How to Build an AI Image Caption Generator in Vue 3
Introduction & Context Images are everywhere—but they often lack proper descriptions. Whether for accessibility, SEO, or UX, adding meaningful captions is essential. But what if we could generate them automatically using AI? In this article, we’ll walk you through building a Vue 3 component that uses the Hugging Face Inference API to generate human-like captions for uploaded images. You’ll learn how to create a drag-and-drop uploader, handle image previews, and get smart captions with just a few lines of code. Perfect for frontend devs looking to bring intelligent features into visual interfaces! Goals and What You’ll Learn By the end of this tutorial, you’ll be able to: Build a drag-and-drop or file upload component Send images to an AI image captioning model Display the uploaded image preview and its AI-generated caption Handle loading and error states gracefully Enhance accessibility with alt-text or voiceover features Tech Stack Vue 3 + Axios for API calls Hugging Face Inference API (BLIP or similar image-to-text model) Tailwind CSS (optional for styling) Prerequisites Create a free account on Hugging Face. Generate an API token from your account settings. Store it in an .env file: VITE_HUGGINGFACE_API_KEY=your_token_here Building the Component: ImageCaptioner.vue AI Image Caption Generator {{ loading ? 'Generating...' : 'Generate Caption' }} Caption: {{ caption }} import { ref } from 'vue' import axios from 'axios' const imageUrl = ref(null) const imageBlob = ref(null) const caption = ref('') const loading = ref(false) const handleUpload = (e) => { const file = e.target.files[0] if (!file) return imageBlob.value = file imageUrl.value = URL.createObjectURL(file) caption.value = '' } const generateCaption = async () => { if (!imageBlob.value) return loading.value = true caption.value = '' const formData = new FormData() formData.append('file', imageBlob.value) try { const response = await axios.post( 'https://api-inference.huggingface.co/models/Salesforce/blip-image-captioning-base', imageBlob.value, { headers: { Authorization: `Bearer ${import.meta.env.VITE_HUGGINGFACE_API_KEY}`, 'Content-Type': 'application/octet-stream' } } ) caption.value = response.data[0]?.generated_text || 'No caption returned.' } catch (err) { console.error('Error:', err) caption.value = 'An error occurred. Try again.' } finally { loading.value = false } } Accessibility and UX Improvements Image Preview: Helps users confirm they uploaded the correct file. Disabled Button State: Prevents repeated submissions. Alt Text: Use the generated caption as an alt attribute for better accessibility.
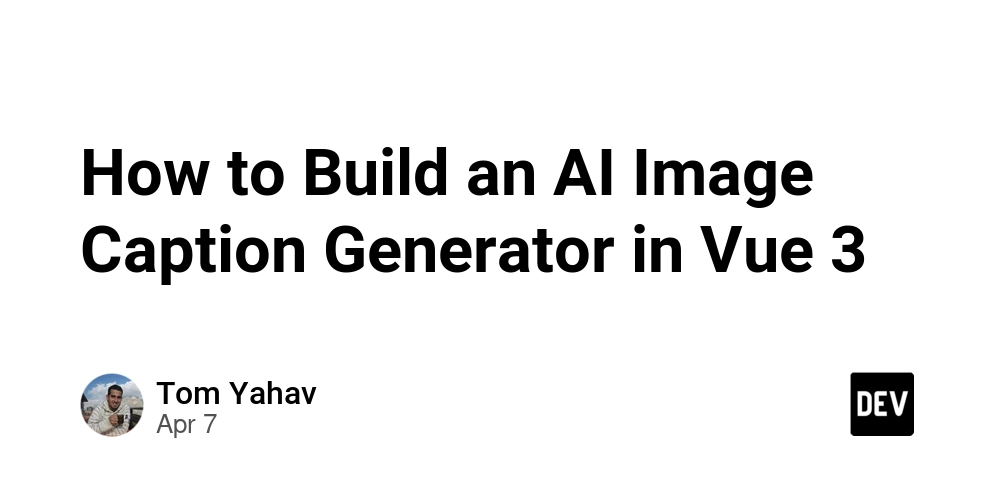
Introduction & Context
Images are everywhere—but they often lack proper descriptions. Whether for accessibility, SEO, or UX, adding meaningful captions is essential. But what if we could generate them automatically using AI?
In this article, we’ll walk you through building a Vue 3 component that uses the Hugging Face Inference API to generate human-like captions for uploaded images. You’ll learn how to create a drag-and-drop uploader, handle image previews, and get smart captions with just a few lines of code.
Perfect for frontend devs looking to bring intelligent features into visual interfaces!
Goals and What You’ll Learn
- By the end of this tutorial, you’ll be able to:
- Build a drag-and-drop or file upload component
- Send images to an AI image captioning model
- Display the uploaded image preview and its AI-generated caption
- Handle loading and error states gracefully
- Enhance accessibility with alt-text or voiceover features
Tech Stack
- Vue 3 +
- Axios for API calls
- Hugging Face Inference API (BLIP or similar image-to-text model)
- Tailwind CSS (optional for styling)
Prerequisites
- Create a free account on Hugging Face.
- Generate an API token from your account settings.
Store it in an .env
file:
VITE_HUGGINGFACE_API_KEY=your_token_here
Building the Component: ImageCaptioner.vue
AI Image Caption Generator
Caption:
{{ caption }}
Accessibility and UX Improvements
Image Preview: Helps users confirm they uploaded the correct file.
Disabled Button State: Prevents repeated submissions.
Alt Text: Use the generated caption as an alt attribute for better accessibility.