Intl API for Internationalization
A Definitive Guide to the Intl API for Internationalization in JavaScript Table of Contents Introduction Historical and Technical Context Understanding the Intl API 3.1. The Structure of the Intl API 3.2. The Key Interfaces In-depth Code Examples 4.1. Number Formatting 4.2. Date and Time Formatting 4.3. Collation 4.4. Pluralization Advanced Implementation Techniques 5.1. Using Locales Dynamically 5.2. Custom Formatting Performance Considerations & Optimization Strategies Comparison with Alternative Approaches Real-World Use Cases Potential Pitfalls and Advanced Debugging Techniques Conclusion References and Resources 1. Introduction As the world becomes increasingly interconnected, the need for developers to implement internationalization (i18n) in their applications has grown. The Intl API in JavaScript provides built-in solutions for common internationalization tasks, making it a powerful tool for developers working in a global context. This comprehensive guide delves into the nuances of the Intl API, demonstrating its capabilities and offering practical, in-depth examples along with advanced techniques. 2. Historical and Technical Context Prior to the introduction of the Intl API in ECMAScript 2015 (ES6), developers often relied on complex libraries (such as Globalize.js or moment.js) or manually coded solutions for internationalization. The need for standardized methods arose from the challenges of supporting multiple locales, different numeral systems, and culturally varying formats. The Intl API was designed to address these issues by providing native support for a wide array of internationalization features, leveraging the International Components for Unicode (ICU) library, which is renowned for its extensive locale data and capabilities. 3. Understanding the Intl API 3.1. The Structure of the Intl API The Intl API comprises several constructors that provide functionality across various aspects of internationalization: Intl.NumberFormat Intl.DateTimeFormat Intl.Collator Intl.RelativeTimeFormat Intl.ListFormat Intl.PluralRules Each of these constructors can be customized via options and can accept a locale string to specify the desired locale format. 3.2. The Key Interfaces Each interface has specific properties and methods that allow developers to format dates, numbers, and strings according to localization standards. Intl.NumberFormat: Formats numbers in localized styles. Intl.DateTimeFormat: Localizes date and time representations. Intl.Collator: Handles string comparison with locale sensitivity. Intl.RelativeTimeFormat: Formats relative time as per locale. Intl.ListFormat: Formats lists of items in a localized manner. Intl.PluralRules: Determines pluralization logic based on locale. 4. In-depth Code Examples 4.1. Number Formatting const number = 1234567.89; const germanFormatter = new Intl.NumberFormat('de-DE', { style: 'currency', currency: 'EUR', minimumFractionDigits: 2, }); console.log(germanFormatter.format(number)); // Outputs: 1.234.567,89 € In this example, we format a number as currency in a German locale, showcasing how the relevant styles and minimum fraction digits are applied accordingly. 4.2. Date and Time Formatting const date = new Date('2023-10-01T00:00:00Z'); const usDateFormatter = new Intl.DateTimeFormat('en-US', { year: 'numeric', month: 'long', day: 'numeric', }); console.log(usDateFormatter.format(date)); // Outputs: October 1, 2023 This code formats a Date object in the U.S. English style, demonstrating the format's configurability through various options like year, month, and day. 4.3. Collation const collator = new Intl.Collator('en', { sensitivity: 'base', }); const fruits = ['banana', 'Apple', 'cherry']; fruits.sort(collator.compare); console.log(fruits); // Outputs: ["Apple", "banana", "cherry"] Using Intl.Collator, we sort an array of strings, showcasing locale-sensitive string comparison, which can factor in accents and case sensitivity. 4.4. Pluralization const pluralRule = new Intl.PluralRules('en-US'); console.log(pluralRule.select(1)); // Outputs: 'one' console.log(pluralRule.select(2)); // Outputs: 'other' In this example, we determine the pluralization for different quantities, an essential feature for displaying time-based messages and item counts. 5. Advanced Implementation Techniques 5.1. Using Locales Dynamically For applications that need to adapt to user preferences, utilize the navigator.language property to get the browser's preferred language, which can then inform the Intl API usage. const userLocale = navigator.language || 'en-US'; const formatter = new Intl.NumberFormat(userLocale); console.log(formatter.format(1234567.89)); 5.2. Custom Formatting You can extend Int
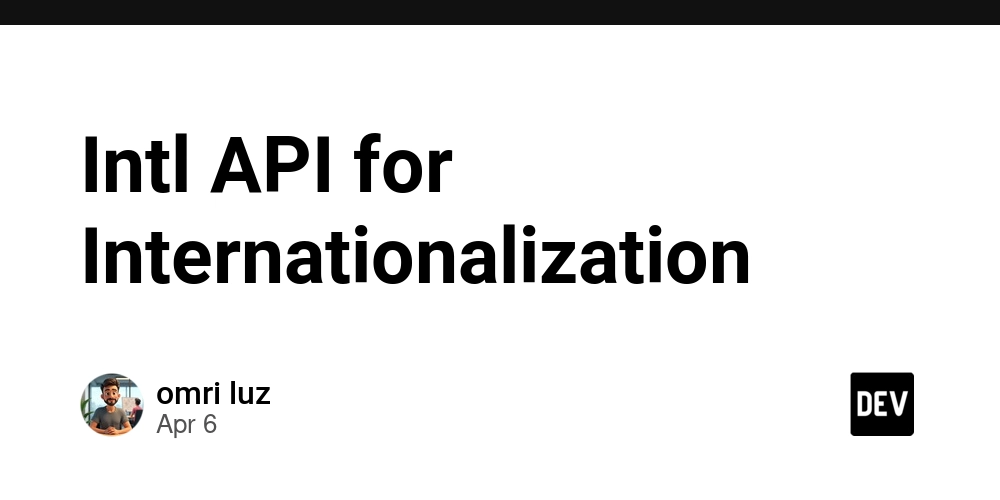
A Definitive Guide to the Intl API for Internationalization in JavaScript
Table of Contents
- Introduction
- Historical and Technical Context
-
Understanding the Intl API
- 3.1. The Structure of the Intl API
- 3.2. The Key Interfaces
-
In-depth Code Examples
- 4.1. Number Formatting
- 4.2. Date and Time Formatting
- 4.3. Collation
- 4.4. Pluralization
-
Advanced Implementation Techniques
- 5.1. Using Locales Dynamically
- 5.2. Custom Formatting
- Performance Considerations & Optimization Strategies
- Comparison with Alternative Approaches
- Real-World Use Cases
- Potential Pitfalls and Advanced Debugging Techniques
- Conclusion
- References and Resources
1. Introduction
As the world becomes increasingly interconnected, the need for developers to implement internationalization (i18n) in their applications has grown. The Intl
API in JavaScript provides built-in solutions for common internationalization tasks, making it a powerful tool for developers working in a global context. This comprehensive guide delves into the nuances of the Intl
API, demonstrating its capabilities and offering practical, in-depth examples along with advanced techniques.
2. Historical and Technical Context
Prior to the introduction of the Intl
API in ECMAScript 2015 (ES6), developers often relied on complex libraries (such as Globalize.js or moment.js) or manually coded solutions for internationalization. The need for standardized methods arose from the challenges of supporting multiple locales, different numeral systems, and culturally varying formats.
The Intl
API was designed to address these issues by providing native support for a wide array of internationalization features, leveraging the International Components for Unicode (ICU) library, which is renowned for its extensive locale data and capabilities.
3. Understanding the Intl API
3.1. The Structure of the Intl API
The Intl
API comprises several constructors that provide functionality across various aspects of internationalization:
Intl.NumberFormat
Intl.DateTimeFormat
Intl.Collator
Intl.RelativeTimeFormat
Intl.ListFormat
Intl.PluralRules
Each of these constructors can be customized via options and can accept a locale string to specify the desired locale format.
3.2. The Key Interfaces
Each interface has specific properties and methods that allow developers to format dates, numbers, and strings according to localization standards.
-
Intl.NumberFormat
: Formats numbers in localized styles. -
Intl.DateTimeFormat
: Localizes date and time representations. -
Intl.Collator
: Handles string comparison with locale sensitivity. -
Intl.RelativeTimeFormat
: Formats relative time as per locale. -
Intl.ListFormat
: Formats lists of items in a localized manner. -
Intl.PluralRules
: Determines pluralization logic based on locale.
4. In-depth Code Examples
4.1. Number Formatting
const number = 1234567.89;
const germanFormatter = new Intl.NumberFormat('de-DE', {
style: 'currency',
currency: 'EUR',
minimumFractionDigits: 2,
});
console.log(germanFormatter.format(number)); // Outputs: 1.234.567,89 €
In this example, we format a number as currency in a German locale, showcasing how the relevant styles and minimum fraction digits are applied accordingly.
4.2. Date and Time Formatting
const date = new Date('2023-10-01T00:00:00Z');
const usDateFormatter = new Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: 'long',
day: 'numeric',
});
console.log(usDateFormatter.format(date)); // Outputs: October 1, 2023
This code formats a Date object in the U.S. English style, demonstrating the format's configurability through various options like year, month, and day.
4.3. Collation
const collator = new Intl.Collator('en', {
sensitivity: 'base',
});
const fruits = ['banana', 'Apple', 'cherry'];
fruits.sort(collator.compare);
console.log(fruits); // Outputs: ["Apple", "banana", "cherry"]
Using Intl.Collator
, we sort an array of strings, showcasing locale-sensitive string comparison, which can factor in accents and case sensitivity.
4.4. Pluralization
const pluralRule = new Intl.PluralRules('en-US');
console.log(pluralRule.select(1)); // Outputs: 'one'
console.log(pluralRule.select(2)); // Outputs: 'other'
In this example, we determine the pluralization for different quantities, an essential feature for displaying time-based messages and item counts.
5. Advanced Implementation Techniques
5.1. Using Locales Dynamically
For applications that need to adapt to user preferences, utilize the navigator.language
property to get the browser's preferred language, which can then inform the Intl
API usage.
const userLocale = navigator.language || 'en-US';
const formatter = new Intl.NumberFormat(userLocale);
console.log(formatter.format(1234567.89));
5.2. Custom Formatting
You can extend Intl
capabilities by creating custom formatting functions while leveraging Intl
for base formatting.
function customFormatCurrency(number, locale, currency) {
const formatter = new Intl.NumberFormat(locale, {
style: 'currency',
currency,
});
return formatter.format(number);
}
console.log(customFormatCurrency(12345.67, 'en-US', 'USD')); // $12,345.67
6. Performance Considerations & Optimization Strategies
When utilizing the Intl
API, creating new instances of formatting objects can be expensive due to their complexity. It's a best practice to instantiate these objects once and reuse them throughout your application.
const germanFormatter = new Intl.NumberFormat('de-DE');
const usFormatter = new Intl.NumberFormat('en-US');
function formatPrice(price, locale) {
return locale === 'de-DE' ? germanFormatter.format(price) : usFormatter.format(price);
}
7. Comparison with Alternative Approaches
Libraries to Consider
Moment.js: Though primarily focused on date manipulation, Moment.js has some i18n capabilities, but it has been deprecated in favor of more modern solutions like Luxon or date-fns.
FormatJS: An extensive suite for i18n that offers capabilities beyond what is exposed directly by the
Intl
API, allowing for more detailed handling of messages, variable interpolation, and more.
Pros and Cons
Approach | Pros | Cons |
---|---|---|
Intl API |
Native, performance-optimized; easy to use | Limited in certain advanced areas compared to libraries like FormatJS |
Moment.js | Rich feature set but large footprint; performance overhead | Deprecated |
FormatJS | Comprehensive and plenty of features; supports complex i18n scenarios | More complex and can be verbose |
8. Real-World Use Cases
E-commerce Applications: Using
Intl.NumberFormat
to handle currency formats dynamically based on user locale improves user experience significantly. For instance, when a user from Germany shops online, prices automatically show in Euros, formatted correctly.Calendar Applications: Applications that manage events can utilize
Intl.DateTimeFormat
to represent date and time in users' preferred formats, enhancing accessibility and clarity.Content Management Systems: Many CMS platforms implement
Intl
for localized content delivery, such as translations or adapting item counts to show singular vs. plural forms in user interfaces.
9. Potential Pitfalls and Advanced Debugging Techniques
Common Pitfalls
Locale Availability: Not all browsers support all locales. Always have a fallback for unsupported locales.
Caching Issues: To avoid creating new instances of formatter objects unnecessarily, cache your formatters effectively.
Debugging Techniques
When debugging internationalization issues, consider the following:
Use the console: Log the locale being set (
console.log(Intl.NumberFormat.supportedLocalesOf(locale));
) to confirm support.Polyfills: Employ polyfills (like
Intl.js
) only when necessary, as they may lead to performance degradation in heavy-use scenarios.Testing with Different Locales: Automated tests should include various locales to ensure functionality across different formats and pluralization rules.
10. Conclusion
The Intl
API is an indispensable modern tool for developers aiming to build internationally aware applications. By leveraging its extensive features and capabilities, you can create user experiences that resonate with diverse user bases. This guide serves as a comprehensive resource to not only understand but also to utilize the Intl
API effectively within your JavaScript applications.
11. References and Resources
- MDN Web Docs - Intl API
- ECMA-402 Specification
- ICU Project
- FormatJS
- Advanced i18n Techniques with JavaScript
This exhaustive exploration of the Intl
API should equip senior developers with both the foundational and advanced knowledge necessary to implement robust internationalization practices in their applications.