Why I recommend Optimizing File and Folder Composition for Server Components First!
With Next.js just announcing version 15, and evolving rapidly, server components have become a core focus. The new data-fetching paradigms and routing enhancements further solidify the framework’s push toward server-side rendering (SSR) as the default approach. To keep up with these changes, I’ve adopted a mindset of “server components first” while managing client-side functionality separately. Here’s why I believe this approach should be embraced by more teams, along with practical guidelines for structuring Next.js projects. The Mindset: Server Components First Server components are emphasized to allow developers to fully leverage SSR while also offering client-side flexibility. By default, the assumption is that all pages will be server-rendered, benefiting: Performance: Offloading rendering to the server reduces the amount of JavaScript that runs on the client, leading to faster load times and a smoother user experience. Data Fetching: Fetch data directly within server components using standard fetch and async/await. This approach takes full advantage of SSR without needing complex configurations, letting the server handle the heavy lifting while keeping the client-side lightweight. SEO: Server-rendered pages deliver fully populated HTML to search engine crawlers, improving indexing and boosting search engine rankings. Handling Client-Side Functionality Separately Client-side interactivity remains crucial for certain features, such as form handling, animations, or dynamic state management. With Next.js, you can manage this by using the 'use client' directive, which declares that a component should be rendered on the client side. Here’s how to structure this: Isolate client functionality in separate components: Create individual files for client-specific components and include the 'use client' directive at the top. Leverage composition patterns to manage complexity: Utilize the composition patterns described in Next.js documentation to maintain clarity between server-rendered and client-rendered components. This ensures that client components are only introduced when necessary. For example, consider a page that includes a server-rendered list but also requires a client-side filter: page.tsx: This is the server component for rendering the list. ProductFilter.tsx: A client component with the 'use client' directive to manage filter interactions. What If There Are No Server-Specific Needs? When server-side rendering isn’t required, you can add the 'use client' directive directly to the page.tsx file. This approach is suitable for scenarios where a page's content doesn’t benefit from being pre-rendered on the server, such as purely interactive dashboards or forms. Structuring Your Next.js Project in Version 14+ To keep the project organized, follow a structure that clearly separates server and client components, utilizing composition patterns where appropriate. Here’s an example: app/ ├─ products/ │ ├─ page.tsx // Server component for rendering the product list & imports ProductList.tsx │ ├─ ProductList.tsx // Client page with 'use client' directive & imports ProductFilter.tsx │ ├─ ProductFilter.tsx // Client component with 'use client' directive ├─ checkout/ │ ├─ page.tsx // 'use client' directive if entirely client-side This folder organization facilitates using different composition patterns like partial hydration (splitting pages into server and client sections) or lifting state management into a client component where necessary. Data Fetching in Next.js The new recommended way of data-fetching strategy involves: Using fetch directly in server components: Data-fetching operations are performed inside server components, making SSR straightforward. Async functions for data retrieval: Server components can use async/await for data-fetching without extra configurations. An example implementation: // app/products/page.tsx import React from 'react'; export default async function ProductsPage() { const res = await fetch('https://api.example.com/products'); const products = await res.json(); return ( Product List {products.map((product) => ( {product.name} ))} ); } Composition Patterns for Enhanced Control According to Next.js’s documentation on composition patterns, different approaches can be taken to balance server-side rendering and client-side interactivity: Partial Hydration: Split a page into server and client components, keeping most content server-rendered for performance while allowing dynamic sections to be interactive. Client Component Islands: Place interactive islands (client components) within server-rendered content. This approach helps reduce the amount of JavaScript needed on the client side while still providing rich interactivity. Serve
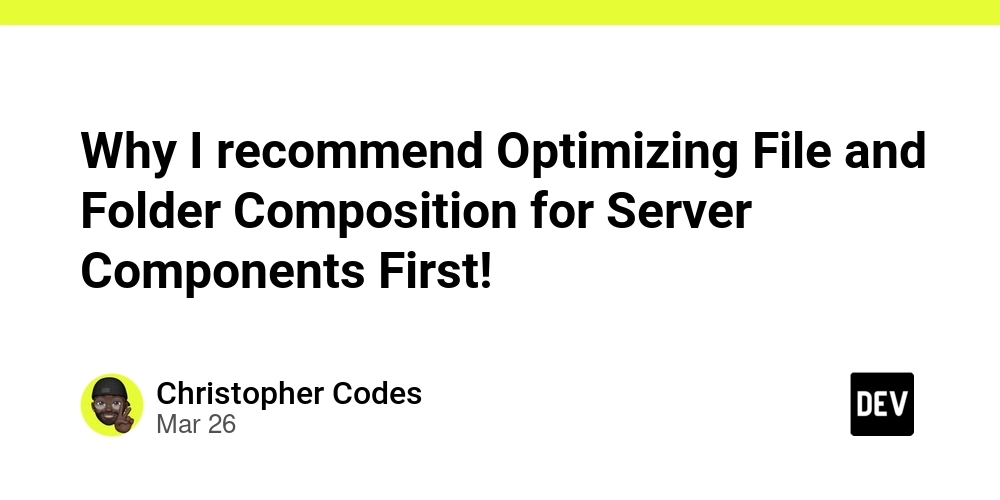
With Next.js just announcing version 15, and evolving rapidly, server components have become a core focus. The new data-fetching paradigms and routing enhancements further solidify the framework’s push toward server-side rendering (SSR) as the default approach. To keep up with these changes, I’ve adopted a mindset of “server components first” while managing client-side functionality separately. Here’s why I believe this approach should be embraced by more teams, along with practical guidelines for structuring Next.js projects.
The Mindset: Server Components First
Server components are emphasized to allow developers to fully leverage SSR while also offering client-side flexibility. By default, the assumption is that all pages will be server-rendered, benefiting:
- Performance: Offloading rendering to the server reduces the amount of JavaScript that runs on the client, leading to faster load times and a smoother user experience.
- Data Fetching: Fetch data directly within server components using standard fetch and async/await. This approach takes full advantage of SSR without needing complex configurations, letting the server handle the heavy lifting while keeping the client-side lightweight.
- SEO: Server-rendered pages deliver fully populated HTML to search engine crawlers, improving indexing and boosting search engine rankings.
Handling Client-Side Functionality Separately
Client-side interactivity remains crucial for certain features, such as form handling, animations, or dynamic state management. With Next.js, you can manage this by using the 'use client' directive, which declares that a component should be rendered on the client side. Here’s how to structure this:
- Isolate client functionality in separate components: Create individual files for client-specific components and include the 'use client' directive at the top.
- Leverage composition patterns to manage complexity: Utilize the composition patterns described in Next.js documentation to maintain clarity between server-rendered and client-rendered components. This ensures that client components are only introduced when necessary.
For example, consider a page that includes a server-rendered list but also requires a client-side filter:
-
page.tsx
: This is the server component for rendering the list. -
ProductFilter.tsx
: A client component with the'use client'
directive to manage filter interactions.
What If There Are No Server-Specific Needs?
When server-side rendering isn’t required, you can add the 'use client' directive directly to the page.tsx file. This approach is suitable for scenarios where a page's content doesn’t benefit from being pre-rendered on the server, such as purely interactive dashboards or forms.
Structuring Your Next.js Project in Version 14+
To keep the project organized, follow a structure that clearly separates server and client components, utilizing composition patterns where appropriate. Here’s an example:
app/
├─ products/
│ ├─ page.tsx // Server component for rendering the product list & imports ProductList.tsx
│ ├─ ProductList.tsx // Client page with 'use client' directive & imports ProductFilter.tsx
│ ├─ ProductFilter.tsx // Client component with 'use client' directive
├─ checkout/
│ ├─ page.tsx // 'use client' directive if entirely client-side
This folder organization facilitates using different composition patterns like partial hydration (splitting pages into server and client sections) or lifting state management into a client component where necessary.
Data Fetching in Next.js
The new recommended way of data-fetching strategy involves:
-
Using
fetch
directly in server components: Data-fetching operations are performed inside server components, making SSR straightforward. -
Async functions for data retrieval: Server components can use
async/await
for data-fetching without extra configurations. An example implementation:
// app/products/page.tsx
import React from 'react';
export default async function ProductsPage() {
const res = await fetch('https://api.example.com/products');
const products = await res.json();
return (
Product List
{products.map((product) => (
- {product.name}
))}
);
}
Composition Patterns for Enhanced Control
According to Next.js’s documentation on composition patterns, different approaches can be taken to balance server-side rendering and client-side interactivity:
- Partial Hydration: Split a page into server and client components, keeping most content server-rendered for performance while allowing dynamic sections to be interactive.
- Client Component Islands: Place interactive islands (client components) within server-rendered content. This approach helps reduce the amount of JavaScript needed on the client side while still providing rich interactivity.
- Server-Rendered Shells: Build the outer structure (shell) of your application with server components and populate it with client components for specific sections that need user interactivity.
These patterns ensure that client-side JavaScript usage is minimized, leading to better performance and user experiences.
Why Adopt This Mentality?
- Improved Performance: By defaulting to server components and the new data-fetching approach, your application will benefit from optimized SSR, resulting in faster page loads.
- Enhanced SEO: Server-rendered pages provide search engines with fully populated HTML, making it easier to index content.
- Reduced Client Bundle Size: Isolating client logic with 'use client' minimizes JavaScript sent to the browser.
- Simplified Development: The new data-fetching approach integrates directly into server components, eliminating the need for helper functions.
- Scalability and Maintainability: Using composition patterns makes it easy to scale features and manage rendering logic.
Conclusion
The shift toward “server components first” in Next.js aligns perfectly with the composition patterns described in the Next.js documentation. This approach simplifies SSR while maximizing performance and SEO benefits. By adopting this mentality and following the composition strategies, teams can quickly build scalable, fast, and optimized Awesome applications.
Happy coding