Playing with The DOM
A couple months ago I decided to jump into the tech world by enrolling in a bootcamp. It's been an interesting journey, from starting with the classic "Hello, World!" to building a small project. I'm currently learning JavaScript, and even though it has been a roller coaster of emotions I'm enjoying the process. As my assessment for my final phase approaches, I'm building an app to showcase everything I've learned. One topic I've really enjoyed working with is manipulating the DOM. What's the DOM? Document Object Model (DOM) is the way your browser turns HTML into something JavaScript can understand and manipulate. when a website loads, takes the HTML and turns it into the DOM making it possible to find elements, modify text and add or removes elements. In my short time working with the DOM I've had a lot of fun specially using methods to find elements like getElementById(), getElementsByClassName(), getElementsByTagName(), querySelector() and querySelectorAll(). getElementById() Let's start with one of my favorites methods. This one allows you to quickly access an element by introducing its id and just returns one element at the time due that ids are expected to be unique. Here is an example of its implementation in my current project: const search = document.getElementById('search-input'); getElementsByClassName() This method returns all elements with a given class name in the form of an HTMLCollection (which is similar to an array). You can loop through them and manipulate each one. Hello! Sup? Tinier heading document.getElementsByClassName('banner'); getElementsByTagName() If you don't know the ID or class of an element but know the tag, this method it's perfect because by using the tag it gives you an HTMLCollection of it. This is paragraph 1. This is paragraph 2. This is paragraph 3. const paragraphs = document.getElementsByTagName("p"); for (let i = 0; i { item.textContent = `Updated Item ${index + 1}`; }); .textContent vs .innerHTML I've also been using properties like .textContent and .innerHTML, and I've learned when to use each one. With .textContent, you can set plain text while ignoring any HTML tags. With .innerHTML, you can set or return the HTML content inside an element, allowing you to replace or add HTML tags and structure. However, when using .innerHTML, you're essentially rebuilding the element, which can sometimes remove IDs, classes, and other attributes. If you're working with a database or accepting user input, this can lead to cross-site scripting (XSS)—a type of security vulnerability where malicious HTML can be injected. In that regard, .textContent is the safer option. const renderMovies = (movieArray) =>{ const movieList = document.getElementById('movie-list'); movieList.innerHTML = ""; movieArray.forEach(movie =>{ movieList.innerHTML +=` ${movie.title} (${movie.year}) Genre: ${movie.genre} Add to Watchlist ` }) h1.textContent = "Hello, world!"; Creating and Appending Elements with createElement() Another cool method I've started using is document.createElement(). This lets me build elements completely from scratch in JavaScript. Instead of using innerHTML, I can create an element, set its content or attributes, and then add it to the page using .appendChild() or .append(). Here's a simple example: const selectedMovie = allMovies.find(movie => movie.id == movieId) const block = document.createElement('div'); block.id = `watchlist-item-${movieId}` block.innerHTML = ` ${selectedMovie.title} Remove ` console.log(selectedMovie.title); document.getElementById('watchlist').appendChild(block) }; }); Using createElement() is cleaner and often reduces the risk of accidentally removing other elements or opening up security issues like cross-site scripting. JavaScript Events When working with the DOM, one of the coolest things is making elements respond to user actions like getting a button to do something when it's clicked. With JavaScript, we have the ability to listen for events that happen in the browser and run code in response. This makes our pages more interactive and dynamic. These events can include things like clicking a button, submitting a form, moving the mouse, or even pressing a key. Common Event Listeners -Click Used when you want something to happen when the user clicks a button or element. document.getElementById('watchlist').addEventListener('click', (e) => { if(e.target.classList.contains('remove-watchlist-btn')) { e.target.closest('div').remove(); } }) -S
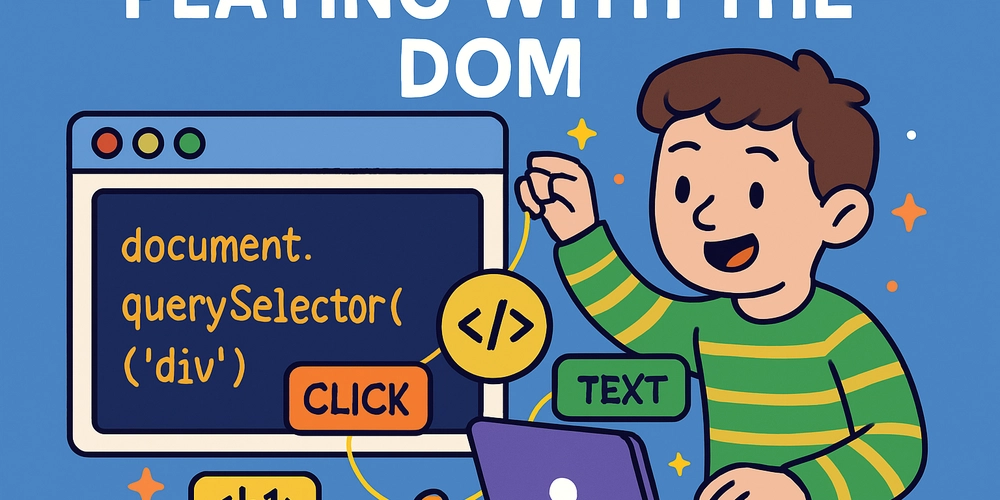
A couple months ago I decided to jump into the tech world by enrolling in a bootcamp. It's been an interesting journey, from starting with the classic "Hello, World!" to building a small project. I'm currently learning JavaScript, and even though it has been a roller coaster of emotions I'm enjoying the process.
As my assessment for my final phase approaches, I'm building an app to showcase everything I've learned. One topic I've really enjoyed working with is manipulating the DOM.
What's the DOM?
Document Object Model (DOM) is the way your browser turns HTML into something JavaScript can understand and manipulate.
when a website loads, takes the HTML and turns it into the DOM making it possible to find elements, modify text and add or removes elements. In my short time working with the DOM I've had a lot of fun specially using methods to find elements like getElementById(), getElementsByClassName(), getElementsByTagName(), querySelector() and querySelectorAll().
getElementById()
Let's start with one of my favorites methods. This one allows you to quickly access an element by introducing its id and just returns one element at the time due that ids are expected to be unique. Here is an example of its implementation in my current project:
const search = document.getElementById('search-input');
getElementsByClassName()
This method returns all elements with a given class name in the form of an HTMLCollection (which is similar to an array). You can loop through them and manipulate each one.
class="banner">
Hello!
class="banner">
Sup?
class="banner">
Tinier heading
document.getElementsByClassName('banner');
getElementsByTagName()
If you don't know the ID or class of an element but know the tag, this method it's perfect because by using the tag it gives you an HTMLCollection of it.
This is paragraph 1.
This is paragraph 2.
This is paragraph 3.
const paragraphs = document.getElementsByTagName("p");
for (let i = 0; i < paragraphs.length; i++) {
paragraphs[i].textContent = `Updated paragraph ${i + 1}`;
}
querySelector()
Another of my favorite is this one! This one returns the first CSS selectors or group of CSS selector that matches. When you use querySelector() you need to specified what Kind of element you are looking for, for example if you're looking:
- IDs use #, like document.querySelector('#list').
- Classes use (.), like document.querySelector('.button').
- Tags use just the name, like document.querySelector('h1')
const ramenDetail = document.querySelector('#ramen-detail')
ramenDetail.querySelector('.detail-image').src = ramen.image;
ramenDetail.querySelector('h2').textContent = ramen.name;
ramenDetail.querySelector('h3').textContent = ramen.restaurant;
document.getElementById('rating-display').textContent = ramen.rating;
document.getElementById('comment-display').textContent = ramen.comment
console.log('currentRamen:',currentRamen)
};
However with querySelector() you could even use it for more specific nested selections:
class="ranked-list">
1
2
3
class="unranked-list">
6
2
4
const li2 = document.querySelector("ul.ranked-list li ul li");
li2;
//=> 2
querySelectorAll()
Last but not least querySelectorAll() works pretty much like querySelector() but instead of returning the first element matching, it'll give you a nodeList of all elements matching:
class="item">Item 1
class="item">Item 2
class="item">Item 3
const listItems = document.querySelectorAll(".item");
listItems.forEach((item, index) => {
item.textContent = `Updated Item ${index + 1}`;
});
.textContent vs .innerHTML
I've also been using properties like .textContent and .innerHTML, and I've learned when to use each one. With .textContent, you can set plain text while ignoring any HTML tags. With .innerHTML, you can set or return the HTML content inside an element, allowing you to replace or add HTML tags and structure. However, when using .innerHTML, you're essentially rebuilding the element, which can sometimes remove IDs, classes, and other attributes. If you're working with a database or accepting user input, this can lead to cross-site scripting (XSS)—a type of security vulnerability where malicious HTML can be injected. In that regard, .textContent is the safer option.
const renderMovies = (movieArray) =>{
const movieList = document.getElementById('movie-list');
movieList.innerHTML = "";
movieArray.forEach(movie =>{
movieList.innerHTML +=`
${movie.title} (${movie.year})
Genre: ${movie.genre}
${movie.poster}" width="100">
movie.id}">Add to Watchlist
`
})
h1.textContent = "Hello, world!";
Creating and Appending Elements with createElement()
Another cool method I've started using is document.createElement(). This lets me build elements completely from scratch in JavaScript. Instead of using innerHTML, I can create an element, set its content or attributes, and then add it to the page using .appendChild() or .append().
Here's a simple example:
const selectedMovie = allMovies.find(movie => movie.id == movieId)
const block = document.createElement('div');
block.id = `watchlist-item-${movieId}`
block.innerHTML = `
${selectedMovie.title}
${selectedMovie.poster}" width="100">
`
console.log(selectedMovie.title);
document.getElementById('watchlist').appendChild(block)
};
});
Using createElement() is cleaner and often reduces the risk of accidentally removing other elements or opening up security issues like cross-site scripting.
JavaScript Events
When working with the DOM, one of the coolest things is making elements respond to user actions like getting a button to do something when it's clicked. With JavaScript, we have the ability to listen for events that happen in the browser and run code in response. This makes our pages more interactive and dynamic. These events can include things like clicking a button, submitting a form, moving the mouse, or even pressing a key.
Common Event Listeners
-Click
Used when you want something to happen when the user clicks a button or element.
document.getElementById('watchlist').addEventListener('click', (e) => {
if(e.target.classList.contains('remove-watchlist-btn')) {
e.target.closest('div').remove();
}
})
-Submit
Used for forms. You can prevent the page from refreshing using event.preventDefault().
const addSubmitListener = (e) => {
const form = document.getElementById('new-ramen');
form.addEventListener('submit', (e) => {
e.preventDefault();
console.log('new ramen:', newRamen);
});
}
-Input
Fires when a user types into an input field—great for live search or form validation.
const search = document.getElementById('search-input')
search.addEventListener('input', (e) =>{
const searchTerm = e.target.value.toLowerCase();
const filteredMovies = allMovies.filter(movie =>
movie.title.toLowerCase().includes(searchTerm));
renderMovies(filteredMovies);
});
Final Thoughts
As I continue learning, I’m looking forward to using the DOM in more advanced projects. Working with it again and again has made me feel more confident than when I first started this journey. I still face challenges, but looking back at what I’ve built already reminds me how far I’ve come—and that helps me keep moving forward. I can’t wait to keep learning and deepening my understanding.