How to Copy Matching Directories in Bash Without a Script?
In this article, we'll discuss how to efficiently copy files from one directory to another while ensuring you only deal with specific matching patterns. Specifically, if you have a directory structure and want to copy a file named goodfile from source_dir to corresponding subdirectories in dest_dir with names matching the pattern ??-??, you can achieve this using simple bash commands. Let's dive right in! Understanding the Directory Structure The task at hand is to copy files based on a particular naming convention. You have the following directory structure: Source Directory: /tmp/source_dir/ aa-aa/ bb-bb/ cc-cc/ morejunk/ somejunk/ Destination Directory: /tmp/dest_dir/ aa-aa/ bb-bb/ blah/ blahblah/ In this example, you're trying to copy the goodfile from source_dir to matching directories in dest_dir that follow the format ??-??, like aa-aa and bb-bb. Why Did the Previous Commands Fail? When you attempted the commands using xargs, the main issues were related to how the substitutions were made within the command. In bash, {} is often used as a placeholder for the output from the previous command. However, you need to ensure it's correctly interpreted in the context of file paths. Step-by-Step Solution Let's rewrite the command to accomplish the task effectively. Using a combination of a loop and proper command substitution will yield better results. Follow the steps below: Step 1: Change to the Destination Directory First, we'll switch to the dest_dir. cd /tmp/dest_dir Step 2: Using a Loop to Copy Files Now, we can use a for loop to iterate over directories that match the required pattern. This way, we can construct the correct paths for copying goodfile. for dir in ??-??; do if [ -d "$dir" ]; then cp "/tmp/source_dir/$dir/goodfile" "$dir/" fi done In this loop: ??-?? identifies the directories in dest_dir matching the specified pattern. The check if [ -d "$dir" ]; ensures that we only attempt to copy from directories. The cp command transfers goodfile from the corresponding source directory to the matched destination. Step 3: Verifying the Operation To ensure that files have been copied correctly, you can list the contents of your destination directory using: ls /tmp/dest_dir/??-??/ This command will help confirm the presence of goodfile in the directories matching the specified pattern. Frequently Asked Questions Can I use this method for other types of files? Yes, you can adjust the loop condition and the file names accordingly to copy different files based on specified criteria. What if I want to copy files recursively? For recursive copying, consider adding the -r option to the cp command: pc -r ... Is there a way to handle errors during copying? To handle potential errors, you can add error checking after the cp command: if cp ...; then echo "File copied successfully" else echo "Failed to copy file" fi This strategy will help you manage scenarios where files may not exist or other copy issues arise. Conclusion Copying files between directories in bash based on matching patterns can be done efficiently with just a few commands. By using a loop to traverse your destination directory and copy the desired files from the source, you avoid the potential pitfalls of using xargs in this situation. This gives you a flexible and effective approach to manage your directories and files!
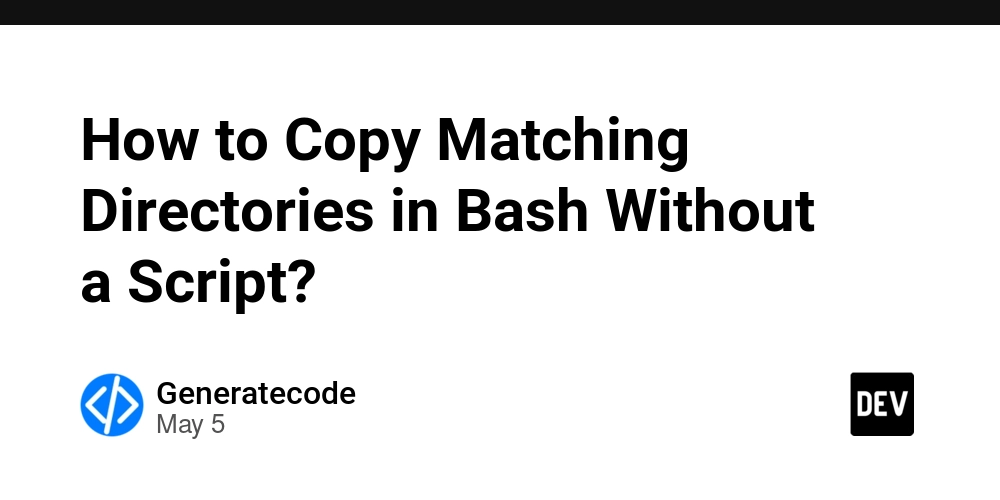
In this article, we'll discuss how to efficiently copy files from one directory to another while ensuring you only deal with specific matching patterns. Specifically, if you have a directory structure and want to copy a file named goodfile
from source_dir
to corresponding subdirectories in dest_dir
with names matching the pattern ??-??
, you can achieve this using simple bash commands. Let's dive right in!
Understanding the Directory Structure
The task at hand is to copy files based on a particular naming convention. You have the following directory structure:
-
Source Directory:
/tmp/source_dir/
- aa-aa/
- bb-bb/
- cc-cc/
- morejunk/
- somejunk/
-
Destination Directory:
/tmp/dest_dir/
- aa-aa/
- bb-bb/
- blah/
- blahblah/
In this example, you're trying to copy the goodfile
from source_dir
to matching directories in dest_dir
that follow the format ??-??
, like aa-aa
and bb-bb
.
Why Did the Previous Commands Fail?
When you attempted the commands using xargs
, the main issues were related to how the substitutions were made within the command. In bash, {}
is often used as a placeholder for the output from the previous command. However, you need to ensure it's correctly interpreted in the context of file paths.
Step-by-Step Solution
Let's rewrite the command to accomplish the task effectively. Using a combination of a loop and proper command substitution will yield better results. Follow the steps below:
Step 1: Change to the Destination Directory
First, we'll switch to the dest_dir
.
cd /tmp/dest_dir
Step 2: Using a Loop to Copy Files
Now, we can use a for
loop to iterate over directories that match the required pattern. This way, we can construct the correct paths for copying goodfile
.
for dir in ??-??; do
if [ -d "$dir" ]; then
cp "/tmp/source_dir/$dir/goodfile" "$dir/"
fi
done
In this loop:
-
??-??
identifies the directories indest_dir
matching the specified pattern. - The check
if [ -d "$dir" ];
ensures that we only attempt to copy from directories. - The
cp
command transfersgoodfile
from the corresponding source directory to the matched destination.
Step 3: Verifying the Operation
To ensure that files have been copied correctly, you can list the contents of your destination directory using:
ls /tmp/dest_dir/??-??/
This command will help confirm the presence of goodfile
in the directories matching the specified pattern.
Frequently Asked Questions
Can I use this method for other types of files?
Yes, you can adjust the loop condition and the file names accordingly to copy different files based on specified criteria.
What if I want to copy files recursively?
For recursive copying, consider adding the -r
option to the cp
command:
pc -r ...
Is there a way to handle errors during copying?
To handle potential errors, you can add error checking after the cp
command:
if cp ...; then
echo "File copied successfully"
else
echo "Failed to copy file"
fi
This strategy will help you manage scenarios where files may not exist or other copy issues arise.
Conclusion
Copying files between directories in bash based on matching patterns can be done efficiently with just a few commands. By using a loop to traverse your destination directory and copy the desired files from the source, you avoid the potential pitfalls of using xargs
in this situation. This gives you a flexible and effective approach to manage your directories and files!