After hours of trial and error, this is the most effective way I found to properly set up Stimulus in an existing Rails 7 app using Importmap. If you’ve run into issues like MIME type errors or Stimulus controllers not working, this should solve them. ✅ Step-by-Step Guide 1. Install Importmap (if not already installed) bin/rails importmap:install This command will: Set up config/importmap.rb Create app/javascript and the application.js file Insert the correct tags into your layout 2. Install Turbo and Stimulus bin/rails turbo:install stimulus:install This will: Create the controllers/ directory Add a sample hello_controller.js Register Stimulus with application.js Pin everything properly in importmap.rb ✅ Make Sure Your Layout Includes the Right Tags In app/views/layouts/application.html.erb, check that you have: Do not use: ✅ You're Done! You should now have: No more MIME type errors Working Stimulus controllers Turbo ready if you want to use it ✨ Bonus Here’s what a basic Stimulus controller looks like: // app/javascript/controllers/hello_controller.js import { Controller } from "@hotwired/stimulus" export default class extends Controller { static values = { name: String } greet() { alert(`Hello, ${this.nameValue}!`) } } And how you use it in your view: Greet Hope this helps someone save a few hours of head-scratching!
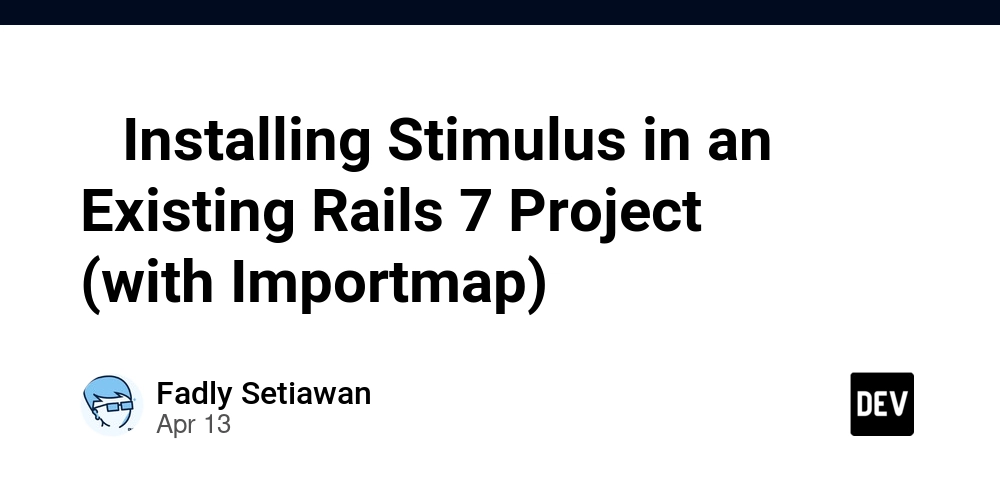
After hours of trial and error, this is the most effective way I found to properly set up Stimulus in an existing Rails 7 app using Importmap. If you’ve run into issues like MIME type errors or Stimulus controllers not working, this should solve them.
✅ Step-by-Step Guide
1. Install Importmap (if not already installed)
bin/rails importmap:install
This command will:
- Set up
config/importmap.rb
- Create
app/javascript
and theapplication.js
file - Insert the correct tags into your layout
2. Install Turbo and Stimulus
bin/rails turbo:install stimulus:install
This will:
- Create the
controllers/
directory - Add a sample
hello_controller.js
- Register Stimulus with
application.js
- Pin everything properly in
importmap.rb
✅ Make Sure Your Layout Includes the Right Tags
In app/views/layouts/application.html.erb
, check that you have:
<%= javascript_importmap_tags %>
<%= stylesheet_link_tag "application", "data-turbo-track": "reload" %>
Do not use:
<%= javascript_pack_tag "application" %>
✅ You're Done!
You should now have:
- No more MIME type errors
- Working Stimulus controllers
- Turbo ready if you want to use it
✨ Bonus
Here’s what a basic Stimulus controller looks like:
// app/javascript/controllers/hello_controller.js
import { Controller } from "@hotwired/stimulus"
export default class extends Controller {
static values = { name: String }
greet() {
alert(`Hello, ${this.nameValue}!`)
}
}
And how you use it in your view:
data-controller="hello" data-hello-name-value="World">
Hope this helps someone save a few hours of head-scratching!