Day 14 Java Learning:
What Is Constructor Chaining? Constructor chaining is the practice of calling one constructor from another within the same class (using this(...)) or calling a superclass’s constructor from a subclass (using super(...)). It ensures that initialization flows in a clear, hierarchical manner: Within the Same Class A constructor calls another constructor in the same class: public class Person { private String name; private int age; public Person() { this("Unknown"); // chain to single-arg } public Person(String name) { this(name, 0); // chain to two-arg } public Person(String name, int age) { this.name = name; // final initialization this.age = age; } } Between Class and Superclass A subclass constructor invokes its superclass constructor: class Animal { Animal(String species) { … } } class Dog extends Animal { Dog() { super("Canine"); // calls Animal(String) } } Why Use Constructor Chaining? Avoid Duplication of Initialization Code Common setup logic lives in one “ultimate” constructor, preventing you from copying field assignments across multiple constructors. Enforce Initialization Order Ensures that superclass state is set up before subclass extends or overrides it, and that within-class defaults are applied before more detailed constructors run. Clean, Maintainable Code When you add or change a field, you only update initialization in one place. When and Where to Use It Multiple Constructors with Shared Logic Whenever more than one constructor needs to perform some common setup (e.g., defaulting fields, logging construction), chain to a primary constructor rather than duplicating code. Inheritance Hierarchies Always call super(...) in subclass constructors to ensure the parent class’s initialization runs. If you omit super(...), the compiler inserts a no‑arg super()—so be explicit when the superclass has only parameterized constructors. Immutable Classes In classes with final fields, chaining lets you funnel all construction through a single constructor that assigns every final field exactly once. Rules of Constructor Chaining this(...) or super(...) Must Be First Statement In any constructor, you can either call another constructor in the same class (this(...)) or call a superclass constructor (super(...)), but not both, and it must appear before any other code. Cannot Combine this and super in Same Constructor You may only choose one. If you call this(...), that constructor in turn will (eventually) call super(...) or rely on the implicit no‑arg super(). No Circular Chains Constructor calls must eventually terminate in a constructor that doesn’t chain further within the class (and only calls super or nothing). Default super() Insertion If you don’t explicitly call super(...), the compiler inserts a no‑arg super()—which fails at compile time if the superclass lacks a no‑arg constructor. Example Putting It All Together class Vehicle { private String type; Vehicle(String type) { this.type = type; } } class Car extends Vehicle { private String model; private int year; public Car() { this("Sedan"); // calls Car(String) } public Car(String model) { this(model, 2025); // calls Car(String,int) } public Car(String model, int year) { super("Car"); // calls Vehicle(String) this.model = model; this.year = year; } } new Car() → Car() → Car("Sedan") → Car("Sedan", 2025) → super("Car") Ensures Vehicle is initialized first, then Car fields. Day 14 Key Takeaways Constructor chaining uses this(...) for intra-class and super(...) for inter-class calls. Why? To centralize initialization, avoid duplication, and enforce order. Rules? Chain call first, choose either this or super, no circular calls, and rely on implicit super() if omitted.
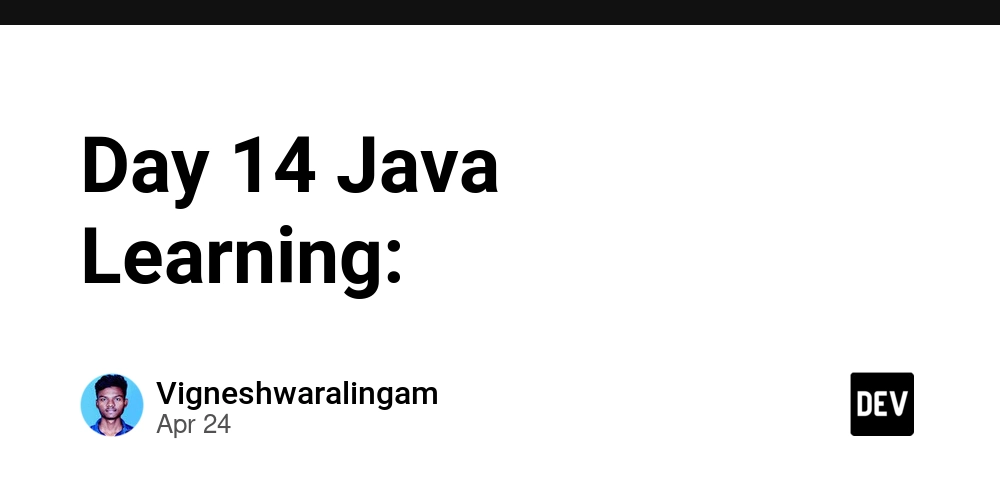
What Is Constructor Chaining?
Constructor chaining is the practice of calling one constructor from another within the same class (using this(...)
) or calling a superclass’s constructor from a subclass (using super(...)
). It ensures that initialization flows in a clear, hierarchical manner:
- Within the Same Class A constructor calls another constructor in the same class:
public class Person {
private String name;
private int age;
public Person() {
this("Unknown"); // chain to single-arg
}
public Person(String name) {
this(name, 0); // chain to two-arg
}
public Person(String name, int age) {
this.name = name; // final initialization
this.age = age;
}
}
- Between Class and Superclass A subclass constructor invokes its superclass constructor:
class Animal {
Animal(String species) { … }
}
class Dog extends Animal {
Dog() {
super("Canine"); // calls Animal(String)
}
}
Why Use Constructor Chaining?
- Avoid Duplication of Initialization Code Common setup logic lives in one “ultimate” constructor, preventing you from copying field assignments across multiple constructors.
- Enforce Initialization Order Ensures that superclass state is set up before subclass extends or overrides it, and that within-class defaults are applied before more detailed constructors run.
- Clean, Maintainable Code When you add or change a field, you only update initialization in one place.
When and Where to Use It
- Multiple Constructors with Shared Logic Whenever more than one constructor needs to perform some common setup (e.g., defaulting fields, logging construction), chain to a primary constructor rather than duplicating code.
-
Inheritance Hierarchies
Always call
super(...)
in subclass constructors to ensure the parent class’s initialization runs. If you omitsuper(...)
, the compiler inserts a no‑argsuper()
—so be explicit when the superclass has only parameterized constructors. -
Immutable Classes
In classes with
final
fields, chaining lets you funnel all construction through a single constructor that assigns everyfinal
field exactly once.
Rules of Constructor Chaining
-
this(...)
orsuper(...)
Must Be First Statement- In any constructor, you can either call another constructor in the same class (
this(...)
) or call a superclass constructor (super(...)
), but not both, and it must appear before any other code.
- In any constructor, you can either call another constructor in the same class (
-
Cannot Combine
this
andsuper
in Same Constructor You may only choose one. If you callthis(...)
, that constructor in turn will (eventually) callsuper(...)
or rely on the implicit no‑argsuper()
. -
No Circular Chains
Constructor calls must eventually terminate in a constructor that doesn’t chain further within the class (and only calls
super
or nothing). -
Default
super()
Insertion If you don’t explicitly callsuper(...)
, the compiler inserts a no‑argsuper()
—which fails at compile time if the superclass lacks a no‑arg constructor.
Example Putting It All Together
class Vehicle {
private String type;
Vehicle(String type) {
this.type = type;
}
}
class Car extends Vehicle {
private String model;
private int year;
public Car() {
this("Sedan"); // calls Car(String)
}
public Car(String model) {
this(model, 2025); // calls Car(String,int)
}
public Car(String model, int year) {
super("Car"); // calls Vehicle(String)
this.model = model;
this.year = year;
}
}
-
new Car()
→Car()
→Car("Sedan")
→Car("Sedan", 2025)
→super("Car")
- Ensures Vehicle is initialized first, then Car fields.
Day 14 Key Takeaways
-
Constructor chaining uses
this(...)
for intra-class andsuper(...)
for inter-class calls. - Why? To centralize initialization, avoid duplication, and enforce order.
-
Rules? Chain call first, choose either
this
orsuper
, no circular calls, and rely on implicitsuper()
if omitted.