Effortlessly Navigate and Search Large-Scale Repositories with fzf and Ripgrep
In a fast-paced development environment, efficiency is key. Searching for files, commits, or code snippets in a Git repository can be time-consuming if you're relying solely on built-in Git commands. Fortunately, tools like Fuzzy Finder (fzf) and Ripgrep (rg) can significantly enhance your Git workflow, making navigation and searching seamless. Let’s explore how to use these tools with your simple-repo example, starting with setting up local branches for feature-branch and bugfix-branch. Installing fzf and ripgrep on Ubuntu To install both tools on Ubuntu, use the following commands: sudo apt update sudo apt install fzf ripgrep -y Verify the installations: fzf --version rg --version What Are Fuzzy Finder and Ripgrep? Fuzzy Finder (fzf): A command-line fuzzy finder that helps you quickly search and navigate through lists, including files, Git commits, branches, and more. Ripgrep (rg): A blazing-fast search tool for finding text within files. It is significantly faster than traditional tools like grep and respects .gitignore by default. Using fzf and rg in Your Git Workflow Let’s use your simple-repo as an example to demonstrate how these tools can enhance your workflow. 1. Cloning the Repository Clone your repository from GitHub: git clone https://github.com/markbosire/simple-repo.git cd simple-repo 2. Setting Up Local Branches After cloning, you’ll only have the master branch locally. To create local branches for feature-branch and bugfix-branch that track their remote counterparts, use the following commands: git checkout -b feature-branch origin/feature-branch git checkout -b bugfix-branch origin/bugfix-branch Now, when you run git branch, you’ll see: bugfix-branch feature-branch * master 3. Finding and Checking Out Branches Instead of manually typing out branch names, use fzf to interactively search and switch branches. List and Checkout Local Branches git branch | fzf | xargs git checkout Example Usage If you type feature, fzf will filter branches like feature-branch. Selecting it will check out that branch. 4. Searching Through Git History Searching through commit messages can be tedious with git log. Use fzf to search through your commit history and checkout a commit: git log --oneline --graph --decorate --color --all | fzf | cut -d' ' -f1 | xargs git checkout Example Usage If you type feature, fzf will filter commits related to the feature-branch. Selecting a commit will check it out. 5. Searching for a File in the Repository If you need to find a file quickly within your simple-repo: git ls-files | fzf To open the selected file in VS Code: git ls-files | fzf | xargs code Example Usage If you type file, fzf will filter files like file1.txt and file2.txt. Selecting one will open it in VS Code. 6. Finding Specific Code Snippets with Ripgrep When searching for code snippets within your repository, ripgrep (rg) is much faster than grep. For example, to find occurrences of Hello in your simple-repo: rg Hello Example Usage If you type Hello, ripgrep will list occurrences across files. Expected Output: file1.txt:1:Hello, World! To integrate ripgrep with fzf for interactive searching: rg --files | fzf --preview='rg --color=always --line-number {}' 7. Searching for Changed Files in Git To search within modified or staged files: git diff --name-only | fzf For searching unstaged changes: git status -s | awk '{print $2}' | fzf Example Usage If you modify file1.txt and type file, fzf will show the modified file. 8. Grepping Through Git-Tracked Files Only By default, ripgrep searches all files, including ignored ones. To limit it to only Git-tracked files: rg --files | fzf --preview='rg --color=always --line-number {}' Alternatively, to search for a specific pattern in your simple-repo: rg "simple" $(git ls-files) Example Usage If you type simple, ripgrep will show occurrences in file2.txt. Expected Output: file2.txt:1:This is a simple repository. 9. Interactive Staging Staging selected files interactively: git status -s | fzf | awk '{print $2}' | xargs git add Example Usage If you modify file1.txt and type file, fzf will show the modified file. Selecting it will stage the file. Conclusion Using fzf and ripgrep in your Git workflow can greatly improve your efficiency, making it easier to navigate repositories, find relevant information, and streamline daily tasks. By integrating these tools into your workflow with your simple-repo, you can reduce friction and focus more on coding rather than searching for files or commits.
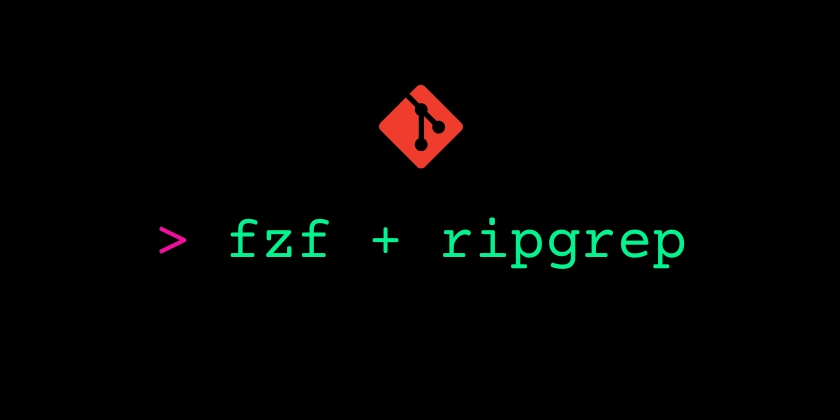
In a fast-paced development environment, efficiency is key. Searching for files, commits, or code snippets in a Git repository can be time-consuming if you're relying solely on built-in Git commands. Fortunately, tools like Fuzzy Finder (fzf) and Ripgrep (rg) can significantly enhance your Git workflow, making navigation and searching seamless.
Let’s explore how to use these tools with your simple-repo example, starting with setting up local branches for feature-branch
and bugfix-branch
.
Installing fzf and ripgrep on Ubuntu
To install both tools on Ubuntu, use the following commands:
sudo apt update
sudo apt install fzf ripgrep -y
Verify the installations:
fzf --version
rg --version
What Are Fuzzy Finder and Ripgrep?
- Fuzzy Finder (fzf): A command-line fuzzy finder that helps you quickly search and navigate through lists, including files, Git commits, branches, and more.
-
Ripgrep (rg): A blazing-fast search tool for finding text within files. It is significantly faster than traditional tools like
grep
and respects.gitignore
by default.
Using fzf and rg in Your Git Workflow
Let’s use your simple-repo as an example to demonstrate how these tools can enhance your workflow.
1. Cloning the Repository
Clone your repository from GitHub:
git clone https://github.com/markbosire/simple-repo.git
cd simple-repo
2. Setting Up Local Branches
After cloning, you’ll only have the master
branch locally. To create local branches for feature-branch
and bugfix-branch
that track their remote counterparts, use the following commands:
git checkout -b feature-branch origin/feature-branch
git checkout -b bugfix-branch origin/bugfix-branch
Now, when you run git branch
, you’ll see:
bugfix-branch
feature-branch
* master
3. Finding and Checking Out Branches
Instead of manually typing out branch names, use fzf to interactively search and switch branches.
List and Checkout Local Branches
git branch | fzf | xargs git checkout
Example Usage
If you type feature
, fzf will filter branches like feature-branch
. Selecting it will check out that branch.
4. Searching Through Git History
Searching through commit messages can be tedious with git log
. Use fzf to search through your commit history and checkout a commit:
git log --oneline --graph --decorate --color --all | fzf | cut -d' ' -f1 | xargs git checkout
Example Usage
If you type feature
, fzf will filter commits related to the feature-branch
. Selecting a commit will check it out.
5. Searching for a File in the Repository
If you need to find a file quickly within your simple-repo:
git ls-files | fzf
To open the selected file in VS Code:
git ls-files | fzf | xargs code
Example Usage
If you type file
, fzf will filter files like file1.txt
and file2.txt
. Selecting one will open it in VS Code.
6. Finding Specific Code Snippets with Ripgrep
When searching for code snippets within your repository, ripgrep (rg) is much faster than grep
. For example, to find occurrences of Hello
in your simple-repo:
rg Hello
Example Usage
If you type Hello
, ripgrep will list occurrences across files.
Expected Output:
file1.txt:1:Hello, World!
To integrate ripgrep with fzf for interactive searching:
rg --files | fzf --preview='rg --color=always --line-number {}'
7. Searching for Changed Files in Git
To search within modified or staged files:
git diff --name-only | fzf
For searching unstaged changes:
git status -s | awk '{print $2}' | fzf
Example Usage
If you modify file1.txt
and type file
, fzf will show the modified file.
8. Grepping Through Git-Tracked Files Only
By default, ripgrep searches all files, including ignored ones. To limit it to only Git-tracked files:
rg --files | fzf --preview='rg --color=always --line-number {}'
Alternatively, to search for a specific pattern in your simple-repo:
rg "simple" $(git ls-files)
Example Usage
If you type simple
, ripgrep will show occurrences in file2.txt
.
Expected Output:
file2.txt:1:This is a simple repository.
9. Interactive Staging
Staging selected files interactively:
git status -s | fzf | awk '{print $2}' | xargs git add
Example Usage
If you modify file1.txt
and type file
, fzf will show the modified file. Selecting it will stage the file.
Conclusion
Using fzf and ripgrep in your Git workflow can greatly improve your efficiency, making it easier to navigate repositories, find relevant information, and streamline daily tasks. By integrating these tools into your workflow with your simple-repo, you can reduce friction and focus more on coding rather than searching for files or commits.