Spring Core Fundamentals: A Beginner Guide
Spring is one of the most popular frameworks in the Java ecosystem, known for its powerful dependency injection capabilities and extensive ecosystem. Whether you're just starting with Spring or looking to refresh your knowledge, this guide will walk you through the core concepts that make Spring such a powerful framework. Understanding Spring vs Spring Boot Before diving into the core concepts, let's clarify the difference between Spring and Spring Boot: Feature Spring Framework Spring Boot Definition A comprehensive framework providing infrastructure support for Java applications An extension of Spring that simplifies development with pre-configured defaults Configuration Requires extensive manual configuration Provides auto-configuration, reducing manual setup Standalone Apps Requires external servers Comes with embedded servers for standalone applications Boilerplate Code Requires more configuration code Reduces boilerplate with opinionated defaults Dependency Management Manual dependency management Uses Spring Boot Starter dependencies At its core, Spring framework is primarily a dependency injection container with additional convenience layers for database access, proxies, aspect-oriented programming, and web MVC. Dependency Injection: The Heart of Spring Dependency Injection (DI) is a design pattern where a class receives its dependencies from an external source rather than creating them itself. This promotes: Loose coupling between components Improved testability Better code maintainability Spring's core functionality is implementing this pattern effectively through its IoC container. Inversion of Control: Shifting Responsibility Inversion of Control (IoC) is a design principle where the control of object creation and lifecycle management is transferred from your application code to the Spring framework. Traditional Approach vs IoC Without IoC: class Car { private Engine engine; public Car() { this.engine = new Engine(); // Object creation inside the class (tight coupling) } } With IoC (Spring's approach): @Component class Car { private final Engine engine; @Autowired public Car(Engine engine) { this.engine = engine; // Spring injects the dependency } } The key difference? With Spring's IoC, your application code doesn't control dependency creation - Spring does. Types of Dependency Injection in Spring Spring supports three primary types of dependency injection: 1. Constructor-Based Injection @Component class Car { private final Engine engine; // immutable @Autowired public Car(Engine engine) { this.engine = engine; } } Advantages: Dependencies are explicit Encourages immutability Objects are always created with required dependencies This is generally the recommended approach in modern Spring applications. 2. Setter-Based Injection @Component class Car { private Engine engine; @Autowired public void setEngine(Engine engine) { this.engine = engine; // optional dependency } } Advantages: Allows optional dependencies Provides flexibility in modifying dependencies at runtime Disadvantages: Objects might be in an incomplete state if dependencies aren't provided More verbose than constructor injection 3. Field-Based Injection @Component class Car { @Autowired private Engine engine; } Advantages: Less boilerplate code Simple to implement Disadvantages: Harder to test Cannot enforce immutability Dependencies aren't explicitly declared Spring IoC Container: Managing Your Beans Spring provides two types of IoC containers to manage the lifecycle of beans: BeanFactory: The Lightweight Container Provides basic bean management capabilities Uses lazy initialization - beans are created only when requested Uses less memory and is suitable for resource-constrained environments ApplicationContext: The Feature-Rich Container Extends BeanFactory with enterprise features Uses eager initialization - beans are created at startup Provides advanced features like: Annotation-based dependency injection Event handling Internationalization support AOP integration Spring Boot automatically uses ApplicationContext for its enhanced feature set. Understanding Spring Beans A bean in Spring is simply an object created and managed by the Spring IoC container. Spring creates instances of classes annotated with @Component (or its specialized annotations), manages their lifecycle, and injects them where needed. Bean Scopes: Controlling Instance Creation Spring offers several bean scopes that determine how instances are created and shared: Scope Description singleton (default) A single shared instance of the bean is created for the entire application prototype A new instance is
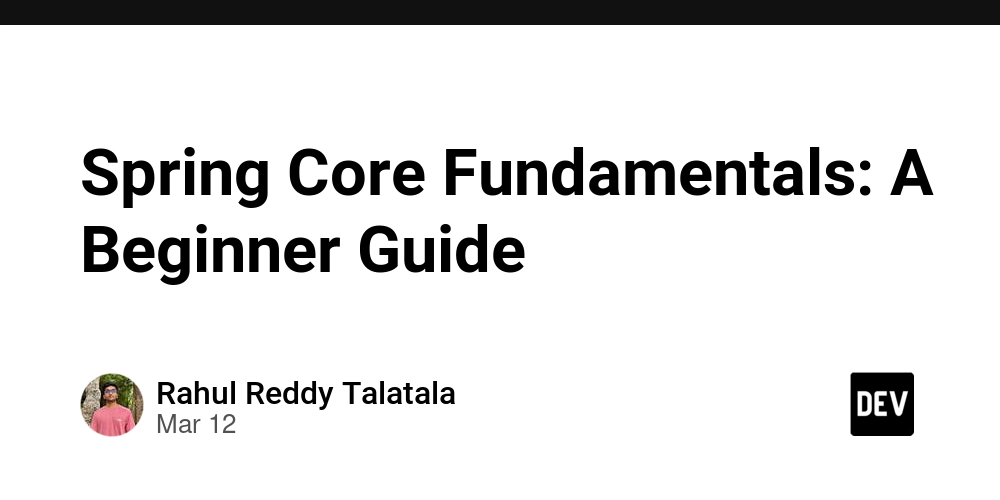
Spring is one of the most popular frameworks in the Java ecosystem, known for its powerful dependency injection capabilities and extensive ecosystem. Whether you're just starting with Spring or looking to refresh your knowledge, this guide will walk you through the core concepts that make Spring such a powerful framework.
Understanding Spring vs Spring Boot
Before diving into the core concepts, let's clarify the difference between Spring and Spring Boot:
Feature | Spring Framework | Spring Boot |
---|---|---|
Definition | A comprehensive framework providing infrastructure support for Java applications | An extension of Spring that simplifies development with pre-configured defaults |
Configuration | Requires extensive manual configuration | Provides auto-configuration, reducing manual setup |
Standalone Apps | Requires external servers | Comes with embedded servers for standalone applications |
Boilerplate Code | Requires more configuration code | Reduces boilerplate with opinionated defaults |
Dependency Management | Manual dependency management | Uses Spring Boot Starter dependencies |
At its core, Spring framework is primarily a dependency injection container with additional convenience layers for database access, proxies, aspect-oriented programming, and web MVC.
Dependency Injection: The Heart of Spring
Dependency Injection (DI) is a design pattern where a class receives its dependencies from an external source rather than creating them itself. This promotes:
- Loose coupling between components
- Improved testability
- Better code maintainability
Spring's core functionality is implementing this pattern effectively through its IoC container.
Inversion of Control: Shifting Responsibility
Inversion of Control (IoC) is a design principle where the control of object creation and lifecycle management is transferred from your application code to the Spring framework.
Traditional Approach vs IoC
Without IoC:
class Car {
private Engine engine;
public Car() {
this.engine = new Engine(); // Object creation inside the class (tight coupling)
}
}
With IoC (Spring's approach):
@Component
class Car {
private final Engine engine;
@Autowired
public Car(Engine engine) {
this.engine = engine; // Spring injects the dependency
}
}
The key difference? With Spring's IoC, your application code doesn't control dependency creation - Spring does.
Types of Dependency Injection in Spring
Spring supports three primary types of dependency injection:
1. Constructor-Based Injection
@Component
class Car {
private final Engine engine; // immutable
@Autowired
public Car(Engine engine) {
this.engine = engine;
}
}
Advantages:
- Dependencies are explicit
- Encourages immutability
- Objects are always created with required dependencies
This is generally the recommended approach in modern Spring applications.
2. Setter-Based Injection
@Component
class Car {
private Engine engine;
@Autowired
public void setEngine(Engine engine) {
this.engine = engine; // optional dependency
}
}
Advantages:
- Allows optional dependencies
- Provides flexibility in modifying dependencies at runtime
Disadvantages:
- Objects might be in an incomplete state if dependencies aren't provided
- More verbose than constructor injection
3. Field-Based Injection
@Component
class Car {
@Autowired
private Engine engine;
}
Advantages:
- Less boilerplate code
- Simple to implement
Disadvantages:
- Harder to test
- Cannot enforce immutability
- Dependencies aren't explicitly declared
Spring IoC Container: Managing Your Beans
Spring provides two types of IoC containers to manage the lifecycle of beans:
BeanFactory: The Lightweight Container
- Provides basic bean management capabilities
- Uses lazy initialization - beans are created only when requested
- Uses less memory and is suitable for resource-constrained environments
ApplicationContext: The Feature-Rich Container
- Extends BeanFactory with enterprise features
- Uses eager initialization - beans are created at startup
- Provides advanced features like:
- Annotation-based dependency injection
- Event handling
- Internationalization support
- AOP integration
Spring Boot automatically uses ApplicationContext for its enhanced feature set.
Understanding Spring Beans
A bean in Spring is simply an object created and managed by the Spring IoC container. Spring creates instances of classes annotated with @Component
(or its specialized annotations), manages their lifecycle, and injects them where needed.
Bean Scopes: Controlling Instance Creation
Spring offers several bean scopes that determine how instances are created and shared:
Scope | Description |
---|---|
singleton (default) | A single shared instance of the bean is created for the entire application |
prototype | A new instance is created each time the bean is requested |
request | A new instance is created for each HTTP request (web applications only) |
session | A new instance is created for each user session (web applications only) |
application | A single instance for the entire ServletContext (web applications only) |
You can specify the scope using the @Scope
annotation:
@Component
@Scope("prototype")
class PrototypeBean {
// Each request for this bean will create a new instance
}
Essential Spring Annotations
Spring provides a rich set of annotations to simplify configuration:
Component Annotations
- @Component: Generic annotation for any Spring-managed component
- @Service: For service layer classes containing business logic
- @Repository: For data access objects handling database operations
- @Controller: For Spring MVC controllers handling web requests
- @RestController: For RESTful controllers (combines @Controller and @ResponseBody)
Configuration Annotations
- @Configuration: Marks a class as a source of bean definitions
- @bean: Explicitly declares a bean method within a @Configuration class
- @ComponentScan: Tells Spring where to look for annotated components
Dependency Injection Annotations
- @Autowired: Marks a constructor, field, or setter method for automatic dependency injection
@Configuration
@ComponentScan("com.example")
class AppConfig {
@Bean
public Engine customEngine() {
return new TurboEngine();
}
}
Aspect-Oriented Programming with Spring
Spring AOP allows you to separate cross-cutting concerns (like logging, security, transactions) from your business logic. It does this by using proxies to intercept method calls and apply additional behavior.
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.Service.*(..))")
public void logBefore() {
System.out.println("Method execution started...");
}
}
Key AOP concepts include:
- Aspect: Module containing cross-cutting logic
- Join Point: Execution point where aspect can be applied
- Advice: Action performed at a join point (before, after, around)
- Pointcut: Expression that determines where advice should be applied
- Weaving: Process of applying aspects to target objects
A real-world example is Spring's @Transactional
annotation, which uses AOP to handle transaction management without cluttering your business logic.
Conclusion
Spring Core provides a robust foundation for building Java applications by implementing dependency injection and inversion of control. By managing dependencies externally, Spring helps create more modular, testable, and maintainable applications.
Understanding these fundamental concepts - IoC, DI, beans, annotations, and AOP - will give you a solid foundation for working with the Spring ecosystem, whether you're using Spring Framework directly or Spring Boot.
The true power of Spring comes from how these concepts work together, allowing you to focus on your business logic while Spring handles the infrastructure concerns.
Curious about building a rock-solid e-commerce system with Spring Boot microservices? Dive into my latest blog where I break it all down—resilience, scalability, and the magic of Spring! Check it out here.
Happy Springing!