Mastering Database Connection Pooling in Go: Performance Best Practices
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Database connection pooling is essential for applications that make frequent database calls. In Go, connection pooling helps manage resources, improve performance, and enhance application resilience. I've spent considerable time working with database connections in production environments, and I'll share my knowledge on implementing efficient connection pooling in Go. Understanding Database Connection Pooling Connection pooling maintains a set of reusable database connections. Rather than creating a new connection for each request, applications borrow connections from the pool, use them, and return them when done. This approach significantly reduces the overhead of establishing connections. Go's standard library already provides connection pooling through the database/sql package. However, using it effectively requires understanding the configuration parameters and implementation details. Core Components of Database Connection Pooling The Go database/sql package manages connections internally. When you call db.Open(), it doesn't immediately establish a connection but sets up the pool. Connections are created when needed and returned to the pool after use. import ( "database/sql" _ "github.com/lib/pq" // PostgreSQL driver ) func main() { db, err := sql.Open("postgres", "postgres://user:password@localhost/dbname?sslmode=disable") if err != nil { log.Fatal(err) } defer db.Close() // The pool is now ready for use } Configuring the Connection Pool Proper configuration is crucial for optimal performance. Three main settings control pool behavior: // Set maximum number of open connections db.SetMaxOpenConns(25) // Set maximum number of idle connections db.SetMaxIdleConns(5) // Set maximum lifetime of a connection db.SetConnMaxLifetime(5 * time.Minute) Each setting serves a specific purpose: MaxOpenConns limits the total number of connections, preventing database overload MaxIdleConns keeps connections ready for reuse, improving response times ConnMaxLifetime ensures connections are recycled regularly, avoiding stale connections I've found that setting MaxOpenConns to match your application's concurrency level plus a small buffer works well. For MaxIdleConns, a value between 25-50% of MaxOpenConns is generally effective. Monitoring Pool Performance Monitoring is vital for optimizing connection pool settings. The database/sql package exposes statistics through the DB.Stats() method: func logDBStats(db *sql.DB) { stats := db.Stats() log.Printf( "DB Stats: Open=%d, InUse=%d, Idle=%d, WaitCount=%d, WaitDuration=%s, MaxIdleClosed=%d, MaxLifetimeClosed=%d", stats.OpenConnections, stats.InUse, stats.Idle, stats.WaitCount, stats.WaitDuration, stats.MaxIdleClosed, stats.MaxLifetimeClosed, ) } I recommend logging these metrics periodically to identify bottlenecks. High WaitCount and WaitDuration values indicate that your application needs more connections. Frequent MaxIdleClosed connections suggest your MaxIdleConns value may be too low. Building a Resilient Connection Pool Production systems need resilience against temporary database failures. Here's a pattern I've used successfully: func connectWithRetry() (*sql.DB, error) { var db *sql.DB var err error maxRetries := 5 for retries := 0; retries
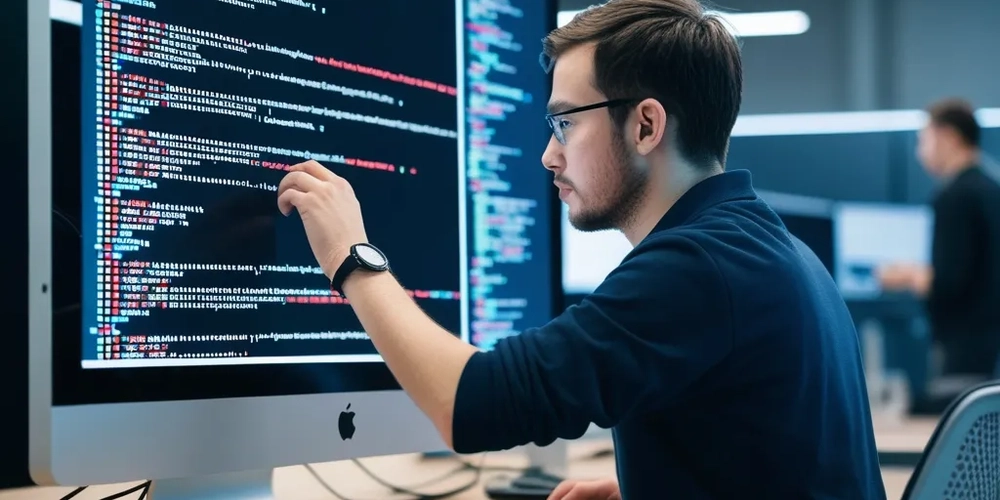
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Database connection pooling is essential for applications that make frequent database calls. In Go, connection pooling helps manage resources, improve performance, and enhance application resilience. I've spent considerable time working with database connections in production environments, and I'll share my knowledge on implementing efficient connection pooling in Go.
Understanding Database Connection Pooling
Connection pooling maintains a set of reusable database connections. Rather than creating a new connection for each request, applications borrow connections from the pool, use them, and return them when done. This approach significantly reduces the overhead of establishing connections.
Go's standard library already provides connection pooling through the database/sql
package. However, using it effectively requires understanding the configuration parameters and implementation details.
Core Components of Database Connection Pooling
The Go database/sql
package manages connections internally. When you call db.Open()
, it doesn't immediately establish a connection but sets up the pool. Connections are created when needed and returned to the pool after use.
import (
"database/sql"
_ "github.com/lib/pq" // PostgreSQL driver
)
func main() {
db, err := sql.Open("postgres", "postgres://user:password@localhost/dbname?sslmode=disable")
if err != nil {
log.Fatal(err)
}
defer db.Close()
// The pool is now ready for use
}
Configuring the Connection Pool
Proper configuration is crucial for optimal performance. Three main settings control pool behavior:
// Set maximum number of open connections
db.SetMaxOpenConns(25)
// Set maximum number of idle connections
db.SetMaxIdleConns(5)
// Set maximum lifetime of a connection
db.SetConnMaxLifetime(5 * time.Minute)
Each setting serves a specific purpose:
-
MaxOpenConns
limits the total number of connections, preventing database overload -
MaxIdleConns
keeps connections ready for reuse, improving response times -
ConnMaxLifetime
ensures connections are recycled regularly, avoiding stale connections
I've found that setting MaxOpenConns
to match your application's concurrency level plus a small buffer works well. For MaxIdleConns
, a value between 25-50% of MaxOpenConns
is generally effective.
Monitoring Pool Performance
Monitoring is vital for optimizing connection pool settings. The database/sql
package exposes statistics through the DB.Stats()
method:
func logDBStats(db *sql.DB) {
stats := db.Stats()
log.Printf(
"DB Stats: Open=%d, InUse=%d, Idle=%d, WaitCount=%d, WaitDuration=%s, MaxIdleClosed=%d, MaxLifetimeClosed=%d",
stats.OpenConnections,
stats.InUse,
stats.Idle,
stats.WaitCount,
stats.WaitDuration,
stats.MaxIdleClosed,
stats.MaxLifetimeClosed,
)
}
I recommend logging these metrics periodically to identify bottlenecks. High WaitCount
and WaitDuration
values indicate that your application needs more connections. Frequent MaxIdleClosed
connections suggest your MaxIdleConns
value may be too low.
Building a Resilient Connection Pool
Production systems need resilience against temporary database failures. Here's a pattern I've used successfully:
func connectWithRetry() (*sql.DB, error) {
var db *sql.DB
var err error
maxRetries := 5
for retries := 0; retries < maxRetries; retries++ {
db, err = sql.Open("postgres", "postgres://user:password@localhost/dbname?sslmode=disable")
if err != nil {
log.Printf("Failed to open DB: %v, retrying in %d seconds", err, retries+1)
time.Sleep(time.Duration(retries+1) * time.Second)
continue
}
// Test the connection
err = db.Ping()
if err == nil {
return db, nil
}
log.Printf("Failed to connect to DB: %v, retrying in %d seconds", err, retries+1)
time.Sleep(time.Duration(retries+1) * time.Second)
}
return nil, fmt.Errorf("failed to connect to the database after %d attempts", maxRetries)
}
This function attempts to connect multiple times with exponential backoff, making your application more robust against temporary network issues or database restarts.
Context Management for Timeouts
Using contexts with database operations helps prevent application hangs due to slow database responses:
func queryWithTimeout(db *sql.DB, query string, args ...interface{}) (*sql.Rows, error) {
// Create context with timeout
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
// Execute query with context
rows, err := db.QueryContext(ctx, query, args...)
if err != nil {
if ctx.Err() == context.DeadlineExceeded {
return nil, fmt.Errorf("query timed out after 5 seconds")
}
return nil, err
}
return rows, nil
}
This approach ensures that database operations don't block indefinitely, improving application responsiveness.
Advanced Connection Pool Implementation
For more control over connection pooling, we can build a custom wrapper that extends the standard functionality:
type DBPool struct {
db *sql.DB
metrics *Metrics
}
type Metrics struct {
mu sync.Mutex
queries int64
errors int64
totalTime time.Duration
}
func NewDBPool(dsn string) (*DBPool, error) {
db, err := sql.Open("postgres", dsn)
if err != nil {
return nil, err
}
// Configure pool
db.SetMaxOpenConns(25)
db.SetMaxIdleConns(5)
db.SetConnMaxLifetime(5 * time.Minute)
// Test connection
if err := db.Ping(); err != nil {
return nil, err
}
return &DBPool{
db: db,
metrics: &Metrics{},
}, nil
}
func (p *DBPool) Query(query string, args ...interface{}) (*sql.Rows, error) {
p.metrics.mu.Lock()
p.metrics.queries++
p.metrics.mu.Unlock()
start := time.Now()
rows, err := p.db.Query(query, args...)
p.metrics.mu.Lock()
p.metrics.totalTime += time.Since(start)
if err != nil {
p.metrics.errors++
}
p.metrics.mu.Unlock()
return rows, err
}
func (p *DBPool) Stats() (queries, errors int64, avgTime time.Duration) {
p.metrics.mu.Lock()
defer p.metrics.mu.Unlock()
avgTime = time.Duration(0)
if p.metrics.queries > 0 {
avgTime = p.metrics.totalTime / time.Duration(p.metrics.queries)
}
return p.metrics.queries, p.metrics.errors, avgTime
}
This wrapper adds performance tracking while maintaining the underlying pool functionality.
Connection Pooling with pgx
For PostgreSQL specifically, the pgx library offers enhanced connection pooling:
import (
"context"
"log"
"github.com/jackc/pgx/v4/pgxpool"
)
func main() {
config, err := pgxpool.ParseConfig("postgres://user:password@localhost:5432/database")
if err != nil {
log.Fatalf("Unable to parse connection string: %v", err)
}
// Set pool configuration
config.MaxConns = 10
config.MinConns = 2
config.MaxConnLifetime = 1 * time.Hour
config.MaxConnIdleTime = 30 * time.Minute
// Create the pool
pool, err := pgxpool.ConnectConfig(context.Background(), config)
if err != nil {
log.Fatalf("Unable to create connection pool: %v", err)
}
defer pool.Close()
// Use the pool
var greeting string
err = pool.QueryRow(context.Background(), "SELECT 'Hello, world!'").Scan(&greeting)
if err != nil {
log.Fatalf("Query failed: %v", err)
}
log.Println(greeting)
}
pgx provides valuable features like automatic statement preparation, better type handling, and more detailed metrics.
Connection Pool Management Patterns
In my experience, these patterns have proven effective for connection pool management:
Pool per service: Each microservice maintains its own connection pool sized according to its specific needs.
Health checking: Periodically test connections with lightweight queries:
func startHealthCheck(db *sql.DB, interval time.Duration) {
ticker := time.NewTicker(interval)
go func() {
for range ticker.C {
err := db.PingContext(context.Background())
if err != nil {
log.Printf("Database health check failed: %v", err)
}
}
}()
}
- Graceful shutdown: Close the pool cleanly when your application terminates:
func setupGracefulShutdown(db *sql.DB) {
c := make(chan os.Signal, 1)
signal.Notify(c, os.Interrupt, syscall.SIGTERM)
go func() {
<-c
log.Println("Closing database connections...")
db.Close()
log.Println("Database connections closed")
os.Exit(0)
}()
}
Transaction Management with Connection Pooling
Transactions require special handling with connection pools. A connection is reserved for the entire transaction duration:
func executeTransaction(db *sql.DB) error {
// Begin transaction - this acquires a connection from the pool
tx, err := db.Begin()
if err != nil {
return err
}
// Ensure connection is returned to the pool
defer func() {
if err != nil {
tx.Rollback()
return
}
}()
// Execute transaction operations
_, err = tx.Exec("INSERT INTO users(name) VALUES($1)", "Alice")
if err != nil {
return err
}
_, err = tx.Exec("UPDATE user_counts SET count = count + 1")
if err != nil {
return err
}
// Commit the transaction and return the connection to the pool
return tx.Commit()
}
Long transactions can strain your connection pool by keeping connections occupied. I recommend setting reasonable timeouts and keeping transactions brief.
Testing Connection Pool Behavior
Testing pool behavior under load is crucial. Here's a simple load test function:
func poolLoadTest(db *sql.DB, concurrency, requests int) {
var wg sync.WaitGroup
wg.Add(concurrency)
start := time.Now()
for i := 0; i < concurrency; i++ {
go func(workerID int) {
defer wg.Done()
for j := 0; j < requests; j++ {
var result int
err := db.QueryRow("SELECT pg_sleep(0.01), $1::int", workerID).Scan(&result)
if err != nil {
log.Printf("Worker %d query %d failed: %v", workerID, j, err)
}
}
}(i)
}
wg.Wait()
elapsed := time.Since(start)
log.Printf("Completed %d requests with %d concurrency in %v", concurrency*requests, concurrency, elapsed)
logDBStats(db)
}
This test simulates multiple concurrent clients making database requests. By adjusting concurrency and observing metrics, you can fine-tune your pool settings.
Common Connection Pooling Issues
From my experience, these are common issues with connection pooling:
Pool exhaustion: When all connections are in use and new requests must wait. Symptoms include increasing request latency and
WaitCount
metrics rising.Connection leaks: Connections aren't properly returned to the pool. Watch for steadily increasing
InUse
connections without corresponding traffic increases.Database overload: Too many connections overwhelming the database. Signs include database CPU spikes and connection errors.
Idle connection timeouts: The database server may close idle connections. Setting appropriate
MaxIdleConns
andConnMaxLifetime
values helps manage this.
Optimizing Connection Pool Performance
After implementing basic connection pooling, consider these optimizations:
- Connection warming: Pre-establish connections during startup:
func warmConnectionPool(db *sql.DB, n int) {
var wg sync.WaitGroup
wg.Add(n)
for i := 0; i < n; i++ {
go func() {
defer wg.Done()
db.Ping()
}()
}
wg.Wait()
log.Printf("Warmed up %d connections", n)
}
- Statement preparation: Prepare frequently used statements to reduce parsing overhead:
type PreparedQueries struct {
getUserByID *sql.Stmt
insertUser *sql.Stmt
}
func prepareStatements(db *sql.DB) (*PreparedQueries, error) {
getUserByID, err := db.Prepare("SELECT id, name FROM users WHERE id = $1")
if err != nil {
return nil, err
}
insertUser, err := db.Prepare("INSERT INTO users(name) VALUES($1) RETURNING id")
if err != nil {
getUserByID.Close()
return nil, err
}
return &PreparedQueries{
getUserByID: getUserByID,
insertUser: insertUser,
}, nil
}
- Connection labeling: Add labels to connections for debugging:
func labelConnection(ctx context.Context, conn *sql.Conn) error {
// For PostgreSQL
_, err := conn.ExecContext(ctx, "SELECT pg_catalog.set_config('application_name', 'myapp', false)")
return err
}
Conclusion
Implementing efficient database connection pooling in Go requires understanding both the database and application requirements. Start with the standard database/sql
package and adjust settings based on observed metrics. As your application grows, consider more advanced implementations with custom wrappers or specialized libraries like pgx.
Remember that connection pooling is a balance - too few connections limit throughput, while too many can overload your database. Regular monitoring and testing are essential to maintain this balance as your application evolves.
By implementing proper connection pooling, you'll create a more responsive, reliable, and resource-efficient application that scales effectively with your user base.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva