Node.js Event Loop: The Key to Scalable and Efficient Applications
Table of Contents What is the Node.js Event Loop? The Food Cart Analogy Why Single-Threaded? The Role of Libuv and the Thread Pool The Event Loop Phases Best Practices for Node.js Conclusion and Next Steps Mentionable Articles What is the Node.js Event Loop? The event loop is the core mechanism in Node.js that enables non-blocking I/O operations. Despite Node.js being single-threaded by default, the event loop allows it to perform asynchronous operations by offloading tasks to the system kernel or Libuv's thread pool. This makes Node.js highly efficient for I/O-heavy applications, such as web servers and APIs. The Food Cart Analogy To understand the event loop, imagine Node.js as a food cart with only one chef. This chef serves sandwiches, snacks, and drinks—small, easy-to-prepare items. While the chef is heating a sandwich in the microwave, they might also chop veggies or start frying French fries. The chef doesn't wait for one task to finish before starting another; instead, they efficiently switch between tasks to keep everything moving. This is exactly how the event loop works in Node.js! Why Single-Threaded? Just like a food cart with one chef, Node.js is designed to handle small, quick tasks efficiently. Running multiple threads (like hiring more chefs) would require more resources, such as memory and CPU. In many cases, this isn't necessary. For example, you don't need a dedicated chef just to heat a sandwich in the microwave. Similarly, Node.js doesn't need multiple threads to handle most I/O operations. However, this also means Node.js isn't ideal for CPU-intensive tasks, such as image processing or complex calculations. For these tasks, you might need to use worker threads or choose a language better suited for multithreading. The Role of Libuv and the Thread Pool Behind the scenes, Node.js relies on a library called Libuv to handle asynchronous operations. Libuv manages the event loop and provides a thread pool for operations that cannot be handled asynchronously by the operating system, such as file I/O. The term "Thread Pool" might sound confusing, but think of it like this: When our food cart chef gets overwhelmed by complicated meals, they delegate these tasks to kitchen helpers. By default, the thread pool has 4 helpers (threads) available. When a kitchen helper finishes preparing a meal, they notify the chef, who then serves it to the client. You can increase the number of kitchen helpers by setting the UV_THREADPOOL_SIZE environment variable, but keep in mind that more helpers require more resources. The Event Loop Phases The event loop consists of several phases, each responsible for handling specific types of tasks. Here, we’ll highlight the most important ones for Node.js developers: Timers: Executes setTimeout and setInterval callbacks. Pending Callbacks: Executes deferred I/O callbacks from the previous iteration. Poll: Retrieves new I/O events and executes their callbacks. Check: Executes setImmediate callbacks. Close Callbacks: Executes cleanup callbacks (e.g., socket.on('close')). Best Practices for Node.js To get the most out of Node.js, follow these best practices: Keep Operations Small and Efficient: Just like a food cart chef, Node.js excels at handling small, quick tasks. Avoid long-running or CPU-intensive operations. Use Asynchronous APIs: Always prefer asynchronous methods (e.g., fs.readFile instead of fs.readFileSync) to avoid blocking the event loop. Offload Heavy Tasks: For CPU-intensive tasks, use worker threads (available in Node.js) or external services to avoid blocking the event loop. Mentionable Articles On problems with threads in node.js https://nodejs.org/en/learn/asynchronous-work/event-loop-timers-and-nexttick#the-nodejs-event-loop Conclusion and Next Steps The event loop is what makes Node.js so powerful for I/O-heavy applications. By understanding how it works, you can write more efficient and scalable code. In the next article, we'll dive deeper into the event loop phases and answer the question: Who runs first, setTimeout(() => {}, 0) or setImmediate(() => {})? Feel free to leave feedback and ask questions in the comments section!
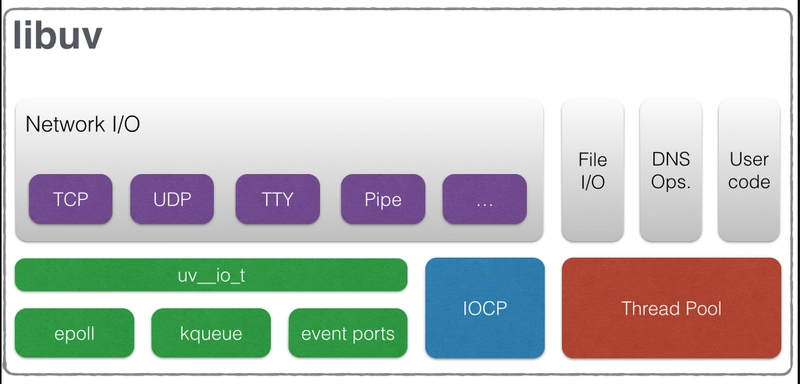
Table of Contents
- What is the Node.js Event Loop?
- The Food Cart Analogy
- Why Single-Threaded?
- The Role of Libuv and the Thread Pool
- The Event Loop Phases
- Best Practices for Node.js
- Conclusion and Next Steps
- Mentionable Articles
What is the Node.js Event Loop?
The event loop is the core mechanism in Node.js that enables non-blocking I/O operations. Despite Node.js being single-threaded by default, the event loop allows it to perform asynchronous operations by offloading tasks to the system kernel or Libuv's thread pool. This makes Node.js highly efficient for I/O-heavy applications, such as web servers and APIs.
The Food Cart Analogy
To understand the event loop, imagine Node.js as a food cart with only one chef. This chef serves sandwiches, snacks, and drinks—small, easy-to-prepare items. While the chef is heating a sandwich in the microwave, they might also chop veggies or start frying French fries. The chef doesn't wait for one task to finish before starting another; instead, they efficiently switch between tasks to keep everything moving. This is exactly how the event loop works in Node.js!
Why Single-Threaded?
Just like a food cart with one chef, Node.js is designed to handle small, quick tasks efficiently. Running multiple threads (like hiring more chefs) would require more resources, such as memory and CPU. In many cases, this isn't necessary. For example, you don't need a dedicated chef just to heat a sandwich in the microwave. Similarly, Node.js doesn't need multiple threads to handle most I/O operations.
However, this also means Node.js isn't ideal for CPU-intensive tasks, such as image processing or complex calculations. For these tasks, you might need to use worker threads or choose a language better suited for multithreading.
The Role of Libuv and the Thread Pool
Behind the scenes, Node.js relies on a library called Libuv to handle asynchronous operations. Libuv manages the event loop and provides a thread pool for operations that cannot be handled asynchronously by the operating system, such as file I/O.
The term "Thread Pool" might sound confusing, but think of it like this: When our food cart chef gets overwhelmed by complicated meals, they delegate these tasks to kitchen helpers. By default, the thread pool has 4 helpers (threads) available. When a kitchen helper finishes preparing a meal, they notify the chef, who then serves it to the client. You can increase the number of kitchen helpers by setting the UV_THREADPOOL_SIZE
environment variable, but keep in mind that more helpers require more resources.
The Event Loop Phases
The event loop consists of several phases, each responsible for handling specific types of tasks. Here, we’ll highlight the most important ones for Node.js developers:
-
Timers: Executes
setTimeout
andsetInterval
callbacks. - Pending Callbacks: Executes deferred I/O callbacks from the previous iteration.
- Poll: Retrieves new I/O events and executes their callbacks.
-
Check: Executes
setImmediate
callbacks. -
Close Callbacks: Executes cleanup callbacks (e.g.,
socket.on('close')
).
Best Practices for Node.js
To get the most out of Node.js, follow these best practices:
- Keep Operations Small and Efficient: Just like a food cart chef, Node.js excels at handling small, quick tasks. Avoid long-running or CPU-intensive operations.
-
Use Asynchronous APIs: Always prefer asynchronous methods (e.g.,
fs.readFile
instead offs.readFileSync
) to avoid blocking the event loop. - Offload Heavy Tasks: For CPU-intensive tasks, use worker threads (available in Node.js) or external services to avoid blocking the event loop.
Mentionable Articles
- On problems with threads in node.js
- https://nodejs.org/en/learn/asynchronous-work/event-loop-timers-and-nexttick#the-nodejs-event-loop
Conclusion and Next Steps
The event loop is what makes Node.js so powerful for I/O-heavy applications. By understanding how it works, you can write more efficient and scalable code. In the next article, we'll dive deeper into the event loop phases and answer the question: Who runs first, setTimeout(() => {}, 0)
or setImmediate(() => {})
?
Feel free to leave feedback and ask questions in the comments section!