Lightweight and High-Performance Rust HTTP Server Library for Modern Web Services
Introduction Hyperlane is a cutting-edge Rust library designed to simplify the development of high-performance web services. Built with a focus on efficiency and flexibility, it empowers developers to create robust HTTP servers with minimal overhead. Whether you’re building REST APIs, real-time applications, or scalable backend services, Hyperlane offers a seamless development experience with its rich feature set and cross-platform compatibility. Core Features Lightweight & High Performance Hyperlane leverages Rust’s memory safety and concurrency features, combined with the Tokio async runtime, to deliver exceptional performance. It handles asynchronous I/O efficiently, making it ideal for high-throughput applications. Full HTTP/1.1 Support Parses HTTP requests and builds responses with ease. Supports essential features like keep-alive connections, request routing, and header manipulation. Real-Time Communication Native support for WebSocket and Server-Sent Events (SSE), enabling efficient bidirectional and unidirectional real-time data flows. Middleware Architecture Flexible request and response middleware system allows for modular logic, such as logging, authentication, and header handling. Cross-Platform Compatibility Built with pure Rust and the standard library, Hyperlane runs seamlessly on Windows, Linux, and macOS, with consistent API behavior across all platforms. Minimal Dependencies Relies on battle-tested crates like Tokio for async operations, ensuring stability without platform-specific dependencies. Quick Start Guide 1. Installation Add Hyperlane to your Rust project using Cargo: cargo add hyperlane 2. Get Started with a Sample Project Clone the official quick-start repository to explore a production-ready template: git clone https://github.com/ltpp-universe/hyperlane-quick-start.git Navigate to the project directory and run it with: cargo run For background execution: cargo run -d Code Example: Building a Simple Server The following example demonstrates a basic Hyperlane server with middleware, routing, and WebSocket support: use hyperlane::*; // Request middleware to set common headers async fn request_middleware(ctx: Context) { let socket_addr = ctx.get_socket_addr_or_default_string().await; ctx.set_response_header(SERVER, HYPERLANE) .await .set_response_header(CONNECTION, CONNECTION_KEEP_ALIVE) .await .set_response_header(CONTENT_TYPE, content_type_charset(TEXT_PLAIN, UTF8)) .await .set_response_header(DATE, gmt()) .await .set_response_header("SocketAddr", socket_addr) .await; } // Response middleware for logging async fn response_middleware(ctx: Context) { let _ = ctx.send().await; let request = ctx.get_request_string().await; let response = ctx.get_response_string().await; ctx.log_info(&request, log_handler) .await .log_info(&response, log_handler) .await; } // Root route handler async fn root_route(ctx: Context) { ctx.set_response_status_code(200) .await .set_response_body("Hello hyperlane => /") .await; } // WebSocket route handler async fn websocket_route(ctx: Context) { let request_body = ctx.get_request_body().await; let _ = ctx.send_response_body(request_body).await; } #[tokio::main] async fn main() { let mut server = Server::new(); server .host("0.0.0.0") .await .port(60000) .await .enable_nodelay() .await .log_dir("./logs") .await // Configure middleware and routes .request_middleware(request_middleware) .await .response_middleware(response_middleware) .await .route("/", root_route) .await .route("/websocket", websocket_route) .await; // Run the server server.run().await.unwrap(); } Performance Benchmarks Hyperlane’s performance rivals leading frameworks, as shown in these independent benchmarks: 1000 Concurrent Requests, 1 Million Total Requests Framework/Stack QPS (Requests/Second) Tokio 308,596.26 Hyperlane 307,568.90 Rocket 267,931.52 Rust Standard Library 260,514.56 Go Standard Library 226,550.34 Gin (Go) 224,296.16 Node.js Standard Library 85,357.18 360 Concurrent Requests, 60s Duration Framework/Stack QPS (Requests/Second) Tokio 340,130.92 Hyperlane 324,323.71 Rocket 298,945.31 Rust Standard Library 291,218.96 Gin (Go) 242,570.16 Go Standard Library 234,178.93 Node.js Standard Library 139,412.13 Community & Support License: Released under the permissive MIT License, ideal for open-source and commercial projects. Contributions: Welco
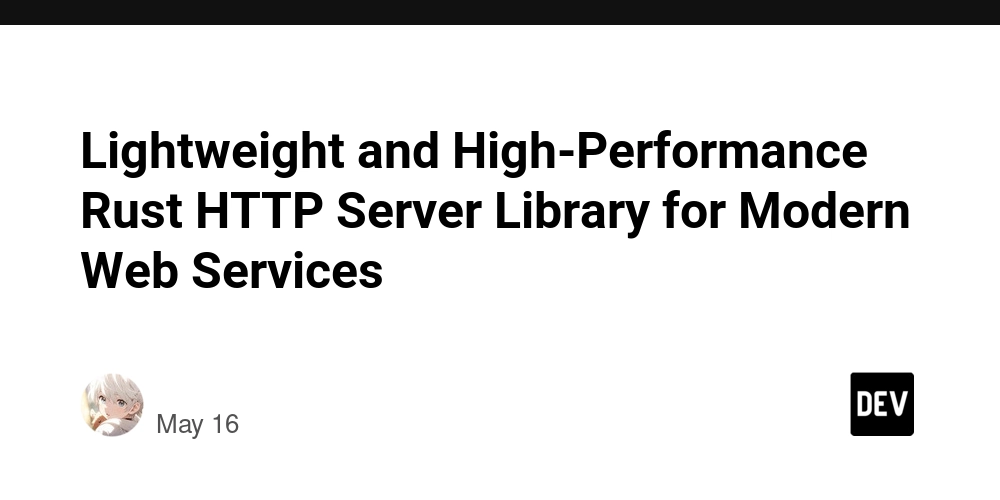
Introduction
Hyperlane is a cutting-edge Rust library designed to simplify the development of high-performance web services. Built with a focus on efficiency and flexibility, it empowers developers to create robust HTTP servers with minimal overhead. Whether you’re building REST APIs, real-time applications, or scalable backend services, Hyperlane offers a seamless development experience with its rich feature set and cross-platform compatibility.
Core Features
Lightweight & High Performance
Hyperlane leverages Rust’s memory safety and concurrency features, combined with the Tokio async runtime, to deliver exceptional performance. It handles asynchronous I/O efficiently, making it ideal for high-throughput applications.-
Full HTTP/1.1 Support
- Parses HTTP requests and builds responses with ease.
- Supports essential features like keep-alive connections, request routing, and header manipulation.
-
Real-Time Communication
- Native support for WebSocket and Server-Sent Events (SSE), enabling efficient bidirectional and unidirectional real-time data flows.
-
Middleware Architecture
- Flexible request and response middleware system allows for modular logic, such as logging, authentication, and header handling.
Cross-Platform Compatibility
Built with pure Rust and the standard library, Hyperlane runs seamlessly on Windows, Linux, and macOS, with consistent API behavior across all platforms.Minimal Dependencies
Relies on battle-tested crates like Tokio for async operations, ensuring stability without platform-specific dependencies.
Quick Start Guide
1. Installation
Add Hyperlane to your Rust project using Cargo:
cargo add hyperlane
2. Get Started with a Sample Project
Clone the official quick-start repository to explore a production-ready template:
git clone https://github.com/ltpp-universe/hyperlane-quick-start.git
Navigate to the project directory and run it with:
cargo run
For background execution:
cargo run -d
Code Example: Building a Simple Server
The following example demonstrates a basic Hyperlane server with middleware, routing, and WebSocket support:
use hyperlane::*;
// Request middleware to set common headers
async fn request_middleware(ctx: Context) {
let socket_addr = ctx.get_socket_addr_or_default_string().await;
ctx.set_response_header(SERVER, HYPERLANE)
.await
.set_response_header(CONNECTION, CONNECTION_KEEP_ALIVE)
.await
.set_response_header(CONTENT_TYPE, content_type_charset(TEXT_PLAIN, UTF8))
.await
.set_response_header(DATE, gmt())
.await
.set_response_header("SocketAddr", socket_addr)
.await;
}
// Response middleware for logging
async fn response_middleware(ctx: Context) {
let _ = ctx.send().await;
let request = ctx.get_request_string().await;
let response = ctx.get_response_string().await;
ctx.log_info(&request, log_handler)
.await
.log_info(&response, log_handler)
.await;
}
// Root route handler
async fn root_route(ctx: Context) {
ctx.set_response_status_code(200)
.await
.set_response_body("Hello hyperlane => /")
.await;
}
// WebSocket route handler
async fn websocket_route(ctx: Context) {
let request_body = ctx.get_request_body().await;
let _ = ctx.send_response_body(request_body).await;
}
#[tokio::main]
async fn main() {
let mut server = Server::new();
server
.host("0.0.0.0")
.await
.port(60000)
.await
.enable_nodelay()
.await
.log_dir("./logs")
.await
// Configure middleware and routes
.request_middleware(request_middleware)
.await
.response_middleware(response_middleware)
.await
.route("/", root_route)
.await
.route("/websocket", websocket_route)
.await;
// Run the server
server.run().await.unwrap();
}
Performance Benchmarks
Hyperlane’s performance rivals leading frameworks, as shown in these independent benchmarks:
1000 Concurrent Requests, 1 Million Total Requests
Framework/Stack | QPS (Requests/Second) |
---|---|
Tokio | 308,596.26 |
Hyperlane | 307,568.90 |
Rocket | 267,931.52 |
Rust Standard Library | 260,514.56 |
Go Standard Library | 226,550.34 |
Gin (Go) | 224,296.16 |
Node.js Standard Library | 85,357.18 |
360 Concurrent Requests, 60s Duration
Framework/Stack | QPS (Requests/Second) |
---|---|
Tokio | 340,130.92 |
Hyperlane | 324,323.71 |
Rocket | 298,945.31 |
Rust Standard Library | 291,218.96 |
Gin (Go) | 242,570.16 |
Go Standard Library | 234,178.93 |
Node.js Standard Library | 139,412.13 |
Community & Support
- License: Released under the permissive MIT License, ideal for open-source and commercial projects.
- Contributions: Welcome! Submit issues or pull requests on GitHub.
- Contact: For questions, reach the team at [ltpp-universe root@ltpp.vip](mailto:root@ltpp.vip).
Get Started Today
Hyperlane simplifies the process of building fast, reliable web services in Rust. Whether you’re a seasoned Rust developer or new to the language, its intuitive API and performance make it a top choice for modern backend development.
- Official Documentation: https://docs.ltpp.vip/hyperlane/
- API Reference: https://docs.rs/hyperlane/latest/hyperlane/
- Quick-Start Project: https://github.com/ltpp-universe/hyperlane-quick-start
Join the growing community and experience the power of Rust for high-performance web development with Hyperlane!