How to Build Scalable Web Applications with ASP.NET
How to Build Scalable Web Applications with ASP.NET Building scalable web applications is crucial for handling growth in user traffic, data volume, and feature complexity. ASP.NET, Microsoft’s robust web framework, provides powerful tools to create high-performance, scalable applications. In this guide, we’ll explore best practices for scalability, architecture patterns, and performance optimizations. If you're looking to monetize your web development skills, consider checking out MillionFormula for opportunities to turn your expertise into income. 1. Choosing the Right Architecture A well-designed architecture is the foundation of a scalable application. Two popular patterns for ASP.NET applications are: a. Layered Architecture Separates concerns into Presentation, Business Logic, and Data Access layers. csharp Copy // Example of a layered architecture in ASP.NET public class ProductsController : Controller { private readonly IProductService _productService; public ProductsController(IProductService productService) { _productService = productService; } public IActionResult GetProducts() { var products = _productService.GetAllProducts(); return Ok(products); } } b. Microservices Decomposes the application into small, independent services. ASP.NET Core works well with Docker and Kubernetes for microservices deployments. csharp Copy // Example of a minimal API in ASP.NET Core for a microservice var builder = WebApplication.CreateBuilder(args); var app = builder.Build(); app.MapGet("/products", () => { return Results.Ok(ProductRepository.GetAll()); }); app.Run(); For more on microservices, check Microsoft’s Microservices Architecture Guide. 2. Database Scalability Strategies a. Caching with Redis Reduce database load by caching frequently accessed data. csharp Copy // Using Redis Cache in ASP.NET builder.Services.AddStackExchangeRedisCache(options => { options.Configuration = "localhost:6379"; }); // Example usage public class ProductService { private readonly IDistributedCache _cache; public ProductService(IDistributedCache cache) { _cache = cache; } public async Task GetProductsAsync() { var cachedProducts = await _cache.GetStringAsync("products"); if (cachedProducts != null) return JsonSerializer.Deserialize(cachedProducts); var products = await FetchFromDatabase(); await _cache.SetStringAsync("products", JsonSerializer.Serialize(products)); return products; } } b. Database Sharding & Read Replicas Sharding: Distributes data across multiple databases. Read Replicas: Offload read operations to secondary databases. For EF Core sharding, consider EF Core Sharding Libraries. 3. Asynchronous Programming Avoid blocking calls to improve scalability. csharp Copy // Using async/await in ASP.NET public async Task GetProductsAsync() { var products = await _productService.GetAllProductsAsync(); return Ok(products); } 4. Load Balancing & Horizontal Scaling Deploy multiple instances behind a load balancer (e.g., Azure Load Balancer, NGINX). bash Copy # Example Docker Compose for scaling services: webapp: image: my-aspnet-app deploy: replicas: 4 For cloud deployments, use Azure App Service Autoscaling. 5. Optimizing Performance a. Response Compression csharp Copy builder.Services.AddResponseCompression(options => { options.Providers.Add(); }); b. Minimize Large Object Allocations Use ArrayPool and MemoryPool for buffer management. csharp Copy // Using ArrayPool to reduce GC pressure var buffer = ArrayPool.Shared.Rent(1024); try { // Process buffer } finally { ArrayPool.Shared.Return(buffer); } c. Use CDN for Static Files csharp Copy app.UseStaticFiles(new StaticFileOptions { OnPrepareResponse = ctx => { ctx.Context.Response.Headers.Append("Cache-Control", "public,max-age=31536000"); } }); 6. Monitoring & Logging Track performance with: Application Insights (Azure Monitor) Serilog for structured logging csharp Copy // Serilog configuration builder.Host.UseSerilog((ctx, config) => { config.WriteTo.Console() .WriteTo.File("logs/log.txt"); }); Conclusion Building scalable ASP.NET applications involves: ✔ Choosing the right architecture (Layered or Microservices) ✔ Optimizing database access (Caching, Sharding) ✔ Using async programming ✔ Leveraging load balancing ✔ Monitoring performance By following these best practices, you can ensure your application handles growth efficiently. If you're looking to monetize your ASP.NET skills, explore MillionFormula for ways to turn your expertise into revenue.
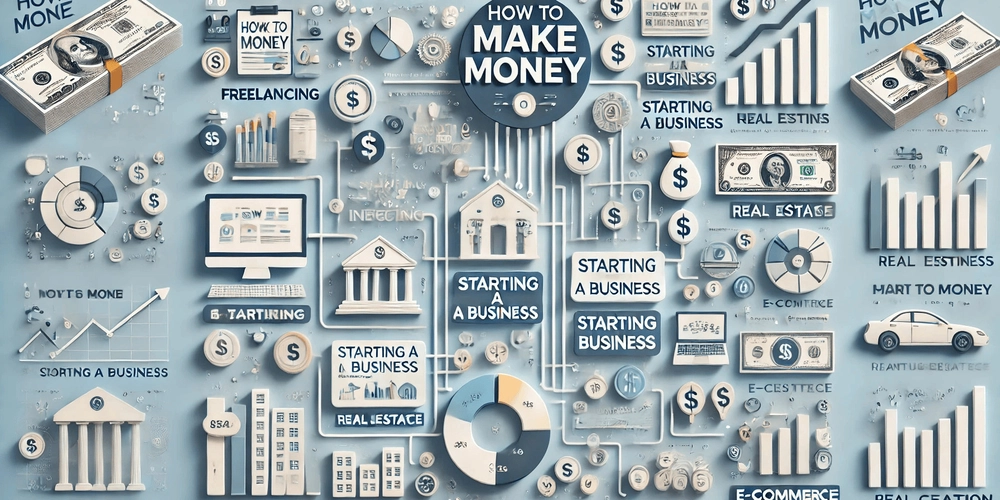
How to Build Scalable Web Applications with ASP.NET
Building scalable web applications is crucial for handling growth in user traffic, data volume, and feature complexity. ASP.NET, Microsoft’s robust web framework, provides powerful tools to create high-performance, scalable applications. In this guide, we’ll explore best practices for scalability, architecture patterns, and performance optimizations.
If you're looking to monetize your web development skills, consider checking out MillionFormula for opportunities to turn your expertise into income.
1. Choosing the Right Architecture
A well-designed architecture is the foundation of a scalable application. Two popular patterns for ASP.NET applications are:
a. Layered Architecture
Separates concerns into Presentation, Business Logic, and Data Access layers.
csharp
Copy
// Example of a layered architecture in ASP.NET public class ProductsController : Controller { private readonly IProductService _productService; public ProductsController(IProductService productService) { _productService = productService; } public IActionResult GetProducts() { var products = _productService.GetAllProducts(); return Ok(products); } }
b. Microservices
Decomposes the application into small, independent services. ASP.NET Core works well with Docker and Kubernetes for microservices deployments.
csharp
Copy
// Example of a minimal API in ASP.NET Core for a microservice var builder = WebApplication.CreateBuilder(args); var app = builder.Build(); app.MapGet("/products", () => { return Results.Ok(ProductRepository.GetAll()); }); app.Run();
For more on microservices, check Microsoft’s Microservices Architecture Guide.
2. Database Scalability Strategies
a. Caching with Redis
Reduce database load by caching frequently accessed data.
csharp
Copy
// Using Redis Cache in ASP.NET builder.Services.AddStackExchangeRedisCache(options => { options.Configuration = "localhost:6379"; }); // Example usage public class ProductService { private readonly IDistributedCache _cache; public ProductService(IDistributedCache cache) { _cache = cache; } public async Task<List<Product>> GetProductsAsync() { var cachedProducts = await _cache.GetStringAsync("products"); if (cachedProducts != null) return JsonSerializer.Deserialize<List<Product>>(cachedProducts); var products = await FetchFromDatabase(); await _cache.SetStringAsync("products", JsonSerializer.Serialize(products)); return products; } }
b. Database Sharding & Read Replicas
- Sharding: Distributes data across multiple databases.
- Read Replicas: Offload read operations to secondary databases.
For EF Core sharding, consider EF Core Sharding Libraries.
3. Asynchronous Programming
Avoid blocking calls to improve scalability.
csharp
Copy
// Using async/await in ASP.NET public async Task<IActionResult> GetProductsAsync() { var products = await _productService.GetAllProductsAsync(); return Ok(products); }
4. Load Balancing & Horizontal Scaling
Deploy multiple instances behind a load balancer (e.g., Azure Load Balancer, NGINX).
bash
Copy
# Example Docker Compose for scaling services: webapp: image: my-aspnet-app deploy: replicas: 4
For cloud deployments, use Azure App Service Autoscaling.
5. Optimizing Performance
a. Response Compression
csharp Copybuilder.Services.AddResponseCompression(options => { options.Providers.Add<GzipCompressionProvider>(); });
b. Minimize Large Object Allocations
Use ArrayPool
and MemoryPool
for buffer management.
csharp
Copy
// Using ArrayPool to reduce GC pressure var buffer = ArrayPool<byte>.Shared.Rent(1024); try { // Process buffer } finally { ArrayPool<byte>.Shared.Return(buffer); }
c. Use CDN for Static Files
csharp
Copy
app.UseStaticFiles(new StaticFileOptions { OnPrepareResponse = ctx => { ctx.Context.Response.Headers.Append("Cache-Control", "public,max-age=31536000"); } });
6. Monitoring & Logging
Track performance with:
- Application Insights (Azure Monitor)
- Serilog for structured logging
csharp
Copy
// Serilog configuration builder.Host.UseSerilog((ctx, config) => { config.WriteTo.Console() .WriteTo.File("logs/log.txt"); });
Conclusion
Building scalable ASP.NET applications involves:
✔ Choosing the right architecture (Layered or Microservices)
✔ Optimizing database access (Caching, Sharding)
✔ Using async programming
✔ Leveraging load balancing
✔ Monitoring performance
By following these best practices, you can ensure your application handles growth efficiently.
If you're looking to monetize your ASP.NET skills, explore MillionFormula for ways to turn your expertise into revenue.
Happy coding!