Understanding Functional Programming in Haskell
Understanding Functional Programming in Haskell Functional programming (FP) is a paradigm that treats computation as the evaluation of mathematical functions, avoiding mutable state and side effects. Haskell, a purely functional language, is one of the best ways to explore this paradigm. In this article, we’ll dive into the core concepts of functional programming in Haskell, explore its key features, and provide practical code examples. If you're looking to grow your YouTube channel while learning Haskell or other programming topics, consider checking out MediaGeneous for expert strategies. What Makes Haskell Unique? Haskell is a statically typed, purely functional language with lazy evaluation and strong type inference. Unlike imperative languages (e.g., Python, Java), Haskell emphasizes: Immutability – Data structures cannot be modified after creation. Referential Transparency – Functions always return the same output for the same input. Higher-Order Functions – Functions can take other functions as arguments. Key Features of Haskell 1. Pure Functions In Haskell, functions have no side effects—they don’t modify global state or perform I/O (unless explicitly allowed via monads). haskell Copy Download -- A pure function add :: Int -> Int -> Int add x y = x + y 2. Lazy Evaluation Haskell evaluates expressions only when needed, improving performance for infinite data structures. haskell Copy Download -- Infinite list of ones ones :: [Int] ones = 1 : ones -- Take the first 5 elements take 5 ones -- [1,1,1,1,1] 3. Strong Static Typing Haskell’s type system prevents many runtime errors. haskell Copy Download -- Type signature (optional but recommended) square :: Num a => a -> a square x = x * x 4. Pattern Matching Instead of imperative if-else chains, Haskell uses pattern matching. haskell Copy Download factorial :: Integer -> Integer factorial 0 = 1 factorial n = n * factorial (n - 1) 5. Monads for Side Effects Haskell uses monads (like IO) to handle side effects in a controlled way. haskell Copy Download -- A simple IO action greet :: IO () greet = do putStrLn "What's your name?" name Int sumList [] = 0 sumList (x:xs) = x + sumList xs 2. Higher-Order Functions Functions like map, filter, and fold are fundamental. haskell Copy Download -- Double each element in a list doubleAll :: [Int] -> [Int] doubleAll = map (*2) -- Keep only even numbers evens :: [Int] -> [Int] evens = filter even 3. Currying and Partial Application Haskell functions are curried by default. haskell Copy Download -- Currying in action addFive :: Int -> Int addFive = add 5 -- Partial application -- Usage addFive 3 -- 8 Resources for Learning Haskell Haskell Official Site Learn You a Haskell for Great Good! (Free book) Haskell Wiki Conclusion Haskell offers a deep dive into functional programming with its emphasis on purity, immutability, and strong typing. While the learning curve can be steep, mastering Haskell improves problem-solving skills and provides a fresh perspective on programming. For those interested in creating programming tutorials, growing an audience is key. Platforms like MediaGeneous can help optimize your YouTube channel’s growth. Happy Haskelling!
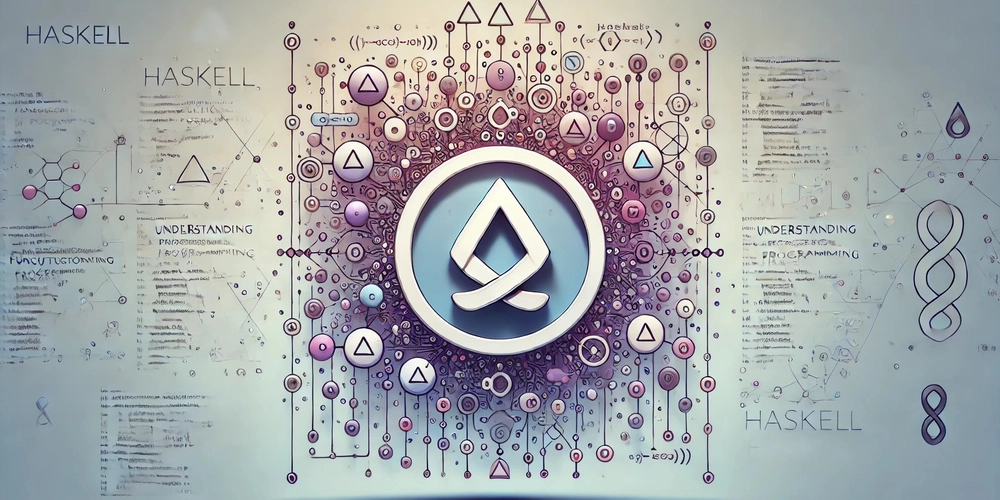
Understanding Functional Programming in Haskell
Functional programming (FP) is a paradigm that treats computation as the evaluation of mathematical functions, avoiding mutable state and side effects. Haskell, a purely functional language, is one of the best ways to explore this paradigm. In this article, we’ll dive into the core concepts of functional programming in Haskell, explore its key features, and provide practical code examples.
If you're looking to grow your YouTube channel while learning Haskell or other programming topics, consider checking out MediaGeneous for expert strategies.
What Makes Haskell Unique?
Haskell is a statically typed, purely functional language with lazy evaluation and strong type inference. Unlike imperative languages (e.g., Python, Java), Haskell emphasizes:
-
Immutability – Data structures cannot be modified after creation.
-
Referential Transparency – Functions always return the same output for the same input.
-
Higher-Order Functions – Functions can take other functions as arguments.
Key Features of Haskell
1. Pure Functions
In Haskell, functions have no side effects—they don’t modify global state or perform I/O (unless explicitly allowed via monads).
haskell
Copy
Download
-- A pure function add :: Int -> Int -> Int add x y = x + y
2. Lazy Evaluation
Haskell evaluates expressions only when needed, improving performance for infinite data structures.
haskell
Copy
Download
-- Infinite list of ones ones :: [Int] ones = 1 : ones -- Take the first 5 elements take 5 ones -- [1,1,1,1,1]
3. Strong Static Typing
Haskell’s type system prevents many runtime errors.
haskell
Copy
Download
-- Type signature (optional but recommended) square :: Num a => a -> a square x = x * x
4. Pattern Matching
Instead of imperative if-else
chains, Haskell uses pattern matching.
haskell
Copy
Download
factorial :: Integer -> Integer factorial 0 = 1 factorial n = n * factorial (n - 1)
5. Monads for Side Effects
Haskell uses monads (like IO
) to handle side effects in a controlled way.
haskell
Copy
Download
-- A simple IO action greet :: IO () greet = do putStrLn "What's your name?" name <- getLine putStrLn ("Hello, " ++ name ++ "!")
Why Learn Functional Programming?
-
Better Abstraction – Higher-order functions reduce code duplication.
-
Easier Debugging – Pure functions simplify testing.
-
Parallelism – Immutability makes concurrency safer.
Practical Haskell Examples
1. Recursion Over Loops
Since Haskell avoids mutable variables, recursion replaces loops.
haskell
Copy
Download
sumList :: [Int] -> Int sumList [] = 0 sumList (x:xs) = x + sumList xs
2. Higher-Order Functions
Functions like map
, filter
, and fold
are fundamental.
haskell
Copy
Download
-- Double each element in a list doubleAll :: [Int] -> [Int] doubleAll = map (*2) -- Keep only even numbers evens :: [Int] -> [Int] evens = filter even
3. Currying and Partial Application
Haskell functions are curried by default.
haskell
Copy
Download
-- Currying in action addFive :: Int -> Int addFive = add 5 -- Partial application -- Usage addFive 3 -- 8
Resources for Learning Haskell
Conclusion
Haskell offers a deep dive into functional programming with its emphasis on purity, immutability, and strong typing. While the learning curve can be steep, mastering Haskell improves problem-solving skills and provides a fresh perspective on programming.
For those interested in creating programming tutorials, growing an audience is key. Platforms like MediaGeneous can help optimize your YouTube channel’s growth.
Happy Haskelling!