Mastering PHP's Type Hints: A Comprehensive Guide to Writing Robust Code
PHP has evolved significantly over the years, transforming from a loosely-typed scripting language into a powerful, modern programming language with features that rival strongly-typed languages. One of the standout features introduced in PHP 5 and enhanced in PHP 7+ is type hints (also known as type declarations). Type hints allow developers to specify the expected data types for function parameters, return values, and class properties, leading to cleaner, more reliable, and maintainable code. In this extensive blog post, we’ll explore the power of PHP’s type hints, why they matter, and how to use them effectively. We’ll cover every aspect of type hints with practical code examples, best practices, and tips for integrating them into your projects. Whether you’re a beginner or a seasoned PHP developer, this guide will help you master type hints and elevate your coding game. Table of Contents What Are Type Hints? Why Use Type Hints? Key Features of PHP Type Hints Scalar Type Hints Return Type Hints Strict Typing Nullable Types Union Types Intersection Types Class and Interface Type Hints Iterable and Callable Types Void Return Type Mixed Type Best Practices for Using Type Hints Static Analysis Tools for Type Hints Common Pitfalls and How to Avoid Them Conclusion What Are Type Hints? Type hints in PHP allow developers to explicitly declare the expected data type of a function’s parameters, return values, or class properties. Introduced in PHP 5 for objects and arrays, type hints were expanded in PHP 7 to include scalar types (e.g., int, float, string, bool) and additional features like return types and strict typing. By specifying types, you enforce constraints on the data that can be passed to or returned from functions, making your code more predictable and easier to debug. For example, without type hints, a function might accept any data type, leading to unexpected behavior: function add($a, $b) { return $a + $b; } echo add(5, "10"); // Output: 15 (string "10" is coerced to int) With type hints, you can ensure only integers are accepted: function add(int $a, int $b): int { return $a + $b; } echo add(5, "10"); // TypeError: Argument 2 must be int This simple example demonstrates how type hints prevent type-related bugs and make code behavior explicit. Why Use Type Hints? Type hints offer several benefits that improve code quality and developer productivity: Error Prevention: Catch type-related bugs at runtime (or earlier with static analysis tools). Improved Readability: Explicit types make code self-documenting, reducing the need for comments. Better Collaboration: Teams can understand function contracts without digging into implementation details. Easier Maintenance: Type hints act as a contract, making it easier to refactor or extend code. Tooling Support: Static analysis tools like Psalm and PHPStan leverage type hints for deeper code analysis. Performance Optimization: In some cases, type hints enable PHP’s JIT compiler to optimize code execution. By adopting type hints, you align your PHP codebase with modern programming practices, making it more robust and scalable. Key Features of PHP Type Hints Let’s dive into the core features of PHP type hints, with detailed explanations and practical code examples for each. 1. Scalar Type Hints Introduced in PHP 7.0, scalar type hints allow you to specify basic data types (int, float, string, bool) for function parameters and return values. Example: function calculateArea(float $length, float $width): float { return $length * $width; } echo calculateArea(5.5, 3.2); // Output: 17.6 // This will throw a TypeError echo calculateArea("5", 3.2); // TypeError: Argument 1 must be float In this example, the calculateArea function only accepts float values for $length and $width and guarantees a float return value. This prevents accidental type coercion (e.g., strings being converted to numbers). 2. Return Type Hints PHP 7.0 also introduced return type hints, allowing you to specify the type of value a function must return. Example: function getUserId(string $username): int { // Simulate database lookup $users = ['alice' => 1, 'bob' => 2]; return $users[$username] ?? throw new Exception("User not found"); } echo getUserId('alice'); // Output: 1 // This would throw a TypeError if the return value isn't an int Here, the getUserId function declares that it returns an int. If the function tries to return a non-integer (e.g., a string), PHP throws a TypeError. 3. Strict Typing By default, PHP uses coercive typing, meaning it attempts to convert values to the expected type (e.g., "5" to 5). To enforce strict type checking, you can use declare(strict_types=1) at the top of your PHP file. This ensures that only exact types are accepted, with no coercion. Example:
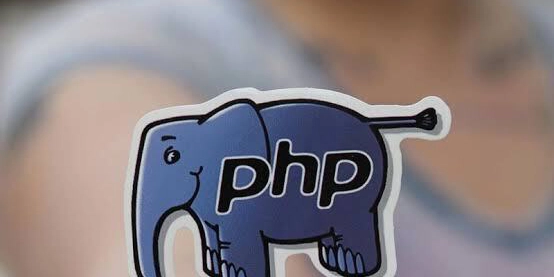
PHP has evolved significantly over the years, transforming from a loosely-typed scripting language into a powerful, modern programming language with features that rival strongly-typed languages. One of the standout features introduced in PHP 5 and enhanced in PHP 7+ is type hints (also known as type declarations). Type hints allow developers to specify the expected data types for function parameters, return values, and class properties, leading to cleaner, more reliable, and maintainable code.
In this extensive blog post, we’ll explore the power of PHP’s type hints, why they matter, and how to use them effectively. We’ll cover every aspect of type hints with practical code examples, best practices, and tips for integrating them into your projects. Whether you’re a beginner or a seasoned PHP developer, this guide will help you master type hints and elevate your coding game.
Table of Contents
- What Are Type Hints?
- Why Use Type Hints?
-
Key Features of PHP Type Hints
- Scalar Type Hints
- Return Type Hints
- Strict Typing
- Nullable Types
- Union Types
- Intersection Types
- Class and Interface Type Hints
- Iterable and Callable Types
- Void Return Type
- Mixed Type
- Best Practices for Using Type Hints
- Static Analysis Tools for Type Hints
- Common Pitfalls and How to Avoid Them
- Conclusion
What Are Type Hints?
Type hints in PHP allow developers to explicitly declare the expected data type of a function’s parameters, return values, or class properties. Introduced in PHP 5 for objects and arrays, type hints were expanded in PHP 7 to include scalar types (e.g., int
, float
, string
, bool
) and additional features like return types and strict typing. By specifying types, you enforce constraints on the data that can be passed to or returned from functions, making your code more predictable and easier to debug.
For example, without type hints, a function might accept any data type, leading to unexpected behavior:
function add($a, $b) {
return $a + $b;
}
echo add(5, "10"); // Output: 15 (string "10" is coerced to int)
With type hints, you can ensure only integers are accepted:
function add(int $a, int $b): int {
return $a + $b;
}
echo add(5, "10"); // TypeError: Argument 2 must be int
This simple example demonstrates how type hints prevent type-related bugs and make code behavior explicit.
Why Use Type Hints?
Type hints offer several benefits that improve code quality and developer productivity:
- Error Prevention: Catch type-related bugs at runtime (or earlier with static analysis tools).
- Improved Readability: Explicit types make code self-documenting, reducing the need for comments.
- Better Collaboration: Teams can understand function contracts without digging into implementation details.
- Easier Maintenance: Type hints act as a contract, making it easier to refactor or extend code.
- Tooling Support: Static analysis tools like Psalm and PHPStan leverage type hints for deeper code analysis.
- Performance Optimization: In some cases, type hints enable PHP’s JIT compiler to optimize code execution.
By adopting type hints, you align your PHP codebase with modern programming practices, making it more robust and scalable.
Key Features of PHP Type Hints
Let’s dive into the core features of PHP type hints, with detailed explanations and practical code examples for each.
1. Scalar Type Hints
Introduced in PHP 7.0, scalar type hints allow you to specify basic data types (int
, float
, string
, bool
) for function parameters and return values.
Example:
function calculateArea(float $length, float $width): float {
return $length * $width;
}
echo calculateArea(5.5, 3.2); // Output: 17.6
// This will throw a TypeError
echo calculateArea("5", 3.2); // TypeError: Argument 1 must be float
In this example, the calculateArea
function only accepts float
values for $length
and $width
and guarantees a float
return value. This prevents accidental type coercion (e.g., strings being converted to numbers).
2. Return Type Hints
PHP 7.0 also introduced return type hints, allowing you to specify the type of value a function must return.
Example:
function getUserId(string $username): int {
// Simulate database lookup
$users = ['alice' => 1, 'bob' => 2];
return $users[$username] ?? throw new Exception("User not found");
}
echo getUserId('alice'); // Output: 1
// This would throw a TypeError if the return value isn't an int
Here, the getUserId
function declares that it returns an int
. If the function tries to return a non-integer (e.g., a string), PHP throws a TypeError
.
3. Strict Typing
By default, PHP uses coercive typing, meaning it attempts to convert values to the expected type (e.g., "5"
to 5
). To enforce strict type checking, you can use declare(strict_types=1)
at the top of your PHP file. This ensures that only exact types are accepted, with no coercion.
Example:
declare(strict_types=1);
function divide(int $numerator, int $denominator): float {
return $numerator / $denominator;
}
echo divide(10, 2); // Output: 5.0
// This will throw a TypeError
echo divide("10", 2); // TypeError: Argument 1 must be int
Without strict_types=1
, PHP would coerce "10"
to 10
. Strict typing is especially useful for preventing subtle bugs in large applications.
4. Nullable Types
Introduced in PHP 7.1, nullable types allow a parameter or return value to be either the specified type or null
. This is denoted by prefixing the type with a question mark (e.g., ?int
).
Example:
function findUserEmail(string $username): ?string {
$emails = ['alice' => 'alice@example.com', 'bob' => null];
return $emails[$username] ?? null;
}
echo findUserEmail('alice'); // Output: alice@example.com
echo findUserEmail('bob'); // Output: null
The ?string
return type indicates that the function returns either a string
or null
, making the function’s behavior explicit.
5. Union Types
PHP 8.0 introduced union types, allowing a parameter or return value to accept multiple types, denoted by separating types with a pipe (|
).
Example:
function formatPrice(int|float $price): string {
return '$' . number_format($price, 2);
}
echo formatPrice(10); // Output: $10.00
echo formatPrice(9.99); // Output: $9.99
// This will throw a TypeError
echo formatPrice("10"); // TypeError: Argument 1 must be int|float
Union types are ideal when a function needs to handle multiple valid types, such as numbers in a pricing function.
6. Intersection Types
Introduced in PHP 8.1, intersection types allow you to specify that a parameter or return value must implement multiple interfaces or extend multiple classes, denoted by &
.
Example:
interface Loggable {
public function log(): string;
}
interface Serializable {
public function serialize(): string;
}
function processObject(Loggable&Serializable $obj): string {
return $obj->log() . ' ' . $obj->serialize();
}
class MyClass implements Loggable, Serializable {
public function log(): string {
return "Logged";
}
public function serialize(): string {
return "Serialized";
}
}
echo processObject(new MyClass()); // Output: Logged Serialized
Intersection types are powerful for ensuring an object adheres to multiple interfaces, common in complex systems.
7. Class and Interface Type Hints
Type hints were originally introduced in PHP 5 for classes, interfaces, and arrays. You can specify that a parameter or return value must be an instance of a specific class or implement a specific interface.
Example:
interface Logger {
public function log(string $message): void;
}
class FileLogger implements Logger {
public function log(string $message): void {
file_put_contents('log.txt', $message . PHP_EOL, FILE_APPEND);
}
}
function setLogger(Logger $logger): void {
$logger->log("System started");
}
setLogger(new FileLogger()); // Logs "System started" to log.txt
Here, the setLogger
function accepts any object that implements the Logger
interface, promoting loose coupling and flexibility.
8. Iterable and Callable Types
PHP supports iterable
(for arrays or objects implementing Traversable
) and callable
(for functions, methods, or objects with __invoke
) as type hints.
Example (Iterable):
function processItems(iterable $items): void {
foreach ($items as $item) {
echo $item . PHP_EOL;
}
}
processItems([1, 2, 3]); // Output: 1 2 3
processItems(new ArrayIterator([4, 5, 6])); // Output: 4 5 6
Example (Callable):
function executeCallback(callable $callback): void {
$callback();
}
executeCallback(function () {
echo "Callback executed\n";
}); // Output: Callback executed
These types are useful for functions that need to work with collections or dynamic behavior.
9. Void Return Type
The void
return type, introduced in PHP 7.1, indicates that a function does not return a value (i.e., it implicitly returns null
).
Example:
function logMessage(string $message): void {
echo $message . PHP_EOL;
}
logMessage("Hello, World!"); // Output: Hello, World!
Attempting to return a value from a void
function will result in a TypeError
.
10. Mixed Type
Introduced in PHP 8.0, the mixed
type indicates that a parameter or return value can be any type. It’s a fallback when no specific type can be enforced.
Example:
function debug(mixed $value): void {
var_dump($value);
}
debug(42); // Output: int(42)
debug("hello"); // Output: string(5) "hello"
debug([1, 2, 3]); // Output: array(3) { ... }
Use mixed
sparingly, as it reduces the benefits of type safety. It’s best for cases where flexibility is genuinely needed.
Best Practices for Using Type Hints
To maximize the benefits of type hints, follow these best practices:
-
Enable Strict Typing: Always use
declare(strict_types=1)
in new projects to avoid type coercion and ensure predictable behavior. -
Use Specific Types: Prefer specific types (e.g.,
int
orstring
) overmixed
to enforce constraints and improve clarity. -
Leverage Nullable and Union Types: Use
?type
ortype1|type2
to handle edge cases explicitly. - Document Complex Types: For complex union or intersection types, add PHPDoc comments to improve IDE support and readability.
- Combine with Static Analysis: Use tools like Psalm or PHPStan to catch type-related issues before runtime.
- Apply Type Hints Consistently: Use type hints for all parameters, return types, and class properties to maintain a uniform codebase.
- Test Edge Cases: Write unit tests to verify behavior for nullable types, union types, and invalid inputs.
Static Analysis Tools for Type Hints
Static analysis tools enhance the power of type hints by catching issues during development, before code reaches production. Popular tools include:
- Psalm: A static analysis tool that checks for type errors, unused code, and other issues. It supports advanced type inference and custom type annotations.
- PHPStan: Another powerful static analysis tool that focuses on finding type-related bugs and ensuring type safety.
- PHP_CodeSniffer: While primarily a code style checker, it can enforce type hint usage in your codebase.
Example with Psalm:
Install Psalm via Composer:
composer require --dev vimeo/psalm
Run Psalm on your codebase:
vendor/bin/psalm
Psalm will analyze your code and report type mismatches, such as passing a string
to a function expecting an int
.
Common Pitfalls and How to Avoid Them
-
Forgetting Strict Typing:
-
Problem: Without
declare(strict_types=1)
, PHP coerces types, leading to unexpected behavior. -
Solution: Always include
declare(strict_types=1)
at the top of your files.
-
Problem: Without
-
Overusing Mixed Type:
-
Problem: Using
mixed
reduces type safety and makes code harder to reason about. - Solution: Use specific types or union types whenever possible.
-
Problem: Using
-
Ignoring Nullable Types:
-
Problem: Forgetting to account for
null
can lead to runtime errors. -
Solution: Use
?type
ortype|null
for parameters and return values that can benull
.
-
Problem: Forgetting to account for
-
Inconsistent Type Usage:
- Problem: Mixing typed and untyped functions in a codebase creates confusion.
- Solution: Enforce type hints across your project using coding standards and CI checks.
-
Not Testing Type Edge Cases:
-
Problem: Failing to test invalid or edge-case inputs can lead to uncaught
TypeError
exceptions. - Solution: Write comprehensive unit tests with tools like PHPUnit.
-
Problem: Failing to test invalid or edge-case inputs can lead to uncaught
Conclusion
PHP’s type hints are a powerful tool for writing robust, maintainable, and self-documenting code. By leveraging scalar types, return types, strict typing, nullable types, union types, intersection types, and more, you can catch errors early, improve collaboration, and build applications that scale. Combine type hints with static analysis tools like Psalm or PHPStan to take your code quality to the next level.
Whether you’re building a small script or a large enterprise application, adopting type hints will make your PHP code more reliable and easier to maintain. Start incorporating type hints into your projects today, and share your experiences or tips in the comments below!
Further Reading: