Maths Algorithms in Python Without Libraries (No math, pandas, or matplotlib)
In today's blog, we'll explore how to implement fundamental math algorithms in Python without using any external libraries like math, pandas, or matplotlib. This kind of practice helps sharpen your core algorithmic thinking and improves your confidence when solving coding problems, especially in interviews or constrained environments. Problem One: Check for Perfect Square Task: You are given an integer n. Your goal is to determine if n is a perfect square. A perfect square is a number that is the product of an integer with itself. For example: ✅ 1 = 1 × 1 ✅ 4 = 2 × 2 ✅ 9 = 3 × 3 ❌ 2, 3, 5 are not perfect squares ✅ Approach: Handle edge cases: If n < 0, it's not a perfect square. If n is 0 or 1, it's automatically a perfect square. Loop from 1 to n // 2 and check: If i * i == n, return True. If i * i > n, stop early and return False. Code: def is_perfect_square(n): if n n: break return False Problem Two: Find the Next Prime Number Task: Write a function next_prime(n) that returns the smallest prime number strictly greater than n. A prime number is only divisible by 1 and itself (e.g., 2, 3, 5, 7, 11...). Approach: Create a helper function is_prime(num): Return False if num < 2. Check divisibility from 2 to √num. In the next_prime function: Start from n + 1. Keep incrementing until you find a number where is_prime() returns True. Code: def is_prime(num): if num
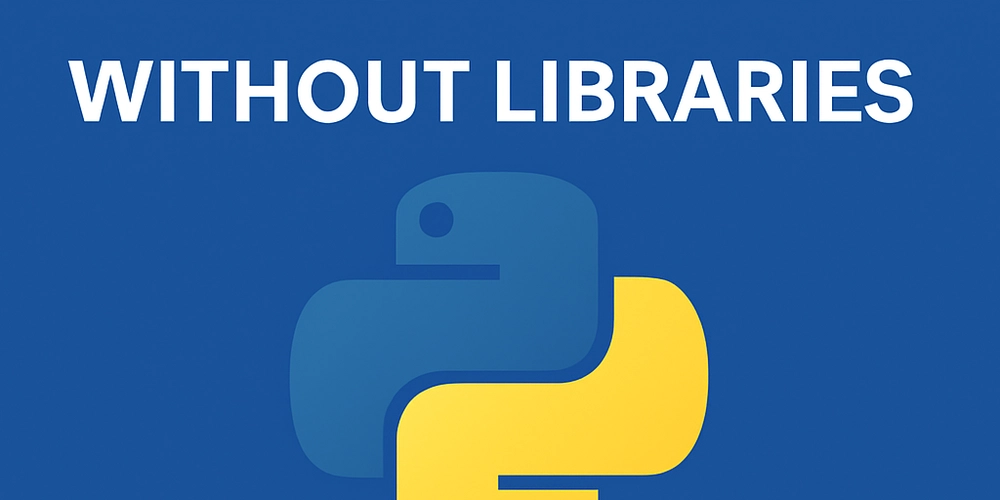
In today's blog, we'll explore how to implement fundamental math algorithms in Python without using any external libraries like math
, pandas
, or matplotlib
.
This kind of practice helps sharpen your core algorithmic thinking and improves your confidence when solving coding problems, especially in interviews or constrained environments.
Problem One: Check for Perfect Square
Task:
You are given an integer n
. Your goal is to determine if n
is a perfect square.
A perfect square is a number that is the product of an integer with itself. For example:
- ✅ 1 = 1 × 1
- ✅ 4 = 2 × 2
- ✅ 9 = 3 × 3
- ❌ 2, 3, 5 are not perfect squares
✅ Approach:
- Handle edge cases:
- If
n < 0
, it's not a perfect square. - If
n
is0
or1
, it's automatically a perfect square.
- If
- Loop from
1
ton // 2
and check:- If
i * i == n
, returnTrue
. - If
i * i > n
, stop early and returnFalse
.
- If
Code:
def is_perfect_square(n):
if n < 0:
return False
if n == 0 or n == 1:
return True
for i in range(1, n // 2 + 1):
if i * i == n:
return True
elif i * i > n:
break
return False
Problem Two: Find the Next Prime Number
Task:
Write a function next_prime(n)
that returns the smallest prime number strictly greater than n
.
A prime number is only divisible by 1 and itself (e.g., 2, 3, 5, 7, 11...).
Approach:
- Create a helper function
is_prime(num)
:- Return
False
ifnum < 2
. - Check divisibility from
2
to√num
.
- Return
- In the
next_prime
function:- Start from
n + 1
. - Keep incrementing until you find a number where
is_prime()
returnsTrue
.
- Start from
Code:
def is_prime(num):
if num < 2:
return False
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
def next_prime(n):
next_p = n + 1
while True:
if is_prime(next_p):
return next_p
next_p += 1
Practicing these foundational problems without built-in tools builds your problem-solving muscles and makes you a more versatile developer.