Legacy PHP Codebase: A Developer’s Worst Nightmare
The Pain of Maintaining a PHP Web Application Maintaining apps, in general, can be a headache, but PHP seems to take the crown when it comes to abandoned projects. You’d be surprised how many PHP apps are still running outdated versions of Laravel, CodeIgniter, or even pure PHP scripts written in a style that should have been buried decades ago. A client once told me, "If you can buy it, why build it?" Another said, "Use a library and modify it as needed. Don’t build from scratch!" We’ve all heard the wisdom of not reinventing the wheel, and yes, dependencies help us move faster. But sometimes, they come back to haunt us. I’m here to tell you—if you have the time and resources, build your system from scratch! It might be the only thing you’ve ever done right. The Horror of Legacy PHP Codebases Ever dealt with an old PHP codebase? If you haven’t, consider yourself lucky. I’ve been hired multiple times to work on some truly cursed projects—ones that make you question the life choices that led you to become a developer. One project, in particular, holds a special place in my heart (for all the wrong reasons). The app relied on about 48 third-party packages, most of which were either: Outdated No longer maintained Missing documentation Completely vanished from the internet Trying to update the app was nearly impossible. Replacing packages wasn’t straightforward because many of them had no direct alternatives, and some repos had been deleted entirely, meaning we couldn't even fork them to maintain our own versions. My rational suggestion? Rebuild it from scratch. The client's response? "That’s too much work. Can’t we just patch it?" They proposed an alternative: replacing each failing dependency with a third-party service. Soon, we had an authentication service, email service, file storage service, admin, and more. In theory, this seemed like a good idea—until the challenge became how to connect everything efficiently. Enter the API Gateway An API Gateway is a central point that manages, routes, and secures API requests to various services. Think of it as a traffic cop standing at the intersection of your microservices. Instead of the frontend calling multiple services directly, everything goes through the API Gateway, which: ✅ Authenticates requests ✅ Logs activity for monitoring and debugging ✅ Applies rate-limiting to prevent abuse ✅ Converts responses into a consistent format ✅ Routes traffic dynamically This turned out to be a game-changer for the project. Instead of spaghetti code with random HTTP calls scattered everywhere, all external and internal services now went through a single controlled entry point. Quick Example of API Gateway in Action class ApiGateway { private $services = [ 'auth' => 'https://auth.example.com', 'storage' => 'https://storage.example.com', ]; public function handleRequest($service, $endpoint, $params) { if (!isset($this->services[$service])) { throw new Exception("Service not found"); } $url = $this->services[$service] . $endpoint; return file_get_contents($url . '?' . http_build_query($params)); } } This simple example demonstrates how an API Gateway can centralize service requests, making an application more manageable. The Double-Edged Sword of Frameworks and Libraries At this point, you might be thinking, "Nixx, are you saying we should never use frameworks or libraries?" No, I’m not! I love frameworks, but you have to use them wisely. Advantages of Using Frameworks and Libraries
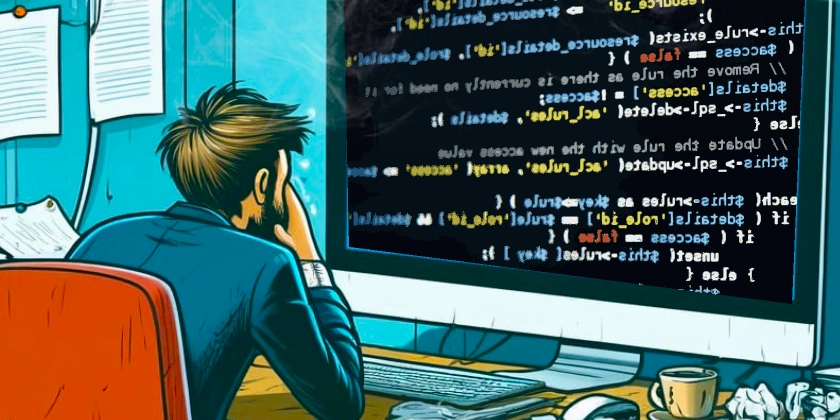
The Pain of Maintaining a PHP Web Application
Maintaining apps, in general, can be a headache, but PHP seems to take the crown when it comes to abandoned projects. You’d be surprised how many PHP apps are still running outdated versions of Laravel, CodeIgniter, or even pure PHP scripts written in a style that should have been buried decades ago.
A client once told me, "If you can buy it, why build it?" Another said, "Use a library and modify it as needed. Don’t build from scratch!" We’ve all heard the wisdom of not reinventing the wheel, and yes, dependencies help us move faster. But sometimes, they come back to haunt us.
I’m here to tell you—if you have the time and resources, build your system from scratch! It might be the only thing you’ve ever done right.
The Horror of Legacy PHP Codebases
Ever dealt with an old PHP codebase? If you haven’t, consider yourself lucky. I’ve been hired multiple times to work on some truly cursed projects—ones that make you question the life choices that led you to become a developer.
One project, in particular, holds a special place in my heart (for all the wrong reasons). The app relied on about 48 third-party packages, most of which were either:
- Outdated
- No longer maintained
- Missing documentation
- Completely vanished from the internet
Trying to update the app was nearly impossible. Replacing packages wasn’t straightforward because many of them had no direct alternatives, and some repos had been deleted entirely, meaning we couldn't even fork them to maintain our own versions.
My rational suggestion? Rebuild it from scratch.
The client's response? "That’s too much work. Can’t we just patch it?"
They proposed an alternative: replacing each failing dependency with a third-party service. Soon, we had an authentication service, email service, file storage service, admin, and more. In theory, this seemed like a good idea—until the challenge became how to connect everything efficiently.
Enter the API Gateway
An API Gateway is a central point that manages, routes, and secures API requests to various services. Think of it as a traffic cop standing at the intersection of your microservices.
Instead of the frontend calling multiple services directly, everything goes through the API Gateway, which:
✅ Authenticates requests
✅ Logs activity for monitoring and debugging
✅ Applies rate-limiting to prevent abuse
✅ Converts responses into a consistent format
✅ Routes traffic dynamically
This turned out to be a game-changer for the project. Instead of spaghetti code with random HTTP calls scattered everywhere, all external and internal services now went through a single controlled entry point.
Quick Example of API Gateway in Action
class ApiGateway
{
private $services = [
'auth' => 'https://auth.example.com',
'storage' => 'https://storage.example.com',
];
public function handleRequest($service, $endpoint, $params) {
if (!isset($this->services[$service])) {
throw new Exception("Service not found");
}
$url = $this->services[$service] . $endpoint;
return file_get_contents($url . '?' . http_build_query($params));
}
}
This simple example demonstrates how an API Gateway can centralize service requests, making an application more manageable.
The Double-Edged Sword of Frameworks and Libraries
At this point, you might be thinking, "Nixx, are you saying we should never use frameworks or libraries?" No, I’m not! I love frameworks, but you have to use them wisely.
Advantages of Using Frameworks and Libraries