DevOps: Dockerizing Your Spring Boot App
Introduction: Ship Faster, Scale Smarter What if you could deploy your app across any environment in minutes, without the dreaded “it works on my machine” excuse? In 2023, 75% of enterprises adopted Docker to streamline deployments, slashing setup time by up to 60%. Dockerizing your Spring Boot app is a cornerstone of modern DevOps, enabling consistent, portable, and scalable applications. Whether you're a beginner deploying your first Java app or a seasoned DevOps engineer optimizing CI/CD pipelines, mastering Docker with Spring Boot is your ticket to faster releases and a standout career. Docker packages apps into containers, ensuring they run identically everywhere, while Spring Boot simplifies Java development with embedded servers. This guide follows a developer’s journey from deployment nightmares to DevOps mastery, covering core concepts, practical steps, and advanced techniques. With Java code, Dockerfiles, a flow chart, case studies, and a dash of humor, this article is your ultimate resource to dockerize like a pro. Let’s containerize and conquer! The Story: From Deployment Disasters to Docker Triumphs Meet Priya, a Java developer at a retail startup. Her team’s Spring Boot app crashed in production due to environment mismatches, costing hours of debugging. Frustrated, Priya discovered Docker, containerizing the app to ensure consistency across dev, test, and prod. Deployments became seamless, and the team scaled to handle Black Friday traffic. This problem-solution arc mirrors Docker’s rise since 2013, transforming DevOps by standardizing deployments. Let’s dive into how you can dockerize your Spring Boot app and avoid Priya’s pain. Section 1: What Is Dockerizing a Spring Boot App? Defining Docker and Spring Boot Docker is a platform that packages applications into containers—lightweight, portable units with code, dependencies, and runtime. Spring Boot is a Java framework for building production-ready apps with minimal configuration, often using an embedded Tomcat server. Dockerizing a Spring Boot app means packaging it into a Docker container, ensuring it runs consistently across environments (dev, staging, production). Analogy: Docker is like a lunchbox—your Spring Boot app (the sandwich) and its dependencies (condiments) are packed together, ready to go anywhere without spilling. Why Dockerize Your Spring Boot App? Consistency: Eliminate environment-specific bugs. Portability: Run on any system with Docker installed. Scalability: Deploy multiple containers to handle load. DevOps Efficiency: Streamline CI/CD pipelines. Career Boost: Docker skills are in high demand. Common Misconception Myth: Docker is too complex for small projects. Truth: Docker simplifies even small apps by ensuring consistent setups. Takeaway: Dockerizing Spring Boot apps ensures consistency and scalability, making it accessible for projects of all sizes. Section 2: Getting Started with Docker and Spring Boot Prerequisites Java 17+: For Spring Boot. Maven: For building the app. Docker: Installed locally (Docker Desktop for Windows/Mac). Spring Boot Project: A basic app (e.g., REST API). Creating a Simple Spring Boot App Let’s build a REST API to dockerize. pom.xml: 4.0.0 com.example demo 0.0.1-SNAPSHOT org.springframework.boot spring-boot-starter-parent 3.2.0 org.springframework.boot spring-boot-starter-web RestController: package com.example.demo; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class HelloController { @GetMapping("/hello") public String sayHello() { return "Hello, Dockerized Spring Boot!"; } } Application: package com.example.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } } Explanation: Setup: A minimal Spring Boot app with a /hello endpoint. Purpose: Returns a greeting, testable locally (http://localhost:8080/hello). Real-World Use: Basis for APIs in e-commerce or microservices. Dockerizing the App Create a Dockerfile to containerize the app. Dockerfile: # Use official OpenJDK 17 base image FROM openjdk:17-jdk-slim # Set working directory WORKDIR /app # Copy Maven-built JAR file COPY target/demo-0.0.1-SNAPSHOT.jar app.jar # Expose port 8080 EXPOSE 8080 # Run the JAR file ENTRYPOINT ["java", "-jar", "app.jar"] Steps to Dockerize: Build the app: mvn clean package Build the Docker image: docker build -t demo-app . Run the container: docker run -p 8080:8080 dem
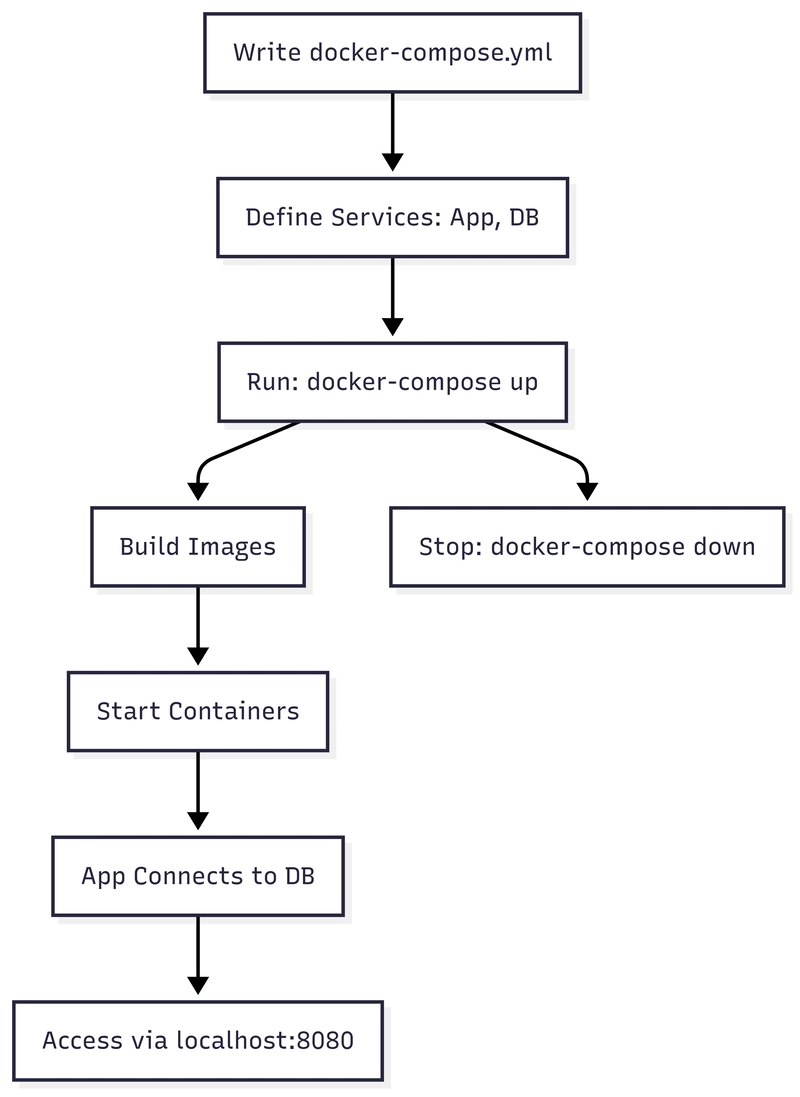
Introduction: Ship Faster, Scale Smarter
What if you could deploy your app across any environment in minutes, without the dreaded “it works on my machine” excuse? In 2023, 75% of enterprises adopted Docker to streamline deployments, slashing setup time by up to 60%. Dockerizing your Spring Boot app is a cornerstone of modern DevOps, enabling consistent, portable, and scalable applications. Whether you're a beginner deploying your first Java app or a seasoned DevOps engineer optimizing CI/CD pipelines, mastering Docker with Spring Boot is your ticket to faster releases and a standout career.
Docker packages apps into containers, ensuring they run identically everywhere, while Spring Boot simplifies Java development with embedded servers. This guide follows a developer’s journey from deployment nightmares to DevOps mastery, covering core concepts, practical steps, and advanced techniques. With Java code, Dockerfiles, a flow chart, case studies, and a dash of humor, this article is your ultimate resource to dockerize like a pro. Let’s containerize and conquer!
The Story: From Deployment Disasters to Docker Triumphs
Meet Priya, a Java developer at a retail startup. Her team’s Spring Boot app crashed in production due to environment mismatches, costing hours of debugging. Frustrated, Priya discovered Docker, containerizing the app to ensure consistency across dev, test, and prod. Deployments became seamless, and the team scaled to handle Black Friday traffic. This problem-solution arc mirrors Docker’s rise since 2013, transforming DevOps by standardizing deployments. Let’s dive into how you can dockerize your Spring Boot app and avoid Priya’s pain.
Section 1: What Is Dockerizing a Spring Boot App?
Defining Docker and Spring Boot
Docker is a platform that packages applications into containers—lightweight, portable units with code, dependencies, and runtime. Spring Boot is a Java framework for building production-ready apps with minimal configuration, often using an embedded Tomcat server.
Dockerizing a Spring Boot app means packaging it into a Docker container, ensuring it runs consistently across environments (dev, staging, production).
Analogy: Docker is like a lunchbox—your Spring Boot app (the sandwich) and its dependencies (condiments) are packed together, ready to go anywhere without spilling.
Why Dockerize Your Spring Boot App?
- Consistency: Eliminate environment-specific bugs.
- Portability: Run on any system with Docker installed.
- Scalability: Deploy multiple containers to handle load.
- DevOps Efficiency: Streamline CI/CD pipelines.
- Career Boost: Docker skills are in high demand.
Common Misconception
Myth: Docker is too complex for small projects.
Truth: Docker simplifies even small apps by ensuring consistent setups.
Takeaway: Dockerizing Spring Boot apps ensures consistency and scalability, making it accessible for projects of all sizes.
Section 2: Getting Started with Docker and Spring Boot
Prerequisites
- Java 17+: For Spring Boot.
- Maven: For building the app.
- Docker: Installed locally (Docker Desktop for Windows/Mac).
- Spring Boot Project: A basic app (e.g., REST API).
Creating a Simple Spring Boot App
Let’s build a REST API to dockerize.
pom.xml:
xmlns="http://maven.apache.org/POM/4.0.0">
4.0.0
com.example
demo
0.0.1-SNAPSHOT
org.springframework.boot
spring-boot-starter-parent
3.2.0
org.springframework.boot
spring-boot-starter-web
RestController:
package com.example.demo;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, Dockerized Spring Boot!";
}
}
Application:
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
Explanation:
-
Setup: A minimal Spring Boot app with a
/hello
endpoint. -
Purpose: Returns a greeting, testable locally (
http://localhost:8080/hello
). - Real-World Use: Basis for APIs in e-commerce or microservices.
Dockerizing the App
Create a Dockerfile to containerize the app.
Dockerfile:
# Use official OpenJDK 17 base image
FROM openjdk:17-jdk-slim
# Set working directory
WORKDIR /app
# Copy Maven-built JAR file
COPY target/demo-0.0.1-SNAPSHOT.jar app.jar
# Expose port 8080
EXPOSE 8080
# Run the JAR file
ENTRYPOINT ["java", "-jar", "app.jar"]
Steps to Dockerize:
- Build the app:
mvn clean package
- Build the Docker image:
docker build -t demo-app .
- Run the container:
docker run -p 8080:8080 demo-app
Explanation:
- Dockerfile: Uses OpenJDK 17, copies the JAR, exposes port 8080, and runs the app.
- Commands: Build the JAR, create an image, and run it as a container.
- Real-World Use: Deploys a Spring Boot API in a consistent environment.
Takeaway: Create a simple Spring Boot app and dockerize it with a basic Dockerfile to ensure portability.
Section 3: Core Docker Concepts for Spring Boot
Images and Containers
-
Image: A blueprint with app code, dependencies, and runtime (e.g.,
demo-app
). - Container: A running instance of an image, isolated and lightweight.
Dockerfile Best Practices
-
Minimize Layers: Combine commands (e.g.,
RUN apt-get update && apt-get install
). -
Use Slim Base Images:
openjdk:17-jdk-slim
reduces size. -
Leverage .dockerignore: Exclude unnecessary files (e.g.,
target/
).
.dockerignore:
target/
*.log
.git/
Multi-Stage Builds
Reduce image size by building and running in separate stages.
Dockerfile (Multi-Stage):
# Build stage
FROM maven:3.8.6-openjdk-17 AS builder
WORKDIR /build
COPY pom.xml .
COPY src ./src
RUN mvn clean package -DskipTests
# Run stage
FROM openjdk:17-jdk-slim
WORKDIR /app
COPY --from=builder /build/target/demo-0.0.1-SNAPSHOT.jar app.jar
EXPOSE 8080
ENTRYPOINT ["java", "-jar", "app.jar"]
Explanation: Builds the JAR in a Maven stage, then copies only the JAR to a slim runtime image, reducing size by 50%.
Humor: A bloated Docker image is like packing a suitcase with bricks—lighten up with multi-stage builds!