Master Video Editing in Rust with FFmpeg in Just 3 Minutes
Master Video Editing in Rust with FFmpeg in Just 3 Minutes Introduction In today's era dominated by short-form video content, efficient video editing has become crucial for content creators and developers. Whether you're trimming video intros and endings, extracting highlights, making GIFs, or removing unwanted ads, video editing is a necessity. However, editing one video is easy, but handling large batches of videos can be challenging: Manual editing: Time-consuming, inefficient, and impractical for large quantities. Professional tools (Premiere, After Effects): Powerful but lacking in automation for batch processing. FFmpeg command-line tool: Powerful yet complex; easy to make mistakes with intricate parameters. Rust developers specifically face additional complexities when integrating FFmpeg directly: Complexity: FFmpeg's native C API is powerful but complicated, requiring extensive understanding of low-level details. Memory safety: Directly interacting with the C API can lead to memory leaks, unsafe operations, and reduced stability. Productivity loss: High learning curve, complex API structures, and tedious configurations hinder development efficiency. The Solution: Integrating FFmpeg Elegantly in Rust To address these challenges, Rust developers commonly use wrappers around FFmpeg's native C API. Libraries like ez-ffmpeg offer ergonomic and safe interfaces, simplifying FFmpeg integration through user-friendly, memory-safe Rust abstractions. Such libraries typically abstract complex details, enabling developers to achieve video editing automation easily and securely. Quick Example: Clipping Videos with Rust Suppose you have a video test.mp4 and want to clip a 3-second segment starting at the 5-second mark, outputting to output.mp4. Here's how you can accomplish this: 1. Install FFmpeg macOS: brew install ffmpeg Windows: vcpkg install ffmpeg # Set VCPKG_ROOT if installing for the first time 2. Add Rust Dependency Include ez-ffmpeg in your project's Cargo.toml: [dependencies] ez-ffmpeg = "*" 3. Write the Rust Code use ez_ffmpeg::{FfmpegContext, Input, Output}; fn main() -> Result { FfmpegContext::builder() .input(Input::from("test.mp4") .set_start_time_us(5_000_000) // Start at 5 seconds .set_recording_time_us(3_000_000)) // Record for 3 seconds .output("output.mp4") .build()?.start()?.wait()?; Ok(()) } Understanding the Code Here's what's happening: Input video: .input(Input::from("test.mp4") Specifies the input video file. Setting Clip Duration .set_start_time_us(5_000_000) // Start at 5 seconds .set_recording_time_us(3_000_000) // Duration 3 seconds Output File .output("output.mp4") Defines the resulting clipped video. Executing the Process .build()?.start()?.wait()?; Builds the FFmpeg task Starts the clipping process Waits until completion Additional Clipping Scenarios Clip only the first 10 seconds .input(Input::from("test.mp4").set_recording_time_us(10_000_000)) Clip from 10 seconds to the end .input(Input::from("test.mp4").set_start_time_us(10_000_000)) Clip and convert to GIF FfmpegContext::builder() .input(Input::from("test.mp4") .set_start_time_us(5_000_000) .set_recording_time_us(3_000_000)) .output("output.gif") .build()?.start()?.wait()?; This creates a 3-second GIF animation. Summary By leveraging Rust libraries that encapsulate FFmpeg functionality, developers can: Simplify usage: Use intuitive chain-call APIs to accomplish tasks quickly. Ensure safety: Benefit from Rust's inherent memory safety to avoid common pitfalls associated with direct C API usage. Boost productivity: Focus on core logic rather than complex FFmpeg configurations, significantly increasing efficiency. If you're working on Rust applications related to short-form videos, live-streaming, or content creation, using such libraries can greatly streamline your workflow.
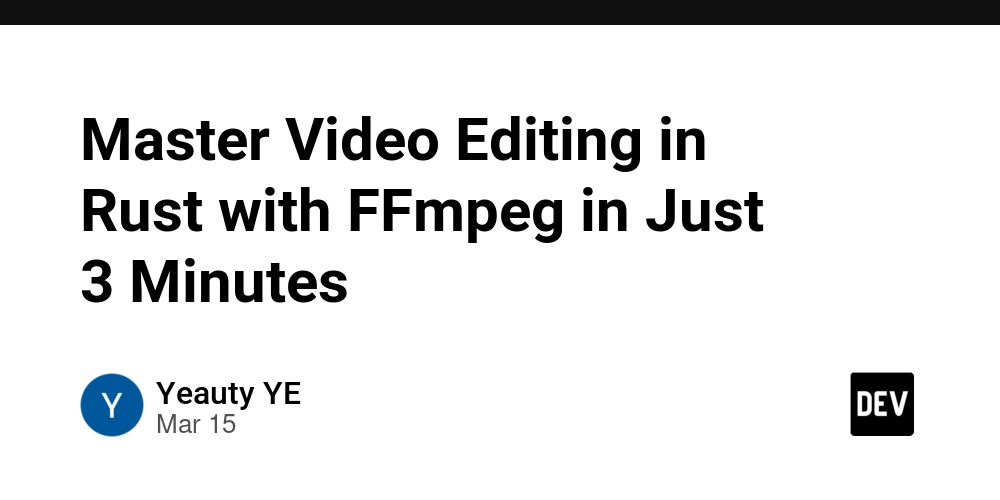
Master Video Editing in Rust with FFmpeg in Just 3 Minutes
Introduction
In today's era dominated by short-form video content, efficient video editing has become crucial for content creators and developers. Whether you're trimming video intros and endings, extracting highlights, making GIFs, or removing unwanted ads, video editing is a necessity.
However, editing one video is easy, but handling large batches of videos can be challenging:
- Manual editing: Time-consuming, inefficient, and impractical for large quantities.
- Professional tools (Premiere, After Effects): Powerful but lacking in automation for batch processing.
- FFmpeg command-line tool: Powerful yet complex; easy to make mistakes with intricate parameters.
Rust developers specifically face additional complexities when integrating FFmpeg directly:
- Complexity: FFmpeg's native C API is powerful but complicated, requiring extensive understanding of low-level details.
- Memory safety: Directly interacting with the C API can lead to memory leaks, unsafe operations, and reduced stability.
- Productivity loss: High learning curve, complex API structures, and tedious configurations hinder development efficiency.
The Solution: Integrating FFmpeg Elegantly in Rust
To address these challenges, Rust developers commonly use wrappers around FFmpeg's native C API. Libraries like ez-ffmpeg
offer ergonomic and safe interfaces, simplifying FFmpeg integration through user-friendly, memory-safe Rust abstractions. Such libraries typically abstract complex details, enabling developers to achieve video editing automation easily and securely.
Quick Example: Clipping Videos with Rust
Suppose you have a video test.mp4
and want to clip a 3-second segment starting at the 5-second mark, outputting to output.mp4
. Here's how you can accomplish this:
1. Install FFmpeg
macOS:
brew install ffmpeg
Windows:
vcpkg install ffmpeg
# Set VCPKG_ROOT if installing for the first time
2. Add Rust Dependency
Include ez-ffmpeg
in your project's Cargo.toml
:
[dependencies]
ez-ffmpeg = "*"
3. Write the Rust Code
use ez_ffmpeg::{FfmpegContext, Input, Output};
fn main() -> Result<(), Box<dyn std::error::Error>> {
FfmpegContext::builder()
.input(Input::from("test.mp4")
.set_start_time_us(5_000_000) // Start at 5 seconds
.set_recording_time_us(3_000_000)) // Record for 3 seconds
.output("output.mp4")
.build()?.start()?.wait()?;
Ok(())
}
Understanding the Code
Here's what's happening:
- Input video:
.input(Input::from("test.mp4")
Specifies the input video file.
- Setting Clip Duration
.set_start_time_us(5_000_000) // Start at 5 seconds
.set_recording_time_us(3_000_000) // Duration 3 seconds
- Output File
.output("output.mp4")
Defines the resulting clipped video.
- Executing the Process
.build()?.start()?.wait()?;
- Builds the FFmpeg task
- Starts the clipping process
- Waits until completion
Additional Clipping Scenarios
Clip only the first 10 seconds
.input(Input::from("test.mp4").set_recording_time_us(10_000_000))
Clip from 10 seconds to the end
.input(Input::from("test.mp4").set_start_time_us(10_000_000))
Clip and convert to GIF
FfmpegContext::builder()
.input(Input::from("test.mp4")
.set_start_time_us(5_000_000)
.set_recording_time_us(3_000_000))
.output("output.gif")
.build()?.start()?.wait()?;
This creates a 3-second GIF animation.
Summary
By leveraging Rust libraries that encapsulate FFmpeg functionality, developers can:
- Simplify usage: Use intuitive chain-call APIs to accomplish tasks quickly.
- Ensure safety: Benefit from Rust's inherent memory safety to avoid common pitfalls associated with direct C API usage.
- Boost productivity: Focus on core logic rather than complex FFmpeg configurations, significantly increasing efficiency.
If you're working on Rust applications related to short-form videos, live-streaming, or content creation, using such libraries can greatly streamline your workflow.