuration. Click the Create user button to create the Terraform user. Access Keys Configuration: Once the user is created, click on the username. Scroll down to the Access keys section. Click Create access key. Select Use Case: In the pop-up, choose Command Line Interface (CLI) as the use case. Check the confirmation box and click Next. Set Description and Create Key: Optionally, set a description tag for the access key. Click Create access key to generate the credentials. Store Access Keys Securely: Copy the Access Key and Secret Key immediately. Store them securely — this is the only time you can view or download the secret access key. If you lose it, you will need to create a new one. C. Configure AWS CLI Open a terminal and run the command aws configure. When prompted, enter the IAM user's Access Key ID and Secret Access Key that were generated in the previous step. Additionally, specify your default region (e.g., us-east-1) and output format (e.g., json). Note: Always protect your access key and secret key, and periodically rotate them to minimize the risk of compromise. D. Create a basic Terraform script Now that our AWS account is set up and the CLI is configured, we can start working with Terraform to define our infrastructure. The files used to describe infrastructure in Terraform are called "Terraform configurations." To get started, create a dedicated directory for your project, as each configuration must reside in its own working directory. This directory will hold all the necessary configuration files. mkdir terraform-aws Change into the directory cd terraform-aws Create a main.tf file and paste in the configuration below. terraform { required_providers { aws = { source = "hashicorp/aws" version = "~> 5.0" } } required_version = ">= 1.4.0" } # Configure the AWS Provider provider "aws" { region = "us-east-1" } resource "aws_instance" "app_server" { ami = "ami-05238ab1443fdf48f" #Substitute this with the AMI ID you wish to use. instance_type = "t2.micro" tags = { Name = "MyServerInstance" } } This Terraform code sets up an AWS EC2 instance in the us-east-1 region, using the specified AMI (ami-05238ab1443fdf48f) and a t2.micro instance type, with a tag called MyServerInstance. It also specifies that Terraform version 1.4.0 or higher and AWS provider version 5.x.x are required. E. Initialize, Plan, and Apply: When you create or check out a configuration, run the following commands; terraform init: Initializes the working directory and downloads the required providers (e.g., AWS). $ terraform init _Initializing the backend... Initializing provider plugins... - Finding hashicorp/aws versions matching "~> 5.0"... - Installing hashicorp/aws v5.94.1... - Installed hashicorp/aws v5.94.1 (signed by HashiCorp) Terraform has created a lock file .terraform.lock.hcl to record the provider selections it made above. Include this f
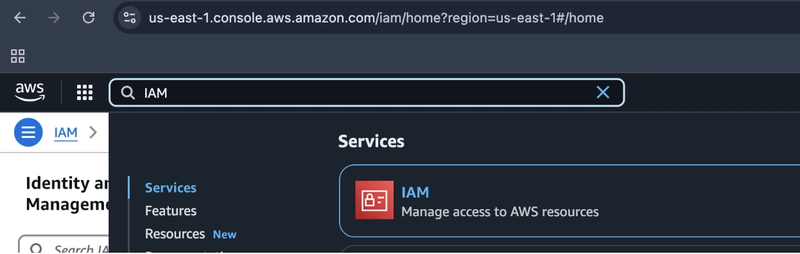
Introduction
Terraform, developed by HashiCorp, is an Infrastructure as Code (IaC) tool that allows you to define and manage your infrastructure using easy-to-read, declarative configuration files. It automates the entire lifecycle of your infrastructure—from provisioning to updates and teardown.
Why Use Terraform for AWS?
- Repeatable and consistent environments
- Trackable via Version control for infrastructure
- Automated infrastructure setup
Prerequisites
- Terraform Installed: You need to install Terraform before you can start using it. Refer to the official Terraform documentation for detailed installation steps, whether you're using a package manager or installing manually based on your operating system.
- The AWS CLI installed: Check the official AWS CLI installation guide for step-by-step instructions tailored to your operating system.
Getting Started
A. Create AWS account and associated credentials
If you don't already have one, create an AWS account, this serves as your entry point to Amazon Web Services, where you can manage cloud resources such as servers, storage, databases e.t.c for your applications. The associated credentials enable you to create and manage resources within your account.
B. Steps to Create AWS Credentials for Terraform
-
Login to AWS Console:
- Access the AWS Management Console and search for the IAM service.
-
Create a New User:
- Click on Users in the IAM dashboard.
- Click the Create user button and enter the required user details.
-
Configure User Access:
- Uncheck the option "Provide user access to the AWS Management Console" (this is not needed because Terraform is managed via code, not the UI).
-
Set Permissions:
- Click Next: To set user permissions.
- For testing, assign the "AdministratorAccess" policy to the user.
-
Review and Create User:
- Click Next: To review your configuration.
- Click the Create user button to create the Terraform user.
-
Access Keys Configuration:
- Once the user is created, click on the username.
- Scroll down to the Access keys section.
- Click Create access key.
-
Select Use Case:
- In the pop-up, choose Command Line Interface (CLI) as the use case.
- Check the confirmation box and click Next.
-
Set Description and Create Key:
- Optionally, set a description tag for the access key.
- Click Create access key to generate the credentials.
-
Store Access Keys Securely:
- Copy the Access Key and Secret Key immediately.
- Store them securely — this is the only time you can view or download the secret access key. If you lose it, you will need to create a new one.
C. Configure AWS CLI
Open a terminal and run the command aws configure
. When prompted, enter the IAM user's Access Key ID and Secret Access Key that were generated in the previous step. Additionally, specify your default region (e.g., us-east-1
) and output format (e.g., json
).
Note: Always protect your access key and secret key, and periodically rotate them to minimize the risk of compromise.
D. Create a basic Terraform script
Now that our AWS account is set up and the CLI is configured, we can start working with Terraform to define our infrastructure. The files used to describe infrastructure in Terraform are called "Terraform configurations."
- To get started, create a dedicated directory for your project, as each configuration must reside in its own working directory. This directory will hold all the necessary configuration files.
mkdir terraform-aws
- Change into the directory
cd terraform-aws
- Create a main.tf file and paste in the configuration below.
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "~> 5.0"
}
}
required_version = ">= 1.4.0"
}
# Configure the AWS Provider
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "app_server" {
ami = "ami-05238ab1443fdf48f" #Substitute this with the AMI ID you wish to use.
instance_type = "t2.micro"
tags = {
Name = "MyServerInstance"
}
}
This Terraform code sets up an AWS EC2 instance in the us-east-1
region, using the specified AMI (ami-05238ab1443fdf48f
) and a t2.micro
instance type, with a tag called MyServerInstance
. It also specifies that Terraform version 1.4.0 or higher and AWS provider version 5.x.x are required.
E. Initialize, Plan, and Apply:
When you create or check out a configuration, run the following commands;
-
terraform init
: Initializes the working directory and downloads the required providers (e.g., AWS).
$ terraform init
_Initializing the backend...
Initializing provider plugins...
- Finding hashicorp/aws versions matching "~> 5.0"...
- Installing hashicorp/aws v5.94.1...
- Installed hashicorp/aws v5.94.1 (signed by HashiCorp)
Terraform has created a lock file .terraform.lock.hcl to record the provider
selections it made above. Include this file in your version control repository
so that Terraform can guarantee to make the same selections by default when
you run "terraform init" in the future.
Terraform has been successfully initialized!
You may now begin working with Terraform. Try running "terraform plan" to see
any changes that are required for your infrastructure. All Terraform commands
should now work.
If you ever set or change modules or backend configuration for Terraform,
rerun this command to reinitialize your working directory. If you forget, other
commands will detect it and remind you to do so if necessary._
-
terraform fmt
: Automatically formats configurations for improved readability. -
terraform validate
: Checks that the configuration is syntactically correct and internally consistent.
_$ terraform validate
Success! The configuration is valid._
-
terraform plan
: Generates an execution plan, describing the actions Terraform will take to modify the infrastructure. It shows you what will happen but doesn't make any changes. I've shortened some of the output for brevity.
$ terraform plan
Terraform used the selected providers to generate the following execution plan. Resource actions are indicated with the following symbols:
+ create
Terraform will perform the following actions:
# aws_instance.app_server will be created
+ resource "aws_instance" "app_server" {
+ ami = "ami-05238ab1443fdf48f"
+ arn = (known after apply)
+ associate_public_ip_address = (known after apply)
##...
Plan: 1 to add, 0 to change, 0 to destroy.
-
terraform apply
: Applies the changes to your infrastructure based on the plan. It will ask for confirmation (e.g., typing "yes") before proceeding. I've shortened some of the output for brevity.
_$ terraform apply
##...
Plan: 1 to add, 0 to change, 0 to destroy.
Do you want to perform these actions?
Terraform will perform the actions described above.
Only 'yes' will be accepted to approve.
Enter a value:_
- Post-Execution: Once terraform apply completes, check the EC2 console for the newly created instance.
-
terraform destroy
: Destroys all the infrastructure managed by the Terraform configuration. It will prompt for confirmation before deleting the resources, ensuring you can safely tear down the setup when no longer needed.
Conclusion
Using Terraform for Infrastructure as Code (IaC) on AWS simplifies and automates the provisioning and management of cloud resources. By defining your infrastructure in configuration files, you ensure consistency, repeatability, and version control. With Terraform's tools—like terraform init
, terraform plan
, terraform apply
, and terraform destroy
— you can easily create, modify, and delete resources, making it an essential tool for modern DevOps practices. Whether you’re just starting or scaling your infrastructure, Terraform helps streamline the process and enhances your ability to manage cloud environments efficiently.