HATEOAS in Spring Boot
When developing RESTful APIs, one important concept that often comes up is HATEOAS (Hypermedia as the Engine of Application State). But what exactly is HATEOAS, and is it mandatory for a REST API? In this blog post, we'll explore what HATEOAS is, its benefits, its drawbacks, and how to implement it in a Spring Boot application. What is HATEOAS? HATEOAS is a constraint of the REST architecture that enables a client to interact with a REST API dynamically through hypermedia links. Instead of relying on hardcoded endpoint URLs, the client can discover available actions based on links provided in API responses. For example, a typical API response without HATEOAS might look like this: { "id": 1, "name": "John Doe", "email": "john.doe@example.com" } With HATEOAS, the response could include links to related resources or actions: { "id": 1, "name": "John Doe", "email": "john.doe@example.com", "_links": { "self": { "href": "/users/1" }, "orders": { "href": "/users/1/orders" } } } Is HATEOAS Mandatory for a REST API? No, HATEOAS is not mandatory for a REST API, but it is a recommended practice for creating truly RESTful services. Many APIs today do not implement HATEOAS, opting instead for a more static approach where the client already knows all the endpoints in advance. However, implementing HATEOAS brings several advantages: Discoverability: Clients can navigate the API without prior knowledge of the structure. Decoupling: The API can evolve without breaking existing clients. Better API UX: Clients receive guidance on available actions dynamically. Drawbacks of HATEOAS While HATEOAS provides significant benefits, it also comes with some drawbacks that developers should consider: Increased Complexity: Implementing HATEOAS requires additional effort to generate and maintain hypermedia links, making the API more complex. Larger Payloads: Responses become larger due to the added links, which may impact performance and increase bandwidth usage. Client-Side Overhead: Clients must parse and process links dynamically, which can make consuming the API more challenging. Limited Adoption: Many API clients and tools do not natively support HATEOAS, making integration more difficult. Performance Trade-offs: Generating links dynamically on the server side can introduce performance overhead, particularly in high-load systems. Implementing HATEOAS in Spring Boot Spring Boot provides excellent support for HATEOAS through the spring-boot-starter-hateoas dependency. Here’s how to implement it in a simple API: 1. Add Dependency In your pom.xml, add the following dependency: org.springframework.boot spring-boot-starter-hateoas 2. Modify Your Controller Let’s say you have a UserController. Modify it to include HATEOAS links: @RestController @RequestMapping("/users") public class UserController { @GetMapping("/{id}") public EntityModel getUser(@PathVariable Long id) { User user = new User(id, "John Doe", "john.doe@example.com"); Link selfLink = linkTo(methodOn(UserController.class).getUser(id)).withSelfRel(); Link ordersLink = linkTo(methodOn(OrderController.class).getOrdersByUser(id)).withRel("orders"); return EntityModel.of(user, selfLink, ordersLink); } } Note: The best practice is to create an assembler or delegate the logic to a service, keeping the controller clean. This improves separation of responsibilities and makes code easier to maintain. 3. Sample Response When calling /users/1, you get a response with hypermedia links: { "id": 1, "name": "John Doe", "email": "john.doe@example.com", "_links": { "self": { "href": "http://localhost:8080/users/1" }, "orders": { "href": "http://localhost:8080/users/1/orders" } } } Conclusion HATEOAS is a powerful concept that makes REST APIs more discoverable and flexible. While it is not mandatory, implementing it in your Spring Boot application can provide significant advantages. However, it is important to weigh its benefits against its potential drawbacks, such as increased complexity and performance considerations. Whether or not to use HATEOAS depends on your project needs and the level of flexibility required for your API consumers.
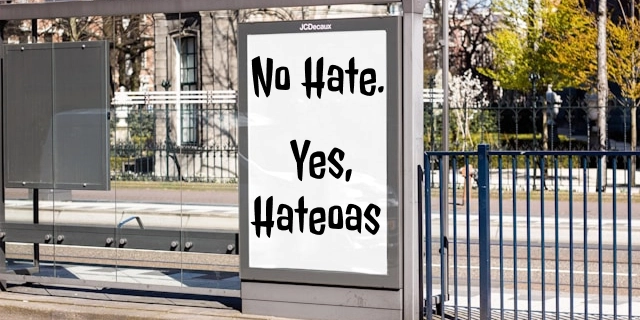
When developing RESTful APIs, one important concept that often comes up is HATEOAS (Hypermedia as the Engine of Application State). But what exactly is HATEOAS, and is it mandatory for a REST API? In this blog post, we'll explore what HATEOAS is, its benefits, its drawbacks, and how to implement it in a Spring Boot application.
What is HATEOAS?
HATEOAS is a constraint of the REST architecture that enables a client to interact with a REST API dynamically through hypermedia links. Instead of relying on hardcoded endpoint URLs, the client can discover available actions based on links provided in API responses.
For example, a typical API response without HATEOAS might look like this:
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
With HATEOAS, the response could include links to related resources or actions:
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com",
"_links": {
"self": { "href": "/users/1" },
"orders": { "href": "/users/1/orders" }
}
}
Is HATEOAS Mandatory for a REST API?
No, HATEOAS is not mandatory for a REST API, but it is a recommended practice for creating truly RESTful services. Many APIs today do not implement HATEOAS, opting instead for a more static approach where the client already knows all the endpoints in advance. However, implementing HATEOAS brings several advantages:
- Discoverability: Clients can navigate the API without prior knowledge of the structure.
- Decoupling: The API can evolve without breaking existing clients.
- Better API UX: Clients receive guidance on available actions dynamically.
Drawbacks of HATEOAS
While HATEOAS provides significant benefits, it also comes with some drawbacks that developers should consider:
- Increased Complexity: Implementing HATEOAS requires additional effort to generate and maintain hypermedia links, making the API more complex.
- Larger Payloads: Responses become larger due to the added links, which may impact performance and increase bandwidth usage.
- Client-Side Overhead: Clients must parse and process links dynamically, which can make consuming the API more challenging.
- Limited Adoption: Many API clients and tools do not natively support HATEOAS, making integration more difficult.
- Performance Trade-offs: Generating links dynamically on the server side can introduce performance overhead, particularly in high-load systems.
Implementing HATEOAS in Spring Boot
Spring Boot provides excellent support for HATEOAS through the spring-boot-starter-hateoas
dependency. Here’s how to implement it in a simple API:
1. Add Dependency
In your pom.xml
, add the following dependency:
org.springframework.boot
spring-boot-starter-hateoas
2. Modify Your Controller
Let’s say you have a UserController
. Modify it to include HATEOAS links:
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public EntityModel<User> getUser(@PathVariable Long id) {
User user = new User(id, "John Doe", "john.doe@example.com");
Link selfLink = linkTo(methodOn(UserController.class).getUser(id)).withSelfRel();
Link ordersLink = linkTo(methodOn(OrderController.class).getOrdersByUser(id)).withRel("orders");
return EntityModel.of(user, selfLink, ordersLink);
}
}
Note: The best practice is to create an assembler or delegate the logic to a service, keeping the controller clean. This improves separation of responsibilities and makes code easier to maintain.
3. Sample Response
When calling /users/1
, you get a response with hypermedia links:
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com",
"_links": {
"self": { "href": "http://localhost:8080/users/1" },
"orders": { "href": "http://localhost:8080/users/1/orders" }
}
}
Conclusion
HATEOAS is a powerful concept that makes REST APIs more discoverable and flexible. While it is not mandatory, implementing it in your Spring Boot application can provide significant advantages. However, it is important to weigh its benefits against its potential drawbacks, such as increased complexity and performance considerations. Whether or not to use HATEOAS depends on your project needs and the level of flexibility required for your API consumers.