Generate an article about good logging practice in backend
Logging is a crucial aspect of backend development. Effective logging allows you to monitor your application's health, debug issues, and understand user behavior. Poor logging, on the other hand, can lead to wasted time, difficult debugging, and security vulnerabilities. This article outlines some best practices for implementing effective logging in your backend systems. Key Principles: Log Levels: Utilize different log levels (DEBUG, INFO, WARNING, ERROR, CRITICAL) to categorize log messages based on their severity. This allows you to filter and prioritize information effectively. DEBUG is for detailed debugging information, INFO for general application flow, WARNING for potential issues, ERROR for actual errors, and CRITICAL for system-critical failures. Context is King: Include relevant context in your log messages. This might include timestamps, user IDs, request IDs, affected resources, and any other information that helps pinpoint the source of an issue. Structured Logging: Instead of free-form text, use structured logging formats like JSON. This allows log aggregation tools to easily parse and analyze your logs. Avoid Sensitive Information: Never log sensitive data like passwords, API keys, or credit card numbers. This is a major security risk. Centralized Logging: Aggregate logs from different services and servers into a central location. This simplifies monitoring and analysis. Tools like Elasticsearch, Logstash, and Kibana (ELK stack) or Graylog are excellent for this purpose. Log Exceptions Properly: Don't just log the exception message. Include the stack trace and any relevant context that led to the exception. Performance Considerations: Logging can impact performance. Avoid excessive logging, especially at the DEBUG level in production. Use asynchronous logging where possible to minimize blocking operations. Use a Logging Framework: Leverage a logging framework like Python's logging module or similar frameworks in other languages. These frameworks provide built-in functionalities for log levels, formatting, and handlers. Real-World Scenario: Imagine an e-commerce platform. A user is trying to complete a purchase, but the payment gateway is experiencing intermittent issues. Without proper logging, debugging this issue would be a nightmare. Poor Logging Example (Python): print("Payment failed") Good Logging Example (Python): python import logging logger = logging.getLogger(__
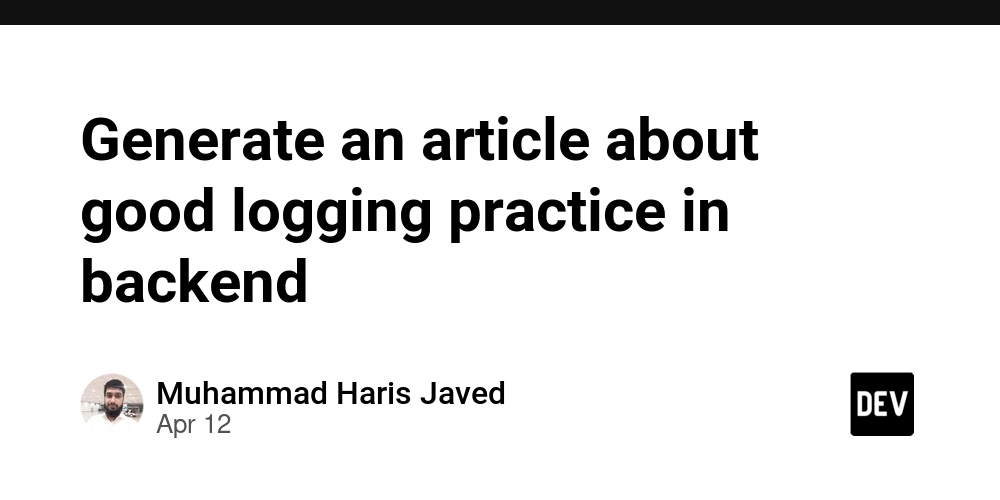
Logging is a crucial aspect of backend development. Effective logging allows you to monitor your application's health, debug issues, and understand user behavior. Poor logging, on the other hand, can lead to wasted time, difficult debugging, and security vulnerabilities. This article outlines some best practices for implementing effective logging in your backend systems.
Key Principles:
Log Levels: Utilize different log levels (DEBUG, INFO, WARNING, ERROR, CRITICAL) to categorize log messages based on their severity. This allows you to filter and prioritize information effectively. DEBUG is for detailed debugging information, INFO for general application flow, WARNING for potential issues, ERROR for actual errors, and CRITICAL for system-critical failures.
Context is King: Include relevant context in your log messages. This might include timestamps, user IDs, request IDs, affected resources, and any other information that helps pinpoint the source of an issue.
Structured Logging: Instead of free-form text, use structured logging formats like JSON. This allows log aggregation tools to easily parse and analyze your logs.
Avoid Sensitive Information: Never log sensitive data like passwords, API keys, or credit card numbers. This is a major security risk.
Centralized Logging: Aggregate logs from different services and servers into a central location. This simplifies monitoring and analysis. Tools like Elasticsearch, Logstash, and Kibana (ELK stack) or Graylog are excellent for this purpose.
Log Exceptions Properly: Don't just log the exception message. Include the stack trace and any relevant context that led to the exception.
Performance Considerations: Logging can impact performance. Avoid excessive logging, especially at the DEBUG level in production. Use asynchronous logging where possible to minimize blocking operations.
Use a Logging Framework: Leverage a logging framework like Python's
logging
module or similar frameworks in other languages. These frameworks provide built-in functionalities for log levels, formatting, and handlers.
Real-World Scenario:
Imagine an e-commerce platform. A user is trying to complete a purchase, but the payment gateway is experiencing intermittent issues. Without proper logging, debugging this issue would be a nightmare.
Poor Logging Example (Python):
print("Payment failed")
Good Logging Example (Python):
python
import logging
logger = logging.getLogger(__