How to Build Responsive Websites with HTML and CSS
How to Build Responsive Websites with HTML and CSS In today's digital age, having a responsive website is no longer optional—it's essential. With users accessing the web from smartphones, tablets, laptops, and desktops, your website must adapt seamlessly to any screen size. In this guide, we'll explore how to build responsive websites using HTML and CSS, covering key concepts like media queries, flexible layouts, and relative units. And if you're looking to monetize your web development skills, check out MillionFormula for proven strategies to make money with your coding expertise. Why Responsive Design Matters Before diving into the code, let's understand why responsive design is crucial: Improved User Experience (UX): A responsive site ensures smooth navigation across all devices. Better SEO Rankings: Google prioritizes mobile-friendly websites in search results. Cost-Effective: Maintaining a single responsive site is cheaper than separate desktop and mobile versions. 1. Setting Up a Basic HTML Structure Every responsive website starts with a solid HTML foundation. Use semantic HTML5 elements for better accessibility and SEO. html Copy Download Run DOCTYPE html> Responsive Website My Responsive Site Home About Contact Welcome! This is a responsive website built with HTML and CSS. © 2024 My Site Key Points: ensures proper scaling on mobile devices. Semantic tags (, , , ) improve SEO and accessibility. 2. Flexible Layouts with CSS A responsive layout should adapt to different screen sizes. Use CSS Flexbox and CSS Grid for dynamic designs. Flexbox Example: css Copy Download .container { display: flex; flex-wrap: wrap; gap: 1rem; } .item { flex: 1 1 200px; /* Flex-grow, flex-shrink, flex-basis */ } CSS Grid Example: css Copy Download .grid-container { display: grid; grid-template-columns: repeat(auto-fit, minmax(250px, 1fr)); gap: 1rem; } Key Points: flex-wrap: wrap allows items to wrap on smaller screens. auto-fit and minmax() in Grid ensure columns adjust dynamically. 3. Using Relative Units (em, rem, %, vw, vh) Absolute units like px can break responsiveness. Instead, use: rem (root-relative) for scalable typography. % for fluid container widths. vw/vh (viewport units) for full-screen elements. css Copy Download html { font-size: 16px; /* Base for rem */ } h1 { font-size: 2rem; /* 32px */ } .container { width: 90%; max-width: 1200px; margin: 0 auto; } 4. Media Queries for Breakpoints Media queries allow CSS adjustments based on screen width. Common breakpoints: css Copy Download /* Mobile-first approach (default styles for small screens) */ body { font-size: 1rem; } /* Tablet (768px and up) */ @media (min-width: 768px) { body { font-size: 1.1rem; } } /* Desktop (1024px and up) */ @media (min-width: 1024px) { body { font-size: 1.2rem; } } Key Points: Mobile-first design ensures better performance. Use min-width to progressively enhance larger screens. 5. Responsive Images Images should scale without distortion. Use: html Copy Download Run Or with srcset for different resolutions: html Copy Download Run 6. Testing Your Responsive Design Use: Chrome DevTools (Device Mode) Responsive Design Checker Real devices for accurate testing Conclusion Building responsive websites with HTML and CSS is essential for modern web development. By using Flexbox, Grid, media queries, and relative units, you can create layouts that work on any device. Want to turn your web dev skills into income? Explore MillionFormula for expert strategies on monetizing your coding expertise. Further Reading: MDN Web Docs: Responsive Design CSS-Tricks: A Complete Guide to Flexbox Google’s Mobile-Friendly Test Happy coding!
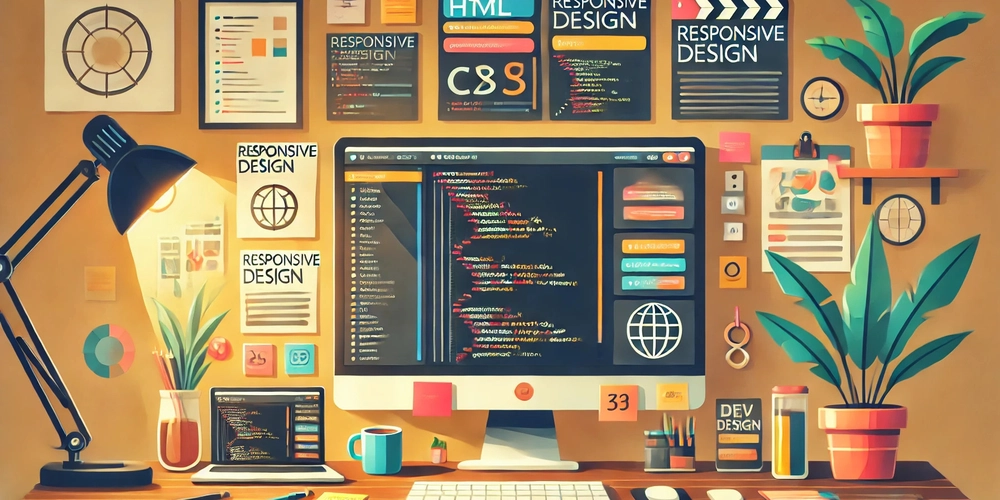
How to Build Responsive Websites with HTML and CSS
In today's digital age, having a responsive website is no longer optional—it's essential. With users accessing the web from smartphones, tablets, laptops, and desktops, your website must adapt seamlessly to any screen size. In this guide, we'll explore how to build responsive websites using HTML and CSS, covering key concepts like media queries, flexible layouts, and relative units.
And if you're looking to monetize your web development skills, check out MillionFormula for proven strategies to make money with your coding expertise.
Why Responsive Design Matters
Before diving into the code, let's understand why responsive design is crucial:
-
Improved User Experience (UX): A responsive site ensures smooth navigation across all devices.
-
Better SEO Rankings: Google prioritizes mobile-friendly websites in search results.
-
Cost-Effective: Maintaining a single responsive site is cheaper than separate desktop and mobile versions.
1. Setting Up a Basic HTML Structure
Every responsive website starts with a solid HTML foundation. Use semantic HTML5 elements for better accessibility and SEO.
html
Copy
Download
Run
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Websitetitle>
<link rel="stylesheet" href="styles.css">
head>
<body>
<header>
<h1>My Responsive Siteh1>
<nav>
<ul>
<li><a href="#">Homea>li>
<li><a href="#">Abouta>li>
<li><a href="#">Contacta>li>
ul>
nav>
header>
<main>
<section>
<h2>Welcome!h2>
<p>This is a responsive website built with HTML and CSS.p>
section>
main>
<footer>
<p>© 2024 My Sitep>
footer>
body>
html>
Key Points:
-
ensures proper scaling on mobile devices.
-
Semantic tags (
,,
,
) improve SEO and accessibility.
2. Flexible Layouts with CSS
A responsive layout should adapt to different screen sizes. Use CSS Flexbox and CSS Grid for dynamic designs.
Flexbox Example:
css
Copy
Download
.container { display: flex; flex-wrap: wrap; gap: 1rem; } .item { flex: 1 1 200px; /* Flex-grow, flex-shrink, flex-basis */ }
CSS Grid Example:
css
Copy
Download
.grid-container { display: grid; grid-template-columns: repeat(auto-fit, minmax(250px, 1fr)); gap: 1rem; }
Key Points:
-
flex-wrap: wrap
allows items to wrap on smaller screens. -
auto-fit
andminmax()
in Grid ensure columns adjust dynamically.
3. Using Relative Units (em, rem, %, vw, vh)
Absolute units like px
can break responsiveness. Instead, use:
-
rem
(root-relative) for scalable typography. -
%
for fluid container widths. -
vw/vh
(viewport units) for full-screen elements.
css
Copy
Download
html { font-size: 16px; /* Base for rem */ } h1 { font-size: 2rem; /* 32px */ } .container { width: 90%; max-width: 1200px; margin: 0 auto; }
4. Media Queries for Breakpoints
Media queries allow CSS adjustments based on screen width. Common breakpoints:
css
Copy
Download
/* Mobile-first approach (default styles for small screens) */ body { font-size: 1rem; } /* Tablet (768px and up) */ @media (min-width: 768px) { body { font-size: 1.1rem; } } /* Desktop (1024px and up) */ @media (min-width: 1024px) { body { font-size: 1.2rem; } }
Key Points:
-
Mobile-first design ensures better performance.
-
Use
min-width
to progressively enhance larger screens.
5. Responsive Images
Images should scale without distortion. Use:
html
Copy
Download
Run
<img
src="image.jpg"
alt="Responsive Image"
style="max-width: 100%; height: auto;"
>
Or with srcset
for different resolutions:
html
Copy
Download
Run
<img
srcset="small.jpg 500w, medium.jpg 1000w, large.jpg 1500w"
sizes="(max-width: 600px) 500px, (max-width: 1200px) 1000px, 1500px"
src="medium.jpg"
alt="Responsive Image"
>
6. Testing Your Responsive Design
Use:
-
Chrome DevTools (Device Mode)
-
Real devices for accurate testing
Conclusion
Building responsive websites with HTML and CSS is essential for modern web development. By using Flexbox, Grid, media queries, and relative units, you can create layouts that work on any device.
Want to turn your web dev skills into income? Explore MillionFormula for expert strategies on monetizing your coding expertise.
Further Reading:
Happy coding!