Learning to Write Secure Code
In today's digital age, writing secure code is no longer optional—it's a necessity. Whether you're building a simple website or a complex enterprise system, insecure code can open the door to data breaches, service disruptions, and damaged reputations. In this post, we'll explore the essentials of secure coding and how to make it a part of your development workflow. Why Secure Coding Matters Protects sensitive user data (passwords, financial info, personal details) Reduces the risk of cyberattacks (SQL injection, XSS, CSRF) Complies with legal and regulatory requirements (GDPR, HIPAA, PCI-DSS) Builds user trust and enhances your product's reliability Top Secure Coding Practices Validate All Input: Sanitize and validate user inputs to prevent injection attacks. Use Parameterized Queries: Prevent SQL injection by using prepared statements. Limit User Permissions: Follow the principle of least privilege for users and services. Hash Passwords Properly: Use strong hashing algorithms like bcrypt or Argon2 with salt. Escape Output: Prevent XSS attacks by escaping output to HTML, JavaScript, etc. Use Secure Protocols: Always prefer HTTPS over HTTP for secure communication. Handle Errors Gracefully: Avoid revealing stack traces or sensitive info in error messages. Keep Dependencies Updated: Regularly audit and update third-party libraries. Example: Avoiding SQL Injection in Python # Insecure user_input = "admin' --" query = "SELECT * FROM users WHERE username = '" + user_input + "'" Secure cursor.execute("SELECT * FROM users WHERE username = %s", (user_input,)) Security Tools Every Developer Should Know Static Analysis Tools: SonarQube, Checkmarx Dependency Scanners: Snyk, npm audit, pip-audit Fuzzing Tools: AFL, libFuzzer Web Scanners: OWASP ZAP, Burp Suite Common Vulnerabilities to Learn SQL Injection Cross-Site Scripting (XSS) Cross-Site Request Forgery (CSRF) Insecure Deserialization Broken Authentication and Authorization Resources to Learn Secure Coding OWASP Foundation - Top 10 vulnerabilities and resources PortSwigger Web Security Academy - Free hands-on labs OWASP Cheat Sheet Series - Best practices for common scenarios Coursera & edX - Secure coding courses from top universities Conclusion Secure coding is not just about fixing bugs—it's about thinking ahead, preventing threats, and building resilient systems. As a developer, learning and applying secure coding practices will make you a better engineer and protect both users and businesses. Start small, integrate security into your daily workflow, and never stop learning.
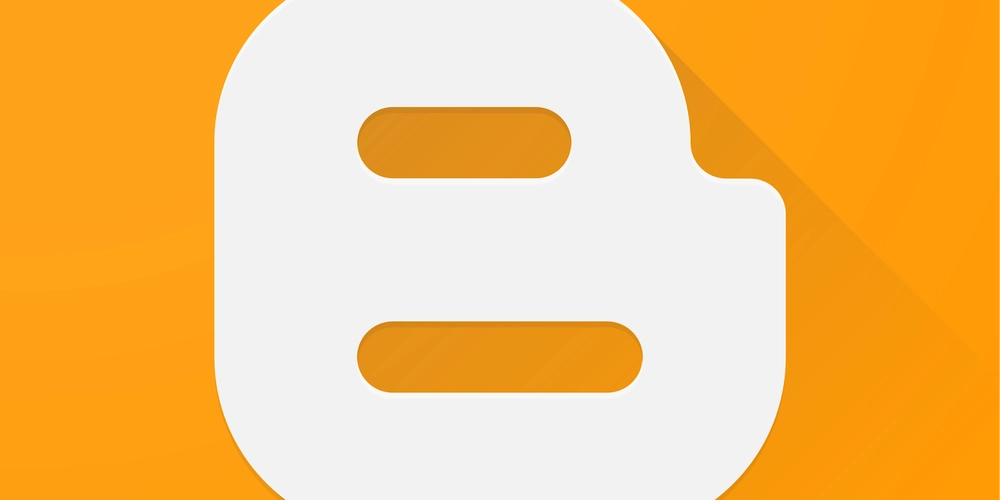
In today's digital age, writing secure code is no longer optional—it's a necessity. Whether you're building a simple website or a complex enterprise system, insecure code can open the door to data breaches, service disruptions, and damaged reputations. In this post, we'll explore the essentials of secure coding and how to make it a part of your development workflow.
Why Secure Coding Matters
- Protects sensitive user data (passwords, financial info, personal details)
- Reduces the risk of cyberattacks (SQL injection, XSS, CSRF)
- Complies with legal and regulatory requirements (GDPR, HIPAA, PCI-DSS)
- Builds user trust and enhances your product's reliability
Top Secure Coding Practices
- Validate All Input: Sanitize and validate user inputs to prevent injection attacks.
- Use Parameterized Queries: Prevent SQL injection by using prepared statements.
- Limit User Permissions: Follow the principle of least privilege for users and services.
- Hash Passwords Properly: Use strong hashing algorithms like bcrypt or Argon2 with salt.
- Escape Output: Prevent XSS attacks by escaping output to HTML, JavaScript, etc.
- Use Secure Protocols: Always prefer HTTPS over HTTP for secure communication.
- Handle Errors Gracefully: Avoid revealing stack traces or sensitive info in error messages.
- Keep Dependencies Updated: Regularly audit and update third-party libraries.
Example: Avoiding SQL Injection in Python
# Insecure
user_input = "admin' --"
query = "SELECT * FROM users WHERE username = '" + user_input + "'"
Secure
cursor.execute("SELECT * FROM users WHERE username = %s", (user_input,))
Security Tools Every Developer Should Know
- Static Analysis Tools: SonarQube, Checkmarx
- Dependency Scanners: Snyk, npm audit, pip-audit
- Fuzzing Tools: AFL, libFuzzer
- Web Scanners: OWASP ZAP, Burp Suite
Common Vulnerabilities to Learn
- SQL Injection
- Cross-Site Scripting (XSS)
- Cross-Site Request Forgery (CSRF)
- Insecure Deserialization
- Broken Authentication and Authorization
Resources to Learn Secure Coding
- OWASP Foundation - Top 10 vulnerabilities and resources
- PortSwigger Web Security Academy - Free hands-on labs
- OWASP Cheat Sheet Series - Best practices for common scenarios
- Coursera & edX - Secure coding courses from top universities
Conclusion
Secure coding is not just about fixing bugs—it's about thinking ahead, preventing threats, and building resilient systems. As a developer, learning and applying secure coding practices will make you a better engineer and protect both users and businesses. Start small, integrate security into your daily workflow, and never stop learning.