Implementing WebAuthn: 6 Practical Techniques for Passwordless Authentication
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Modern web applications demand authentication mechanisms that balance security and user experience. WebAuthn represents a significant advancement in this direction, offering passwordless authentication that enhances security while simplifying the user experience. I'll explore the practical implementation techniques that make WebAuthn effective in today's web environment. Understanding WebAuthn Fundamentals WebAuthn (Web Authentication) is an API that allows websites to implement strong, passwordless authentication using public key cryptography. Rather than sharing secrets across the internet, WebAuthn creates a public-private key pair where the private key never leaves the user's device. The protocol works through a challenge-response mechanism. When a user attempts to register or authenticate, the server sends a challenge that must be signed using the private key stored on the user's device. This approach effectively eliminates password-related vulnerabilities like phishing, credential stuffing, and database breaches. Technique 1: Implementing Robust Challenge Generation The security of WebAuthn depends heavily on proper challenge generation. A cryptographically secure random challenge serves as the foundation for both registration and authentication. For effective implementation, your server must generate unpredictable challenges with sufficient entropy: // Server-side challenge generation (Node.js example) const crypto = require('crypto'); function generateAuthChallenge() { // Generate a 32-byte random challenge const challenge = crypto.randomBytes(32); // Convert to Base64URL format for transmission const challengeBase64 = challenge.toString('base64') .replace(/\+/g, '-') .replace(/\//g, '_') .replace(/=/g, ''); // Store the challenge in the user's session req.session.authChallenge = challengeBase64; return challengeBase64; } The challenge must be stored server-side (typically in a session) to verify the response later. Challenges should be single-use and time-limited to prevent replay attacks. Technique 2: Attestation Verification and Security Levels Attestation provides proof about the authenticator's origin and capabilities. Proper implementation requires verifying the attestation statement to ensure the credentials meet your security requirements. // Using @simplewebauthn/server library const { verifyAttestationResponse } = require('@simplewebauthn/server'); async function verifyRegistration(attestationResponse) { try { const verification = await verifyAttestationResponse({ credential: attestationResponse, expectedChallenge: session.challenge, expectedOrigin: 'https://your-app.com', expectedRPID: 'your-app.com', requireUserVerification: true }); if (verification.verified) { // Store the credential in your database const { credentialID, credentialPublicKey } = verification.registrationInfo; await storeCredential(user.id, credentialID, credentialPublicKey); return true; } return false; } catch (error) { console.error('Attestation verification failed:', error); return false; } } You can implement different attestation levels: None: No attestation information requested Indirect: Anonymized attestation information Direct: Full attestation information including manufacturer details Most applications should use "indirect" attestation for a balance of security and privacy. Technique 3: Secure Credential Storage and Management Proper credential storage is crucial for WebAuthn security. You need to store the credential ID, public key, and relevant metadata for each user. // Database schema example (PostgreSQL) CREATE TABLE user_credentials ( id SERIAL PRIMARY KEY, user_id INTEGER NOT NULL REFERENCES users(id), credential_id BYTEA NOT NULL, public_key BYTEA NOT NULL, counter BIGINT NOT NULL DEFAULT 0, credential_device_type VARCHAR(32) NOT NULL, credential_backed_up BOOLEAN NOT NULL, transports TEXT[] NULL, created_at TIMESTAMP WITH TIME ZONE DEFAULT NOW(), last_used_at TIMESTAMP WITH TIME ZONE NULL ); When implementing credential storage, consider these best practices: // Storing a credential example async function storeUserCredential(userId, credentialData) { const { credentialID, credentialPublicKey, counter, credentialDeviceType, credentialBackedUp } = credentialData; // Base64URL encode binary data for storage const credentialIdEncoded = Buffer.from(credentialID).toString('base64url'); const publicKeyEncoded = Buffer.from(credentialPublicKey).toString('base64url'); await db.query( `INSERT INTO user_credentials (user_id, credential_id, public_key, counter, credential_d
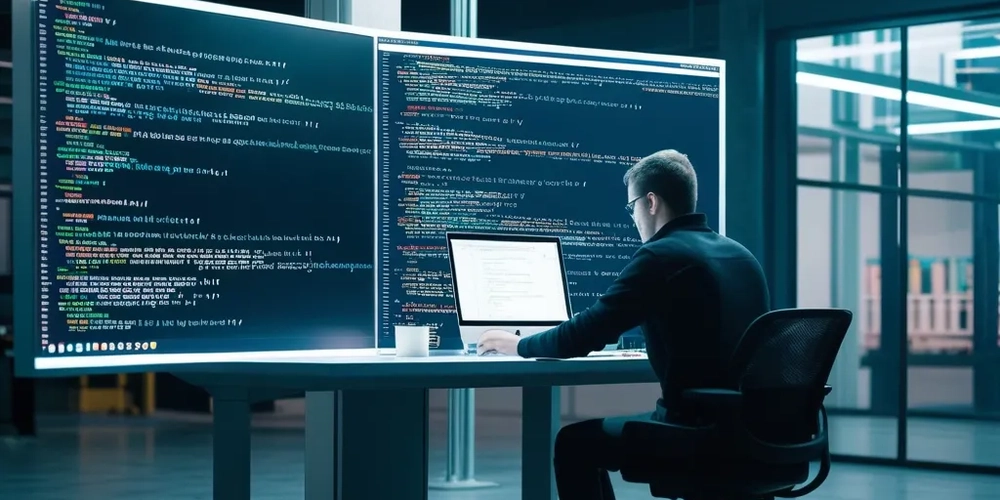
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Modern web applications demand authentication mechanisms that balance security and user experience. WebAuthn represents a significant advancement in this direction, offering passwordless authentication that enhances security while simplifying the user experience. I'll explore the practical implementation techniques that make WebAuthn effective in today's web environment.
Understanding WebAuthn Fundamentals
WebAuthn (Web Authentication) is an API that allows websites to implement strong, passwordless authentication using public key cryptography. Rather than sharing secrets across the internet, WebAuthn creates a public-private key pair where the private key never leaves the user's device.
The protocol works through a challenge-response mechanism. When a user attempts to register or authenticate, the server sends a challenge that must be signed using the private key stored on the user's device. This approach effectively eliminates password-related vulnerabilities like phishing, credential stuffing, and database breaches.
Technique 1: Implementing Robust Challenge Generation
The security of WebAuthn depends heavily on proper challenge generation. A cryptographically secure random challenge serves as the foundation for both registration and authentication.
For effective implementation, your server must generate unpredictable challenges with sufficient entropy:
// Server-side challenge generation (Node.js example)
const crypto = require('crypto');
function generateAuthChallenge() {
// Generate a 32-byte random challenge
const challenge = crypto.randomBytes(32);
// Convert to Base64URL format for transmission
const challengeBase64 = challenge.toString('base64')
.replace(/\+/g, '-')
.replace(/\//g, '_')
.replace(/=/g, '');
// Store the challenge in the user's session
req.session.authChallenge = challengeBase64;
return challengeBase64;
}
The challenge must be stored server-side (typically in a session) to verify the response later. Challenges should be single-use and time-limited to prevent replay attacks.
Technique 2: Attestation Verification and Security Levels
Attestation provides proof about the authenticator's origin and capabilities. Proper implementation requires verifying the attestation statement to ensure the credentials meet your security requirements.
// Using @simplewebauthn/server library
const { verifyAttestationResponse } = require('@simplewebauthn/server');
async function verifyRegistration(attestationResponse) {
try {
const verification = await verifyAttestationResponse({
credential: attestationResponse,
expectedChallenge: session.challenge,
expectedOrigin: 'https://your-app.com',
expectedRPID: 'your-app.com',
requireUserVerification: true
});
if (verification.verified) {
// Store the credential in your database
const { credentialID, credentialPublicKey } = verification.registrationInfo;
await storeCredential(user.id, credentialID, credentialPublicKey);
return true;
}
return false;
} catch (error) {
console.error('Attestation verification failed:', error);
return false;
}
}
You can implement different attestation levels:
- None: No attestation information requested
- Indirect: Anonymized attestation information
- Direct: Full attestation information including manufacturer details
Most applications should use "indirect" attestation for a balance of security and privacy.
Technique 3: Secure Credential Storage and Management
Proper credential storage is crucial for WebAuthn security. You need to store the credential ID, public key, and relevant metadata for each user.
// Database schema example (PostgreSQL)
CREATE TABLE user_credentials (
id SERIAL PRIMARY KEY,
user_id INTEGER NOT NULL REFERENCES users(id),
credential_id BYTEA NOT NULL,
public_key BYTEA NOT NULL,
counter BIGINT NOT NULL DEFAULT 0,
credential_device_type VARCHAR(32) NOT NULL,
credential_backed_up BOOLEAN NOT NULL,
transports TEXT[] NULL,
created_at TIMESTAMP WITH TIME ZONE DEFAULT NOW(),
last_used_at TIMESTAMP WITH TIME ZONE NULL
);
When implementing credential storage, consider these best practices:
// Storing a credential example
async function storeUserCredential(userId, credentialData) {
const {
credentialID,
credentialPublicKey,
counter,
credentialDeviceType,
credentialBackedUp
} = credentialData;
// Base64URL encode binary data for storage
const credentialIdEncoded = Buffer.from(credentialID).toString('base64url');
const publicKeyEncoded = Buffer.from(credentialPublicKey).toString('base64url');
await db.query(
`INSERT INTO user_credentials
(user_id, credential_id, public_key, counter, credential_device_type, credential_backed_up)
VALUES ($1, $2, $3, $4, $5, $6)`,
[userId, credentialIdEncoded, publicKeyEncoded, counter, credentialDeviceType, credentialBackedUp]
);
}
Ensure your database queries are protected against SQL injection, and consider encrypting sensitive fields for additional security.
Technique 4: Implementing User Presence and Verification
WebAuthn supports different levels of user verification, from simple presence checks to biometric verification. This implementation detail affects both security and user experience.
// Client-side authentication options
async function authenticateUser() {
// Get challenge from server
const response = await fetch('/api/auth/challenge');
const { challenge, allowCredentials } = await response.json();
// Configure authentication options
const authOptions = {
challenge: base64URLToBuffer(challenge),
rpId: window.location.hostname,
allowCredentials: allowCredentials.map(cred => ({
id: base64URLToBuffer(cred.id),
type: 'public-key',
transports: cred.transports || ['internal', 'usb', 'ble', 'nfc']
})),
userVerification: 'preferred', // or 'required' for stronger security
timeout: 60000
};
try {
// Start the authentication process
const credential = await navigator.credentials.get({
publicKey: authOptions
});
// Send the response to the server for verification
const result = await verifyWithServer(credential);
if (result.success) {
// Authentication successful
window.location.href = '/dashboard';
}
} catch (error) {
console.error('Authentication failed:', error);
}
}
The userVerification
parameter can be set to:
- discouraged: No verification needed (just presence)
- preferred: Verification if available
- required: Must verify (biometrics/PIN)
For high-security applications, use 'required' to ensure stronger authentication through biometrics or PINs.
Technique 5: Cross-Platform Credential Management
Supporting multiple devices and credential types improves user experience while maintaining security. This requires careful management of different authenticator types.
// Client-side credential creation with platform/cross-platform options
async function registerNewDevice(preferPlatform = true) {
// Get registration options from server
const response = await fetch('/api/auth/registration-options');
const options = await response.json();
// Configure credential creation
const publicKeyOptions = {
challenge: base64URLToBuffer(options.challenge),
rp: {
name: 'Your Application',
id: window.location.hostname
},
user: {
id: base64URLToBuffer(options.userId),
name: options.userName,
displayName: options.userDisplayName
},
pubKeyCredParams: [
{ type: 'public-key', alg: -7 }, // ES256
{ type: 'public-key', alg: -257 } // RS256
],
authenticatorSelection: {
authenticatorAttachment: preferPlatform ? 'platform' : 'cross-platform',
userVerification: 'preferred',
requireResidentKey: false,
},
timeout: 60000,
attestation: 'indirect'
};
try {
const credential = await navigator.credentials.create({
publicKey: publicKeyOptions
});
// Send to server for verification and storage
return await submitCredentialToServer(credential);
} catch (error) {
console.error('Registration failed:', error);
throw error;
}
}
The key elements for cross-platform support include:
- Allowing both platform and cross-platform authenticators
- Supporting various transport methods (USB, NFC, Bluetooth)
- Enabling users to register multiple credentials
When implementing this approach, provide a clear UI that shows users which devices they have registered and allows them to manage these credentials.
Technique 6: Implementing Fallback Authentication Methods
Even with WebAuthn, you need fallback mechanisms for cases where users can't access their authenticators. This requires implementing alternative authentication paths.
// Client-side fallback authentication
async function handleAuthenticationFlow() {
try {
// Try WebAuthn first
await authenticateWithWebAuthn();
} catch (error) {
// If WebAuthn fails, offer alternatives
console.log('WebAuthn authentication failed, offering alternatives');
// Show fallback options UI
showFallbackOptions();
}
}
function showFallbackOptions() {
const fallbackContainer = document.getElementById('fallback-auth');
fallbackContainer.innerHTML = `
Authentication Options
`;
document.getElementById('recovery-code').addEventListener('click', startRecoveryCodeFlow);
document.getElementById('email-link').addEventListener('click', sendMagicLink);
document.getElementById('try-again').addEventListener('click', authenticateWithWebAuthn);
fallbackContainer.style.display = 'block';
}
For the server-side implementation of recovery codes:
// Generate recovery codes during account setup
function generateRecoveryCodes(userId) {
const codes = [];
for (let i = 0; i < 10; i++) {
// Generate a random code (4 blocks of 5 characters)
const code = Array.from({ length: 4 }, () =>
crypto.randomBytes(5).toString('hex')
).join('-');
codes.push(code);
}
// Hash the codes before storing them
const hashedCodes = codes.map(code => bcrypt.hashSync(code, 10));
// Store hashed codes in database
storeUserRecoveryCodes(userId, hashedCodes);
// Return plaintext codes to show to the user once
return codes;
}
A comprehensive fallback strategy typically includes:
- Recovery codes (one-time use, generated during setup)
- Email verification links or temporary access codes
- SMS verification as a last resort (though less secure)
- Customer support assisted recovery for enterprise applications
Practical Implementation Considerations
When implementing these techniques, I've found several practical considerations critical for success:
User Experience Design: The technical implementation must be paired with clear user interfaces. Users need to understand:
- What WebAuthn is and why it's more secure
- How to use their authenticators
- What to do if they lose access to their authenticators
Progressive Enhancement: Start with traditional authentication and gradually introduce WebAuthn:
// Check for WebAuthn support
function isWebAuthnSupported() {
return window.PublicKeyCredential !== undefined &&
typeof window.PublicKeyCredential === 'function';
}
// Conditionally show WebAuthn options
function renderAuthOptions() {
const authContainer = document.getElementById('auth-options');
if (isWebAuthnSupported()) {
authContainer.innerHTML = `
or
`;
document.getElementById('webauthn-auth').addEventListener('click', startWebAuthnAuth);
} else {
authContainer.innerHTML = `
Your browser doesn't support passwordless authentication.
`
;
}
document.getElementById('password-auth').addEventListener('click', showPasswordForm);
}
Browser Compatibility: While WebAuthn support is growing, you need to handle browsers that don't support it:
// Server-side browser capability detection
function detectWebAuthnSupport(req) {
const userAgent = req.headers['user-agent'];
// Simple detection logic (in practice, use a more comprehensive library)
const isChrome = /Chrome\/(\d+)/.test(userAgent);
const chromeVersion = isChrome ? parseInt(RegExp.$1, 10) : 0;
const isFirefox = /Firefox\/(\d+)/.test(userAgent);
const firefoxVersion = isFirefox ? parseInt(RegExp.$1, 10) : 0;
const isSafari = /Version\/(\d+).*Safari/.test(userAgent);
const safariVersion = isSafari ? parseInt(RegExp.$1, 10) : 0;
// Chrome 67+, Firefox 60+, Safari 13+ support WebAuthn
return (isChrome && chromeVersion >= 67) ||
(isFirefox && firefoxVersion >= 60) ||
(isSafari && safariVersion >= 13);
}
Error Handling: WebAuthn operations can fail for various reasons, from user cancellation to hardware issues. Comprehensive error handling improves user experience:
async function handleWebAuthnOperation(operation) {
try {
return await operation();
} catch (error) {
// Standard WebAuthn errors
if (error.name === 'NotAllowedError') {
showUserMessage('The operation was cancelled or timed out');
} else if (error.name === 'SecurityError') {
showUserMessage('The operation failed due to a security issue');
} else if (error.name === 'NotSupportedError') {
showUserMessage('This operation is not supported by your authenticator');
} else if (error.name === 'InvalidStateError') {
showUserMessage('The authenticator is in an invalid state');
} else {
// Generic error
console.error('WebAuthn error:', error);
showUserMessage('An error occurred during authentication. Please try again.');
}
throw error;
}
}
Real-World Success Factors
From my experience implementing WebAuthn, I've identified key factors that determine success:
Clear Communication: Explain the benefits of WebAuthn to users in simple terms - "more secure and easier than passwords."
Gradual Adoption: Introduce WebAuthn as an optional feature before making it the primary authentication method.
Device Management: Give users the ability to view, name, and remove their registered authenticators.
Account Recovery Planning: Design recovery flows before rolling out WebAuthn to avoid user lockouts.
Server Resilience: Implement proper error handling and validation on the server to prevent malformed requests from causing issues.
WebAuthn represents a significant improvement in web authentication. By carefully implementing these six techniques, you can create a secure, user-friendly authentication system that eliminates the vulnerabilities associated with passwords while improving the user experience.
The future of authentication is passwordless, and WebAuthn is leading this transformation. As more browsers and platforms support these standards, the techniques described here will become increasingly valuable for modern web applications seeking to balance security and usability.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva