How to Refresh ListView on Returning to Flutter Screen?
Introduction When developing Flutter applications, managing state and ensuring that the UI refreshes appropriately when navigating between screens can be challenging. In your case, you're attempting to display a list of addresses using a ListView, and you want it to refresh with updated data after returning from a second screen where the user adds or edits an address. This article will explore why your ListView might not be refreshing as expected even when the correct events and states are triggered using the Bloc pattern. Why Isn't the ListView Refreshing? Your current implementation seems to be set up correctly in terms of triggering the FetchDashboard event upon returning to the first screen. However, there are a few common pitfalls that can prevent the ListView from reflecting the updated data: State Not Changing: Even though the FetchDashboard event is fired, if the fetched data is identical to the current state, the ListView may not rebuild. Widget Keys: The ValueKey used in your ListView builder may not effectively cause a rebuild if it does not change with every new state. Unresponsive UI Update: The Flutter widget tree may not react to state changes due to how the BlocBuilder is set up. Step-by-Step Solution Let’s break down the solution to ensure that your ListView refreshes correctly when returning from the second screen. 1. Verify State Changes Make sure that the new fetched data differs from the previous data. You can print the address list after fetching it to verify the contents: if (state is DashboardLoaded) { final addressList = state.dashboardResponse.data.user.billingAddress.data; print('Fetched addresses: $addressList'); // Debugging line // Remaining code... } 2. Modify the ValueKey Ensure that the ValueKey in your ListView.builder is updated whenever a new address is fetched. Instead of using the entire dashboardResponse, consider using an ID or a count of the address list: return ListView.builder( key: ValueKey(addressList.length), // Use length as the key padding: EdgeInsets.zero, shrinkWrap: true, physics: NeverScrollableScrollPhysics(), itemCount: addressList.length, itemBuilder: (context, index) { final address = addressList[index]; return ListTile( title: Text(address.firstName), subtitle: Text(address.city.name), ); }, ); 3. Ensuring Proper State Management Make sure that your Bloc is properly set up to emit new states for every event if the data source changes. The typical pattern for handling data fetching is as follows: void mapEventToState(DashboardEvent event) async { if (event is FetchDashboard) { yield DashboardLoading(); try { final dashboardResponse = await fetchData(); // Emit a new state with the freshly fetched data yield DashboardLoaded(dashboardResponse); } catch (e) { yield AddressError(); } } } 4. Debugging State Changes Ensure that the event is being processed by the Bloc and that new states are produced. You may also log the state transitions from your Bloc to make sure: @override Stream mapEventToState(DashboardEvent event) async* { print('Event Received: $event'); // Your existing code... } 5. Using BlocListener (Optional) As an enhancement, you can use BlocListener in conjunction with BlocBuilder to show toasts or perform navigation based on specific states. This could simplify handling UI updates and side effects without cluttering the builder code: BlocListener( listener: (context, state) { if (state is DashboardLoaded) { Utils.showToast(context, 'Address list updated.'); } }, child: BlocBuilder( builder: (context, state) { // Your list building code... }, ), ) Frequently Asked Questions How do I ensure state is updated in Flutter with Bloc? Make sure your Bloc emits a new state every time data changes. If the fetched data is the same, the UI won't update. Why is my ListView not rebuilding? Rebuilds are triggered based on keys and state changes. Modify keys in your ListView to ensure that they are updated when new data is present. Can I debug state changes in Flutter Bloc? Yes, you can print logs inside your event and state transitions to understand how your state changes occur. Conclusion By following the suggestions outlined in this article, you should be able to ensure that your ListView refreshes correctly upon returning to the first screen. By making small adjustments to how you manage state and key usage in your widget tree, you can keep your UI in sync with the underlying data effectively. Happy coding!
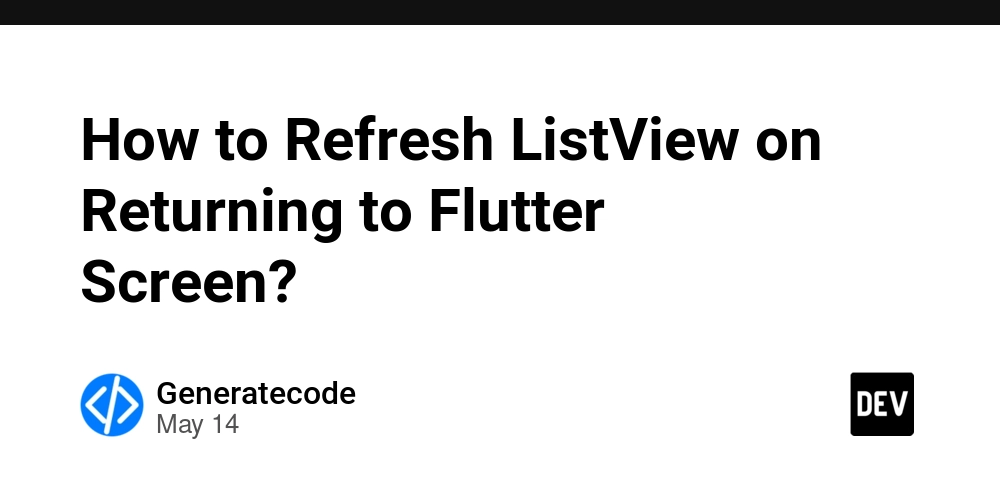
Introduction
When developing Flutter applications, managing state and ensuring that the UI refreshes appropriately when navigating between screens can be challenging. In your case, you're attempting to display a list of addresses using a ListView
, and you want it to refresh with updated data after returning from a second screen where the user adds or edits an address. This article will explore why your ListView
might not be refreshing as expected even when the correct events and states are triggered using the Bloc pattern.
Why Isn't the ListView Refreshing?
Your current implementation seems to be set up correctly in terms of triggering the FetchDashboard
event upon returning to the first screen. However, there are a few common pitfalls that can prevent the ListView
from reflecting the updated data:
-
State Not Changing: Even though the
FetchDashboard
event is fired, if the fetched data is identical to the current state, theListView
may not rebuild. -
Widget Keys: The
ValueKey
used in yourListView
builder may not effectively cause a rebuild if it does not change with every new state. - Unresponsive UI Update: The Flutter widget tree may not react to state changes due to how the BlocBuilder is set up.
Step-by-Step Solution
Let’s break down the solution to ensure that your ListView
refreshes correctly when returning from the second screen.
1. Verify State Changes
Make sure that the new fetched data differs from the previous data. You can print the address list after fetching it to verify the contents:
if (state is DashboardLoaded) {
final addressList = state.dashboardResponse.data.user.billingAddress.data;
print('Fetched addresses: $addressList'); // Debugging line
// Remaining code...
}
2. Modify the ValueKey
Ensure that the ValueKey
in your ListView.builder
is updated whenever a new address is fetched. Instead of using the entire dashboardResponse
, consider using an ID or a count of the address list:
return ListView.builder(
key: ValueKey(addressList.length), // Use length as the key
padding: EdgeInsets.zero,
shrinkWrap: true,
physics: NeverScrollableScrollPhysics(),
itemCount: addressList.length,
itemBuilder: (context, index) {
final address = addressList[index];
return ListTile(
title: Text(address.firstName),
subtitle: Text(address.city.name),
);
},
);
3. Ensuring Proper State Management
Make sure that your Bloc is properly set up to emit new states for every event if the data source changes. The typical pattern for handling data fetching is as follows:
void mapEventToState(DashboardEvent event) async {
if (event is FetchDashboard) {
yield DashboardLoading();
try {
final dashboardResponse = await fetchData();
// Emit a new state with the freshly fetched data
yield DashboardLoaded(dashboardResponse);
} catch (e) {
yield AddressError();
}
}
}
4. Debugging State Changes
Ensure that the event is being processed by the Bloc and that new states are produced. You may also log the state transitions from your Bloc to make sure:
@override
Stream mapEventToState(DashboardEvent event) async* {
print('Event Received: $event');
// Your existing code...
}
5. Using BlocListener (Optional)
As an enhancement, you can use BlocListener
in conjunction with BlocBuilder
to show toasts or perform navigation based on specific states. This could simplify handling UI updates and side effects without cluttering the builder code:
BlocListener(
listener: (context, state) {
if (state is DashboardLoaded) {
Utils.showToast(context, 'Address list updated.');
}
},
child: BlocBuilder(
builder: (context, state) {
// Your list building code...
},
),
)
Frequently Asked Questions
How do I ensure state is updated in Flutter with Bloc?
Make sure your Bloc emits a new state every time data changes. If the fetched data is the same, the UI won't update.
Why is my ListView not rebuilding?
Rebuilds are triggered based on keys and state changes. Modify keys in your ListView to ensure that they are updated when new data is present.
Can I debug state changes in Flutter Bloc?
Yes, you can print logs inside your event and state transitions to understand how your state changes occur.
Conclusion
By following the suggestions outlined in this article, you should be able to ensure that your ListView
refreshes correctly upon returning to the first screen. By making small adjustments to how you manage state and key usage in your widget tree, you can keep your UI in sync with the underlying data effectively. Happy coding!