How to Build an AI-Powered UI Component in Vue 3
Introduction & Context The rise of AI APIs like OpenAI's GPT and Hugging Face Transformers has opened a new frontier for frontend developers. Today, you can enrich your Vue apps with AI-driven features—without being a machine learning expert. This tutorial walks you through building an AI-powered UI component in Vue 3. You'll learn how to connect to an AI API, handle user input, display responses, and create a polished, interactive user experience. Whether you're working on chatbots, intelligent writing assistants, or form enhancers, this post is for you. Goals and What You’ll Learn By the end of this article, you’ll learn how to: Integrate OpenAI’s API into a Vue 3 project Build a smart text component that suggests improved phrasing Handle async requests and loading states with good UX Apply responsive, accessible design to AI interfaces The Project: AI Writing Assistant We’ll build a smart textarea component. Users write a sentence, and the AI suggests a more professional or grammatically improved version. Stack Vue 3 with and Composition API Axios for API requests OpenAI API (you’ll need an API key) Tailwind CSS (optional for styling) Setup the Project Install Vue 3 via Vite: npm create vite@latest ai-text-suggester -- --template vue cd ai-text-suggester npm install npm install axios Add your OpenAI key to an .env file: VITE_OPENAI_KEY=your_api_key_here Build the AITextSuggester.vue Component {{ loading ? 'Generating...' : 'Improve Writing' }} Suggested: {{ suggestion }} import { ref } from 'vue' import axios from 'axios' const inputText = ref('') const suggestion = ref('') const loading = ref(false) const generateSuggestion = async () => { if (!inputText.value.trim()) return loading.value = true suggestion.value = '' try { const response = await axios.post( 'https://api.openai.com/v1/chat/completions', { model: 'gpt-3.5-turbo', messages: [ { role: 'system', content: 'You are a helpful writing assistant that improves clarity and grammar.' }, { role: 'user', content: `Improve this sentence: ${inputText.value}` } ] }, { headers: { Authorization: `Bearer ${import.meta.env.VITE_OPENAI_KEY}` } } ) suggestion.value = response.data.choices[0].message.content.trim() } catch (error) { console.error('Error generating suggestion:', error) suggestion.value = 'Something went wrong. Please try again.' } finally { loading.value = false } } Accessibility and UX Considerations Disabled button state ensures user can’t spam the API. Loading text gives feedback on current action. Clear separation of input and response aids comprehension.
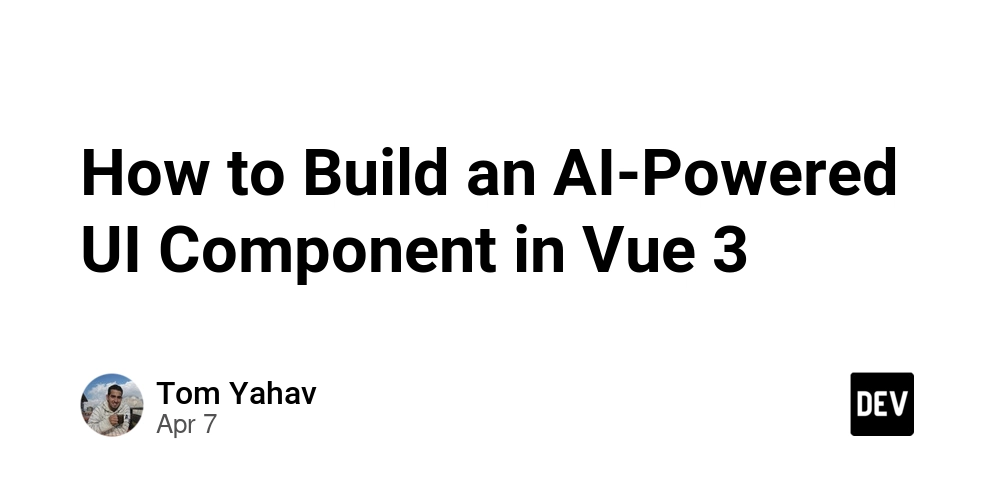
Introduction & Context
The rise of AI APIs like OpenAI's GPT and Hugging Face Transformers has opened a new frontier for frontend developers. Today, you can enrich your Vue apps with AI-driven features—without being a machine learning expert.
This tutorial walks you through building an AI-powered UI component in Vue 3. You'll learn how to connect to an AI API, handle user input, display responses, and create a polished, interactive user experience.
Whether you're working on chatbots, intelligent writing assistants, or form enhancers, this post is for you.
Goals and What You’ll Learn
By the end of this article, you’ll learn how to:
- Integrate OpenAI’s API into a Vue 3 project
- Build a smart text component that suggests improved phrasing
- Handle async requests and loading states with good UX
- Apply responsive, accessible design to AI interfaces
The Project: AI Writing Assistant
We’ll build a smart textarea component. Users write a sentence, and the AI suggests a more professional or grammatically improved version.
Stack
- Vue 3 with
and Composition API
- Axios for API requests
- OpenAI API (you’ll need an API key)
- Tailwind CSS (optional for styling)
Setup the Project
Install Vue 3 via Vite:
npm create vite@latest ai-text-suggester -- --template vue
cd ai-text-suggester
npm install
npm install axios
Add your OpenAI key to an .env
file:
VITE_OPENAI_KEY=your_api_key_here
Build the AITextSuggester.vue
Component
Suggested:
{{ suggestion }}
Accessibility and UX Considerations
- Disabled button state ensures user can’t spam the API.
- Loading text gives feedback on current action.
- Clear separation of input and response aids comprehension.