Building Full-Stack Web Applications with JavaScript and AI
When you first start learning JavaScript, it can feel like a huge task to tackle. There’s so much to learn: from handling front-end interactions with users, to managing back-end logic and storing data. But here’s the good news — JavaScript makes it possible to do all of that with just one language, and with AI, the process of learning and coding has never been more efficient and enjoyable. In this post, we’re diving into how JavaScript powers both the front and back end of web applications. We’ll show you how you can build full-stack apps using JavaScript and how AI tools can make the whole process easier — from learning faster to troubleshooting when things go wrong. The power of full-stack development with javascript Full-stack development means you’re in charge of everything: the back-end server that handles data, and the front-end user interface that lets people interact with your app. When you use JavaScript for both, the whole development process becomes a lot more streamlined. For example, Node.js is a server-side runtime that lets you run JavaScript outside the browser, and with Express.js, you can easily build web servers and APIs. By using the same language on both the client and server, you eliminate the need to learn multiple languages. With AI tools like ChatGPT or GitHub Copilot, you can speed up this process even more. Instead of spending hours looking for solutions, you can ask AI exactly what you need and get fast, working code that helps you learn by doing. Node.js and Express.js Let’s start with the back-end. Node.js is what lets you run JavaScript on the server, and Express.js is a framework that simplifies building web servers. Here’s a basic example: let’s say you want to set up a simple server that sends “Hello, World!” when someone visits your site. With just a few lines of code, you can achieve that: const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); }); With Express, you can easily define routes (like / for home, /about for an about page) and start handling requests. But as your project grows, Express will help you manage more complicated tasks like user authentication, handling form submissions, and interacting with databases. Database integration: MongoDB Now, let’s talk about how to store and manage data. In full-stack development, you’ll need a database to save things like user accounts, blog posts, or any other information you want to persist across sessions. For JavaScript-based applications, MongoDB is a fantastic choice. MongoDB is a NoSQL database, which means it stores data in a flexible, document-based format (think JSON). This makes it perfect for JavaScript apps since JavaScript’s native format is JSON. To get started with MongoDB, you’d typically use Mongoose, a JavaScript library that helps you manage your database. Mongoose allows you to define data models and perform queries asynchronously, making your database interactions smooth and efficient. Creating full-stack web applications with AI Once you’ve got your server and database set up, it’s time to create a dynamic, interactive user interface for your users to interact with. JavaScript lets you manage both the front-end (what users see and interact with) and the back-end (what happens behind the scenes) of your web application. Here’s how you can approach it: Set up the back-end with Node.js and Express to handle requests and manage data. Build the front-end with JavaScript, HTML, and CSS to make the user interface interactive. Connect the front-end to the back-end by making API requests using AJAX or Fetch. Deploy your app on platforms like Heroku or Netlify. With AI, you can generate code snippets for repetitive tasks, troubleshoot errors, or even get suggestions on how to improve the performance of your app. Check your JavaScript skills We’ve put together a short skill check to help you assess where you are. It covers the essentials—just enough to let you know if you should review a few things or move ahead. You can take the assessment at this link. Final thoughts So here we are. If you’ve followed along from the beginning of this series, you’ve covered a lot — starting with the basics of JavaScript and working your way toward building full applications, front to back. That’s no small thing. At this point, you’ve seen how JavaScript works on both ends of a web project. You’ve written your first functions, made decisions with control statements, worked with data using arrays and objects, and connected your app to servers and databases. And you’ve seen how AI tools can help you out along the way — when you get stuck, need a code example, or just want to move a little faster. But this isn’t the end. If anything, it’s the start of whatever you de
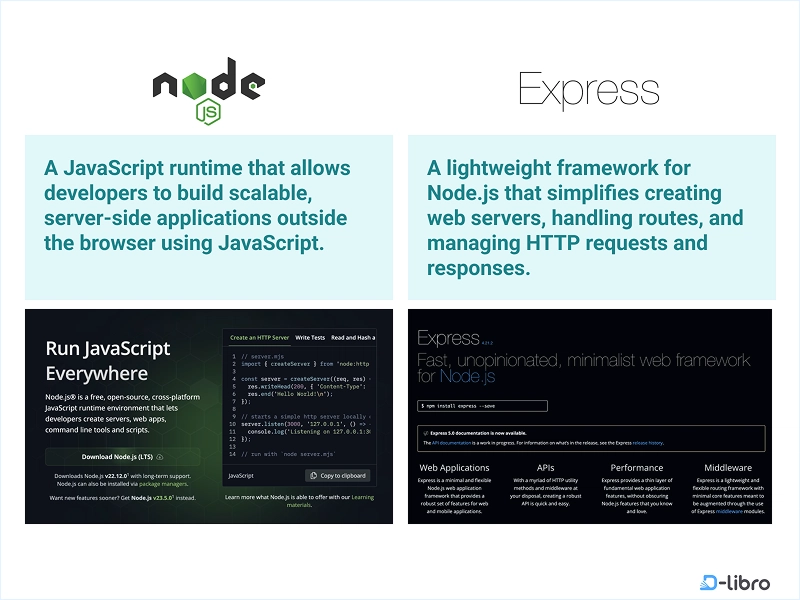
When you first start learning JavaScript, it can feel like a huge task to tackle. There’s so much to learn: from handling front-end interactions with users, to managing back-end logic and storing data. But here’s the good news — JavaScript makes it possible to do all of that with just one language, and with AI, the process of learning and coding has never been more efficient and enjoyable.
In this post, we’re diving into how JavaScript powers both the front and back end of web applications. We’ll show you how you can build full-stack apps using JavaScript and how AI tools can make the whole process easier — from learning faster to troubleshooting when things go wrong.
The power of full-stack development with javascript
Full-stack development means you’re in charge of everything: the back-end server that handles data, and the front-end user interface that lets people interact with your app. When you use JavaScript for both, the whole development process becomes a lot more streamlined.
For example, Node.js is a server-side runtime that lets you run JavaScript outside the browser, and with Express.js, you can easily build web servers and APIs. By using the same language on both the client and server, you eliminate the need to learn multiple languages.
With AI tools like ChatGPT or GitHub Copilot, you can speed up this process even more. Instead of spending hours looking for solutions, you can ask AI exactly what you need and get fast, working code that helps you learn by doing.
Node.js and Express.js
Let’s start with the back-end. Node.js is what lets you run JavaScript on the server, and Express.js is a framework that simplifies building web servers.
Here’s a basic example: let’s say you want to set up a simple server that sends “Hello, World!” when someone visits your site. With just a few lines of code, you can achieve that:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
With Express, you can easily define routes (like / for home, /about for an about page) and start handling requests. But as your project grows, Express will help you manage more complicated tasks like user authentication, handling form submissions, and interacting with databases.
Database integration: MongoDB
Now, let’s talk about how to store and manage data. In full-stack development, you’ll need a database to save things like user accounts, blog posts, or any other information you want to persist across sessions. For JavaScript-based applications, MongoDB is a fantastic choice.
MongoDB is a NoSQL database, which means it stores data in a flexible, document-based format (think JSON). This makes it perfect for JavaScript apps since JavaScript’s native format is JSON.
To get started with MongoDB, you’d typically use Mongoose, a JavaScript library that helps you manage your database. Mongoose allows you to define data models and perform queries asynchronously, making your database interactions smooth and efficient.
Creating full-stack web applications with AI
Once you’ve got your server and database set up, it’s time to create a dynamic, interactive user interface for your users to interact with. JavaScript lets you manage both the front-end (what users see and interact with) and the back-end (what happens behind the scenes) of your web application.
Here’s how you can approach it:
Set up the back-end with Node.js and Express to handle requests and manage data.
Build the front-end with JavaScript, HTML, and CSS to make the user interface interactive.
Connect the front-end to the back-end by making API requests using AJAX or Fetch.
Deploy your app on platforms like Heroku or Netlify.
With AI, you can generate code snippets for repetitive tasks, troubleshoot errors, or even get suggestions on how to improve the performance of your app.
Check your JavaScript skills
We’ve put together a short skill check to help you assess where you are. It covers the essentials—just enough to let you know if you should review a few things or move ahead.
You can take the assessment at this link.
Final thoughts
So here we are. If you’ve followed along from the beginning of this series, you’ve covered a lot — starting with the basics of JavaScript and working your way toward building full applications, front to back. That’s no small thing.
At this point, you’ve seen how JavaScript works on both ends of a web project. You’ve written your first functions, made decisions with control statements, worked with data using arrays and objects, and connected your app to servers and databases. And you’ve seen how AI tools can help you out along the way — when you get stuck, need a code example, or just want to move a little faster.
But this isn’t the end. If anything, it’s the start of whatever you decide to build next.
You don’t need to know everything right now. No one does. What matters is that you’ve built a strong foundation — and that you’re not afraid to try new things. Build a small app. Break it. Fix it. Repeat. That’s how most people get better at this.
And if you ever feel overwhelmed, just go back to something simple. A button. A form. A short script. Keep it light. Keep going.
Thanks for sticking with this series. We hope it helped you feel a bit more confident — and a lot less alone — on your coding journey.
This article is a summary of “Learn JavaScript with AI — A Smarter Way to Code” by D-Libro.