What Are the Best Practices for Writing Dockerfiles?
When working with Docker, the Dockerfile is a critical component that defines how your container images are built. A well-crafted Dockerfile can significantly enhance the performance and security of your applications. This article explores the best practices for writing efficient and secure Dockerfiles. 1. Use Official Base Images Start your Dockerfile with an official base image, which is maintained and updated by the community. This ensures you are using well-tested and secure images. You can find official images on Docker Hub. FROM python:3.9-slim 2. Keep the Dockerfile Simple and Concise A Dockerfile should be easy to read and maintain. Aim for clarity and simplicity, and minimize the number of layers by combining commands where possible. RUN apt-get update && apt-get install -y \ libpq-dev \ && apt-get clean \ && rm -rf /var/lib/apt/lists/* 3. Use .dockerignore Effectively Create a .dockerignore file to exclude unnecessary files and directories from being included in the image, helping to keep your image lean. node_modules .git 4. Leverage Caching Docker caches layers of an image to speed up the building process. Organize your Dockerfile to take full advantage of this caching feature. Frequently changing parts of your application should be placed near the end of the Dockerfile. 5. Use Multistage Builds With multistage builds, you can use a single Dockerfile to build and package your application in separate stages, reducing final image size by discarding build dependencies. FROM golang:alpine AS builder WORKDIR /app COPY . . RUN go build -o myapp FROM alpine WORKDIR /app COPY --from=builder /app/myapp . CMD ["./myapp"] 6. Minimize Layer Sizes Remove unnecessary files and clear caches to minimize layer sizes. RUN apt-get update && apt-get install -y --no-install-recommends \ package1 \ package2 \ && apt-get clean && rm -rf /var/lib/apt/lists/* 7. Avoid Running as Root For security reasons, avoid running your application as the root user. Create a non-root user and switch to it using the USER instruction. RUN useradd -m myuser USER myuser 8. Document with Comments Include comments within your Dockerfile to explain the purpose of commands, making it easier for others (and yourself) to understand your setup. RUN apt-get update && apt-get install -y curl 9. Keep Security in Mind Regularly update the base images and dependencies to patch security vulnerabilities. Additionally, use tools to scan your images for vulnerabilities. Resources for Further Learning For a deeper dive into mastering Docker, check out our recommended Docker book discounts. Additional Resources Explore strategies for migrating legacy applications to Docker in 2025. Learn how to create a Dockerfile from scratch in 2025. By applying these best practices, you can create efficient, secure, and maintainable Dockerfiles that optimize your workflow. Happy Dockerizing!
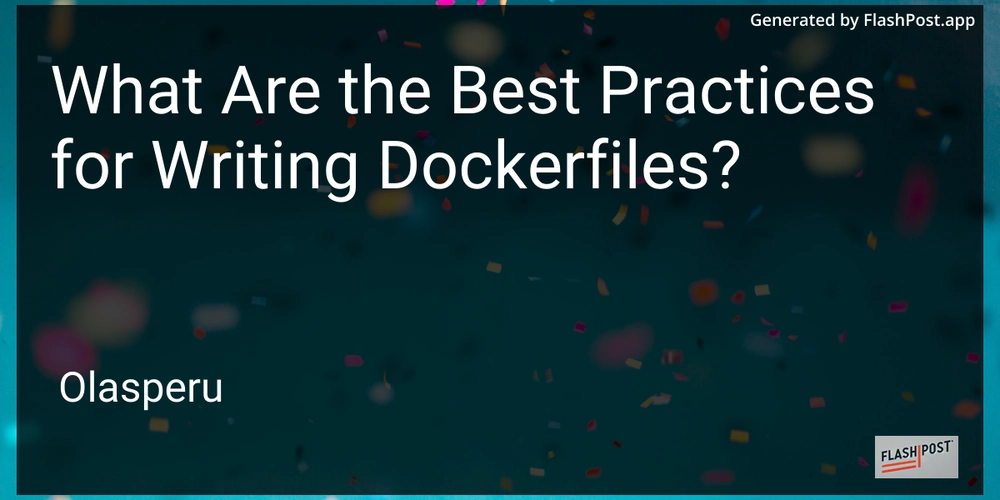
When working with Docker, the Dockerfile is a critical component that defines how your container images are built. A well-crafted Dockerfile can significantly enhance the performance and security of your applications. This article explores the best practices for writing efficient and secure Dockerfiles.
1. Use Official Base Images
Start your Dockerfile with an official base image, which is maintained and updated by the community. This ensures you are using well-tested and secure images. You can find official images on Docker Hub.
FROM python:3.9-slim
2. Keep the Dockerfile Simple and Concise
A Dockerfile should be easy to read and maintain. Aim for clarity and simplicity, and minimize the number of layers by combining commands where possible.
RUN apt-get update && apt-get install -y \
libpq-dev \
&& apt-get clean \
&& rm -rf /var/lib/apt/lists/*
3. Use .dockerignore Effectively
Create a .dockerignore
file to exclude unnecessary files and directories from being included in the image, helping to keep your image lean.
node_modules
.git
4. Leverage Caching
Docker caches layers of an image to speed up the building process. Organize your Dockerfile to take full advantage of this caching feature. Frequently changing parts of your application should be placed near the end of the Dockerfile.
5. Use Multistage Builds
With multistage builds, you can use a single Dockerfile to build and package your application in separate stages, reducing final image size by discarding build dependencies.
FROM golang:alpine AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp
FROM alpine
WORKDIR /app
COPY --from=builder /app/myapp .
CMD ["./myapp"]
6. Minimize Layer Sizes
Remove unnecessary files and clear caches to minimize layer sizes.
RUN apt-get update && apt-get install -y --no-install-recommends \
package1 \
package2 \
&& apt-get clean && rm -rf /var/lib/apt/lists/*
7. Avoid Running as Root
For security reasons, avoid running your application as the root user. Create a non-root user and switch to it using the USER
instruction.
RUN useradd -m myuser
USER myuser
8. Document with Comments
Include comments within your Dockerfile to explain the purpose of commands, making it easier for others (and yourself) to understand your setup.
RUN apt-get update && apt-get install -y curl
9. Keep Security in Mind
Regularly update the base images and dependencies to patch security vulnerabilities. Additionally, use tools to scan your images for vulnerabilities.
Resources for Further Learning
For a deeper dive into mastering Docker, check out our recommended Docker book discounts.
Additional Resources
- Explore strategies for migrating legacy applications to Docker in 2025.
- Learn how to create a Dockerfile from scratch in 2025.
By applying these best practices, you can create efficient, secure, and maintainable Dockerfiles that optimize your workflow. Happy Dockerizing!