Tutorial 22: Using SF Symbols and Custom Icons in Your App
Table of Contents Introduction to SF Symbols Setting Up Your Xcode Project Working with SF Symbols in SwiftUI Customizing SF Symbols Adding Custom Icons to Your App Building a Cute SF Symbols Game Animating Symbols and Icons Exporting and Optimizing Icons Best Practices and Accessibility Considerations Conclusion and Next Steps 1. Introduction to SF Symbols SF Symbols is Apple's library of vector-based icons that seamlessly integrate with iOS, iPadOS, and macOS applications. In this tutorial, we'll explore how to use SF Symbols, customize them, and add custom icons to your app. We'll also build a small game using SF Symbols. 2. Setting Up Your Xcode Project Open Xcode and create a new SwiftUI project. Select "App" under iOS and choose SwiftUI as the interface. Name the project "SFSymbolsGame." Set up basic project configurations. 3. Working with SF Symbols in SwiftUI import SwiftUI struct ContentView: View { var body: some View { VStack { Image(systemName: "star.fill") .font(.system(size: 50)) .foregroundColor(.yellow) Text("Welcome to SF Symbols Game!") .font(.title) .padding() } } } struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } } This displays an SF Symbol and a text label. 4. Customizing SF Symbols Use .font(.system(size:)) to change size. Apply .foregroundColor() for colors. Use .symbolRenderingMode(.hierarchical) for depth effects. 5. Adding Custom Icons to Your App Create or download a custom icon in SVG or PNG. Import it into the asset catalog. Reference it using Image("custom_icon"). 6. Building a Cute SF Symbols Game We'll build a simple game where users tap symbols to score points. Game Logic import SwiftUI struct GameView: View { @State private var score = 0 @State private var symbolName = "star" let symbols = ["star", "heart.fill", "bolt.fill", "flame.fill", "leaf.fill"] var body: some View { VStack { Text("Score: \(score)") .font(.largeTitle) .padding() Image(systemName: symbolName) .font(.system(size: 100)) .foregroundColor(.blue) .onTapGesture { score += 1 symbolName = symbols.randomElement() ?? "star" } Text("Tap the symbol to score!") .font(.headline) .padding() } } } struct GameView_Previews: PreviewProvider { static var previews: some View { GameView() } } Features A randomly changing SF Symbol. Score tracking. Simple tap gesture mechanics. 7. Animating Symbols and Icons Use withAnimation to animate SF Symbols smoothly: withAnimation { symbolName = symbols.randomElement() ?? "star" } 8. Exporting and Optimizing Icons Use Apple's SF Symbols app to export and tweak symbols. 9. Best Practices and Accessibility Considerations Use accessibilityLabel("Star icon") for VoiceOver support. Ensure contrast and clarity for different themes. 10. Conclusion and Next Steps We explored SF Symbols, custom icons, and built a fun game. Next, try adding more animations and sound effects! This tutorial provides the foundation for a one-hour video tutorial, including coding demonstrations and explanations.
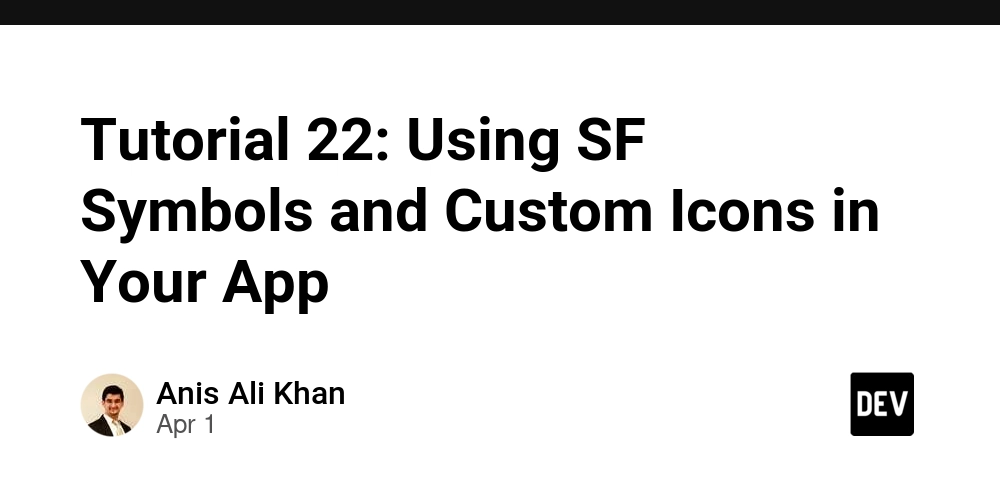
Table of Contents
- Introduction to SF Symbols
- Setting Up Your Xcode Project
- Working with SF Symbols in SwiftUI
- Customizing SF Symbols
- Adding Custom Icons to Your App
- Building a Cute SF Symbols Game
- Animating Symbols and Icons
- Exporting and Optimizing Icons
- Best Practices and Accessibility Considerations
- Conclusion and Next Steps
1. Introduction to SF Symbols
SF Symbols is Apple's library of vector-based icons that seamlessly integrate with iOS, iPadOS, and macOS applications. In this tutorial, we'll explore how to use SF Symbols, customize them, and add custom icons to your app. We'll also build a small game using SF Symbols.
2. Setting Up Your Xcode Project
- Open Xcode and create a new SwiftUI project.
- Select "App" under iOS and choose SwiftUI as the interface.
- Name the project "SFSymbolsGame."
- Set up basic project configurations.
3. Working with SF Symbols in SwiftUI
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Image(systemName: "star.fill")
.font(.system(size: 50))
.foregroundColor(.yellow)
Text("Welcome to SF Symbols Game!")
.font(.title)
.padding()
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
This displays an SF Symbol and a text label.
4. Customizing SF Symbols
- Use
.font(.system(size:))
to change size. - Apply
.foregroundColor()
for colors. - Use
.symbolRenderingMode(.hierarchical)
for depth effects.
5. Adding Custom Icons to Your App
- Create or download a custom icon in SVG or PNG.
- Import it into the asset catalog.
- Reference it using
Image("custom_icon")
.
6. Building a Cute SF Symbols Game
We'll build a simple game where users tap symbols to score points.
Game Logic
import SwiftUI
struct GameView: View {
@State private var score = 0
@State private var symbolName = "star"
let symbols = ["star", "heart.fill", "bolt.fill", "flame.fill", "leaf.fill"]
var body: some View {
VStack {
Text("Score: \(score)")
.font(.largeTitle)
.padding()
Image(systemName: symbolName)
.font(.system(size: 100))
.foregroundColor(.blue)
.onTapGesture {
score += 1
symbolName = symbols.randomElement() ?? "star"
}
Text("Tap the symbol to score!")
.font(.headline)
.padding()
}
}
}
struct GameView_Previews: PreviewProvider {
static var previews: some View {
GameView()
}
}
Features
- A randomly changing SF Symbol.
- Score tracking.
- Simple tap gesture mechanics.
7. Animating Symbols and Icons
Use withAnimation
to animate SF Symbols smoothly:
withAnimation {
symbolName = symbols.randomElement() ?? "star"
}
8. Exporting and Optimizing Icons
Use Apple's SF Symbols app to export and tweak symbols.
9. Best Practices and Accessibility Considerations
- Use
accessibilityLabel("Star icon")
for VoiceOver support. - Ensure contrast and clarity for different themes.
10. Conclusion and Next Steps
We explored SF Symbols, custom icons, and built a fun game. Next, try adding more animations and sound effects!
This tutorial provides the foundation for a one-hour video tutorial, including coding demonstrations and explanations.