Java Virtual Threads: 5 Production-Ready Patterns for High-Performance Concurrency
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Java Virtual Threads represent a significant evolution in concurrent programming. Introduced as a preview feature in Java 19 and finalized in Java 21, they fundamentally change how we approach concurrency in Java applications. I'll explore practical patterns for implementing them effectively in production systems. Understanding Virtual Threads Virtual threads are lightweight threads managed by the JVM rather than the operating system. While platform threads map directly to OS threads and carry significant overhead, virtual threads operate differently. The JVM can manage thousands or even millions of virtual threads using just a handful of platform threads (carrier threads). // Traditional platform thread Thread platformThread = new Thread(() -> { System.out.println("Running on platform thread"); }); platformThread.start(); // Virtual thread Thread virtualThread = Thread.startVirtualThread(() -> { System.out.println("Running on virtual thread"); }); The key distinction is resource usage. Platform threads consume approximately 2MB of stack memory each and involve OS scheduling overhead. Virtual threads require only a few kilobytes and use a cooperative scheduling model within the JVM. Pattern 1: Thread-Per-Request for HTTP Servers The thread-per-request model becomes practical with virtual threads. Each client request can have its own thread without worrying about thread pool sizing or resource exhaustion. public class VirtualThreadServer { public static void main(String[] args) throws IOException { var server = HttpServer.create(new InetSocketAddress(8080), 0); server.createContext("/api", exchange -> { Thread.startVirtualThread(() -> { try { // Process request String response = processRequest(exchange); exchange.sendResponseHeaders(200, response.length()); try (OutputStream os = exchange.getResponseBody()) { os.write(response.getBytes()); } } catch (Exception e) { e.printStackTrace(); } }); }); server.start(); System.out.println("Server started on port 8080"); } private static String processRequest(HttpExchange exchange) { // Simulate work try { Thread.sleep(100); } catch (InterruptedException e) { Thread.currentThread().interrupt(); } return "Response processed by " + Thread.currentThread(); } } This approach scales to handle thousands of concurrent connections with minimal resources. The traditional concerns about thread pool exhaustion become irrelevant. Pattern 2: Structured Concurrency Structured concurrency provides a way to manage the lifecycle of multiple related tasks. Java's ExecutorService works seamlessly with virtual threads: public void processOrders(List orders) throws ExecutionException, InterruptedException { try (var executor = Executors.newVirtualThreadPerTaskExecutor()) { List futures = orders.stream() .map(order -> executor.submit(() -> processOrder(order))) .collect(Collectors.toList()); for (Future future : futures) { OrderResult result = future.get(); // Block until each task completes saveResult(result); } } // AutoCloseable handles shutdown } private OrderResult processOrder(Order order) { // Potentially blocking operations validateOrder(order); checkInventory(order); processPayment(order); return new OrderResult(order, OrderStatus.COMPLETED); } The newVirtualThreadPerTaskExecutor() creates an executor that spawns a new virtual thread for each submitted task. The structured approach ensures all threads complete before the method returns, simplifying resource management. Pattern 3: Parallel Data Processing Virtual threads excel at I/O-bound operations. When processing large datasets that require external service calls, virtual threads can parallelize the work efficiently: public List enrichCustomerData(List customers) throws InterruptedException { List results = Collections.synchronizedList(new ArrayList()); CountDownLatch latch = new CountDownLatch(customers.size()); for (Customer customer : customers) { Thread.startVirtualThread(() -> { try { // Make external API calls to enrich data CreditScore creditScore = creditScoreService.fetch(customer.getId()); PurchaseHistory history = purchaseHistoryService.fetch(customer.getId()); // Combine data EnrichedCustomer enriched = new Enriche
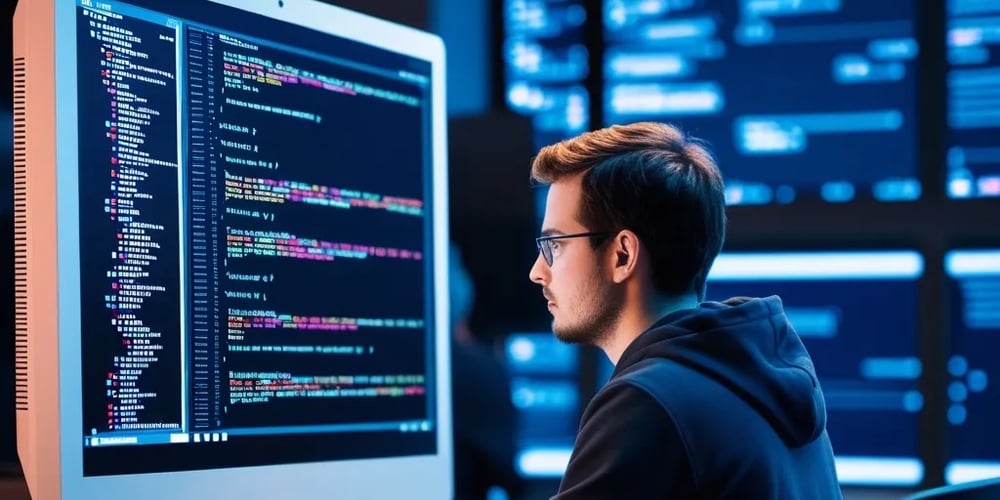
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Java Virtual Threads represent a significant evolution in concurrent programming. Introduced as a preview feature in Java 19 and finalized in Java 21, they fundamentally change how we approach concurrency in Java applications. I'll explore practical patterns for implementing them effectively in production systems.
Understanding Virtual Threads
Virtual threads are lightweight threads managed by the JVM rather than the operating system. While platform threads map directly to OS threads and carry significant overhead, virtual threads operate differently. The JVM can manage thousands or even millions of virtual threads using just a handful of platform threads (carrier threads).
// Traditional platform thread
Thread platformThread = new Thread(() -> {
System.out.println("Running on platform thread");
});
platformThread.start();
// Virtual thread
Thread virtualThread = Thread.startVirtualThread(() -> {
System.out.println("Running on virtual thread");
});
The key distinction is resource usage. Platform threads consume approximately 2MB of stack memory each and involve OS scheduling overhead. Virtual threads require only a few kilobytes and use a cooperative scheduling model within the JVM.
Pattern 1: Thread-Per-Request for HTTP Servers
The thread-per-request model becomes practical with virtual threads. Each client request can have its own thread without worrying about thread pool sizing or resource exhaustion.
public class VirtualThreadServer {
public static void main(String[] args) throws IOException {
var server = HttpServer.create(new InetSocketAddress(8080), 0);
server.createContext("/api", exchange -> {
Thread.startVirtualThread(() -> {
try {
// Process request
String response = processRequest(exchange);
exchange.sendResponseHeaders(200, response.length());
try (OutputStream os = exchange.getResponseBody()) {
os.write(response.getBytes());
}
} catch (Exception e) {
e.printStackTrace();
}
});
});
server.start();
System.out.println("Server started on port 8080");
}
private static String processRequest(HttpExchange exchange) {
// Simulate work
try {
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Response processed by " + Thread.currentThread();
}
}
This approach scales to handle thousands of concurrent connections with minimal resources. The traditional concerns about thread pool exhaustion become irrelevant.
Pattern 2: Structured Concurrency
Structured concurrency provides a way to manage the lifecycle of multiple related tasks. Java's ExecutorService works seamlessly with virtual threads:
public void processOrders(List<Order> orders) throws ExecutionException, InterruptedException {
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
List<Future<OrderResult>> futures = orders.stream()
.map(order -> executor.submit(() -> processOrder(order)))
.collect(Collectors.toList());
for (Future<OrderResult> future : futures) {
OrderResult result = future.get(); // Block until each task completes
saveResult(result);
}
} // AutoCloseable handles shutdown
}
private OrderResult processOrder(Order order) {
// Potentially blocking operations
validateOrder(order);
checkInventory(order);
processPayment(order);
return new OrderResult(order, OrderStatus.COMPLETED);
}
The newVirtualThreadPerTaskExecutor()
creates an executor that spawns a new virtual thread for each submitted task. The structured approach ensures all threads complete before the method returns, simplifying resource management.
Pattern 3: Parallel Data Processing
Virtual threads excel at I/O-bound operations. When processing large datasets that require external service calls, virtual threads can parallelize the work efficiently:
public List<EnrichedCustomer> enrichCustomerData(List<Customer> customers) throws InterruptedException {
List<EnrichedCustomer> results = Collections.synchronizedList(new ArrayList<>());
CountDownLatch latch = new CountDownLatch(customers.size());
for (Customer customer : customers) {
Thread.startVirtualThread(() -> {
try {
// Make external API calls to enrich data
CreditScore creditScore = creditScoreService.fetch(customer.getId());
PurchaseHistory history = purchaseHistoryService.fetch(customer.getId());
// Combine data
EnrichedCustomer enriched = new EnrichedCustomer(customer, creditScore, history);
results.add(enriched);
} finally {
latch.countDown();
}
});
}
latch.await(); // Wait for all threads to complete
return results;
}
This pattern is remarkably efficient because the threads automatically yield during blocking operations (API calls), allowing other virtual threads to make progress.
Pattern 4: Event Loop Integration
Many Java applications use event loops (like Netty or Vert.x). Virtual threads can integrate with these frameworks to simplify the programming model while maintaining performance:
public class VirtualThreadNettyHandler extends SimpleChannelInboundHandler<HttpRequest> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, HttpRequest request) {
Thread.startVirtualThread(() -> {
try {
// Process request in a blocking manner
String result = processRequest(request);
// Write response back to the event loop
ctx.executor().execute(() -> {
FullHttpResponse response = new DefaultFullHttpResponse(
HTTP_1_1, OK,
Unpooled.copiedBuffer(result, CharsetUtil.UTF_8)
);
ctx.writeAndFlush(response);
});
} catch (Exception e) {
ctx.executor().execute(() -> {
ctx.fireExceptionCaught(e);
});
}
});
}
private String processRequest(HttpRequest request) throws Exception {
// Blocking operations are fine here
return expensiveOperation();
}
}
This hybrid approach leverages virtual threads for the business logic while maintaining the non-blocking nature of the event loop for I/O operations.
Pattern 5: Blocking API Adaptation
Many legacy APIs in Java are blocking by design. Virtual threads allow using these APIs without redesigning them to be asynchronous:
public class JdbcRepository {
private final DataSource dataSource;
public JdbcRepository(DataSource dataSource) {
this.dataSource = dataSource;
}
public List<Customer> findAllCustomers() {
List<Customer> customers = new ArrayList<>();
try (Connection conn = dataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement("SELECT * FROM customers");
ResultSet rs = stmt.executeQuery()) {
while (rs.next()) {
Customer customer = new Customer(
rs.getLong("id"),
rs.getString("name"),
rs.getString("email")
);
customers.add(customer);
}
} catch (SQLException e) {
throw new RuntimeException("Error fetching customers", e);
}
return customers;
}
}
// Usage with virtual threads
public void processAllCustomers() {
Thread.startVirtualThread(() -> {
List<Customer> customers = repository.findAllCustomers();
customers.forEach(this::processCustomer);
});
}
When the JDBC driver blocks on I/O, the virtual thread yields to another thread, allowing efficient use of system resources. This works automatically without changing the JDBC API.
Performance Considerations
Virtual threads shine with I/O-bound workloads but offer less benefit for CPU-intensive tasks. I've found the following considerations critical when implementing virtual threads:
- Monitor thread pinning: When virtual threads execute code within a synchronized block, they temporarily "pin" to their carrier thread, preventing yielding during blocking operations.
// Avoid synchronized blocks around blocking operations
synchronized (lock) {
// Don't do this - thread will be pinned
databaseService.queryWithLongRunningOperation();
}
// Better approach
ReentrantLock lock = new ReentrantLock();
lock.lock();
try {
// Preparation work
} finally {
lock.unlock();
}
// Now perform blocking operation without pinning
databaseService.queryWithLongRunningOperation();
- Use thread-local variables carefully: Virtual threads may use more memory when each carries heavy thread-local variables.
// Be cautious with thread locals
private static final ThreadLocal<ExpensiveObject> threadLocal =
ThreadLocal.withInitial(() -> new ExpensiveObject());
// Consider alternatives like passing context explicitly
public void processWithContext(Context context) {
// Use context instead of thread local
}
- Thread pools are usually unnecessary: Creating a thread pool of virtual threads often defeats their purpose. Instead, create new virtual threads as needed.
// Avoid this pattern with virtual threads
ExecutorService virtualThreadPool = Executors.newFixedThreadPool(100,
Thread.ofVirtual().factory());
// Prefer this instead
ExecutorService executor = Executors.newVirtualThreadPerTaskExecutor();
Practical Implementation
I've implemented virtual threads in a high-throughput API service that needed to communicate with multiple downstream services. The transformation was remarkable:
@RestController
public class ProductApiController {
private final CatalogService catalogService;
private final InventoryService inventoryService;
private final PricingService pricingService;
@GetMapping("/products/{id}")
public ProductDetails getProductDetails(@PathVariable String id) {
// These operations happen in the same virtual thread
ProductInfo info = catalogService.getProductInfo(id);
InventoryStatus inventory = inventoryService.checkInventory(id);
PricingData pricing = pricingService.getPricing(id);
return new ProductDetails(info, inventory, pricing);
}
}
With traditional approaches, I'd use CompletableFuture for each service call to avoid blocking. With virtual threads, I write simpler, more maintainable code that performs just as well.
Monitoring and Debugging
Monitoring virtual threads requires different approaches than platform threads. JDK Flight Recorder and thread dumps are adapted to be virtual thread-aware:
// Creating a named virtual thread for easier debugging
Thread vt = Thread.ofVirtual()
.name("order-processor-" + orderId)
.start(() -> processOrder(orderId));
// Get thread dumps with jcmd
// jcmd Thread.dump_to_file -format=json
Thread names and thread groups retain their usefulness for organization and debugging.
Migration Strategy
When migrating to virtual threads, I recommend a phased approach:
- Start with isolated components that have clear I/O bottlenecks
- Update thread creation code but keep using ExecutorService interfaces
- Test thoroughly and monitor for thread pinning issues
- Gradually eliminate artificial thread pool size limitations
// Before migration
ExecutorService executor = Executors.newFixedThreadPool(200);
// After migration - same interface, different implementation
ExecutorService executor = Executors.newVirtualThreadPerTaskExecutor();
This approach maintains compatibility while introducing the benefits of virtual threads.
Conclusion
Java virtual threads provide a powerful tool for building highly concurrent applications without sacrificing code simplicity. They excel at I/O-bound workloads and enable developers to write straightforward sequential code that scales efficiently.
The five patterns I've shared represent my experiences implementing virtual threads in production systems. By understanding these patterns and their appropriate use cases, you can leverage virtual threads to build more efficient, maintainable, and scalable Java applications.
Virtual threads aren't a silver bullet for all concurrency challenges, but they significantly simplify many common scenarios. As Java continues to evolve, these lightweight threads will become an increasingly essential part of the Java developer's toolkit.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva