Common Security Vulnerabilities in Angular Applications and How to Fix Them
In this article, we’ll explore common security issues in Angular applications, how attackers exploit them, and how to secure your code. 1. Cross-Site Scripting (XSS) XSS occurs when an application allows malicious scripts to execute in the browser. Angular sanitization is a built-in mechanisms that checks untrusted content and ensures it’s safe for the DOM. Angular cleans HTML by removing dangerous tags like , blocks unsafe URLs (javascript: schemes), and prevents harmful CSS. Angular automatically sanitizes values bound [innerHTML], , , and [style] to protect your app. However, you can still introduce vulnerabilities if you intentionally bypass security mechanisms or manipulate the DOM directly. Here are some examples of vulnerable code: Vulnerability 1: Using bypassSecurityTrustHtml Unsafely The bypassSecurityTrustHtml method forces Angular to trust the provided HTML, including unsafe content like tags. Angular’s DomSanitizer can be misused to allow malicious scripts. Vulnerable Code: import { Component } from '@angular/core'; import { DomSanitizer, SafeHtml } from '@angular/platform-browser'; @Component({ selector: 'app-inner-html-binding', template: ``, }) export class InnerHtmlBindingComponent { trustedHtmlSnippet: SafeHtml; constructor(private sanitizer: DomSanitizer) { const potentiallyUnsafe = 'Template alert("0wned")'; this.trustedHtmlSnippet = this.sanitizer.bypassSecurityTrustHtml(potentiallyUnsafe); } } Safer Approach: Instead of bypassing security, let Angular sanitize the HTML automatically: import { Component } from '@angular/core'; @Component({ selector: 'app-inner-html-binding', template: ``, }) export class InnerHtmlBindingComponent { safeHtmlSnippet = 'Template alert("0wned")'; // Angular will sanitize this automatically } Vulnerability 2: Directly Manipulating the DOM (ElementRef) Angular’s built-in XSS protection only works in templates, but direct DOM manipulation bypasses it. Using ElementRef and innerHTML allows unsafe content insertion. Vulnerable Code: import { Component, ElementRef, ViewChild } from '@angular/core'; import { FormsModule } from '@angular/forms'; @Component({ selector: 'app-root', imports: [FormsModule], template: ` Post Comment `, }) export class AppComponent { @ViewChild('commentBox', { static: true }) commentBox!: ElementRef; comment: string = ''; postComment() { this.commentBox.nativeElement.innerHTML = this.comment; // ⚠ Vulnerable to XSS! } } By default, modern browsers block scripts inserted via innerHTML (they won't execute tags). However, attackers can still exploit XSS without using tags by injecting malicious event handlers like onerror, onclick, or onmouseover. Since the app is directly inserting user input into the DOM, if a user submits the comment above, the tag loads, fails (because src="x" is invalid), and triggers the onerror JavaScript, showing an alert box. This is a Cross-Site Scripting (XSS) attack. Fix: Use Angular’s Sanitization To avoid XSS, use [innerHTML] instead of ElementRef.innerHTML: import { Component } from '@angular/core'; import { FormsModule } from '@angular/forms'; @Component({ selector: 'app-root', imports: [FormsModule], template: ` Post Comment `, }) export class AppComponent { comment: string = ''; safeComment: string = ''; postComment() { this.safeComment = this.comment; // Angular sanitizes input automatically } } 2. Clickjacking Clickjacking is when someone tricks you into clicking on something different from what you think you're clicking on. For example, they might overlay a malicious button on top of a legitimate one, so when you click, you're actually interacting with something you didn't intend to. Attackers can embed your Angular app inside an and overlay a fake UI to steal user inputs. To check if your website can be embedded in an , create an HTML file (e.g., clickjack-test.html) and add this code: Example of Clickjacking in Action: 1. The Fake Job Ad A scammer creates a webpage claiming: "Earn $500 per day! Click below to apply!" This fake ad targets people searching for jobs. 2. The Clickjacking Trick The fake page loads a real job posting inside an invisible iframe. A hidden "Like" or "Follow" button is placed over the real "Apply Now" button. When the user clicks "Apply Now," they actually like or follow a scam page instead. 3. The Redirection to Avoid Suspicion After the hidden click happens, the user is redirected to the real job listing. The victim sees a legitimate job post, making them think everything is normal. They never realize they just followed a scam page! Why Scammers Use Clickjacking: They collect thousands of likes or follows for credibility. They post fake job offers to lure victims. Users click on scam links, thinking they are real opportunities. Some are tricked into paying f
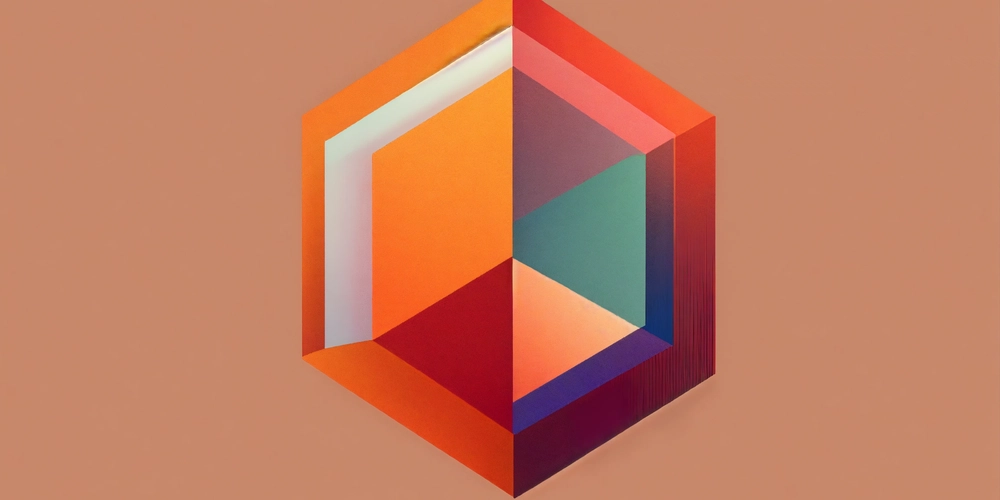
In this article, we’ll explore common security issues in Angular applications, how attackers exploit them, and how to secure your code.
1. Cross-Site Scripting (XSS)
XSS occurs when an application allows malicious scripts to execute in the browser.
Angular sanitization is a built-in mechanisms that checks untrusted content and ensures it’s safe for the DOM.
Angular cleans HTML by removing dangerous tags like '; this.trustedHtmlSnippet = this.sanitizer.bypassSecurityTrustHtml(potentiallyUnsafe); } }
Safer Approach:
Instead of bypassing security, let Angular sanitize the HTML automatically:
import { Component } from '@angular/core';
@Component({
selector: 'app-inner-html-binding',
template: ``,
})
export class InnerHtmlBindingComponent {
safeHtmlSnippet = 'Template '; // Angular will sanitize this automatically
}
Vulnerability 2: Directly Manipulating the DOM (ElementRef)
Angular’s built-in XSS protection only works in templates, but direct DOM manipulation bypasses it.
Using ElementRef and innerHTML allows unsafe content insertion.
Vulnerable Code:
import { Component, ElementRef, ViewChild } from '@angular/core';
import { FormsModule } from '@angular/forms';
@Component({
selector: 'app-root',
imports: [FormsModule],
template: `
`,
})
export class AppComponent {
@ViewChild('commentBox', { static: true }) commentBox!: ElementRef;
comment: string = '';
postComment() {
this.commentBox.nativeElement.innerHTML = this.comment; // ⚠ Vulnerable to XSS!
}
}
By default, modern browsers block scripts inserted via innerHTML (they won't execute