The Mechanics of SELECT FOR UPDATE in SQL
SELECT FOR UPDATE is a row-level locking mechanism in SQL used to lock the rows retrieved in a transaction. Its purpose is to prevent other transactions from modifying or acquiring locks on these rows, and it is commonly used in scenarios where data consistency must be ensured. In MySQL, the specific implementation of SELECT FOR UPDATE is closely tied to the storage engine (such as InnoDB). At its core, it uses row locks to lock the target rows. Core Features Type of Lock: SELECT FOR UPDATE places exclusive locks (X locks) on the rows retrieved by the query. Other transactions cannot modify these rows or place either shared or exclusive locks on them. Scope of Use: Must be used within a transaction (i.e., between BEGIN and COMMIT). Only effective when using a storage engine that supports transactions (such as InnoDB). Behavior: If the target rows are already locked by another transaction, the current transaction will enter a waiting state until the lock is released or a timeout occurs. Implementation Principle InnoDB Row Locking Mechanism The InnoDB storage engine implements row-level locking through indexes. SELECT FOR UPDATE locks all rows that meet the query condition. If the query does not use an index, it will degrade to a table lock, locking the entire table. Locking Process During query execution, InnoDB attempts to place an exclusive lock on each scanned row. If a row is already locked by another transaction, the current transaction waits until that lock is released. Types of Locks Row Lock: Index-based lock that only locks the retrieved rows. Gap Lock: Under the REPEATABLE READ isolation level, if a range query doesn’t hit any rows, a lock is placed on the gap between the range to prevent new rows from being inserted. Effect of Transaction Isolation Levels READ COMMITTED: Each query reads the latest data and only locks the rows returned by the current query. REPEATABLE READ: The query result is based on a transaction snapshot, and the locked range may include non-matching rows (due to gap locking). SERIALIZABLE: Locks all data within the query range. Execution Process The following illustrates the execution process of SELECT FOR UPDATE using InnoDB as an example: Start Transaction: Begin the transaction using BEGIN. Query and Lock: Execute SELECT ... FOR UPDATE to apply an exclusive lock (X lock) on records that meet the condition. Data Operations: Modify or read the locked data. Release Lock: Once the transaction is committed (COMMIT), the lock is released. If the transaction is rolled back (ROLLBACK), the lock is also released. Examples Basic Usage BEGIN; SELECT * FROM orders WHERE order_id = 1 FOR UPDATE; /* Locks the row with order_id = 1 */ UPDATE orders SET status = 'processed' WHERE order_id = 1; COMMIT; Multi-Transaction Contention Scenario Transaction A: BEGIN; SELECT * FROM orders WHERE order_id = 1 FOR UPDATE; -- Locks the row with order_id = 1 Transaction B (before Transaction A commits): BEGIN; SELECT * FROM orders WHERE order_id = 1 FOR UPDATE; -- Waits for Transaction A to release the lock Transaction B cannot acquire the lock until Transaction A either commits or rolls back. Behavior of Gap Locks Under the REPEATABLE READ isolation level, a range query might trigger a gap lock. For example: SELECT * FROM orders WHERE order_id BETWEEN 10 AND 20 FOR UPDATE; If the query returns no results, InnoDB will still place a gap lock on the range between 10 and 20, preventing other transactions from inserting new records within this range. Optimization and Considerations Use of Indexes: Queries that use indexes will apply row-level locks, avoiding degradation to table-level locks. If indexes are not used, the entire table may be locked, negatively affecting concurrency performance. Avoid Long Transactions: Holding locks for too long can cause other transactions to be blocked. It is recommended to minimize transaction duration. Deadlock Detection: InnoDB automatically detects deadlocks and rolls back one of the transactions. It is advisable to design transaction logic carefully to avoid deadlocks. Use According to Business Requirements: Use SELECT FOR UPDATE only when necessary to prevent data modification conflicts, to avoid unnecessary lock contention. Summary SELECT FOR UPDATE is a locking operation in MySQL that uses the row lock mechanism to prevent concurrent modification conflicts. Its implementation relies on transactions, isolation levels, and index optimization. When used appropriately, SELECT FOR UPDATE can effectively protect data consistency, but attention must be paid to performance overhead, avoiding lock contention and deadlocks. We are Leapcell, your top cho
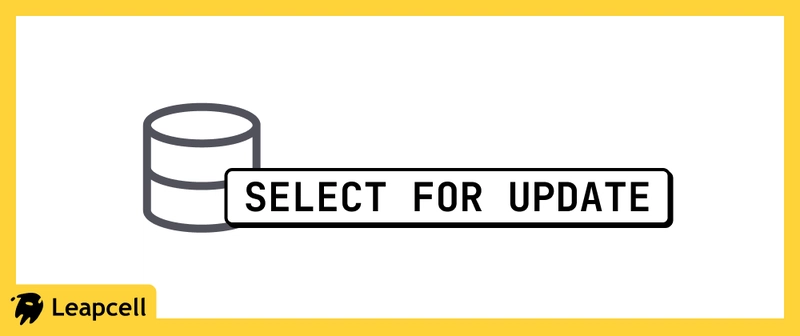
SELECT FOR UPDATE
is a row-level locking mechanism in SQL used to lock the rows retrieved in a transaction.
Its purpose is to prevent other transactions from modifying or acquiring locks on these rows, and it is commonly used in scenarios where data consistency must be ensured.
In MySQL, the specific implementation of SELECT FOR UPDATE
is closely tied to the storage engine (such as InnoDB). At its core, it uses row locks to lock the target rows.
Core Features
Type of Lock:
-
SELECT FOR UPDATE
places exclusive locks (X locks) on the rows retrieved by the query. - Other transactions cannot modify these rows or place either shared or exclusive locks on them.
Scope of Use:
- Must be used within a transaction (i.e., between
BEGIN
andCOMMIT
). - Only effective when using a storage engine that supports transactions (such as InnoDB).
Behavior:
- If the target rows are already locked by another transaction, the current transaction will enter a waiting state until the lock is released or a timeout occurs.
Implementation Principle
InnoDB Row Locking Mechanism
- The InnoDB storage engine implements row-level locking through indexes.
-
SELECT FOR UPDATE
locks all rows that meet the query condition. If the query does not use an index, it will degrade to a table lock, locking the entire table.
Locking Process
- During query execution, InnoDB attempts to place an exclusive lock on each scanned row.
- If a row is already locked by another transaction, the current transaction waits until that lock is released.
Types of Locks
- Row Lock: Index-based lock that only locks the retrieved rows.
-
Gap Lock: Under the
REPEATABLE READ
isolation level, if a range query doesn’t hit any rows, a lock is placed on the gap between the range to prevent new rows from being inserted.
Effect of Transaction Isolation Levels
- READ COMMITTED: Each query reads the latest data and only locks the rows returned by the current query.
- REPEATABLE READ: The query result is based on a transaction snapshot, and the locked range may include non-matching rows (due to gap locking).
- SERIALIZABLE: Locks all data within the query range.
Execution Process
The following illustrates the execution process of SELECT FOR UPDATE
using InnoDB as an example:
-
Start Transaction: Begin the transaction using
BEGIN
. -
Query and Lock: Execute
SELECT ... FOR UPDATE
to apply an exclusive lock (X lock) on records that meet the condition. - Data Operations: Modify or read the locked data.
-
Release Lock: Once the transaction is committed (
COMMIT
), the lock is released. If the transaction is rolled back (ROLLBACK
), the lock is also released.
Examples
Basic Usage
BEGIN;
SELECT * FROM orders WHERE order_id = 1 FOR UPDATE;
/* Locks the row with order_id = 1 */
UPDATE orders SET status = 'processed' WHERE order_id = 1;
COMMIT;
Multi-Transaction Contention Scenario
Transaction A:
BEGIN;
SELECT * FROM orders WHERE order_id = 1 FOR UPDATE;
-- Locks the row with order_id = 1
Transaction B (before Transaction A commits):
BEGIN;
SELECT * FROM orders WHERE order_id = 1 FOR UPDATE;
-- Waits for Transaction A to release the lock
Transaction B cannot acquire the lock until Transaction A either commits or rolls back.
Behavior of Gap Locks
Under the REPEATABLE READ
isolation level, a range query might trigger a gap lock. For example:
SELECT * FROM orders WHERE order_id BETWEEN 10 AND 20 FOR UPDATE;
If the query returns no results, InnoDB will still place a gap lock on the range between 10 and 20, preventing other transactions from inserting new records within this range.
Optimization and Considerations
Use of Indexes:
- Queries that use indexes will apply row-level locks, avoiding degradation to table-level locks.
- If indexes are not used, the entire table may be locked, negatively affecting concurrency performance.
Avoid Long Transactions:
- Holding locks for too long can cause other transactions to be blocked. It is recommended to minimize transaction duration.
Deadlock Detection:
- InnoDB automatically detects deadlocks and rolls back one of the transactions. It is advisable to design transaction logic carefully to avoid deadlocks.
Use According to Business Requirements:
- Use
SELECT FOR UPDATE
only when necessary to prevent data modification conflicts, to avoid unnecessary lock contention.
Summary
-
SELECT FOR UPDATE
is a locking operation in MySQL that uses the row lock mechanism to prevent concurrent modification conflicts. - Its implementation relies on transactions, isolation levels, and index optimization.
- When used appropriately,
SELECT FOR UPDATE
can effectively protect data consistency, but attention must be paid to performance overhead, avoiding lock contention and deadlocks.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ