Message Queues (RabbitMQ, Kafka)
Message Queues: RabbitMQ and Kafka Introduction: Message queues are essential components in distributed systems, enabling asynchronous communication between applications. They decouple services, improve scalability, and enhance resilience. Popular choices include RabbitMQ and Kafka, each with its strengths and weaknesses. Prerequisites: Understanding basic networking and software architecture concepts is helpful. Familiarity with a scripting language (e.g., Python) for interacting with the queues is beneficial. Features: Both RabbitMQ and Kafka provide features like message persistence, routing, and acknowledging message delivery. RabbitMQ excels in advanced routing capabilities using exchanges and bindings, offering features like publish/subscribe and point-to-point messaging. Kafka, on the other hand, is optimized for high-throughput streaming, handling massive volumes of data with partitioned logs and replication. Advantages: Decoupling: Services don't need to know each other's availability. Scalability: Queues can handle increasing message volume efficiently. Reliability: Message persistence ensures no data loss even in failures. Asynchronous processing: Improves responsiveness and avoids blocking. Disadvantages: Complexity: Setting up and managing message queues can be complex. Overhead: Adding a queue introduces extra latency and processing. Potential bottlenecks: Improper configuration can lead to performance issues. Example (Python with RabbitMQ): import pika connection = pika.BlockingConnection(pika.ConnectionParameters(host='localhost')) channel = connection.channel() channel.queue_declare(queue='hello') channel.basic_publish(exchange='', routing_key='hello', body='Hello World!') print(" [x] Sent 'Hello World!'") connection.close() Conclusion: RabbitMQ and Kafka are powerful tools for building robust and scalable applications. RabbitMQ's flexibility and routing capabilities suit applications needing complex message flows, while Kafka's high-throughput design is ideal for large-scale data streaming. The best choice depends on the specific requirements of your system.
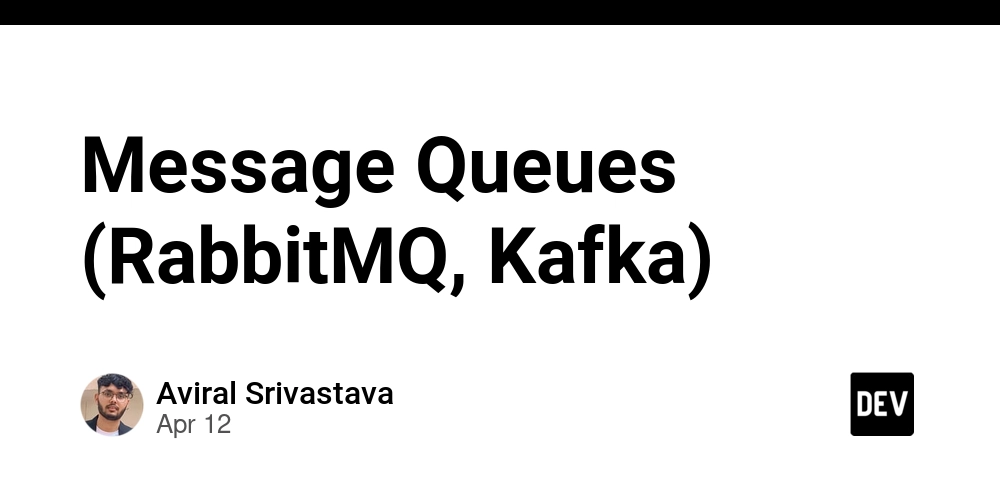
Message Queues: RabbitMQ and Kafka
Introduction:
Message queues are essential components in distributed systems, enabling asynchronous communication between applications. They decouple services, improve scalability, and enhance resilience. Popular choices include RabbitMQ and Kafka, each with its strengths and weaknesses.
Prerequisites:
Understanding basic networking and software architecture concepts is helpful. Familiarity with a scripting language (e.g., Python) for interacting with the queues is beneficial.
Features:
Both RabbitMQ and Kafka provide features like message persistence, routing, and acknowledging message delivery. RabbitMQ excels in advanced routing capabilities using exchanges and bindings, offering features like publish/subscribe and point-to-point messaging. Kafka, on the other hand, is optimized for high-throughput streaming, handling massive volumes of data with partitioned logs and replication.
Advantages:
- Decoupling: Services don't need to know each other's availability.
- Scalability: Queues can handle increasing message volume efficiently.
- Reliability: Message persistence ensures no data loss even in failures.
- Asynchronous processing: Improves responsiveness and avoids blocking.
Disadvantages:
- Complexity: Setting up and managing message queues can be complex.
- Overhead: Adding a queue introduces extra latency and processing.
- Potential bottlenecks: Improper configuration can lead to performance issues.
Example (Python with RabbitMQ):
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters(host='localhost'))
channel = connection.channel()
channel.queue_declare(queue='hello')
channel.basic_publish(exchange='', routing_key='hello', body='Hello World!')
print(" [x] Sent 'Hello World!'")
connection.close()
Conclusion:
RabbitMQ and Kafka are powerful tools for building robust and scalable applications. RabbitMQ's flexibility and routing capabilities suit applications needing complex message flows, while Kafka's high-throughput design is ideal for large-scale data streaming. The best choice depends on the specific requirements of your system.