Go - (5) Errors & Loops
Error Handling Languages like JS use try catch blocks for error handling. But in Go, it is a bit different. Check the example below. func getUser() (string, error) { // ... some code here return username, err } func main() error { name, err := getUser() if err != nil { // if err is not nil, return the error return err } // if no errors, print the username fmt.Printf("User name: %s", name) } Error handling in Go is more convenient because it doesn't need nested error handling like in try-catch blocks. Also, we can see which functions return error by looking at the function signature. Go has a package named errors. It has methods like New() to initialize errors. import ( "errors" ) func getUser() (string, error) { // ... some code here return username, errors.New("couldn't get the username") // a new err is returned } Loops The syntax of Go loops is very similar to loops in JS. The only difference is that we don't use parentheses. An example of a traditional for loop is given below. for i := 0; 0 threshold { return i } }
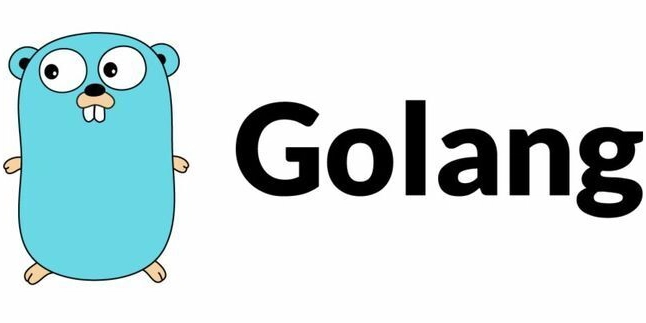
Error Handling
- Languages like JS use
try catch
blocks for error handling. - But in Go, it is a bit different.
Check the example below.
func getUser() (string, error) {
// ... some code here
return username, err
}
func main() error {
name, err := getUser()
if err != nil {
// if err is not nil, return the error
return err
}
// if no errors, print the username
fmt.Printf("User name: %s", name)
}
- Error handling in Go is more convenient because it doesn't need nested error handling like in try-catch blocks.
- Also, we can see which functions return
error
by looking at the function signature. - Go has a package named
errors
. It has methods likeNew()
to initialize errors.
import (
"errors"
)
func getUser() (string, error) {
// ... some code here
return username, errors.New("couldn't get the username") // a new err is returned
}
Loops
The syntax of Go loops is very similar to loops in JS. The only difference is that we don't use parentheses.
An example of a traditional for loop is given below.
for i := 0; 0 < 10; i++ {
fmt.Print(i)
}
I Go loops, the condition in the loop is optional. If you check the example below, it goes out of the loop considering the conditional block.
totalCost := 0.0
threshold := 5.0
for i := 0; ; i++ {
totalCost += 1.0 + (0.01 * float64(i))
if totalCost > threshold {
return i
}
}