Real-time Like Notifications with Redis Pub/Sub in CampusX
Introduction Handling real-time events efficiently is crucial in any social media-like application. In CampusX, I implemented Redis Pub/Sub to manage like notifications seamlessly. This approach ensures that when a user likes a post, the owner of the post receives an instant notification via WebSockets. This blog will break down how Redis Pub/Sub works and how it powers the real-time like notifications in CampusX. What is Redis Pub/Sub? Redis Pub/Sub (Publish/Subscribe) is a messaging system where publishers send messages to specific channels, and subscribers listening to those channels receive the messages instantly. Unlike traditional message queues, Redis Pub/Sub does not store messages. Once a message is delivered to all subscribers, it disappears from memory. Key Components: Publisher: Sends messages to a Redis channel. Subscriber: Listens for messages on a channel. Channel: A topic where messages are broadcasted. Setting Up Redis Pub/Sub for Like Notifications in CampusX 1️⃣ Configuring Redis Client First, I created a Redis configuration file (redis.js) to manage the publisher and subscriber connections: import { createClient } from "redis"; const pub = createClient(); const sub = createClient(); pub.on("error", (err) => console.error("❌ Redis Publisher Error:", err)); sub.on("error", (err) => console.error("❌ Redis Subscriber Error:", err)); async function connectRedis() { try { await pub.connect(); console.log("✅ Redis Publisher Connected"); await sub.connect(); console.log("✅ Redis Subscriber Connected"); } catch (err) { console.error("❌ Redis Connection Error:", err); } } connectRedis(); export { pub, sub }; This ensures that both the publisher and subscriber are connected when the backend starts. 2️⃣ Publishing Like Events Whenever a user likes a post, the backend publishes a message on the like_status channel: import { pub } from "../config/redis.js"; async function publishLikeStatus(status, postOwnerId, userId) { console.log("Published Like Status"); if (!pub.isReady) { console.log("
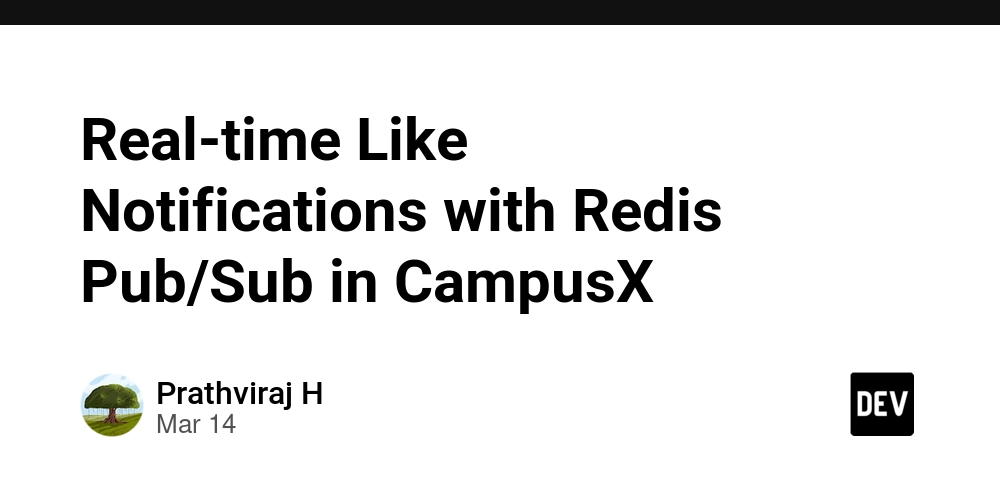
Introduction
Handling real-time events efficiently is crucial in any social media-like application. In CampusX, I implemented Redis Pub/Sub to manage like notifications seamlessly. This approach ensures that when a user likes a post, the owner of the post receives an instant notification via WebSockets.
This blog will break down how Redis Pub/Sub works and how it powers the real-time like notifications in CampusX.
What is Redis Pub/Sub?
Redis Pub/Sub (Publish/Subscribe) is a messaging system where publishers send messages to specific channels, and subscribers listening to those channels receive the messages instantly. Unlike traditional message queues, Redis Pub/Sub does not store messages. Once a message is delivered to all subscribers, it disappears from memory.
Key Components:
- Publisher: Sends messages to a Redis channel.
- Subscriber: Listens for messages on a channel.
- Channel: A topic where messages are broadcasted.
Setting Up Redis Pub/Sub for Like Notifications in CampusX
1️⃣ Configuring Redis Client
First, I created a Redis configuration file (redis.js
) to manage the publisher and subscriber connections:
import { createClient } from "redis";
const pub = createClient();
const sub = createClient();
pub.on("error", (err) => console.error("❌ Redis Publisher Error:", err));
sub.on("error", (err) => console.error("❌ Redis Subscriber Error:", err));
async function connectRedis() {
try {
await pub.connect();
console.log("✅ Redis Publisher Connected");
await sub.connect();
console.log("✅ Redis Subscriber Connected");
} catch (err) {
console.error("❌ Redis Connection Error:", err);
}
}
connectRedis();
export { pub, sub };
This ensures that both the publisher and subscriber are connected when the backend starts.
2️⃣ Publishing Like Events
Whenever a user likes a post, the backend publishes a message on the like_status
channel:
import { pub } from "../config/redis.js";
async function publishLikeStatus(status, postOwnerId, userId) {
console.log("Published Like Status");
if (!pub.isReady) {
console.log("